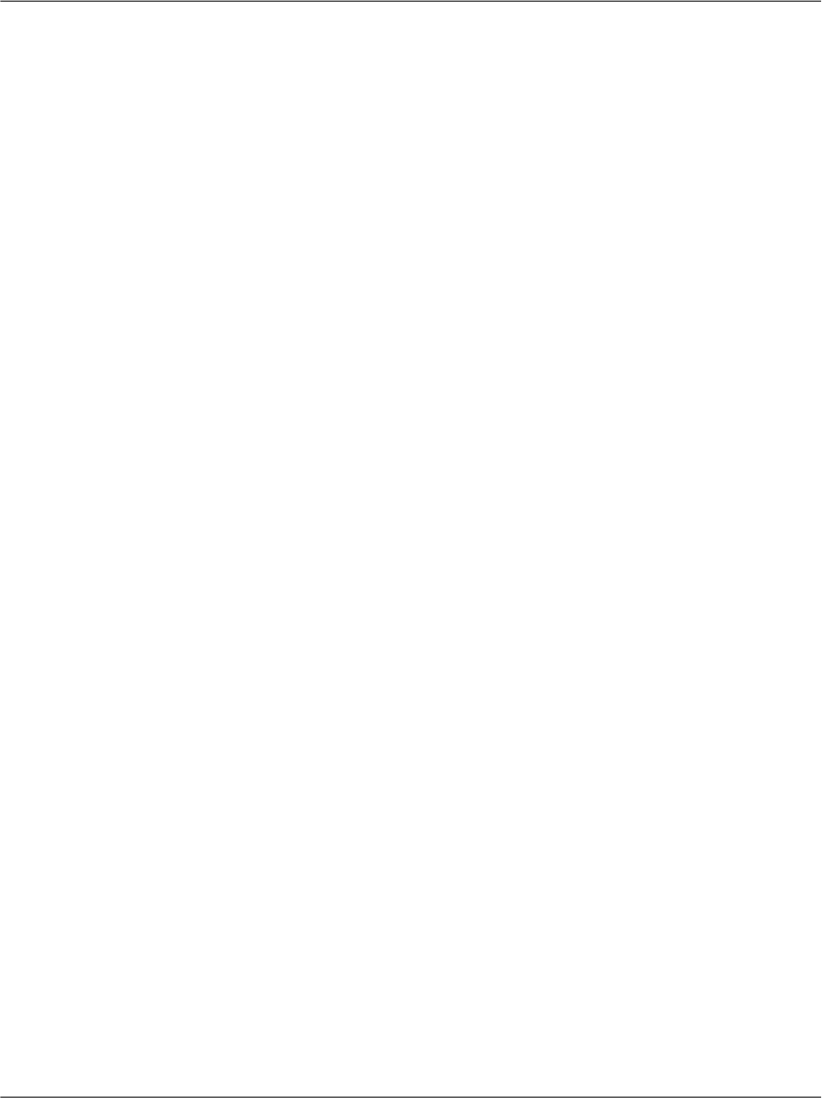
control supports three display modes. You can change the current mode using
its ChangeMode method, providing as parameter one of these values:
DetailsViewMode.ReadOnly
This is the default mode, which is used to display data. When you execute
your project, and load the details of an employee, you see those details in
ReadOnly mode.
DetailsViewMode.Edit
This mode is used to edit an existing record. We saw this mode in action
earlier, when we clicked the Edit button.
DetailsViewMode.Insert
We use this mode to insert a new record. It’s similar to the edit mode, except
all the controls are empty, so you can fill in data for a new item.
If you look at the employeeDetails_ModeChanging, you’ll see it receives a para-
meter named e that is an object of class DetailsViewModeEventArgs. e’s NewMode
property tells us which display mode was requested by the user. Its value will be
DetailsViewMode.Edit when the ModeChanging event is fired as a result of the
Edit button being clicked. We pass this value to the DetailsView control’s
ChangeMode method, which does exactly as its name suggests: it changes the mode
of the DetailsView. With this code, you’ve implemented the functionality to
make both the Edit and Cancell buttons work correctly, as we’ll see in an example
shortly.
However, note that once you switch to edit mode, clicking the Update button
will generate an error, because we still haven’t handled the ItemUpdating event
that’s fired when the user tries to save changes to a record. We’ll create the event
handler later; next, we want to improve our existing solution using templates.
Using Templates
The built-in column types are sufficiently varied and configurable to provide for
most of the functionality you’re likely to need, but in cases where further custom-
ization is required, you can make the desired changes using templates. In the
smart tag menu of the GridView and DetailsView controls, an option called Edit
Columns (for the GridView) or Edit Fields (for the DetailsView) is available. Se-
lecting that option opens a dialog that provides us with a great deal of control
over the options for each column or field.
459
Using Templates