Chapter 1. Introduction to MVC
MVC is an architecture pattern that stands for Model-View-Controller. My definition of MVC is summarized as follows:
- The model manages the data for the application. Each model commonly represents one or more tables within a database.
- The view contains the visual representation of the application. In websites, this is commonly achieved with HTML, CSS, and JavaScript.
- The controller is the middleman between the model and the view. A typical controller would request data from the model and pass it to the view for use in displaying the data. When saving data, it would be the opposite. It would receive data from the view and pass it to the model to save it.
ASP.NET MVC 5 is a framework that implements the Model-View-Controller (MVC) architecture pattern.
The term MVC will be mentioned repeatedly throughout this book. In most scenarios, I will be referring to the MVC framework that implements the MVC pattern.
Creating Your First Project
Visual Studio offers a variety of templates that help you start your project. This book will focus on two specific templates: MVC and Web API.
The MVC template allows the creation of web applications that use the Model-View-Controller architecture pattern. This will be discussed in more detail throughout this book.
The Web API template allows for the creation of RESTful web applications. REST is another type of software architecture pattern that is commonly used for creating APIs or client-server applications. Web API is easily integrated into the MVC architecture pattern, which will allow reuse between your MVC and Web API projects, as will be demonstrated later in this book.
For this first example, I will focus on the MVC template. From the Visual Studio Start Page or from the File Menu, select the New Project option. As you can see, there are many different types of projects that you can create. Under the Templates menu, expand the Visual C# menu. From here, select the Web option. In my default installation, I have only one option of an ASP.NET Web Application. Select this and enter a project name in the space provided. I have chosen BootstrapIntroduction.
Once you have selected a project name, click the OK button. You are now presented with several different Web templates. Select the MVC template. Once the MVC template is selected, you will notice additional options to include Web API and to include a Unit Test project. For this example, I will leave them unselected.
With the MVC template, there are also four different Authentication options:
- No Authentication
- All pages of your website will be publicly available.
- Individual User Accounts
- With this option, your web application will allow users to register and log in by creating their own username and password. It also provides several different Social Login options, including Facebook, Twitter, Google, and Microsoft Account. Any of the various scenarios will store the information in the membership database that is automatically created.
- Organizational Accounts
- With this option, your web application will integrate with Active Directory, Microsoft Azure Active Directory, or Office 365 to secure pages within your application.
- Windows Authentication
- With this option, your web application is secured using the Windows Authentication IIS module. This is common for intranet applications where all of the user accounts exist on the web server hosting the application.
For the purposes of this example, I have changed it to No Authentication, as shown in Figure 1-1.
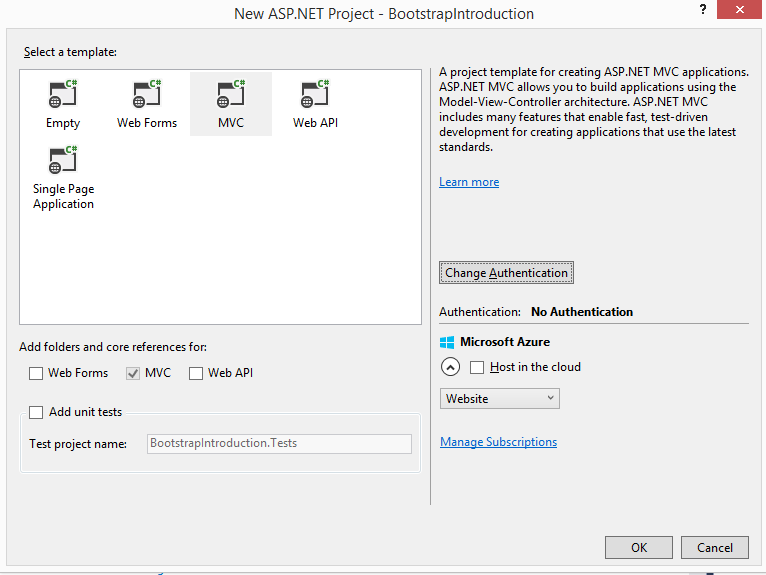
Figure 1-1. Project creation
When you are ready, click OK to complete the project creation. Depending on the speed of your computer, this may take one or two minutes while the project is being configured and preloaded with many useful files and folders to help you get started.
Once completed, you will be able to select Start Debugging from the Debug menu. This will launch your new web application in your default web browser.
When you look at the folders, you will notice that each role in MVC has its own folder. The Views folder commonly contains a subfolder that matches the Controller name because it typically contains more than one view to allow easy organization of your files.
If you expand the Views folder, you will see two subfolders: Home and Shared. The Home folder matches the HomeController
that exists in the Controllers folder. The Shared folder is used for views that contain common code between other views.
This includes layouts or partial views. Layouts are special views that contain the reusable view on each page. A partial view is a reusable view that contains a subset of data that can be included in one or more pages.
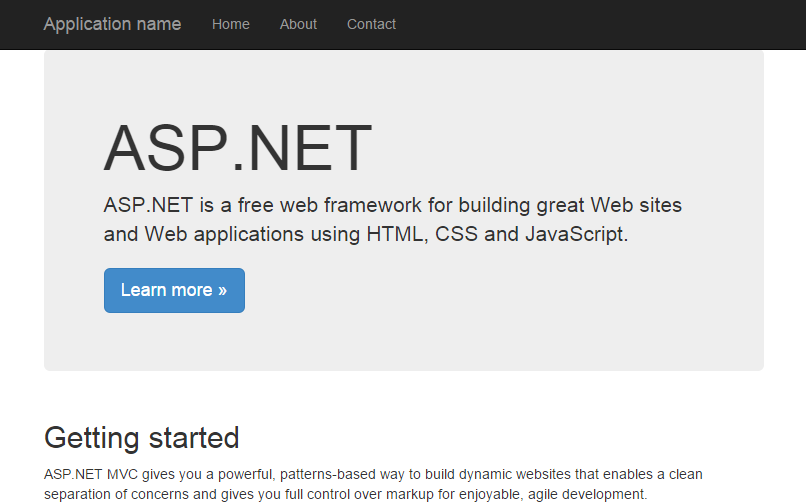
Figure 1-2. The default website
Figure 1-2 is a screenshot of the default website that is created with a new MVC project. The menu contains three links: Home, About, and Contact. Let’s keep those in mind as we begin exploring the code.
Examining the HomeController
Let’s start by looking at the controller. Under the Controllers folder is a file called HomeController.cs, and it should look like Example 1-1.
Example 1-1. HomeController.cs
using
System
;
using
System.Collections.Generic
;
using
System.Linq
;
using
System.Web
;
using
System.Web.Mvc
;
namespace
BootstrapIntroduction.Controllers
{
public
class
HomeController
:
Controller
{
public
ActionResult
Index
()
{
return
View
();
}
public
ActionResult
About
()
{
ViewBag
.
Message
=
"Your application description page."
;
return
View
();
}
public
ActionResult
Contact
()
{
ViewBag
.
Message
=
"Your contact page."
;
return
View
();
}
}
}
The HomeController
is a class that contains three methods: Index
, About
, and Contact
. In MVC terms, these are commonly referred to as actions. An action named Index
is usually the main entry point for a controller. Notice how each action matches the names of the links that were created with the exception of Home
, because that refers to the Index
action.
All controllers in an MVC application will extend the base Controller
class. Each method in the class returns a type called ActionResult
. In most scenarios, all of your actions will return this. We will explore other return types in future examples.
The About
and Contact
actions also introduce the ViewBag
property of the Controller
class. This property allows you to dynamically pass data to your view. Example 1-2 will demonstrate how it is used within the view.
The ViewBag
The ViewBag
property allows you to share data between your controllers and your views. This variable is defined as a dynamic type and contains no predefined properties, which allows you to specify any name for your property and have it contain any type of data.
Finally, each action is returned with a call to the View
function. This method exists in the Controller
class that all controllers extend. It loads the view and executes the Razor code contained within your .cshtml file. In Example 1-1, no parameters are passed into the function, which means that, by default, MVC will look for a view with the same name as the function.
Examining the Views
When you expand the Views folder, there is a subfolder called Home. This contains three files: About.cshtml, Contact.cshtml, and Index.cshtml. Each file is named after its action name in the controller. The extension .cshtml stands for C# HTML. These views allow for Razor syntax, which is a combination of HTML mixed with C#. This provides the ability to implement common coding techniques like conditional statements, loops, and outputting of dynamic data (such as the ViewBag
property previously mentioned).
Example 1-2 is the default About page that is created with the project. Elements that use Razor begin with the @ symbol. When it is combined with a curly brace ({), this allows for multiline C# code. In this example, it is setting the page title in the ViewBag
property to "About"
. The Title
property on the ViewBag
is commonly used in the shared layout to set the title of the page that your browser shows. This example also displays it within an h2
tag.
Example 1-2. About.cshtml
@{ ViewBag.Title = "About"; }<h2>
@ViewBag.Title.</h2>
<h3>
@ViewBag.Message</h3>
<p>
Use this area to provide additional information.</p>
In addition to the Title
property, the Message
property in the ViewBag
is shown in an h3
tag. As you recall, this was set in the controller action for the About
and Contact
methods.
This is a great example of how Razor is interjected with standard HTML to produce dynamic content when rendered to a browser.
You may notice that when you browse the About page there is a lot more HTML than just these few elements. This is accomplished with a shared layout. By default, all views are placed within a default template, which is under the other folder within Views called Shared. If you expand this folder, you will see the _Layout.cshtml file, as shown in Example 1-3.
Example 1-3. _Layout.cshtml
<!DOCTYPE html>
<html>
<head>
<meta
charset=
"utf-8"
/>
<meta
name=
"viewport"
content=
"width=device-width, initial-scale=1.0"
>
<title>
@ViewBag.Title - My ASP.NET Application</title>
@Styles.Render("~/Content/css") @Scripts.Render("~/bundles/modernizr")</head>
<body>
<div
class=
"navbar navbar-inverse navbar-fixed-top"
>
<div
class=
"container"
>
<div
class=
"navbar-header"
>
<button
type=
"button"
class=
"navbar-toggle"
data-toggle=
"collapse"
data-target=
".navbar-collapse"
>
<span
class=
"icon-bar"
></span>
<span
class=
"icon-bar"
></span>
<span
class=
"icon-bar"
></span>
</button>
@Html.ActionLink("Application name", "Index", "Home", new { area = "" }, new { @class = "navbar-brand" })</div>
<div
class=
"navbar-collapse collapse"
>
<ul
class=
"nav navbar-nav"
>
<li>
@Html.ActionLink("Home", "Index", "Home")</li>
<li>
@Html.ActionLink("About", "About", "Home")</li>
<li>
@Html.ActionLink("Contact", "Contact", "Home")</li>
</ul>
</div>
</div>
</div>
<div
class=
"container body-content"
>
@RenderBody()<hr
/>
<footer>
<p>
©
@DateTime.Now.Year - My ASP.NET Application</p>
</footer>
</div>
@Scripts.Render("~/bundles/jquery") @Scripts.Render("~/bundles/bootstrap") @RenderSection("scripts", required: false)</body>
</html>
The default layout contains the reusable HTML that appears on every page within the site (elements such as the page title, header, footer, CSS, JavaScript, etc.).
The view that is being rendered is inserted into this layout when the function RenderBody
is called via Razor code.
There are a lot of other things happening in this shared layout. It is using several helper classes provided by the MVC framework, such as the HtmlHelper class to create links, the Scripts class to include JavaScript files, the Styles class to include CSS files, and RenderSection
to allow your views to mark specific content to be inserted in a specific spot in the shared layout. This will be shown in Chapter 2.
Understanding the URL Structure
When you launched the default website, three links were created:
- The Home link. This took you to the root of the site (/).
- The About link. This took you to /Home/About.
- The Contact link. This took you to /Home/Contact.
These links work because when an MVC project is first created, a default route is configured to make them work. Routes allow your website to tell MVC how it should map a URL to a specific controller and action within your project.
Routes are configured in the App_Start/RouteConfig.cs file. Example 1-4 shows the default route that is configured with a new MVC project.
Example 1-4. Default route
routes
.
MapRoute
(
name
:
"Default"
,
url
:
"{controller}/{action}/{id}"
,
defaults
:
new
{
controller
=
"Home"
,
action
=
"Index"
,
id
=
UrlParameter
.
Optional
}
);
Three important things are defined in this route config:
- A name. Each route must have a unique name.
- The URL. This is a relative URL after the website’s domain name. The URL can contain a mix of static text and variables.
- Default configuration. If any of the variables in the URL are not provided, defaults may be set for them.
If we reanalyze the links mentioned earlier, they work because:
- When we go to / the URL contains no controller, action, or ID. The defaults that are set indicate that it will go to the
HomeController
, theIndex
action, and the ID does not need to exist. - When we go to /Home/About and /Home/Contact, no defaults are used because both the controller and action are specified in the URL.
In fact, the Home link can also be accessed via /Home and /Home/Index as well. However, when URLs are created within a view, MVC picks the shortest, most accurate URL, e.g., just /.
With this route, you can create a new controller and/or action, and it can automatically be accessed by its name and action name.
Summary
If you are new to MVC, this chapter might feel overwhelming. The predefined MVC templates in Visual Studio are quite extensive and provide developers with a large head start in their projects.
Because of this, it’s difficult to cover every detail of what gets created. In this introductory chapter, I have covered many of the core features that will get you started.
There are many more details to cover with Models-Views-Controllers. As this book progresses and covers more advanced topics, they will be explored thoroughly in those chapters.
Get ASP.NET MVC 5 with Bootstrap and Knockout.js now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.