Chapter 2. The Life of a Component
Now that you know how to use the ready-made DOM components, it’s time to learn how to make some of your own.
Bare Minimum
The API to create a new component looks like this:
var
MyComponent
=
React
.
createClass
({
/* specs */
});
The “specs” is a JavaScript object that has one required method called render()
and a number of optional methods and properties. A bare-bones example could look something like this:
var
Component
=
React
.
createClass
({
render
:
function
()
{
return
React
.
DOM
.
span
(
null
,
"I'm so custom"
);
}
});
As you can see, the only thing you must do is implement the render()
method. This method must return a React component, and that’s why you see the span
in the snippet; you cannot just return text.
Using your component in an application is similar to using the DOM components:
ReactDOM
.
render
(
React
.
createElement
(
Component
),
document
.
getElementById
(
"app"
)
);
The result of rendering your custom component is shown in Figure 2-1.
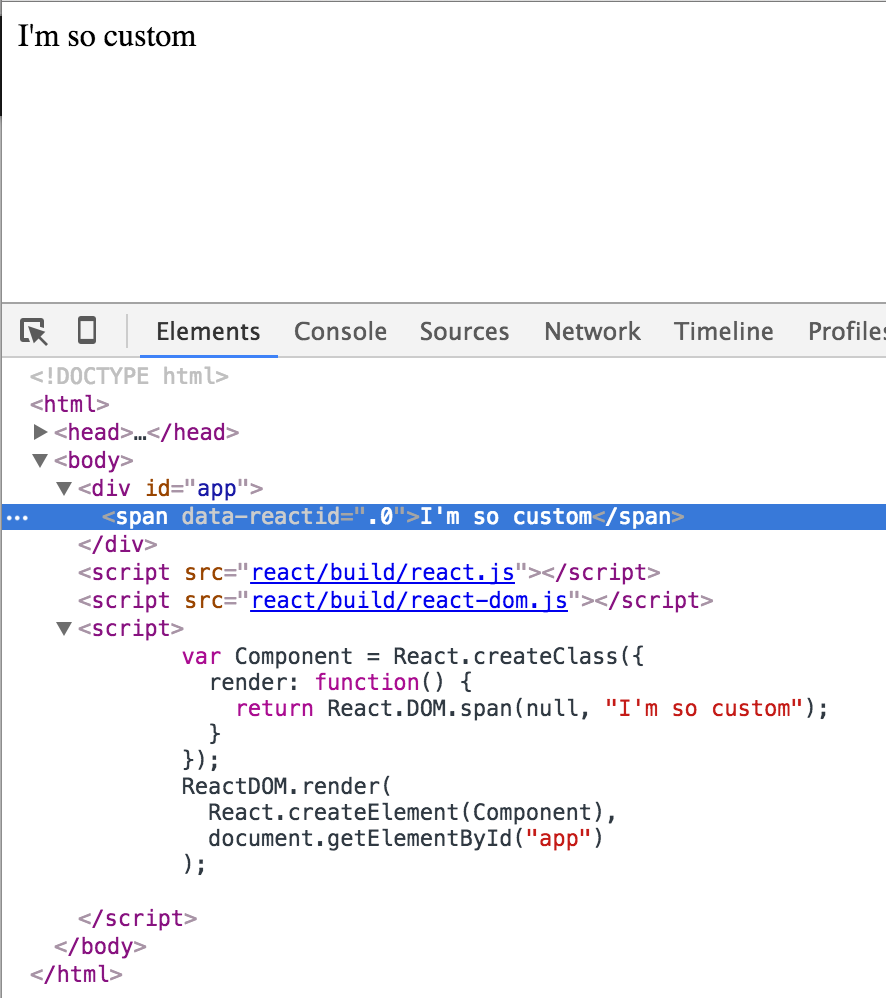
Figure 2-1. Your first custom component
React.createElement()
is one way to create an “instance” of your component. Another way, if you’ll be creating several instances, is to use a factory:
var
ComponentFactory
=
React
.
createFactory
(
Component
);
ReactDOM
.
render
(
ComponentFactory
(),
document
.
getElementById
(
"app"
)
);
Note that the React.DOM.*
methods you already know of are actually just convenience wrappers around ...
Get React: Up & Running now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.