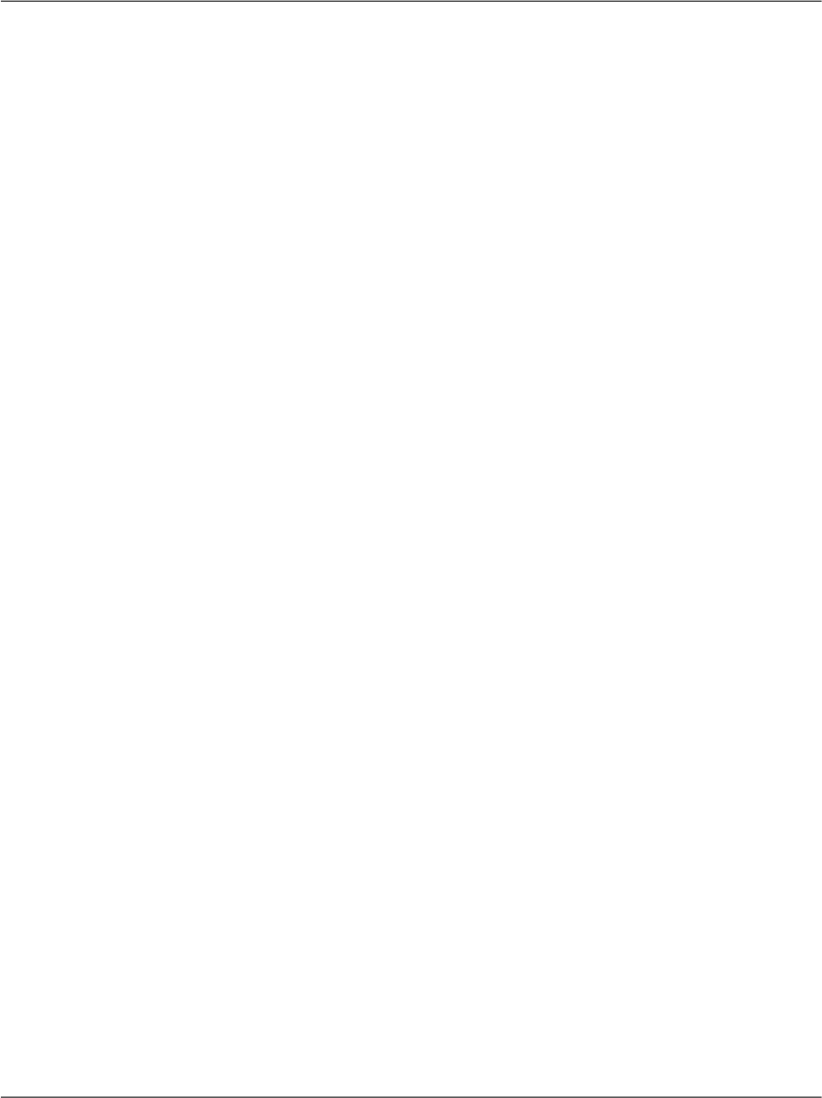
Web User Controls
As you build real-world projects, you’ll frequently encounter pieces of the user
interface that appear in multiple places—headers or footers, navigation links, and
login boxes are just a few examples. Packaging their forms and behaviors into
your own controls will allow you to reuse these components just as you can reuse
ASP.NET’s built-in controls.
Building your own web server controls involves writing advanced VB or C# code,
and is not within the scope of this book, but it’s good to know that it’s possible.
Creating customized web server controls makes sense when you need to build
more complex controls that provide a high level of control and performance, or
you want to create controls that can be integrated easily into many projects.
Those of us without advanced coding skills can develop our own controls by
creating web user controls. These are also powerful and reusable within a given
project; they can expose properties, events, and methods, just like other controls;
and they’re easy to implement.
A web user control is represented by a class that inherits from
System.Web.UI.UserControl, and contains the basic functionality that you need
to extend to create your own controls. The main drawback to using web user
controls is that they’re tightly integrated into the projects in which they’re imple-
mented. As such, it’s more difficult to distribute them, or include them in other
projects, than it is to distribute or reuse web server controls.
Web user controls are implemented very much like normal web forms—they’re
comprised of other controls, HTML markup, and server-side code. The file exten-
sion of a web user control is .ascx.
Creating a Web User Control
Let’s get a feel for web user controls by stepping through a simple example. Let’s
say that in your web site, you have many forms consisting of pairs of Label and
TextBox controls, like the one shown in Figure 4.10.
All the labels must have a fixed width of 100 pixels, and the text boxes must accept
a maximum of 20 characters.
Rather than adding many labels and text boxes to the form, and then having to
set all their properties, let’s make life easier by building a web user control that
126
Chapter 4: Constructing ASP.NET Web Pages