Chapter 1. Welcome to React
React is a popular library used to create user interfaces. It was built at Facebook to address some of the challenges associated with large-scale, data-driven websites. When React was released in 2013, the project was initially viewed with some skepticism because the conventions of React are quite unique.
In an attempt to not intimidate new users, the core React team wrote an article called “Why React?” that recommended that you “Give It [React] Five Minutes.” They wanted to encourage people to work with React first before thinking that their approach was too crazy.
Yes, React is a small library that doesn’t come with everything you might need out of the box to build your application. Give it five minutes.
Yes, in React, you write code that looks like HTML right in your JavaScript. And yes, those tags require preprocessing to run in a browser. And you’ll probably need a build tool like webpack for that. Give it five minutes.
If you read that article—as we did—you may have been dazzled by the promise of a new JavaScript library—a library that would solve all of our problems with the DOM; a library that would always be easy to work with and would never hurt us.
Then the questions start to arise: how do I convert this JSX? How do I load data? Where does the CSS go? What is declarative programming? Every path leads to more questions about how to incorporate this library in your actual day to day work. Every conversation introduces new terminology, new techniques, and more questions.
Obstacles and Roadblocks
By taking a few minutes to learn about React components, you’ve opened a door to a different way of thinking about and approaching web development. However, there are some learning obstacles that you’ll have to overcome to begin writing production code with React.
React Is a Library
First, the React library is small and it is only used for one part of the job. It doesn’t ship with all of the tools that you’d expect from a traditional JavaScript framework. A lot of the decisions about which tools from the ecosystem to use are left up to the developer. Also, new tools emerge all the time, and old ones are cast aside. There are so many different library names continually being added to the discussion that it may feel like it’s impossible to keep up.
New ECMAScript Syntax
React has come of age in an important but chaotic time in the history of JavaScript. The ECMA used to release specifications infrequently. It would sometimes take up to 10 years to release a spec. This meant that developers wouldn’t need to learn new syntax very often.
As of 2015, new language features and syntax additions will be released every year. This replaces a numbered release system (ECMAScript3, ECMAScript 5) with a yearly one (ECMAScript 2016, ECMAScript 2017). As the language evolves, the early adopters in the React community tend to use the new syntax. This often means that documentation assumes knowledge of the latest ECMAScript syntax. If you are not familiar with the latest spec, looking at React code can be daunting.
Popularity of Functional JavaScript
In addition to the changes emerging at a language level, there is a lot of momentum around functional JavaScript programming. JavaScript isn’t necessarily a functional language, but functional techniques can be used in JavaScript code. React emphasizes functional programming over object-oriented programming. This shift in thinking can lead to benefits in areas like testability and performance. But when a lot of React materials assume an understanding of the paradigm, it can be hard to learn so much at once.
JavaScript Tooling Fatigue
It’s a cliche at this point to talk about JavaScript Fatigue, but the source of this fake illness can be traced back to the building process. In the past, you just added JavaScript files to your page. Now the JavaScript file has to be built, usually with an automated continuous delivery process. There’s emerging syntax that has to be transpiled to work in all browsers. There’s JSX that has to be converted to JavaScript. There’s SCSS that you might want to preprocess. These components need to be tested, and they have to pass. You might love React, but now you also need to be a webpack expert, handling code splitting, compression, testing, and on and on.
Why React Doesn’t Have to Be Hard to Learn
The goal of this book is to avoid confusion in the learning process by putting things in sequence and building a strong learning foundation. We’ll start with a syntax upgrade to get you acquainted with the latest JavaScript features, especially the ones that are frequently used with React. Then we’ll give an introduction to functional JavaScript so you can apply these techniques immediately and understand the paradigm that gave birth to React.
From there, we will cover foundational React knowledge including your first components and how and why we need to transpile our code. With the basics in place, we will break ground on a new application that allows users to save and organize colors. We will build this application using React, improve the code with advanced React techniques, introduce Redux as the client data container, and finish off the app by incorporating Jest testing and routing with the React Router. In the final chapter, we will introduce universal and isomorphic code and enhance the color organizer by rendering it on the server.
We hope to get you up to speed with the React ecosystem faster by approaching it this way—not just to scratch the surface, but to equip you with the tools and skills necessary to build real world React applications.
React’s Future
React is still new. It has reached a place where core functionality is fairly stable, but even that can change. Future versions of React will include Fiber, a reimplementation of React’s core algorithm which is aimed at increasing rendering speed. It’s a little early to hypothesize about how this will affect React developers, but it will definitely affect the speed at which apps are rendered and updated.
Many of these changes have to do with the devices that are being targeted. This book covers techniques for developing single-page web applications with React, but we shouldn’t assume that web browsers are the only place that React apps can run. React Native, released in 2015, allows you to take the benefits of React applications into iOS and Android native apps. It’s still early, but React VR, a framework for building interactive, virtual reality apps, has emerged as a way to design 360 degree experiences using React and JavaScript. A command of the React library will set you up to rapidly develop experiences for a range of screen sizes and types.
We hope to provide you with a strong enough foundation to be able to adapt to the changing ecosystem and build applications that can run on platforms beyond the web browser.
Keeping Up with the Changes
As changes are made to React and related tools, sometimes there are breaking changes. In fact, some of the future versions of these tools may break some of the example code in this book. You can still follow along with the code samples. We’ll provide exact version information in the package.json file, so that you can install these packages at the correct version.
Beyond this book, you can stay on top of changes by following along with the official React blog. When new versions of React are released, the core team will write a detailed blog post and changelog about what is new.
There are also a variety of popular React conferences that you can attend for the latest React information. If you can’t attend these in person, React conferences often release the talks on YouTube following the events. These include:
- React Conf
-
Facebook-sponsored conference in the Bay Area
- React Rally
-
Community conference in Salt Lake City
- ReactiveConf
-
Community conference in Bratislava, Slovakia
- React Amsterdam
-
Community conference in Amsterdam
Working with the Files
In this section, we will discuss how to work with the files for this book and how to install some useful React tools.
File Repository
The GitHub repository associated with this book provides all of the code files organized by chapter. The repository is a mix of code files and JSBin samples. If you’ve never used JSBin before, it’s an online code editor similar to CodePen and JSFiddle.
One of the main benefits of JSBin is that you can click the link and immediately start tinkering with the file. When you create or start editing a JSBin, it will generate a unique URL for your code sample, as in Figure 1-1.
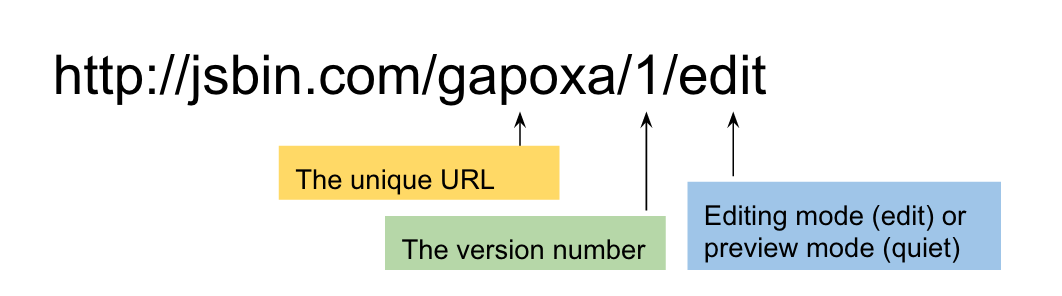
Figure 1-1. JSBin URL
The letters that follow jsbin.com represent the unique URL key. After the next slash is the version number. In the last part of the URL, there will be one of two words: edit for editing mode or quiet for preview mode.
React Developer Tools
There are several developer tools that can be installed as browser extensions or add-ons that you may find useful as well:
- react-detector
-
react-detector is a Chrome extension that lets you know which websites are using React and which are not.
- show-me-the-react
-
This is another tool, available for Firefox and Chrome, that detects React as you browse the internet.
- React Developer Tools (see Figure 1-2)
-
This is a plugin that can extend the functionality of the browser’s developer tools. It creates a new tab in the developer tools where you can view React elements.
If you prefer Chrome, you can install it as an extension; you can also install it as an add-on for Firefox.
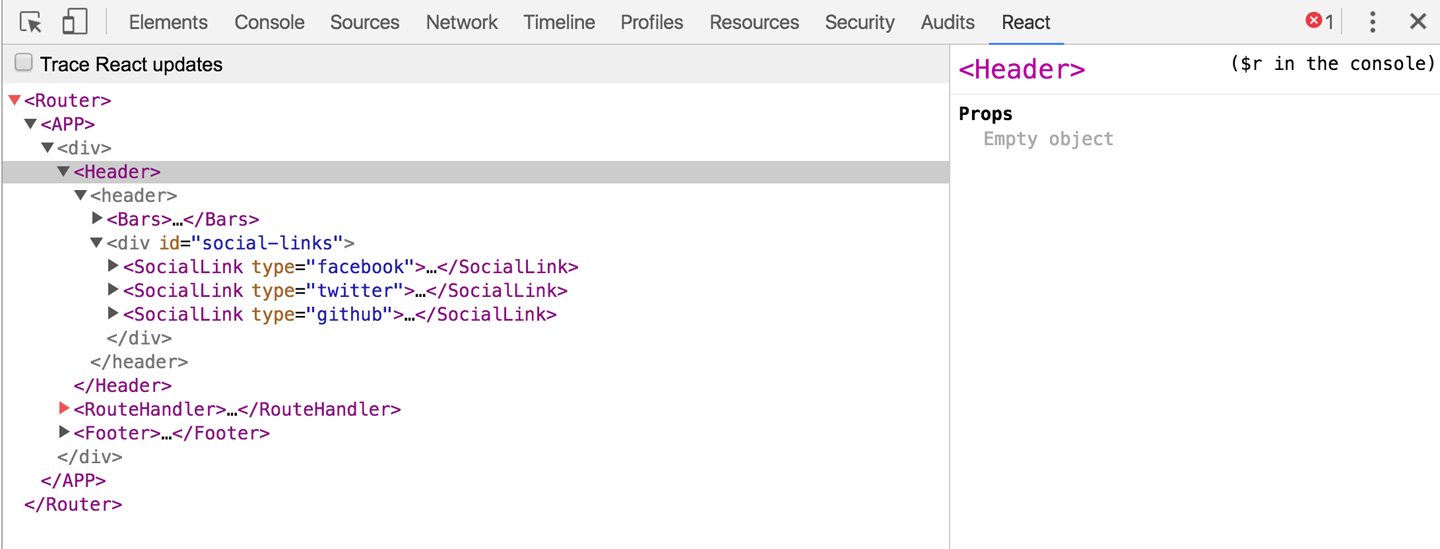
Figure 1-2. Viewing the React Developer Tools
Any time you see react-detector or show-me-the-react as active, you can open the developer tools and get an understanding of how React is being used on the site.
Installing Node.js
Node.js is JavaScript without the browser. It is a runtime environment used to build full-stack JavaScript applications. Node is open source and can be installed on Windows, macOS, Linux, and other platforms. We will be using Node in Chapter 12 when we build an Express server.
You do not need to use Node to use React. However, when working with React, you need to use the Node package manager, npm, to install dependencies. This is automatically installed with the Node installation.
If you’re not sure if Node.js is installed on your machine, you can open a Terminal or Command Prompt window and type:
$ node -v Output: v7.3.0
Ideally, you will have a Node version number of 4 or higher. If you type the command and see an error message that says “Command not found,” Node.js is not installed. This can be done directly from the Node.js website. Just go through the automated steps of the installer, and when you type in the node -v
command again, you’ll see the version number.
Now that you have your environment set up for React development, we are ready to begin overcoming learning obstacles. In Chapter 2, we will address ECMA, and get up to speed with the latest JavaScript syntax that is most commonly found in React code.
Get Learning React now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.