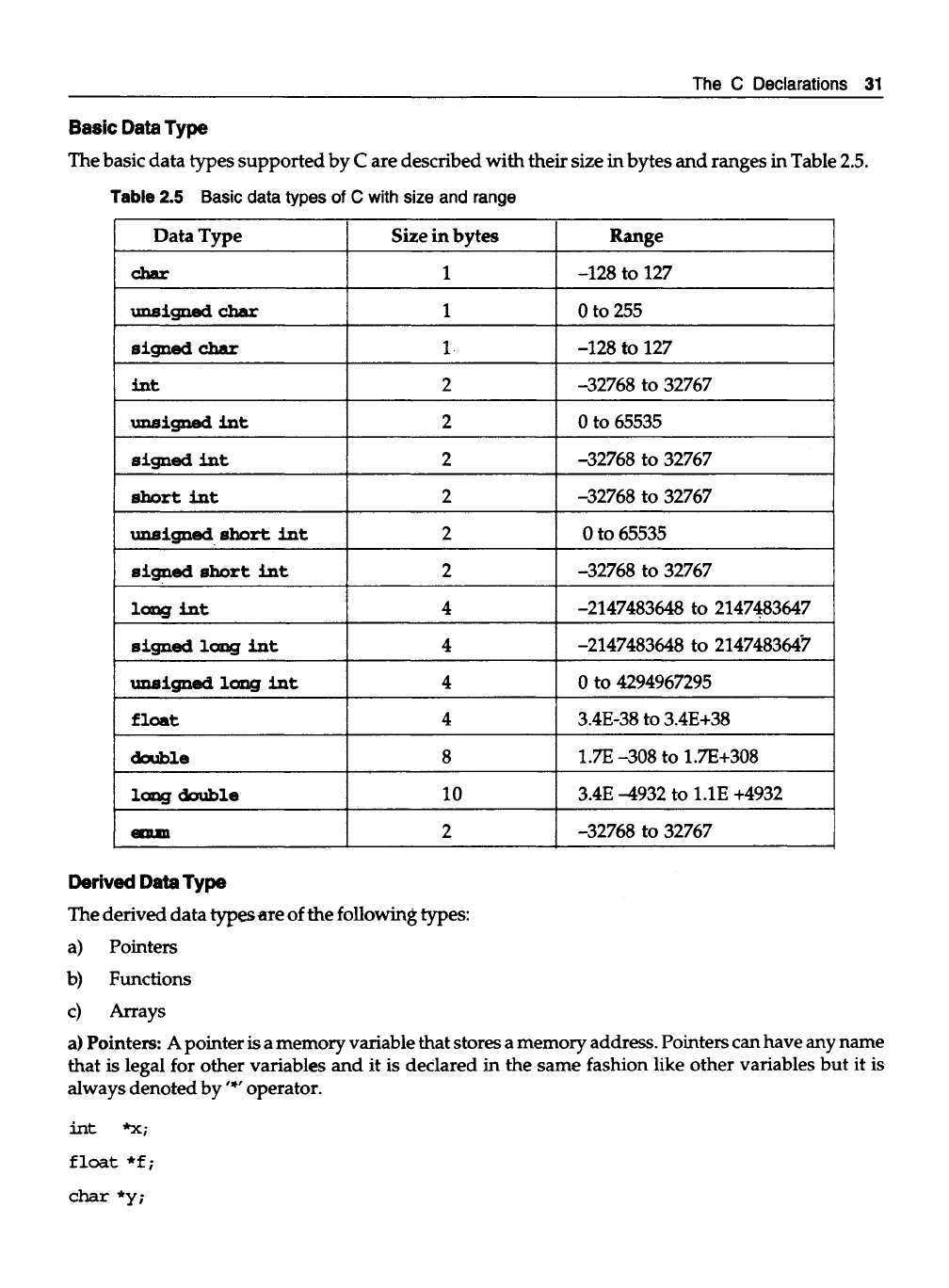
The C Declarations 31
Basic Data Type
The basic data types supported by C are described with their size in bytes and ranges in Table 2.5.
Table 2.5 Basic data types of C with size and range
Data Type
Size in bytes Range
char
1
-128 to 127
unsigned char
1
0 to 255
signed char 1
-128 to 127
int 2 -32768 to 32767
unsigned in t 2 0 to 65535
signed in t 2
-32768 to 32767
short in t 2
-32768 to 32767
unsigned short in t
2 0 to 65535
signed short in t
2 -32768 to 32767
long in t
4 -2147483648 to 2147483647
signed long in t
4 -2147483648 to 2147483647
unsigned long in t
4 0 to 4294967295
float
4 3.4E-38 to 3.4E+38
double
8
1.7E -308 to 1.7E+308
long double
10
3.4E -4932 to 1.1E +4932
enun
2
-32768 to 32767
Derived Data Type
The derived data types are of the following types:
a) Pointers
b) Functions
c) Arrays
a) Pointers: A pointer is a memory variable that stores a memory address. Pointers can have any name
that is legal for other variables and it is declared in the same fashion like other variables but it is
always denoted by operator.
int *x;
float *f ;
char *y;
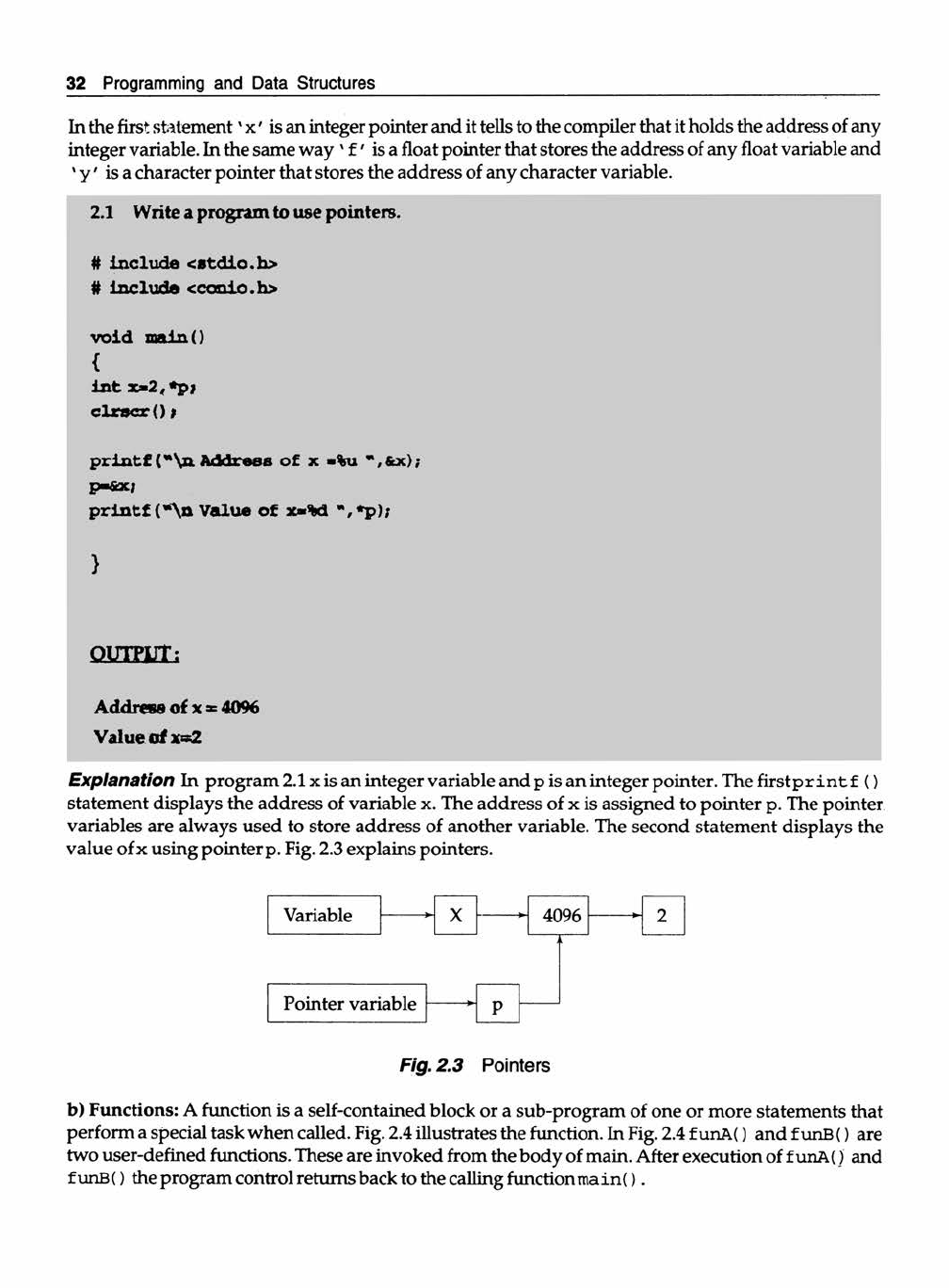
32 Programming and Data Structures
In the first statement' x ' is an integer pointer and it tells to the compiler that it holds the address of any
integer variable. In the same way ' f ' is a float pointer that stores the address of any float variable and
' y ' is a character pointer that stores the address of any character variable.
2.1 Write a program to use pointers.
# include <8tdio.h>
# Include <canio.h>
void xaainO
{
int x»2,*p;
clrscr {) t
print£(m\n Address of x -%u
P - & X f
printf (*\n Value of x«%d *,*p);
OUTPUT;
Address of x * 4096
Valueof x*2
Explanation In program 2.1 x is an integer variable and pis an integer pointer. Thefirstprintf ()
statement displays the address of variable x. The address of x is assigned to pointer p. The pointer
variables are always used to store address of another variable. The second statement displays the
value of x using pointerp. Fig. 2.3 explains pointers.
Fig. 2.3 Pointers
b) Functions: A function is a self-contained block or a sub-program of one or more statements that
perform a special task when called. Fig. 2.4 illustrates the function. In Fig. 2.4 f unA() and f unB () are
two user-defined functions. These are invoked from the body of main. After execution of f unA() and
funBO the program control returns back to the calling function main().

The C Declarations 33
Fig. 2.4 Functions
A simple program on function is described as follows.
22 Write a program to demonstrate user-defined functions.
# Include <conio.h>
# Include <stdio.h>
void m&in()
{
clrscr ();
void show (void);
show ();
}
void show()
{
printf ("\n In function 8ho*r()");
I
OUTPUT:
In function show ()
Explanation In the above program the function show () is defined. The function body contains only
one p rin t f () statement. When the function is executed a message is displayed.
c) Arrays: An array is a collection of elements of similar data types in which each element is located in
separate memory location. For example,
in t b [4];
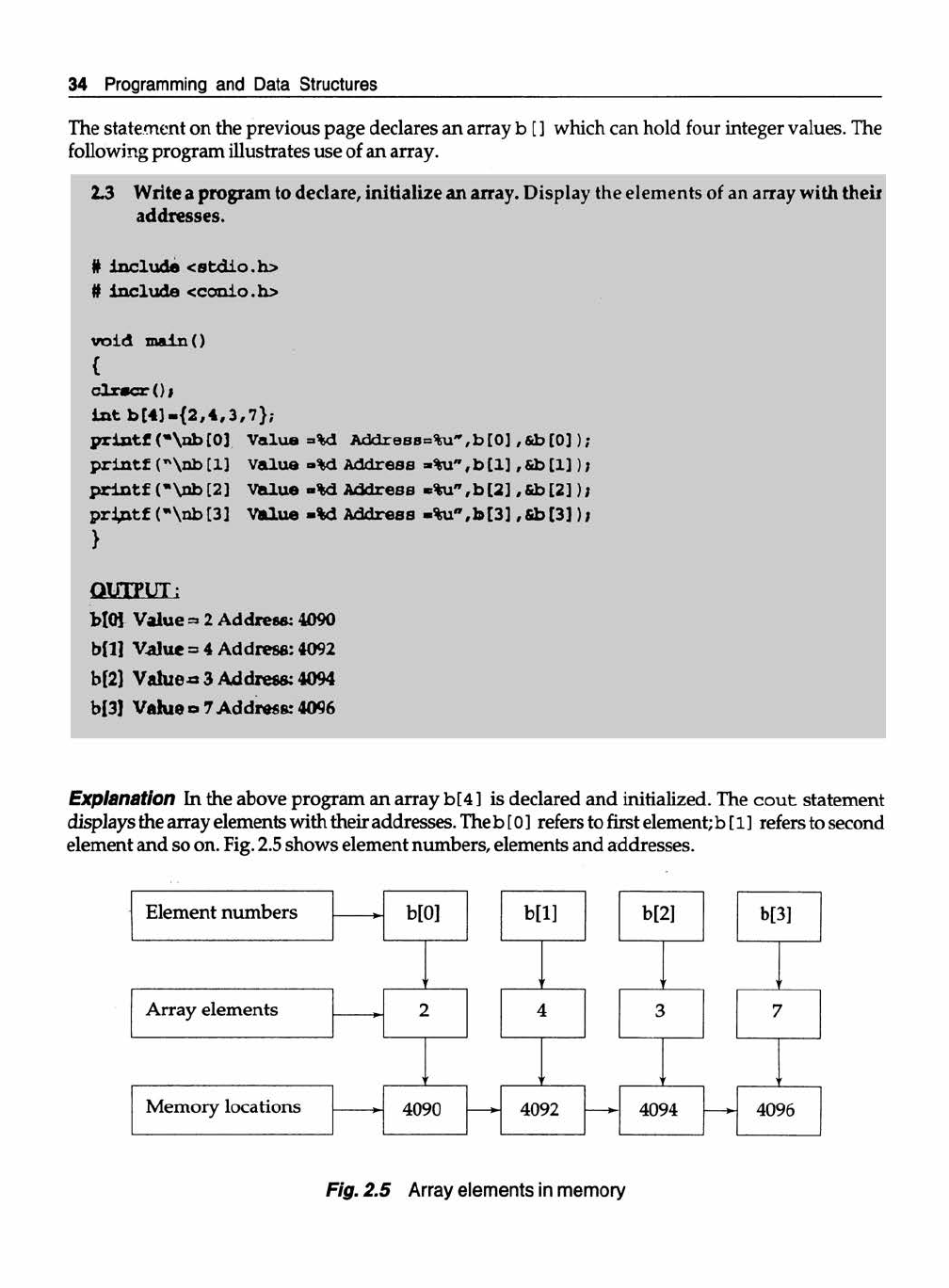
34 Programming and Data Structures
The statement on the previous page declares an array b [ ] which can hold four integer values. The
following program illustrates use of an array.
13 Write a program to declare, initialize an array. Display the elements of an array with their
addresses.
# include <stdio.h>
# include <conio.h>
void main ()
{
clrecr () j
int b[4J«{2,4,3,7};
printf (m\nb[0] Value =%d Address=%u#r ,b [0] ,&b[0]);
printf (*\nb [1] Value »%d Address »%u",b[l] ,&b[l]);
printf ("\nb [2] Value «%d Address *%u",b[2] ,&b[2]);
pri^xtf ("\nb[3] Value »%d Address -%u",b[3] ,&b[3])/
>
o m r y ji
1>I0} Value => 2 Address: 4090
b(l] Value = 4 Address: 4092
b[2) Value-a 3 Address: 4094
b(3] Value o 7 Address: 4096
Explanation In the above program an array b[4 ] is declared and initialized. The cout statement
displays the array elements with their addresses. The b [ 0 ] refers to first element; b [ 1 ] refers to second
element and so on. Fig. 2.5 shows element numbers, elements and addresses.
Fig. 2.5 Array elements in memory
Get Programming and Data Structures now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.