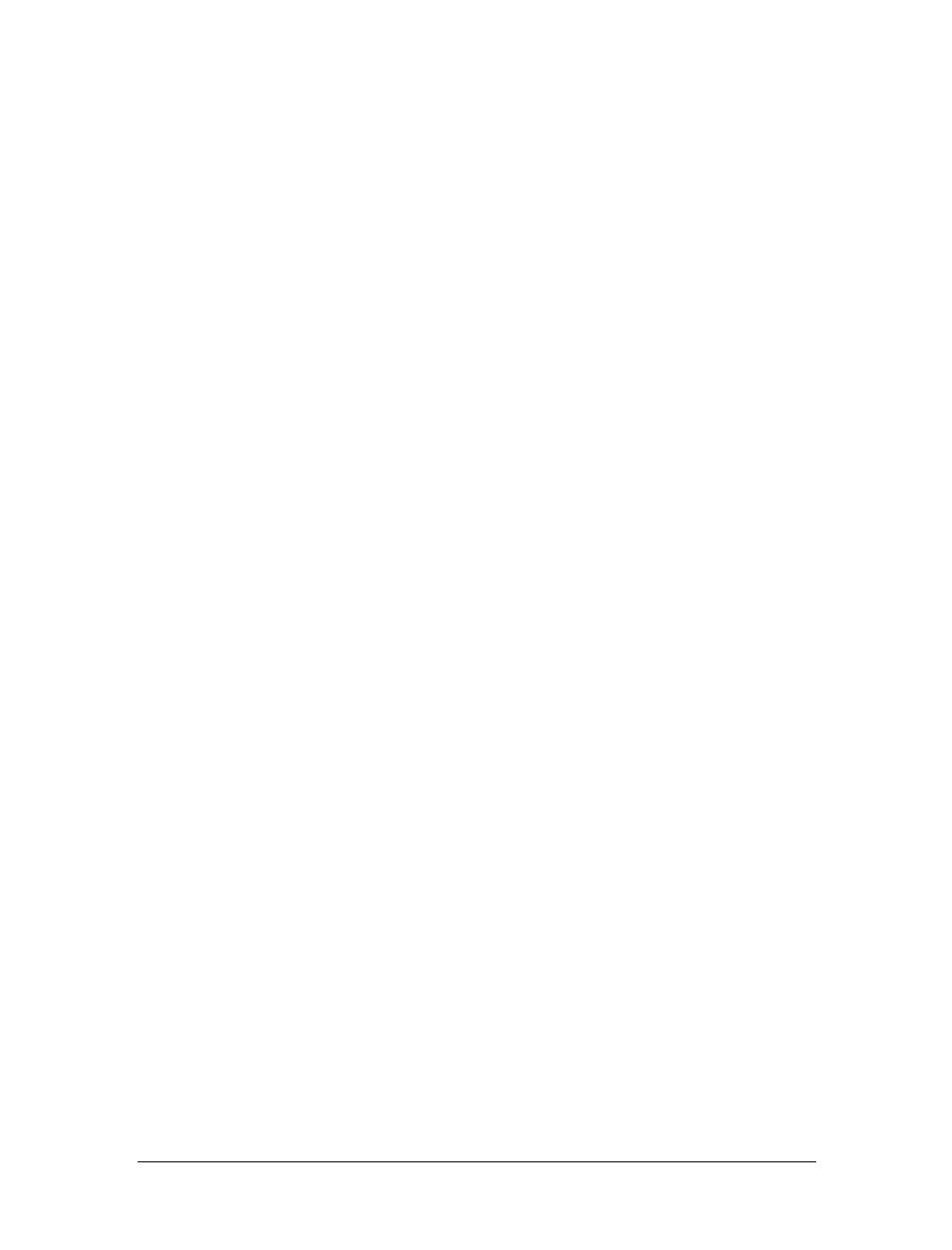
This is the Title of the Book, eMatter Edition
Copyright © 2007 O’Reilly & Associates, Inc. All rights reserved.
Creating New Transport Modules
|
179
applications built using the class. The sample TCP server’s handle method acted as
an infinite loop, where the POP3
handle was wrapped in an explicit loop construct.
It is within the
handle method that the server object is expected to retrieve one or
more requests, using the protocol it is written to support. In many cases, once the
request has been read and stored into memory, it is usually directly usable by the
handle method of the superclass. Many of the derived server classes simply call the
SOAP::Server handle method once they’ve read the request:
my $response = $self->SUPER::handle($request);
Once the response is given back, it is then up to the new code to properly transmit it
back to the client. This is assuming that a client response is part of the design; the
POP3 server doesn’t send a client reply because there is no client connection avail-
able to it.
Other server methods
There are other methods within SOAP::Server that may be overloaded, not to men-
tion hooks such as
on_dispatch. The documentation for SOAP::Lite and Appendix B
of this book may be of help in such tasks.
Writing a Client Transport
Writing an actual new client transport module is trickier than writing a server com-
ponent. The
WishListCustomer::Client class developed earlier in this chapter isn’t an
actual transport class. It was a subclass of
SOAP::Lite itself, designed to interject a
consistent header element into every outgoing request.
A class intended for use as a client’s transport object is very different.
Naming the client class
The name of a client class is defined by the protocol itself and the transport class it is
expected to work with. All the client classes provided by
SOAP::Lite have names that
fit the following form:
${transport_class}::${protocol_uc}::Client
By default, SOAP::Lite objects have a transport object instantiated from the SOAP::
Transport
class. Using Jabber as an example, the class name becomes:
SOAP::Transport::JABBER::Client
SOAP::Lite attempts to load protocol code using the transport object’s class name
and the uppercased protocol string. Keeping with the ongoing example, this means
that when a client tries to set a proxy with a protocol specification of
jabber://,
SOAP::Lite (specifically, the proxy method of SOAP::Transport) tries to load a pack-
age called
SOAP::Transport::JABBER, and expects a client class called SOAP::
Transport::JABBER::Client
within it.
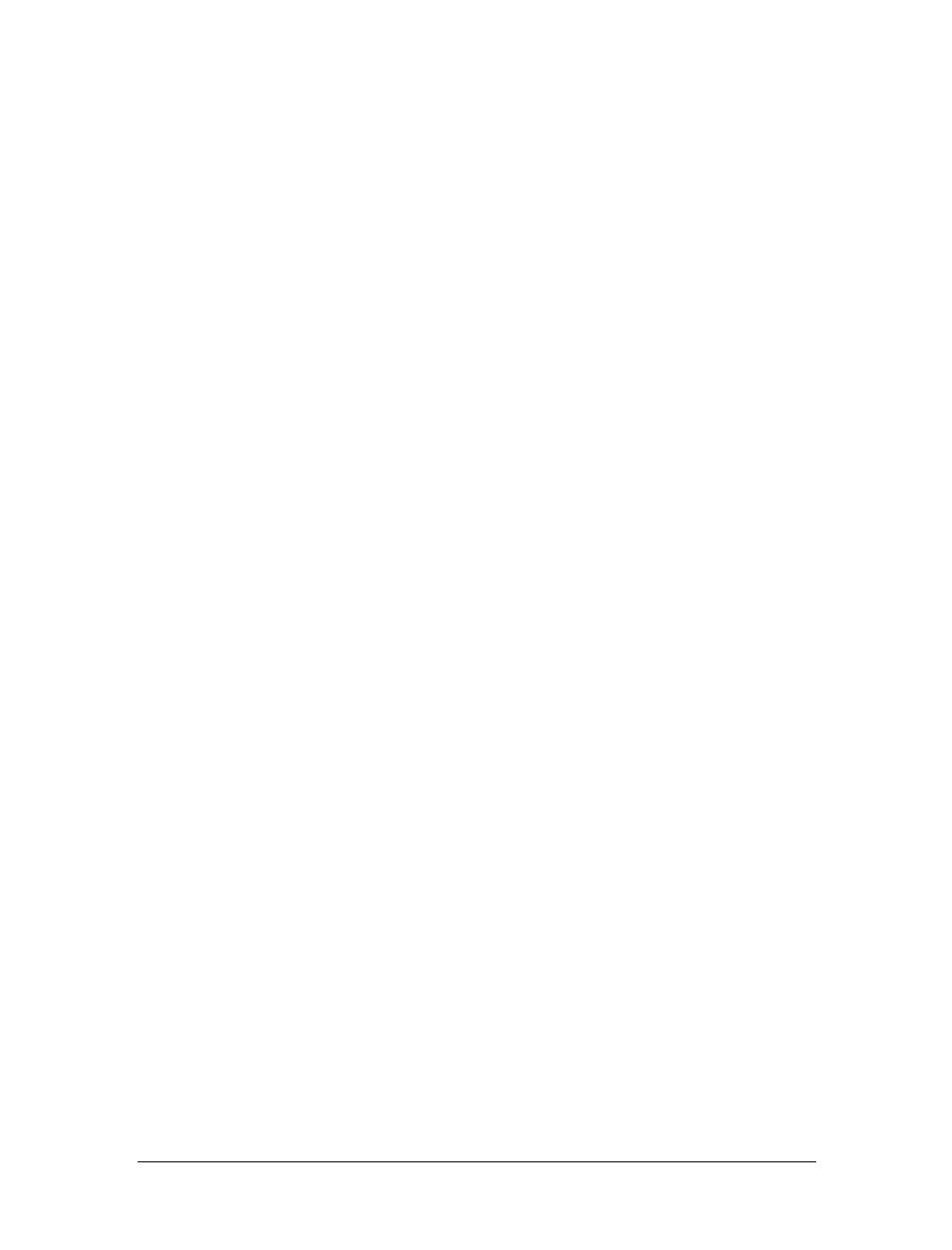
This is the Title of the Book, eMatter Edition
Copyright © 2007 O’Reilly & Associates, Inc. All rights reserved.
180
|
Chapter 8: SOAP Services Without HTTP
This becomes important when choosing the name for the new client class. To work
with the loading mechanism already in place, the class has to either use
SOAP::
Transport
as the leading elements of its name or offer a different transport class that
can be plugged in to the
SOAP::Lite object.
Subclassing
SOAP::Transport isn’t difficult. The constructor isn’t designed to take any
arguments when called, and the only class method provided is
proxy, which is
responsible for either returning the current proxy object (when no arguments are
passed to it) or setting a new one (when given an endpoint string as an argument).
An empty subclass can provide the base elements of the class name for specifying a
transport.
Constructors
A client transport class is expected to inherit from either SOAP::Client or some other
subclass of it. As such, the client class may not need to overload the
new method at
all. As with the server class details earlier, there are plenty of cases in which a client
might subclass an existing transport class just to overload a different method.
In another similarity to the server classes, a client
new should build up from an object
created by calling the superclass
new method. These objects are also based on hash-
table references, so the new client class should design for this.
When a constructor is called, it’s usually called by the
proxy method of a transport
object. Such calls start with two parameters in the list of arguments: the string
endpoint followed by the endpoint itself as passed to the proxy method. Any other
arguments to the
proxy method follow these two. The general design is such that
arguments are expected to be name/value pairs that get converted to a hash table.
The key
endpoint is just one element of the hash table. If the new class constructor is
expected to function in tandem with
SOAP::Transport, this behavior must be
supported.
The send_receive method
Just as handle is the core of a server class, the send_receive method is the core of a
client-transport class. This method is called when the
SOAP::Lite object has turned
an ordinary method call into a serialized request.
When called, the
send_receive method receives a hash table with four keys:
action
The “action” specifier for the request, usually the SOAPAction header in an HTTP
environment.
encoding
The SOAP encoding URN, often dependent on the version of SOAP that serial-
izes the request.
Get Programming Web Services with Perl now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.