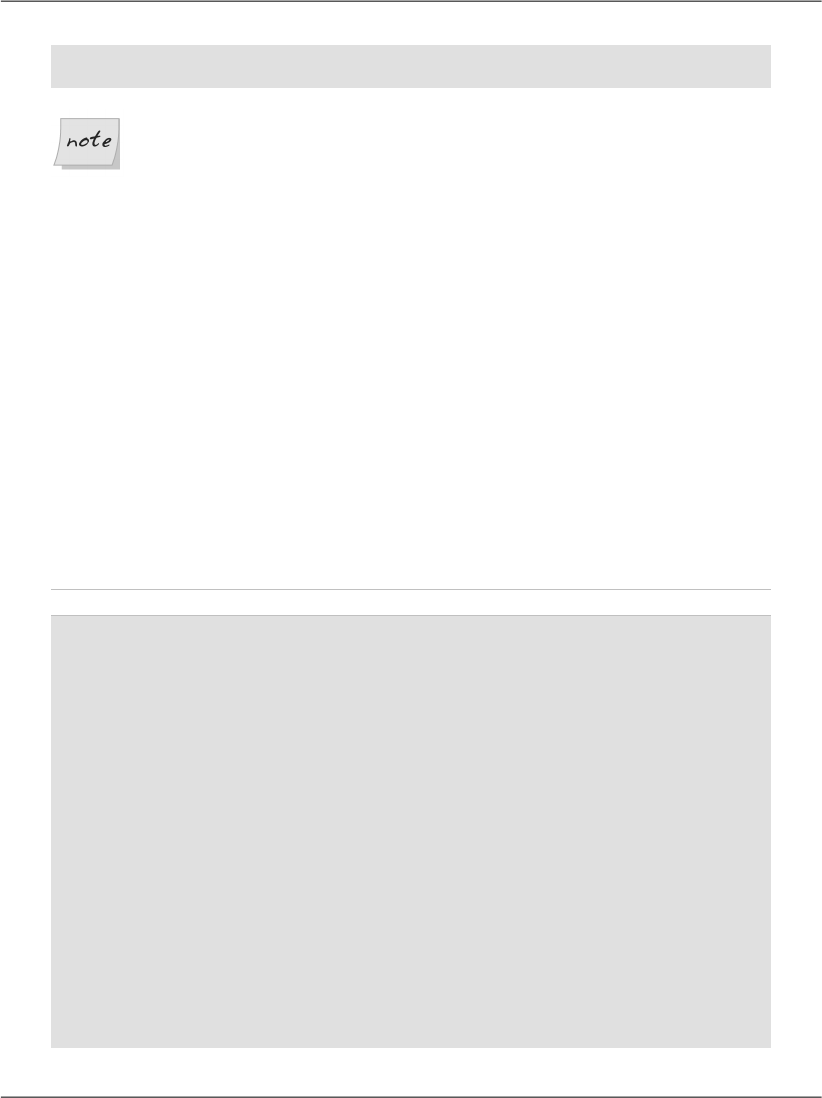
dataSet.Tables["Departments"].DefaultView;
departmentsGrid.DataBind();
DefaultView does not Apply when Binding to a DataSet
It’s interesting to note that if you bind directly to a DataSet that contains
only one table, that table’s DefaultView will not be used; the GridView
will generate a separate DataView itself.
DataViews represent a customized view of a DataSet for sorting, filtering,
searching, editing, and navigation. When binding a GridView directly to a
DataTable, the DefaultView property, which is a DataView object, is accessed
automatically for us. However, if we want to enable sorting, we need to access
the DataView and set its sorting parameters.
The first step to enabling sorting is to set the AllowSorting property to True.
When we do that, the grid’s column headings become hyperlinks. Before we make
those hyperlinks work, we need to handle the grid’s Sorting event, where we
teach the grid what to do when those links are clicked.
Set the AllowSorting property of the GridView control in Departments.aspx
to True, then use the designer to generate the handler for the GridView’s Sorting
event. Then, complete the code as shown:
Visual Basic File: Departments.aspx.vb (excerpt)
Protected Sub departmentsGrid_Sorting(ByVal sender As Object, _
ByVal e As System.Web.UI.WebControls.GridViewSortEventArgs) _
Handles departmentsGrid.Sorting
' Retrieve the name of the clicked column (sort expression)
Dim sortExpression As String = e.SortExpression
' Decide and save the new sort direction
If (sortExpression = gridSortExpression) Then
If gridSortDirection = SortDirection.Ascending Then
gridSortDirection = SortDirection.Descending
Else
gridSortDirection = SortDirection.Ascending
End If
Else
gridSortDirection = WebControls.SortDirection.Ascending
End If
' Save the new sort expression
gridSortExpression = sortExpression
' Rebind the grid to its data source
BindGrid()
End Sub
510
Chapter 12: Advanced Data Access