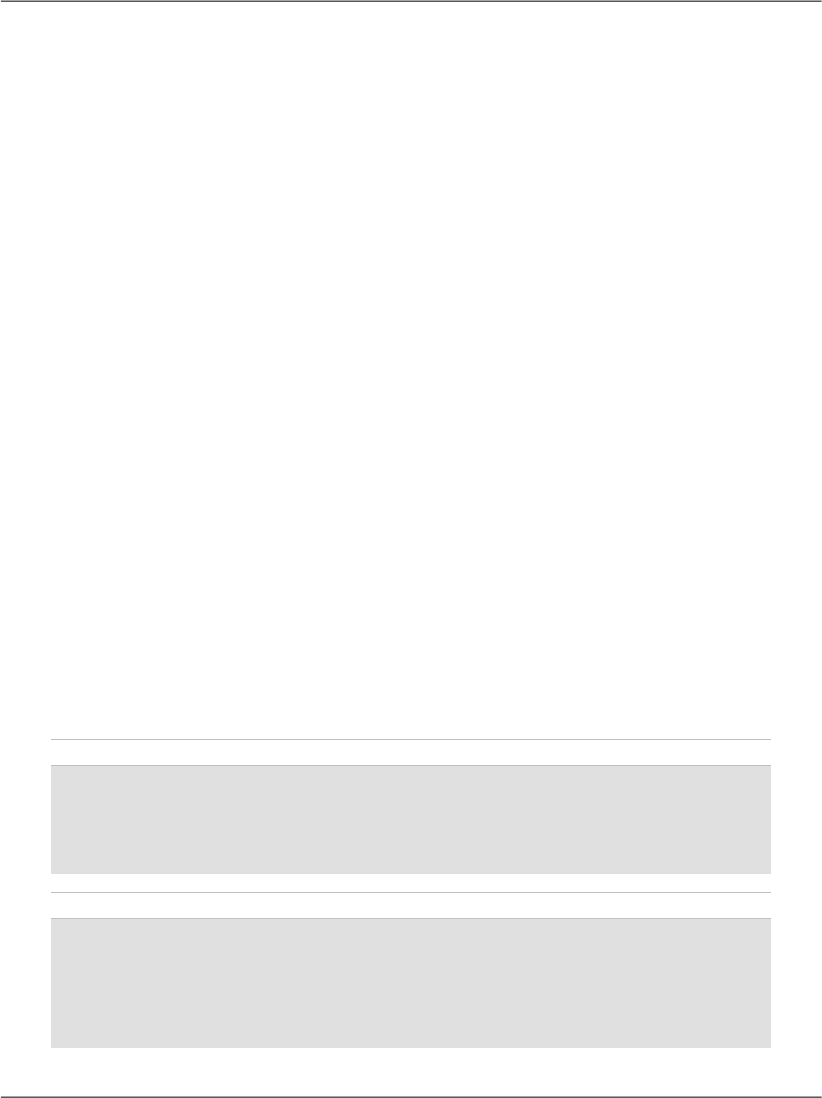
use a single equals sign in C# unless you’re assigning a value to a variable; other-
wise your code will have a very different meaning than you expect!
Breaking Long Lines of Code
Since the message string in the above example was too long to fit on one line in
this book, we used the string concatenation operator to combine two shorter
strings on separate lines to form the complete message. In VB, we also had to
break one line of code into two using the line continuation symbol (_, an under-
score at the end of the line to be continued). Since C# marks the end of each
command with a semicolon (;), you can split a single command over two lines
in this language without having to do anything special.
We’ll use these techniques throughout this book to present long lines of code
within a limited page width. Feel free to recombine the lines in your own code
if you like—there's no length limit on lines of VB and C# code.
Conditional Logic
As you develop ASP.NET applications, there will be many instances in which
you’ll need to perform an action only if a certain condition is met, for instance,
if the user has checked a certain checkbox, selected a certain item from a
DropDownList control, or typed a certain string into a TextBox control. We check
for such occurrences using conditionals, the simplest of which is probably the If
statement. This statement is often used in conjunction with an Else statement,
which specifies what should happen if the condition is not met. So, for instance,
we may wish to check whether or not the name entered in a text box is Zak,
redirecting the user to a welcome page if it is, or to an error page if it’s not:
Visual Basic
If (userName.Text = "Zak") Then
Response.Redirect("ZaksPage.aspx")
Else
Response.Redirect("ErrorPage.aspx")
End If
C#
if (userName.Text == "Zak")
{
Response.Redirect("ZaksPage.aspx");
}
else
{
70
Chapter 3: VB and C# Programming Basics