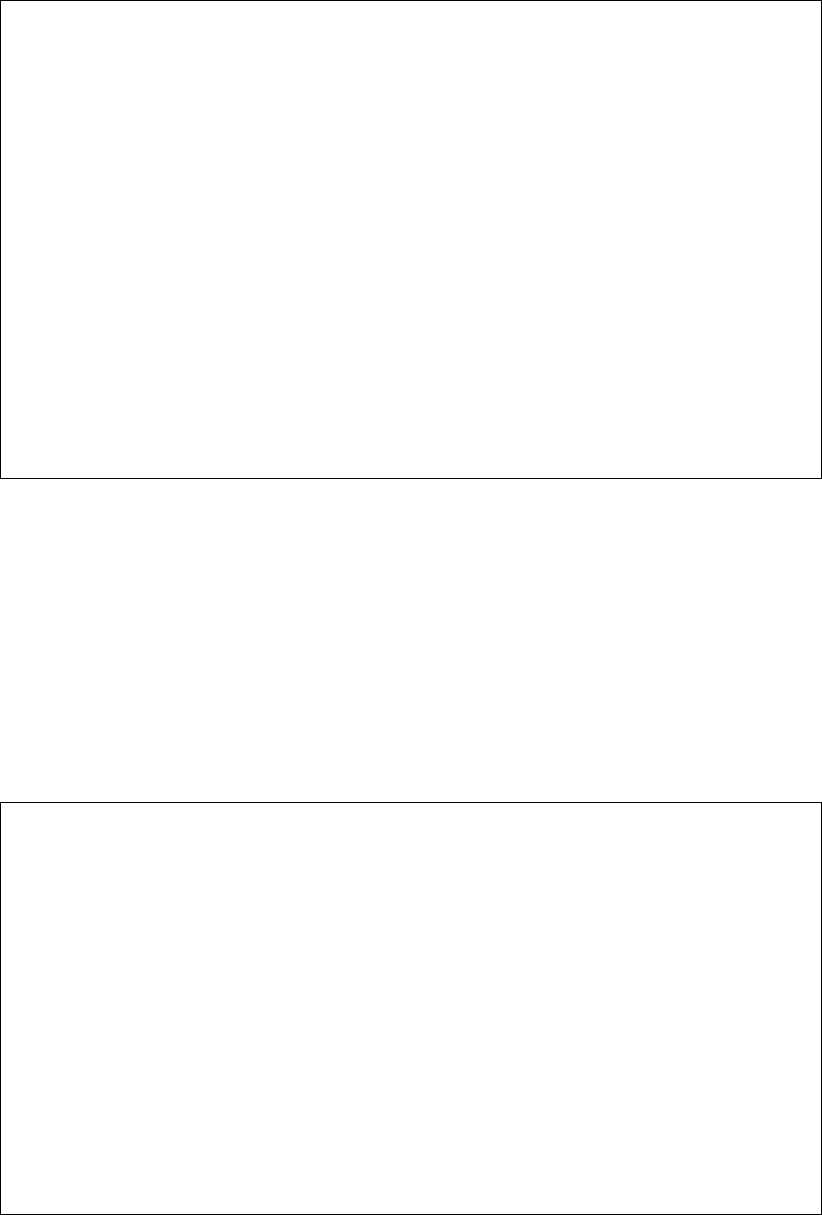
Chapter 9. Moving the server application to Java 367
Figure 9-27 RMIExample getRecord() method
The getRecord() method calls the submit() method of the remoteRequestor object passing
the part number as a parameter. An Item object is returned that contains the details about the
Item (or Part).
If an Item object is returned, the item requested was found in the database. We use the getter
methods provided by the Item object to retrieve the information and display it on the screen.
The populateAllParts() method, shown in Figure 9-28, is called when the user clicks the
Get All Parts button. It calls the submitAll() method of the remoteRequestor object passing
no parameters.
Figure 9-28 RMIExample populateAllParts() method
public String getRecord(String partNo, javax.swing.JTextField partDesc,
javax.swing.JTextField partQty, javax.swing.JTextField partPrice,
javax.swing.JTextField partDate) throws Exception {
Item rtnItem = remoteRequestor.submit(partNo);
if ((rtnItem) != null)
{
partDesc.setText(rtnItem.getItemDesc());
partDate.setText(rtnItem.getItemDate());
partQty.setText((Integer.toString(rtnItem.getItemQuantity())));
partPrice.setText("$" + (rtnItem.getItemPrice()).toString());
}
else {
partDesc.setText("");
partDate.setText("");
partQty.setText("");
partPrice.setText("");
return "Record not found";
}
return "Record found";
}
public void populateAllParts(javax.swing.table.DefaultTableModel defaultTableModel)
throws Exception
{
Item rtnItem = remoteRequestor.submitAll();
if (rtnItem != null) {
for (int i = 0; i < rtnItem.getNumEntries(); i++) {
ItemDetail rtnDetail = rtnItem.getNextEntry(i);
String[] array = new String[5];
array[0] = rtnDetail.getItemId();
array[1] = rtnDetail.getItemDsc();
array[2] = rtnDetail.getItemPrice();
array[3] = rtnDetail.getItemQty();
array[4] = rtnDetail.getItemDate();
defaultTableModel.addRow(array);
}
}
return;
}