Chapter 4. Java EE and Microservices
Java EE began with less than 10 individual specifications, but it has grown over time through subsequent updates and releases to encompass 34. Compared to microservices-based architectures, Java EE and its included specifications were originally designed for a different development and deployment model. Only one monolithic server runtime or cluster hosted many different applications packaged according to standards. Such a model runs opposite to the goal of microservices.
Most Java EE APIs are synchronous, and scaling these resources is done through thread pools. Of course, this has its limits and is not meant to be adjusted for quickly changing requirements or excessive load situations. Given these requirements, it appears as if Java EE isn’t the best choice for developing microservices-based architectures.
But the latest versions of Java EE added a ton of developer productivity to the platform alongside a streamlined package. With the sloping modularity of Java and the JVM, an established platform, and skilled developers alongside individual implementations, Java EE is considered to be a reasonable solution for microservices development.
Matching the Real World
The latest available Java EE specification as of the writing of this report is Java EE 7. It contains 34 individual specifications, as shown in Figure 4-1.
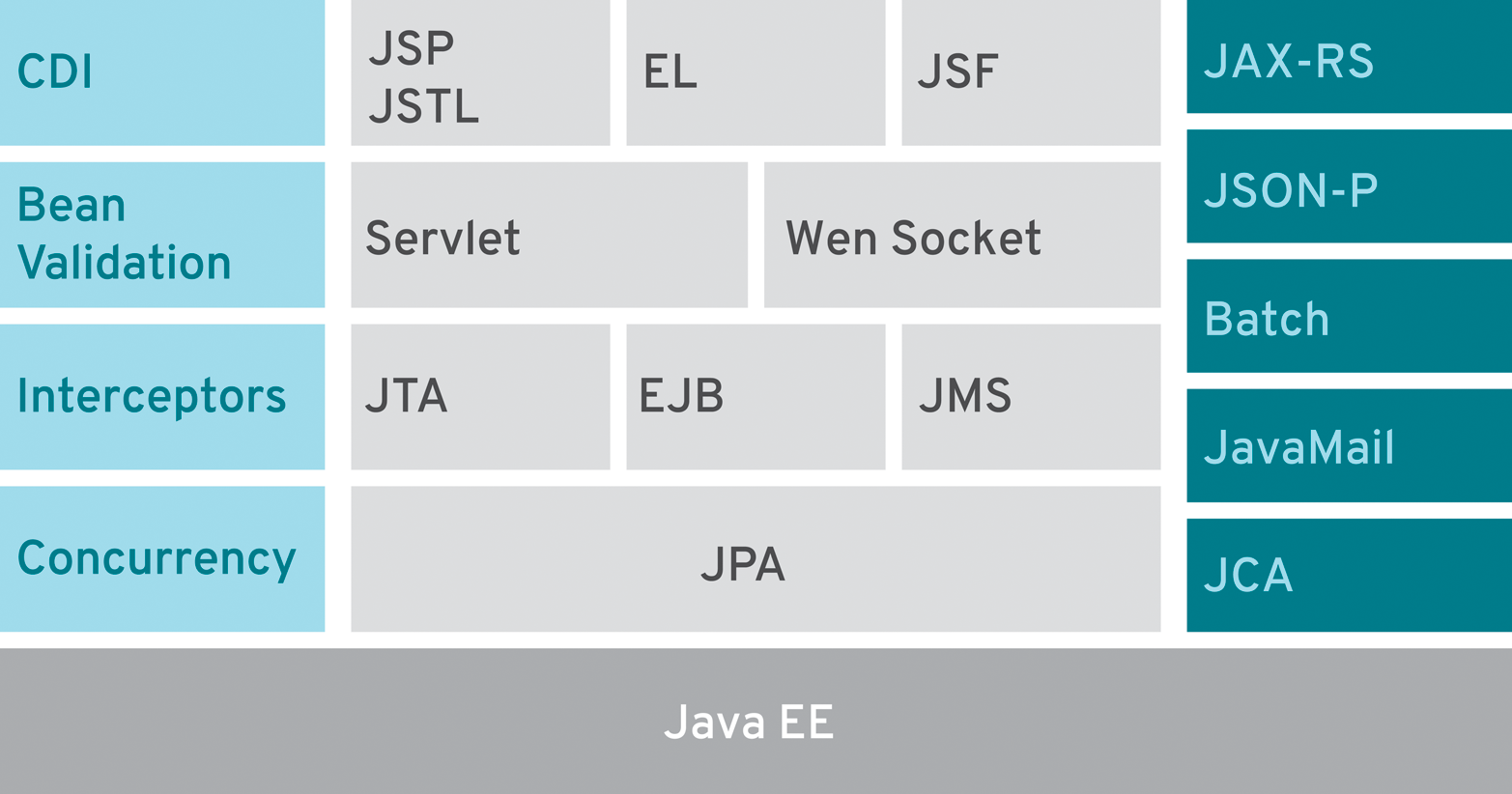
Figure 4-1. Java EE 7 at a glance
There are a lot of technologies that barely offer any advantages to microservices-based architectures, such as the Java Connector Architecture or the Batch Processing API. If you are starting to build microservices architectures on top of Java EE, make sure to look at the asynchronous features and try to use the best available parts. One important item to keep in mind: Java EE was never built to work with distributed applications or microservices. So every decision and line of code should be carefully inspected and validated to maximize asynchronicity.
JAX-RS 2.0
To execute asynchronous requests in JAX-RS, inject a reference to a javax.ws.rs.container.AsyncResponse
interface in the JAX-RS resource method as a parameter. The resume
method on the AsyncResponse
object needs to be called from within a separate thread after the business logic execution is complete, as illustrated here:
@Inject
private
Executor
executor
;
@GET
public
void
asyncGet
(
@Suspended
final
AsyncResponse
asyncResponse
)
{
executor
.
execute
(()
->
{
String
result
=
service
.
timeConsumingOperation
();
asyncResponse
.
resume
(
result
);
});
}
WebSocket 1.0
To send asynchronous messages with the WebSocket API, use the getAsyncRemote
method on the javax.websocket.Session
interface. This is an instance of the nested interface of the javax.websocket.RemoteEndpoint
:
public
void
sendAsync
(
String
message
,
Session
session
){
Future
<
Void
>
future
=
session
.
getAsyncRemote
()
.
sendText
(
message
);
}
Concurrency utilities 1.0
The concurrency utilities for Java EE provide a framework of high-performance threading utilities and thus offer a standard way of accessing low-level asynchronous processing.
Servlet 3.1
The servlet specification also allows the use of asynchronous request processing. The parts that need to be implemented are thread pools (using the ExecutorService
), AsyncContext
, the runnable instance of work, and a Filter
to mark the complete processing chain as asynchronous.
Enterprise JavaBeans (EJB) 3.2
Java EE 6 already introduced the javax.ejb.Asynchronous
annotation. This is placed on a Stateless, Stateful, or Singleton EJB to make all the contained methods asynchronous or placed at the method level itself. A method with the @Asynchronous
annotation can either return void
(fire and forget) or an instance of java.util.concurrent.Future
if the asynchronous method result needs to be tracked. This is done by calling the Future.get()
method.
The Missing Pieces
To build a complete and reliable microservices application, you need something more than what typical Java EE servers provide today. Those missing pieces are relevant, and you only have the chance to build them individually or put an infrastructure in place to deliver them. Those pieces are also called “NoOps” or the outer architecture; see Figure 4-2.
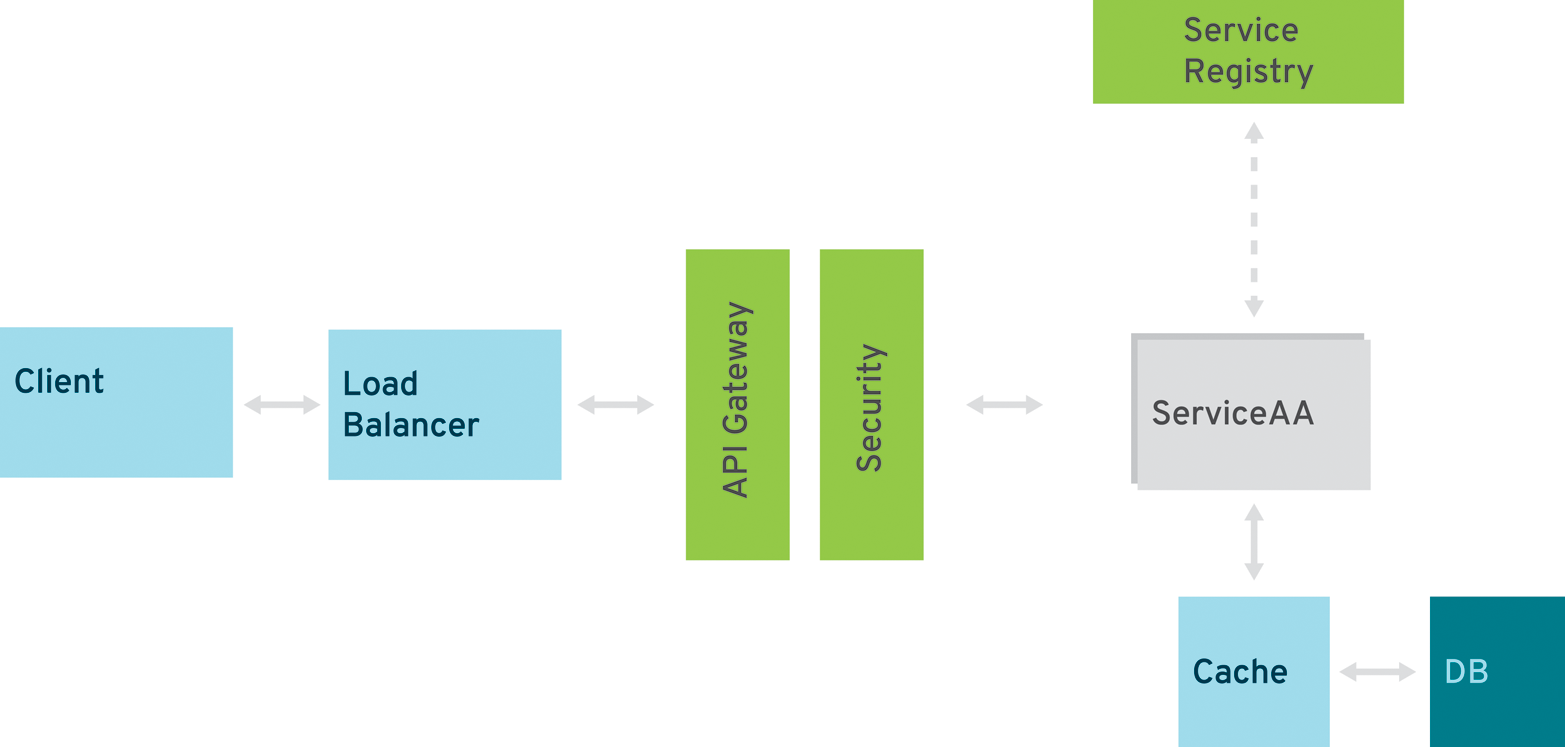
Figure 4-2. Outer architecture for microservices
API Gateway/Management Solution
See “Microservices Best Practices” in Chapter 3 for further information about this solution. API gateway/management is an integral part of any microservices infrastructure.
Service Registry
Multiple microservices are composed to create an application, and each microservice can scale independently. The endpoint of the service may not be known until it’s deployed, especially if it’s deployed in a PaaS. Service registration allows each microservice to register itself with a registry using a logical name. This name is bound to a physical URI and additional metainformation.
By using the logical name, a consumer can locate and invoke the microservice after a simple registry query. If the microservice goes down, then the consumers are notified accordingly or alternative services get returned. The registry should work closely together with the API gateway. There are multiple tools used for service registry and discovery, such as Apache ZooKeper, Consult, etcd, or JBoss APIMan.
Security
In a traditional multitiered server architecture, a server-side web tier deals with authenticating the user by calling out to a relational database or a Lightweight Directory Access Protocol (LDAP) server. An HTTP session is then created containing the required authentication and user details. The security context is propagated between the tiers within the application server so there’s no need to reauthenticate the user.
This is different with microservices because you don’t want to let this expensive operation occur in every single microservices request over and over again. Having a central component that authenticates a user and propagates a token containing the relevant information downstream is unavoidable. Enterprise access management (EAM) systems mostly provide the needed features in an enterprise environment. In addition, some API management solutions also contain security features on top of their government engine. And last but not least, there are dedicated products, like JBoss Keycloak.
Migration Approaches
Putting the discussion in Chapter 3 about greenfield versus brownfield development into practice, there are three different approaches to migrating existing applications to microservices.
Selective Improvements
The most risk-free approach is using selective improvements (Figure 4-3). After the initial assessment, you know exactly which parts of the existing application can take advantage of a microservices architecture. By scraping out those parts into one or more services and adding the necessary glue to the original application, you’re able to scale out the microservices in multiple steps:
-
First, as a separate deployment in the same application server cluster or instance
-
Second, on a separately scaled instance
-
And finally, using a new deployment and scaling approach by switching to a “fat JAR” container
There are many advantages to this approach. While doing archaeology on the existing system, you’ll receive a very good overview about the parts that would make for ideal candidates. And while moving out individual services one at a time, the team has a fair chance to adapt to the new development methodology and make its first experience with the technology stack a positive one.
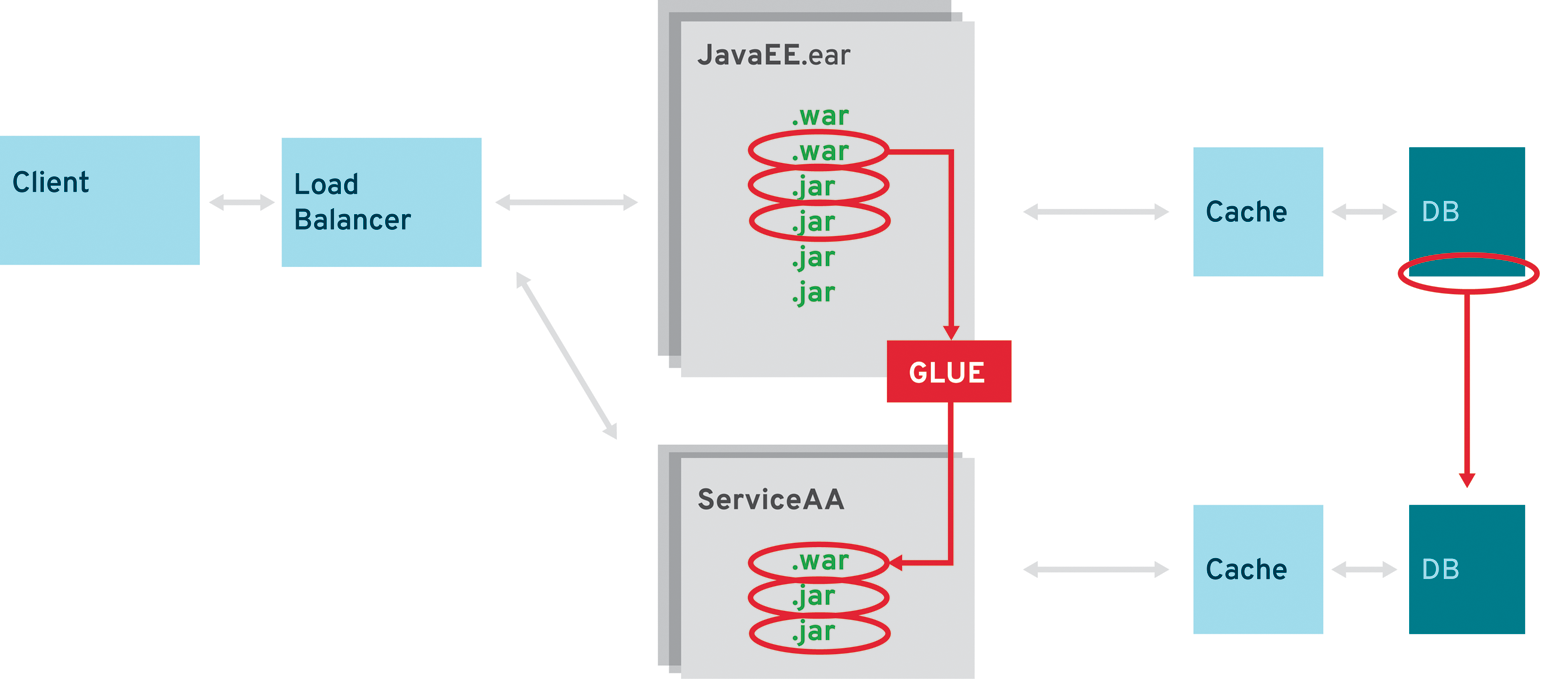
Figure 4-3. Selective improvements
The Strangler Pattern
Comparable but not equal is the second approach where you run two different systems in parallel (Figure 4-4). First coined by Martin Fowler as the StranglerApplication, the refactor/extraction candidates move into a complete new technology stack, and the existing parts of the applications remain untouched. A load balancer or proxy decides which requests need to reach the original application and which go to the new parts. There are some synchronization issues between the two stacks. Most importantly, the existing application can’t be allowed to change the microservices’ databases.
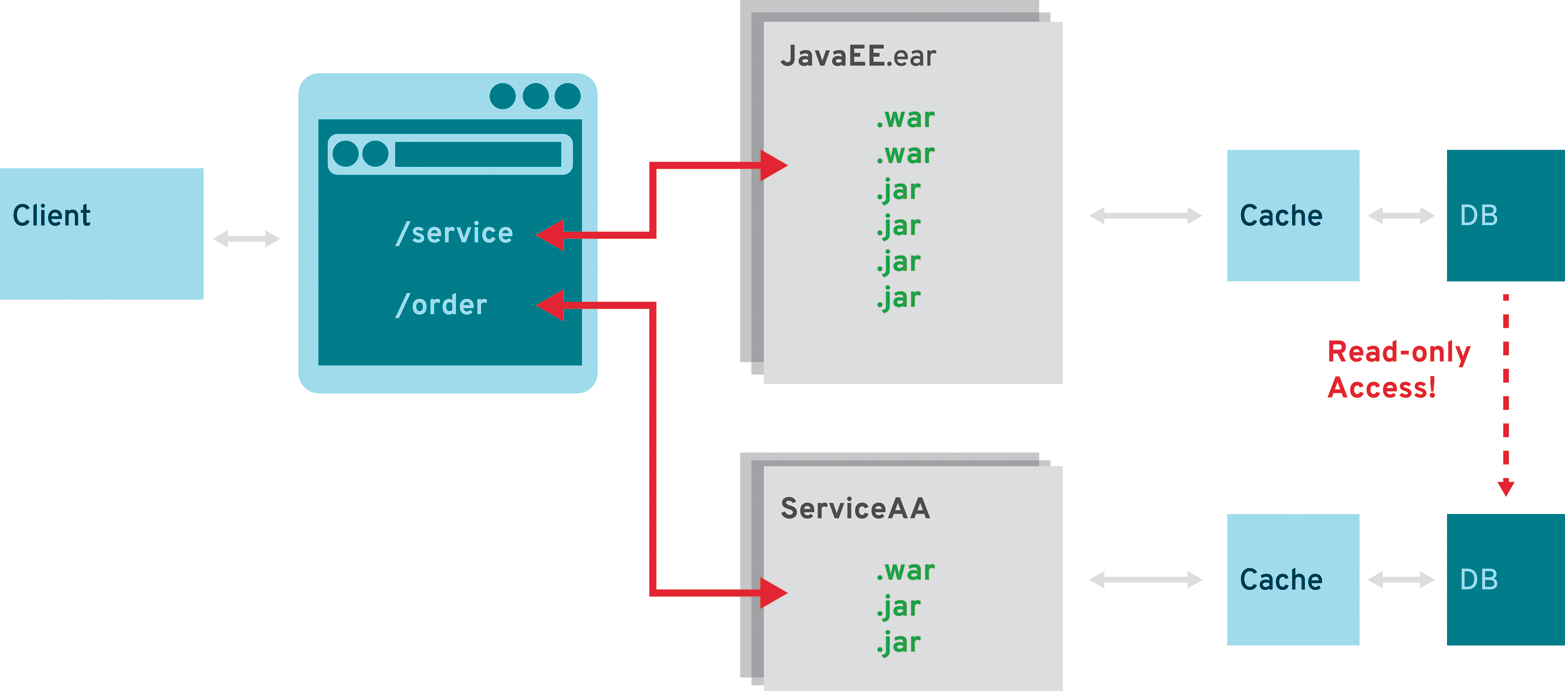
Figure 4-4. Parallel operations: strangler pattern
Big Bang: Refactor an Existing System
In very rare cases, complete refactoring of the original application might be the right way to go. It’s rare because enterprise applications will need ongoing maintenance during the complete refactoring. What’s more, there won’t be enough time to make a complete stop for a couple of weeks—or even months, depending on the size of the application—to rebuild it on a new stack. This is the least recommended approach because it carries a comparably high risk of failure.
Get Modern Java EE Design Patterns now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.