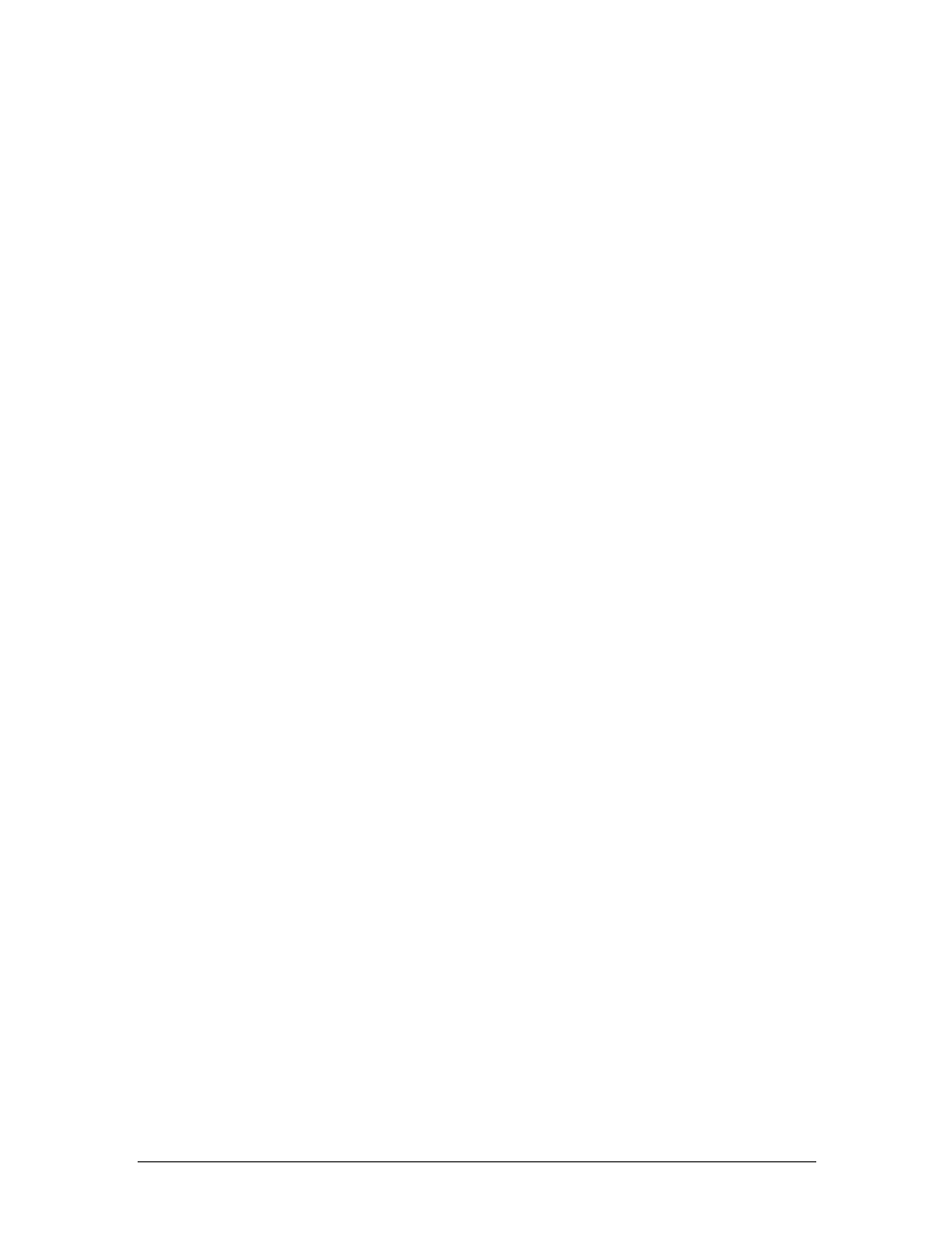
This is the Title of the Book, eMatter Edition
Copyright © 2007 O’Reilly & Associates, Inc. All rights reserved.
Listenable Shared Objects
|
485
UIComponent class, implements many of the core UIComponent methods, and uses
the v2-style setter methods. The SimplePeopleList component has two shortcomings
that will be addressed later in this chapter: it doesn’t provide a way to show the con-
nection status of users, and it relies on code in the main.asc file to populate a shared
object. The PeopleList and Status components developed later provide these fea-
tures.
Listenable Shared Objects
The SimplePeopleList component provides a view of the data in a userList shared
object by listing the shared object’s
data properties. Other components may also
need to use the
userList shared object and be notified when the onSync( ) method is
called. In one respect, the SimplePeopleList treats the userList shared object as it
treats its List component; it simply adds itself as an event listener for onSync events.
Remote shared objects must be modified in order to dispatch events or add and
remove event listeners. When an object or component in this chapter needs to con-
nect to a shared object, it uses the SharedObjectFactory class listed in Example 13-2
instead of the SharedObject class. The SharedObjectFactory class retrieves a remote
shared object and then adds methods and properties to it so that one or more objects
or components can add themselves to the shared object as event listeners. It uses the
EventDispatcher class provided as part of the v2 UI component framework to per-
form part of the customization of each shared object. The code in Example 13-2
must be placed in a file named SharedObjectFactory.as. Read through the heavily
commented example if you are interested in how the SharedObjectFactory converts a
remote shared object into a v2-style event dispatcher.
Example 13-2. The SharedObjectFactory class
class SharedObjectFactory extends Object {
/* getRemote( ) calls SharedObject.getRemote( ) to get a shared object reference
* and then uses the mx.events.EventDispatcher object to make the shared
* object into a v2 component–style event broadcaster. Also, an isConnected
* flag is maintained to implement an onFirstSync event and to keep track of
* the shared object's connection state. Finally, two methods have been added
* to dynamically add and remove remote methods to and from the shared object.
*/
static function getRemote (name, uri, persistence) {
// Get the remote shared object.
var so = SharedObject.getRemote.apply(SharedObject, arguments);
// If it exists and has not been modified to be a broadcaster...
if (so && !so._onFirstSync) {
// Make it an event broadcaster.
mx.events.EventDispatcher.initialize(so);
// Define an _onFirstSync( ) method that can be assigned to onSync
// whenever an attempt is made to connect the shared object.
so._onFirstSync = function (list:Array) {
this.isConnected = true;
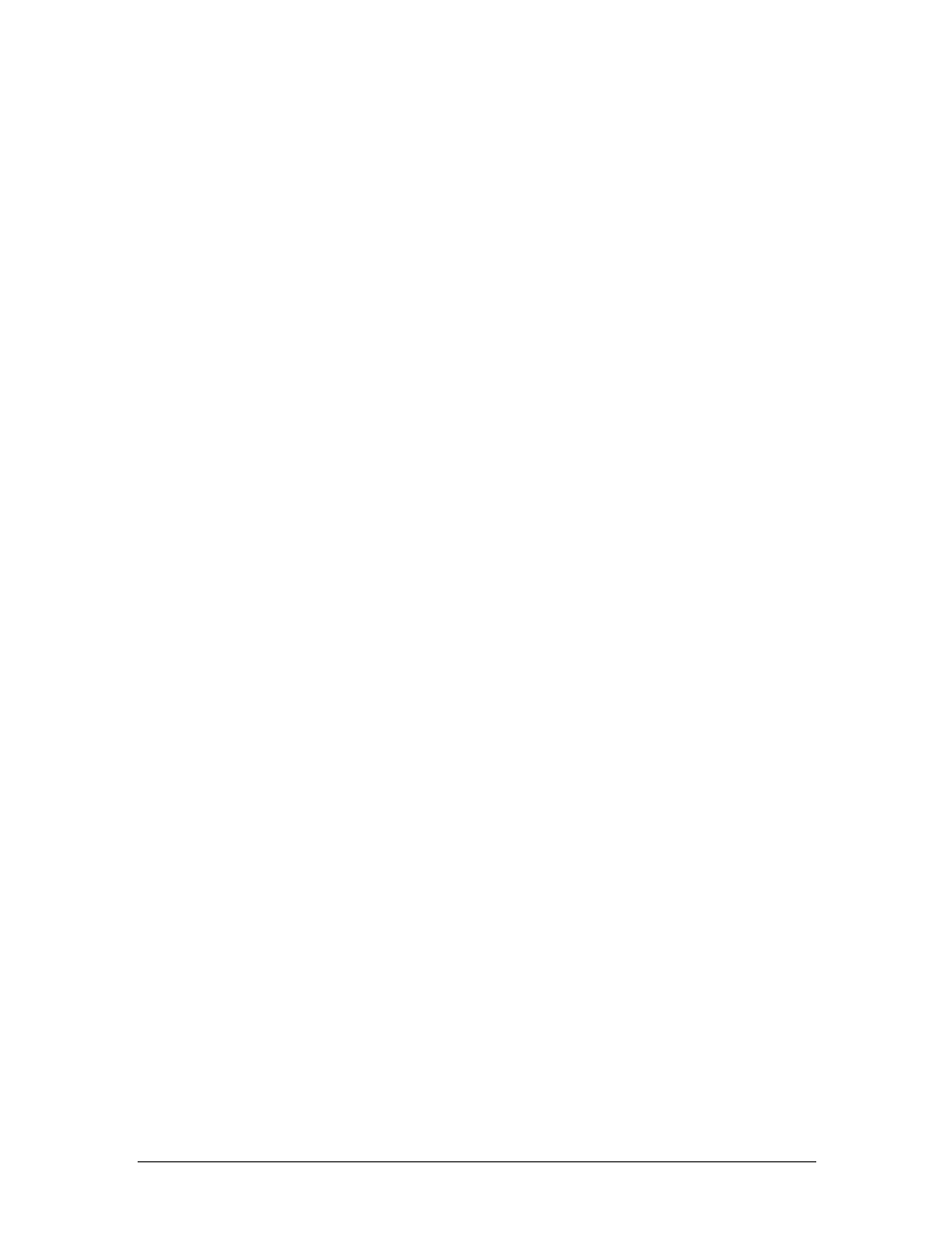
This is the Title of the Book, eMatter Edition
Copyright © 2007 O’Reilly & Associates, Inc. All rights reserved.
486
|
Chapter 13: Building Communication Components
If you use the SharedObjectFactory class in place of the SharedObject class to get a
remote shared object, you should never have to customize another shared object
again. All you have to do is get the shared object with SharedObjectFactory.
this.dispatchEvent({type:"onFirstSync", target: this, list:list});
this.onSync = this._onSync;
this.onSync(list);
};
/* Define an _onSync( ) method to assign to onSync after the first
* onSync( ) has been called. Note the event.list property contains
* the list normally passed to onSync( ).
*/
so._onSync = function (list:Array) {
this.dispatchEvent({type:"onSync", target: this, list:list});
};
// Keep a reference to the SharedObject.connect( ) method.
so._connect = so.connect;
// Replace connect( ) with a method that modifies onSync and isConnected
// before calling the original connect( ) method.
so.connect = function (nc:NetConnection) {
this.onSync = this._onFirstSync;
this.isConnected = false;
this._connect(nc);
};
// Keep a reference to the original SharedObject.close( ) method.
so._close = so.close;
// Modify the close( ) method to set the isConnected flag before
// actually closing the shared object.
so.close = function ( ) {
this.isConnected = false;
this._close( );
};
/* Dynamically attach a remote method to the shared object. Note
* the method simply dispatches an event to any interested listeners
* and includes the arguments object so listeners can deal with the
* remote call themselves. Listeners must use addEventListener( ) on the
* shared object to receive remote method call notifications.
* addRemoteMethod( ) and removeRemoteMethod( ) are discussed later.
*/
so.addRemoteMethod = function (methodName) {
so[methodName] = function ( ) {
this.dispatchEvent({type:methodName, target: this, args:arguments});
};
};
// Delete the remote method if it is no longer needed.
so.removeRemoteMethod = function (methodName) {
delete so[methodName];
};
}
return so;
}
}
Example 13-2. The SharedObjectFactory class (continued)
Get Programming Flash Communication Server now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.