Next-Gen Coders: Lists
Learn about lists in python.
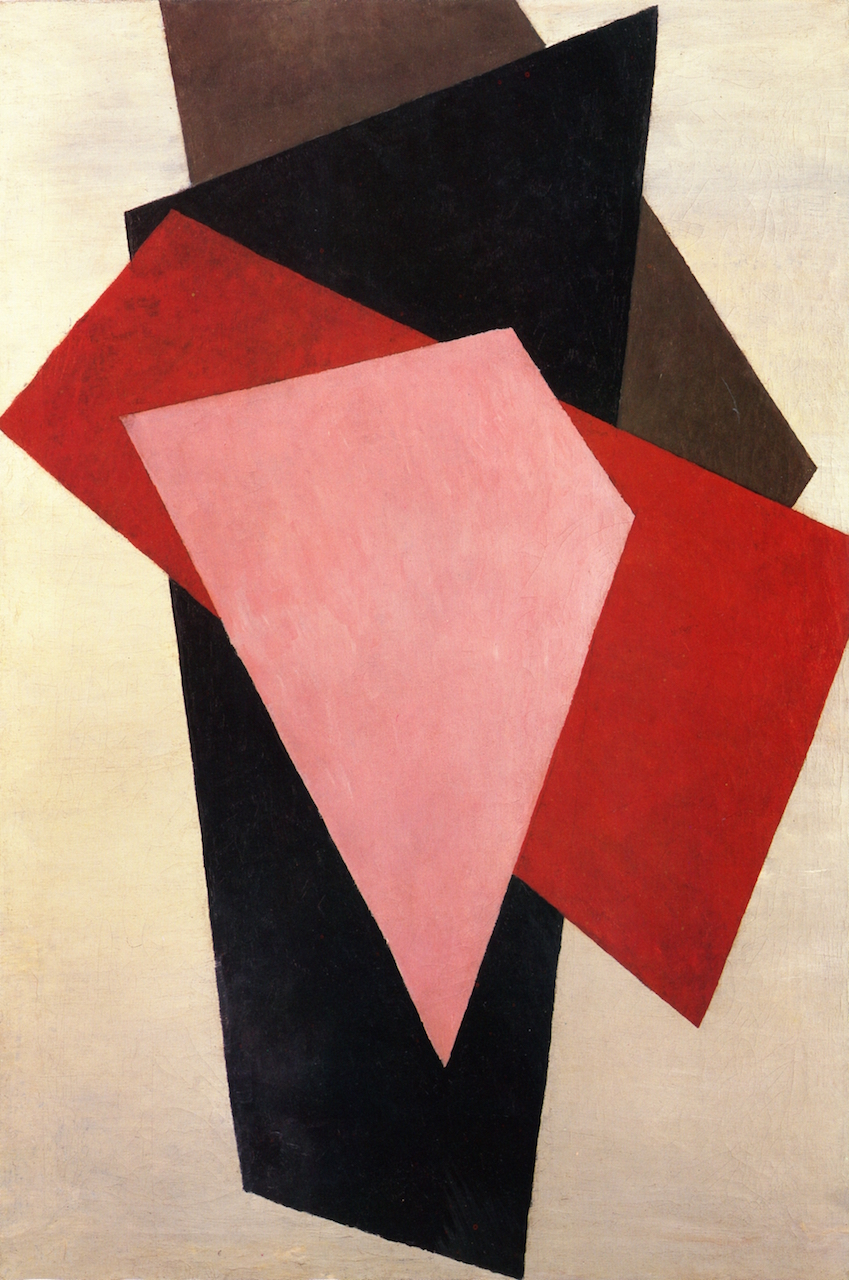
Making lists
So far, when we’ve saved been saving one value to one variable. So, we might save how much something costs to a variable called price
, or we might save the title of a book to a variable called title
.
But what if we want to group some values together? I might want to save all the names of students to a variable called classroom
. I also might want to save a list of words and definitions to a variable called vocabulary
. Does Python have some data types that can do these things? Yes! They’re called lists and dictionaries. In this lesson, we’re going to start learning about lists.
What you need to know…
This chapter is going to use loops quite a bit, so make sure that you’re familiar with them before we move on. If you need a refresher, check out the loops video.
Notes for the mentor
In this lesson, we’re going to start going over more complex data types. Even though lists and dictionaries are more complex than strings and integers, I find most students grasp the concept pretty quickly.
The harder part for many students is understanding indexes. Counting from zero confuses many new programmers, especially since there’s not many pat answers as to why we don’t start at one like we do everywhere else. I usually tell students that we start at zero because, at one point, it was the best option. Most students accept this, because, well, they’re used to arbitrary answers. After all, “because I said so,” is something most students have heard more than once!
We’re also going to be using methods in this lesson. Some students have trouble with the format and will try to do something like this:
my_list
=
[
1
,
2
,
3
]
my_list
append
(
5
)
Just remind them that they need a period between the variable name and the method we’re using. Don’t dive too deeply into what, exactly, a method is. We’ll be going over that in our OOP chapter.
Lists
We’re going to go over two new data types in this lesson, starting with lists.
Lists in Python are just like lists in real life! People make lists all the time: shopping lists, directions to a place, steps to create something. Lists are simply a collection of values, saved to one variable.
In Python, the values in a list are contained in a pair of square brackets ([ ]
). You’ll find these brackets at the end of the first row of letters on your keyboard. Let’s make a few lists.
my_nums
=
[
1
,
2
,
3
,
4
]
animals
=
[
"beta fish"
,
"dog"
,
"cat"
,
"llama"
]
(
my_nums
)
(
animals
)
Note that each value is separated by a comma, and all the values are inside of the pair of square brackets. If you use anything other than square brackets, or if you forget the commas, your program may throw an error or work strangely.
What’s an index?
Every item in your list has a place in line. That place is called its index. We use the index to get that item out of the list or change it.
Indexes start at zero. The first item in the list has an index of zero, the second item has an index of one, and so on.
List
:
[
1
,
2
,
3
,
4
]
^
^
^
^
Index
:
0
1
2
3
To find out what item is stored at a certain index, you put the index in brackets after the variable name. It looks like this:
colors
=
[
"red"
,
"yellow"
,
"blue"
]
(
colors
[
0
])
colors
=
[
"red"
,
"yellow"
,
"blue"
]
(
colors
[
1
])
You can also use the index to change what’s been stored at a certain index. Here, we’re going to switch “blue” for “turquoise”.
colors
=
[
"red"
,
"yellow"
,
"blue"
]
colors
[
2
]
=
"turquoise"
(
colors
)
Now, instead of holding blue, the last item in colors
is turquoise.
Quiz
Given this list:
['apple', 'pear', 'mango', 'grape', 'papaya']
-
What’s the index of “mango”?
-
What’s in the one index?
-
What’s the index of “apple”?
Addding items to a list
We can also add items to a list. This is a good thing, too, since a list isn’t much good if we can’t add to it!
To add an item to a list, we use append()
. append()
is tacked onto the end of the list we want to add to, and we put the item we want to add in the parenthesis. It looks like this:
colors
=
[
"red"
,
"yellow"
,
"blue"
]
colors
.
append
(
"violet"
)
colors
When you use append()
, the item you want to add gets added to the end of the list. What if you wanted to insert an item into a list, though? Let’s say we want to add “orange” to our list, but we want it to go in its proper place: between “red” and “yellow”. To do that, we use insert()
.
insert()
works almost like append()
, but it takes two values:
-
The index where you want to insert the item
-
The item you want to insert
Using insert()
looks like this:
mylist
.
insert
(
index
,
new_value
)
In order to use insert()
, you have to figure out where you want to insert your item. Here’s our list of colors:
[
'red'
,
'yellow'
,
'blue'
]
We want “orange” to be after “red”. “red” is at index zero, so “orange” should be at index one.
Now that we’ve sorted out where we want to put orange, we can use insert()
to update our list:
colors
=
[
"red"
,
"yellow"
,
"blue"
]
colors
.
insert
(
1
,
"orange"
)
colors
Now, “orange” is between “red” and “yellow”, right where it should be!
Removing values from a list
Sometimes, you want to remove an item from a list completely. Maybe you’re done with that task, or you realize you added an item one too many times. To remove an item from a list, we use pop()
.
To use pop()
, we need to know exactly what item we want Python to remove from our list. In order to be super crystal clear about what we want to do, Python needs the index of the item we want to trash. Using pop()
looks like this:
colors
=
[
"red"
,
"yellow"
,
"blue"
,
"apple"
]
colors
.
pop
(
3
)
colors
Above, I accidentally added something that wasn’t a color (“apple”), so I removed it by using pop()
and its index (which was three).
Quiz
Look at the following code, and try to figure out what the list will look like after it’s run.
mylist
=
[
2
,
1
,
8
,
7
]
mylist
.
append
(
5
)
mylist
.
pop
(
1
)
mylist
.
insert
(
2
,
6
)
Lists and loops!
Lists are already pretty great. Grouping items is incredibly useful, making it so that you have fewer variables to keep track of as you code. Where they really shine, though, is when you pair them with for
loops.
In the loops lesson, we learned about repeating code a block of code a certain number of times by using range()
. If we pair a for
loop with a list, though, we can run a block of code for every item in the list.
Using a list with a for
loop looks like this:
for
item
in
my_list
:
code
code
code
Python starts with the first item in the list, runs the code in the code block. Once that code is done, Python does the same thing with the second item in the list, and so on, until it runs out of items.
Let’s use a list with a for
loop to see how this works. We’re going to create a list of animals, then talk about how much we like them.
animals
=
[
'dog'
,
'cat'
,
'pig'
,
'goose'
,
'emu'
]
for
animal
in
animals
:
(
"I love my"
,
animal
)
Run the code, and watch what’s printed out. Every time the for
loop runs, Python takes one of the animals and stores it into the loop variable animal
, then runs the code in the block. Once that code is run, Python moves on to the next animal, and so on.
Quiz
How many iterations is this for
loop going to go through?
nums
=
[
1
,
5
,
2
,
7
]
for
num
in
nums
:
(
"*"
*
num
)