Chapter 6. Functions
A function (also known as a procedure or a subroutine) is an independent section of code that maps zero or more input parameters to zero or more output parameters. As illustrated in Figure 6-1, functions are often represented as a black box.
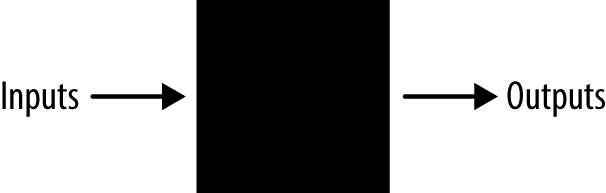
Figure 6-1. A black box function
In previous chapters, the programs we have written in Go have used only one function:
func
main
()
{}
We will now begin writing programs that use more than one function.
Your Second Function
Let’s take another look at the following program from Chapter 5:
func
main
()
{
xs
:=
[]
float64
{
98
,
93
,
77
,
82
,
83
}
total
:=
0.0
for
_
,
v
:=
range
xs
{
total
+=
v
}
fmt
.
Println
(
total
/
float64
(
len
(
xs
)))
}
This program computes the average of a series of numbers. Finding the average like this is a very general problem, so it’s an ideal candidate for definition as a function.
The average
function will need to take in a slice of float64
s and return one float64
. Insert this before the main
function:
func
average
(
xs
[]
float64
)
float64
{
panic
(
"Not Implemented"
)
}
Functions start with the keyword func
, followed by the function’s name. The parameters (inputs) of the function are defined like this: name type, name type, …
. Our function has one parameter (the list of scores) that we named xs
. After the parameters, we put the return type. Collectively, the parameters and the return type are known as the function’s ...
Get Introducing Go now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.