Name
DelayQueue<E extends Delayed>
Synopsis
This BlockingQueue
implementation restricts its elements to
instances of some class E
that implements
the Delay
interface. null
elements are not allowed. Elements on the queue are ordered by the
amount of delay remaining. The element whose getDelay(
)
method returns the smallest value is the first to be
removed from the queue. No element may be removed, however, until its
getDelay( )
method returns zero or a negative
number.
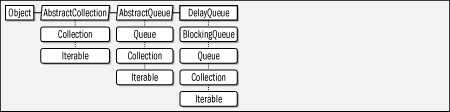
Figure 16-81. java.util.concurrent.DelayQueue<E extends Delayed>
public class DelayQueue<E extends Delayed> extends java.util.AbstractQueue<E> implements BlockingQueue<E> { // Public Constructors public DelayQueue( ); public DelayQueue(java.util.Collection<? extends E> c); // Public Instance Methods public E peek( ); public E poll( ); // Methods Implementing BlockingQueue public boolean add(E o); public int drainTo(java.util.Collection<? super E> c); public int drainTo(java.util.Collection<? super E> c, int maxElements); public boolean offer(E o); public boolean offer(E o, long timeout, TimeUnit unit); public E poll(long timeout, TimeUnit unit) throws InterruptedException; public void put(E o); public int remainingCapacity( ); public E take( ) throws InterruptedException; // Methods Implementing Collection public void clear( ); public java.util.Iterator<E> iterator( ); public boolean remove(Object o); public int size( ); ...
Get Java in a Nutshell, 5th Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.