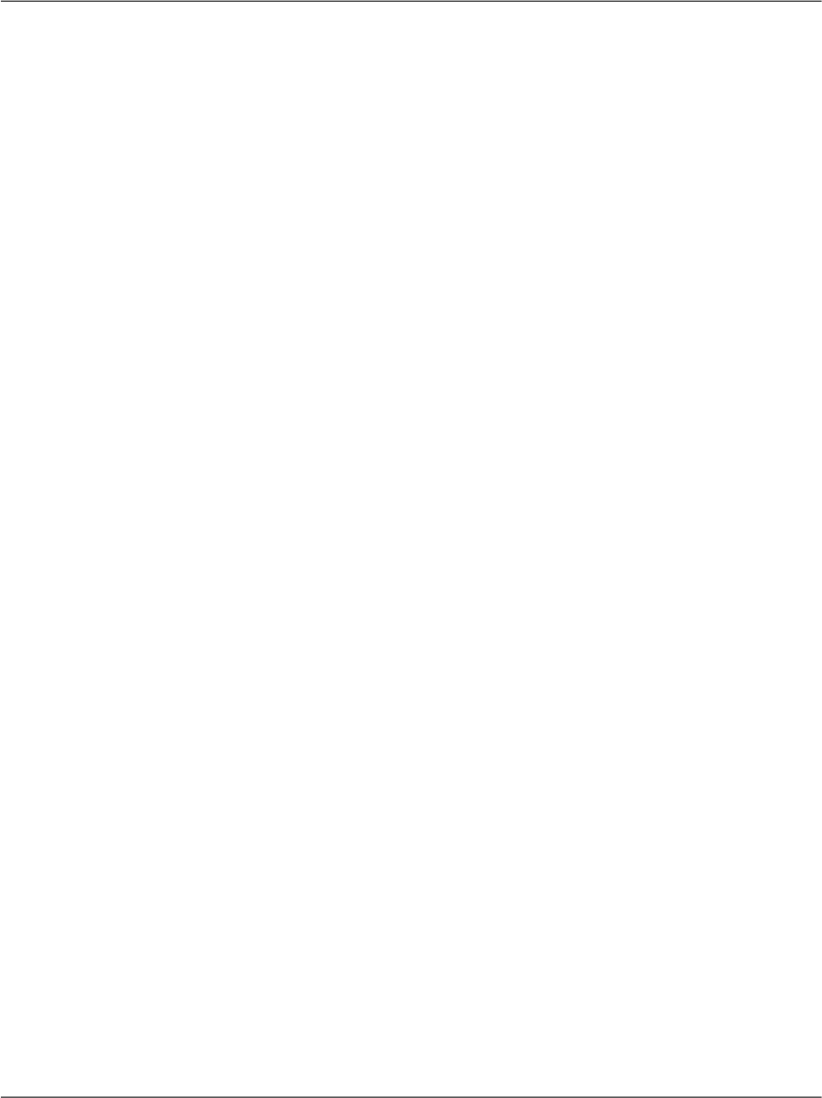
Foreign Keys ............................................................................ 278
Using Database Diagrams ........................................................ 280
Implementing Relationships in the Dorknozzle Database .......... 284
Diagrams and Table Relationships ............................................ 287
Summary ......................................................................................... 292
8. Speaking SQL ..................................................................................... 293
Reading Data from a Single Table ..................................................... 294
Using the SELECT Statement ..................................................... 297
Selecting Certain Fields ............................................................ 299
Selecting Unique Data with DISTINCT ...................................... 300
Row Filtering with WHERE .......................................................... 302
Selecting Ranges of Values with BETWEEN .................................. 303
Matching Patterns with LIKE .................................................... 304
Using the IN Operator .............................................................. 305
Sorting Results Using ORDER BY ................................................ 306
Limiting the Number of Results with TOP ................................. 307
Reading Data from Multiple Tables .................................................. 307
Subqueries ............................................................................... 308
Table Joins ............................................................................... 309
Expressions and Operators ............................................................... 310
Transact-SQL Functions ................................................................... 313
Arithmetic Functions ................................................................ 314
String Functions ....................................................................... 315
Date and Time Functions ......................................................... 317
Working with Groups of Values ........................................................ 318
The COUNT Function ................................................................. 319
Grouping Records Using GROUP BY ........................................... 319
Filtering Groups Using HAVING ................................................. 321
The SUM, AVG, MIN, and MAX Functions ....................................... 322
Updating Existing Data .................................................................... 322
The INSERT Statement ............................................................. 323
The UPDATE Statement ............................................................. 324
The DELETE Statement ............................................................. 325
Stored Procedures ............................................................................ 326
Summary ......................................................................................... 330
9. ADO.NET ........................................................................................... 331
Introducing ADO.NET ..................................................................... 332
Importing the SqlClient Namespace ....................................... 333
Defining the Database Connection ........................................... 334
Preparing the Command .......................................................... 336
vi
Build Your Own ASP.NET 2.0 Web Site Using C# & VB