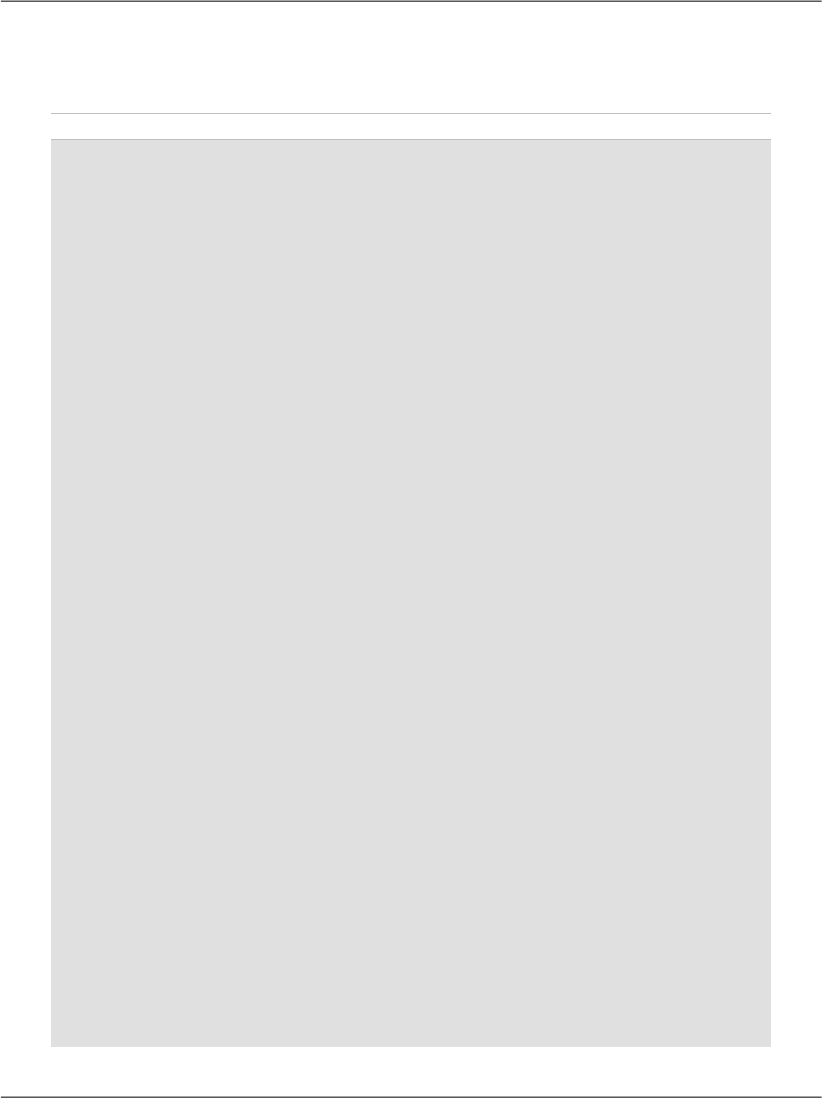
Modify this method by adding code that inserts the user-submitted help desk
request into the database, as shown below:
Visual Basic File: HelpDesk.aspx.vb (excerpt)
Protected Sub submitButton_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles submitButton.Click
If Page.IsValid Then
' Define data objects
Dim conn As SqlConnection
Dim comm As SqlCommand
' Read the connection string from Web.config
Dim connectionString As String = _
ConfigurationManager.ConnectionStrings( _
"Dorknozzle").ConnectionString
' Initialize connection
conn = New SqlConnection(connectionString)
' Create command
comm = New SqlCommand( _
"INSERT INTO HelpDesk (EmployeeID, StationNumber, " & _
"CategoryID, SubjectID, Description, StatusID) " & _
"VALUES (@EmployeeID, @StationNumber, @CategoryID, " & _
"@SubjectID, @Description, @StatusID)", conn)
' Add command parameters
comm.Parameters.Add("@EmployeeID", System.Data.SqlDbType.Int)
comm.Parameters("@EmployeeID").Value = 5
comm.Parameters.Add("@StationNumber", _
System.Data.SqlDbType.Int)
comm.Parameters("@StationNumber").Value = stationTextBox.Text
comm.Parameters.Add("@CategoryID", System.Data.SqlDbType.Int)
comm.Parameters("@CategoryID").Value = _
categoryList.SelectedItem.Value
comm.Parameters.Add("@SubjectID", System.Data.SqlDbType.Int)
comm.Parameters("@SubjectID").Value = _
subjectList.SelectedItem.Value
comm.Parameters.Add("@Description", _
System.Data.SqlDbType.NVarChar, 50)
comm.Parameters("@Description").Value = _
descriptionTextBox.Text
comm.Parameters.Add("@StatusID", System.Data.SqlDbType.Int)
comm.Parameters("@StatusID").Value = 1
' Enclose database code in Try-Catch-Finally
Try
' Open the connection
conn.Open()
' Execute the command
comm.ExecuteNonQuery()
374
Chapter 9: ADO.NET