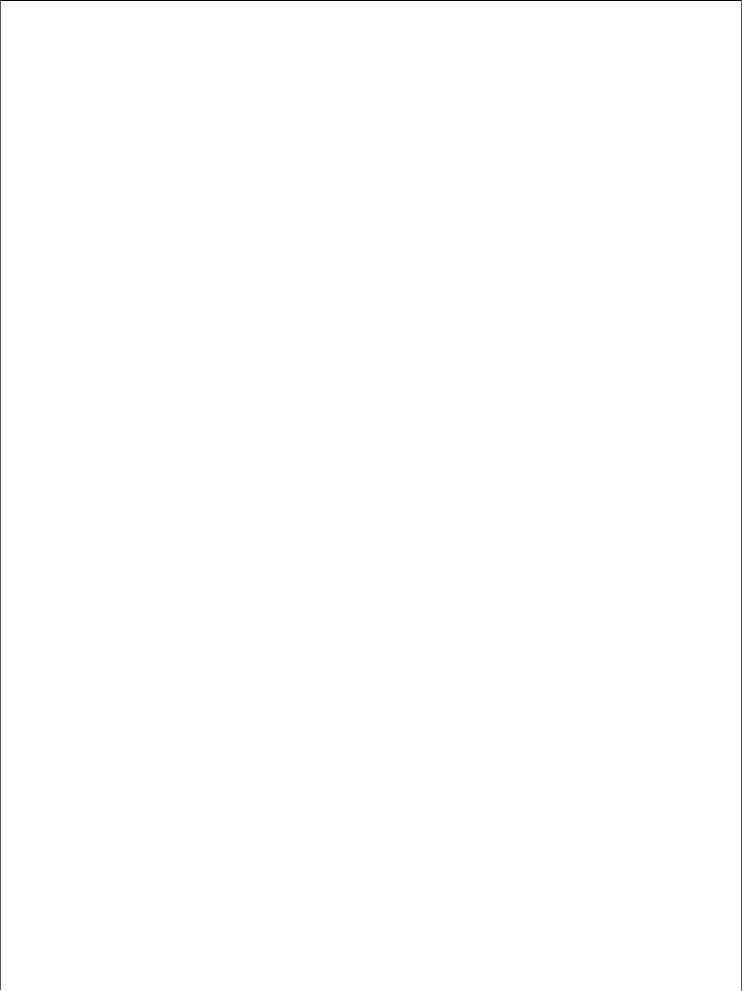
10.6 Example of camera models
This section illustrates the mapping of points into an image frame for the per-
spective and affine camera models. Code 10.1 contains the functions used to create
%***********************************************
%Draw a set of points
%-----------------------------------------------
function DrawPoints(P,colour)
%-----------------------------------------------
[r,c]=size(P);
plot3(P(1,1:c),P(2,1:c),P(3,1:c),'+','color',colour);
%***********************************************
%Draw a set of Image points
%----------------------------------------------
function DrawImagePoints(P,C,colour)
%----------------------------------------------
[r,c]=size(P);
for column=1:c
P(1:r-1,column)=P(1:r-1,column)/P(3,column);
end
plot(P(1,1:c),P(2,1:c),'+','color',colour);
axis([0 C(10) 0 C(11)]);
%***********************************************
%Draw a coordinate frame
%-----------------------------------------------
function DrawWorldFrame(x0,x1,y0,y1,z0,z1);
%-----------------------------------------------
axis equal; %same aspect ratio
axis([x0,x1,y0,y1,z0,z1]);
xlabel('X','FontSize',14);
ylabel('Y','FontSize',14);
zlabel('Z','FontSize',14);
grid on;
hold on;
%***********************************************
%Draw an image plane
%-----------------------------------------------
function DrawImagePlane(C,dx,dy);
%-----------------------------------------------
Appendix 2: Camera geometry fundamentals 371
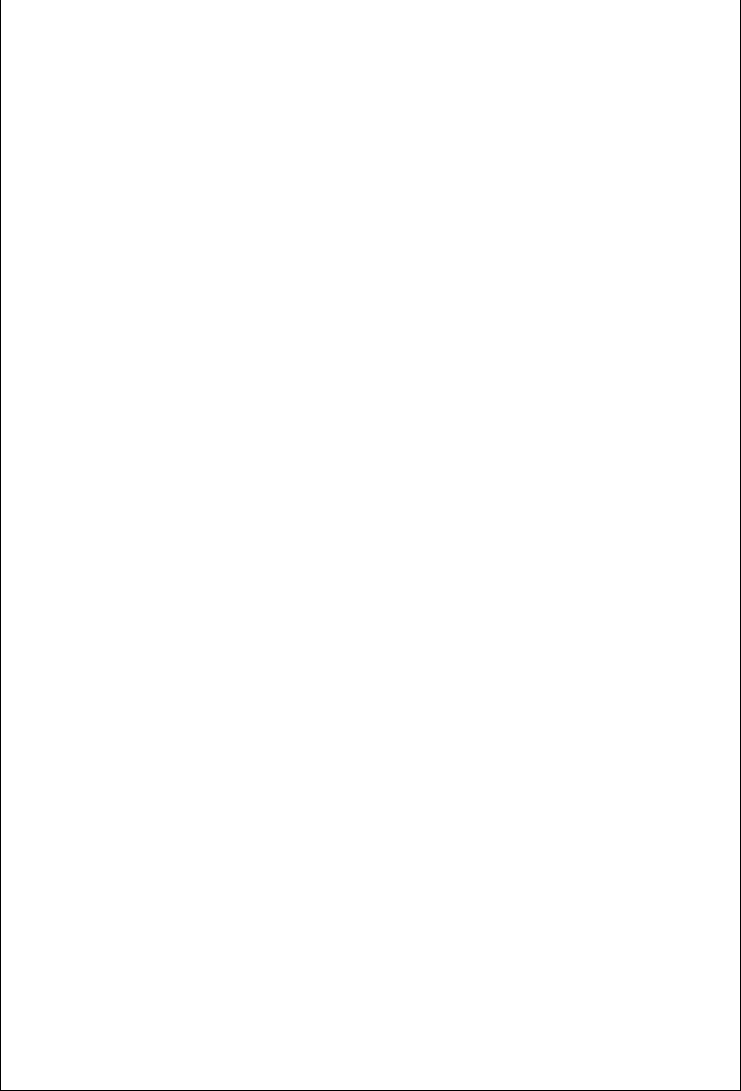
%CW: Camera to world transformation
CW=CameraToWorld(C);
%transform coordinates to world coordinates
P(:,1)=CW*p(:,1);
P(:,2)=CW*p(:,2);
P(:,3)=CW*p(:,3);
P(:,4)=CW*p(:,4);
%Draw image plane
patch(P(1,:),P(2,:),P(3,:),[.9,.9,1]);
%*************************************************
%Draw a line between optical centre and 3D points
%
%-----------------------------------------------------
function DrawPerspectiveProjectionLines (o,P,colour);
%-----------------------------------------------------
[r,c]=size(P);
for i=1:c
plot3([o(1) P(1,i)],[o(2) P(2,i)],[o(3) P(3,i)],'color',colour);
%optic centre
end;
%***********************************************
%Draw a line between image plane and 3D points
%
%-----------------------------------------------
function DrawAffineProjectionLines(z,P,colour);
%-----------------------------------------------
[r,c]=size(P);
for column=1:c
plot3([P(1,column) P(1,column)],[P(2,column) P(2,column)],[z(3)
P(3,column)],'color',colour); %optic centre
end;
%coordinates of 4 points on the
%image plane (to draw a rectangle)
p(1,1)=-dx/2; p(2,1)=-dy/2; p(3,1)=C(7); p(4,1)=1;
p(1,2)=-dx/2; p(2,2)=+dy/2; p(3,2)=C(7); p(4,2)=1;
p(1,3)=+dx/2; p(2,3)=+dy/2; p(3,3)=C(7); p(4,3)=1;
p(1,4)=+dx/2; p(2,4)=-dy/2; p(3,4)=C(7); p(4,4)=1;
%Draw camera origin
plot3(C(1),C(2),C(3),'o'); %optic centre
plot3(C(1),C(2),C(3),'+'); %optic centre
Code 10.1 Drawing functions
372 Feature Extraction and Image Processing
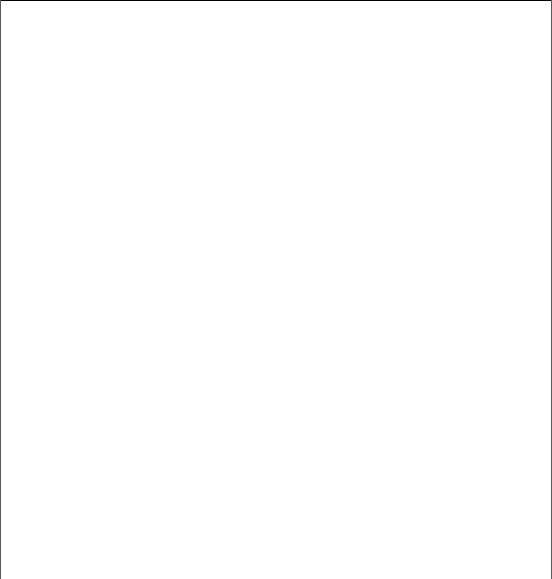
figures: DrawPoints, DrawImagePoints, DrawWorldFrame, DrawImagePlane,
DrawPerspectiveProjectionLines and DrawAffineProjectionLines.
The function DrawPoints draws a set of points given its three world coordinates. In our
example, it is used to draw points in the world. The function DrawImagePoints draws points
on the image plane. It uses homogeneous coordinates, so it implements Equation 10.3. The
function DrawWorldFrame draws the three axes given the location of the origin. This is used to
illustrate the world frame and the origin is always set to zero. The function DrawImagePlane
draws a rectangle that represents the image plane. It also draws a point to illustrate the location
of the centre of the camera. In our examples, we assume that the centre of the camera is at the
position of the focal point, for both the perspective and the affine model.
The functions DrawPerspectiveProjectionLines and DrawAffine
ProjectionLines are used to illustrate the projection of points into the image plane for
the perspective and affine projections, respectively. These functions take a vector of points and
trace a line to the image plane that represent the projection. For perspective, the lines intersects
the focal point and for affine they are projected parallel to the image plane.
In the example implementation, we group the camera parameters in the vector
C =x0y0z0abgfu0v0 kx ky. The first six elements define the location and rotation
parameters. The value of f defines the focal length. The remaining parameters define the loca-
tion of the optical centre and the pixel size. For simplicity, we assume that there is no skew.
Code 10.2 contains the functions that compute camera transformations from camera parameters.
The function CameraToWorld computes a matrix that defines the position of the camera.
%***********************************************
%Compute matrix that transforms coordinates
%in the camera frame to the world frame%
%-----------------------------------------------
function CW=CameraToWorld(C);
%-----------------------------------------------
%rotation
Rx=[cos(C(6)) sin(C(6)) 0
-sin(C(6)) cos(C(6)) 0
0 0 1];
Ry=[cos(C(5)) 0 sin(C(5))
0 1 0
-sin(C(5)) 0 cos(C(5))];
Rz=[1 0 0
0 cos(C(4)) sin(C(4))
0 -sin(C(4)) cos(C(4))];
%translation
T=[C(1) C(2) C(3)]';
%transformation
CW=(Rz*Ry*Rx);
CW(:,4)=T;
Appendix 2: Camera geometry fundamentals 373
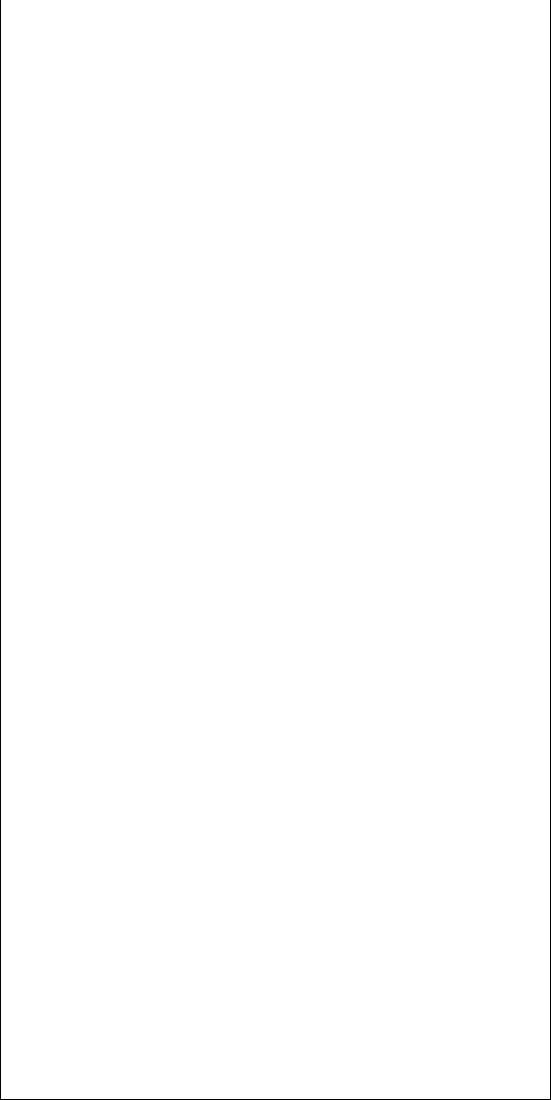
%***********************************************
%Convert from homogeneous coordinates in pixels
%to distance coordinates in the camera frame
%--------------------------------------------
function p=ImageToCamera(P,C);
%--------------------------------------------
%inverse of K
Ki=[1/C(10) 0 -C(8)/C(10)
0 1/C(11) -C(9)/C(11)
0 0 1 ];
%coordinate in distance units
p=Ki*P;
%coordinates in the image plane
p(1,:)=p(1,:)./p(3,:);
p(2,:)=p(2,:)./p(3,:);
p(3,:)=p(3,:)./p(3,:);
%the third coordinate gives the depth
%the focal length C(7) defines depth
p(3,:)=p(3,:).*C(7);
%include homogeneous coordinates
p(4,:)=p(1,:)/p(1,:);
Rz=[1 0 0
0 cos(C(4)) -sin(C(4))
0 sin(C(4)) cos(C(4))];
T=[-c(1) -c(2) -c(3)]';
%transformation
WC=(Rz*Ry*Rx); %rotation inverse
Tp=WC*T; %translation
WC(:,4)=Tp; %compose homogeneous form
%***********************************************
%Compute matrix that transforms coordinates
%in the world frame to the camera frame
%----------------------------------------------
function WC=WorldToCamera(c);
%----------------------------------------------
%translation T'=-R'T
%for R'=inverse rotation
Rx=[cos(C(6)) -sin(C(6)) 0
sin(C(6)) cos(C(6)) 0
0 0 1];
Ry=[cos(C(5)) 0 –sin(C(5))
0 1 0
sin(C(5)) 0 cos(C(5))];
Code 10.2 Transformation function
374 Feature Extraction and Image Processing
Get Feature Extraction & Image Processing, 2nd Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.