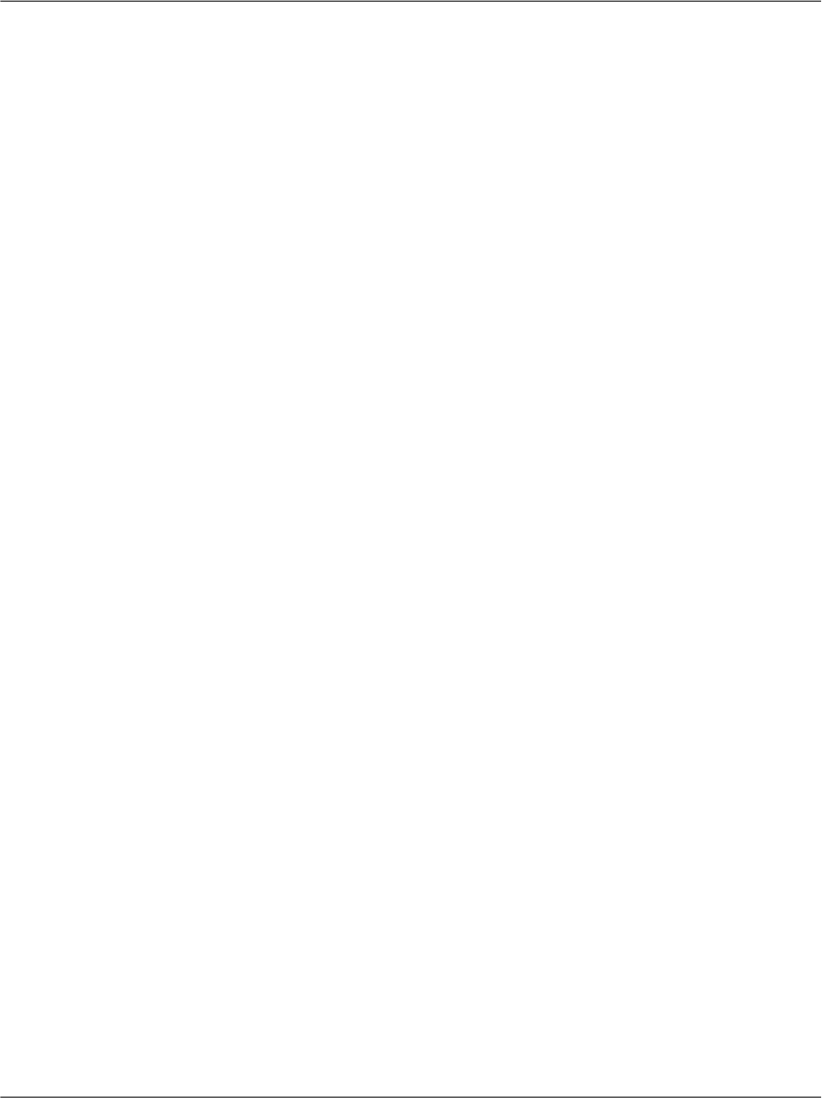
At least one constructor will be defined for most of the classes you will write
(though we can define more than one constructor for a class, as we’ll see shortly),
since it’s likely that some data will need to be initialized for each class at creation
time.
In C# and VB, the constructor is defined as a method that has the same name
as the class, and has no return type.
Scope
You should now understand programming objects to be entities that exist in a
program and are manipulated through the methods and properties they expose.
However, in some cases, we want to create for use inside our class methods that
are not available to code outside that class.
Imagine we’re writing the Sit method inside this class, and we realize that before
the dog can sit, it has to shuffle its back paws forward a little (bear with me on
this one!). We could create a method called ShufflePaws, then call that method
from inside the Sit method. However, we don’t want code in an ASP.NET page
or in some other class to call this method—it’d just be silly. We can prevent it
by controlling the scope of the ShufflePaws method.
Carefully controlling which members of a class are accessible from outside that
class is fundamental to the success of object oriented programming. You can
control the visibility of a class member using a special set of keywords called ac-
cess modifiers:
Public
Defining a property or method of a class as public allows that
property or method to be called from outside the class itself. In
other words, if an instance of this class is created inside another
object (remember, too, that ASP.NET pages themselves are objects),
public methods and properties are freely available to the code that
created that instance of the class. This is the default scope for VB
and C# classes.
Private
If a property or method of a class is private, it cannot be used from
outside the class itself. So, if an instance of this class is created in-
side an object of a different class, the creating object has no access
to private methods or properties of the created object.
Protected
A protected property or method sits somewhere between public
and private. A protected member is accessible from the code within
83
Scope