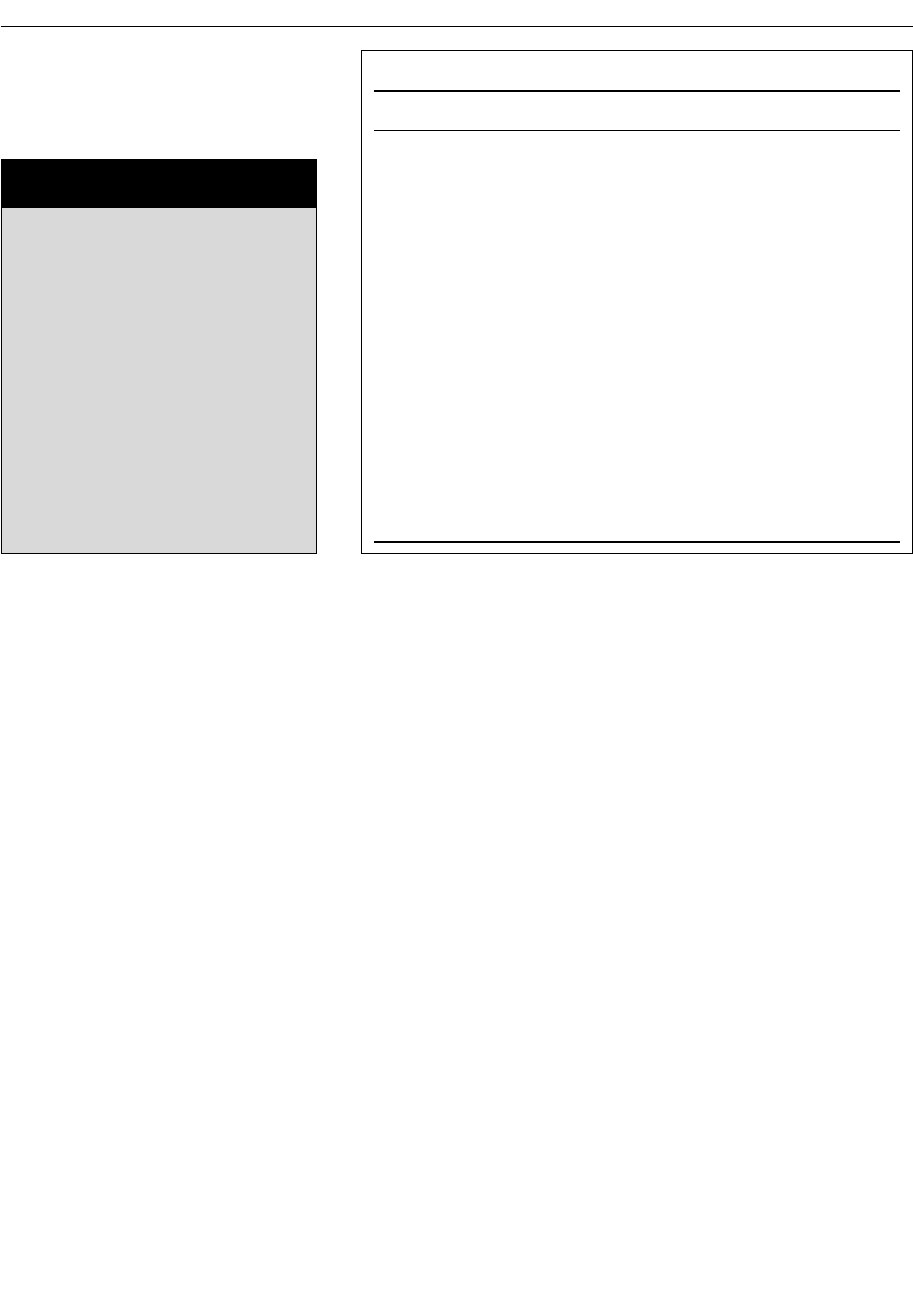
For anything larger than the division tester program (page 67), a pro-
gram should be divided into functions. A function has a name, argu-
ments and a return type, and it appears in three ways:
1. Before it can be called, a function must be prototyped. This is analo-
gous to declaring a variable before using it. Prototyping is accom-
plished by a line of the form:
return_type function_name(arg1_type arg1_name,…argn_type
argn_name);
(The names of arguments are not strictly necessary in a prototype:
including them is sometimes helpful for debugging.)
Prototyping is usually done at the top of the program, or in a
header file.
2. The function must be defined, that is, its statements must be written
out. The function definition is of the form:
return_type function_name(arg1_type arg1_name,…
argn_type argn_name)
{
statements of function
}
(If the function is defined before its first call, then it does not have
to be prototyped. Even so, as a rule, prototyping everything is good
practice.)
3. Function calls (of which there may be many) are typically of the form:
variable = function_name(value1, value2,… valuen);
though assignment of the return value to a variable is not necessary.
70 Software Design for Engineers and Scientists
Table 4.3 Type conversion
Type Converts to
First, C does these conversions:
char int
unsigned char int
signed char int
short int
unsigned short unsigned int
enum int
float double
Then it goes through the following list, and if either of the operands
has the specified type, it converts the other operand to the same type.
long double
double
float
unsigned long
long
unsigned int
More on casts
The process of converting one type
to another (in this case, an
int to a
double) can be accomplished, as
here, with an explicit cast. C also
provides more nuanced control over
type conversions with
static_cast,
reinterpret_cast, const_cast, and,
for handling derived types,
dynamic_cast. These are not cov-
ered in this book. Because they
cover the different uses of casting,
the conventional cast is sometimes
deprecated, but its use is still wide-
spread, and for converting between
built-in types, perfectly acceptable.
4.8 Functions
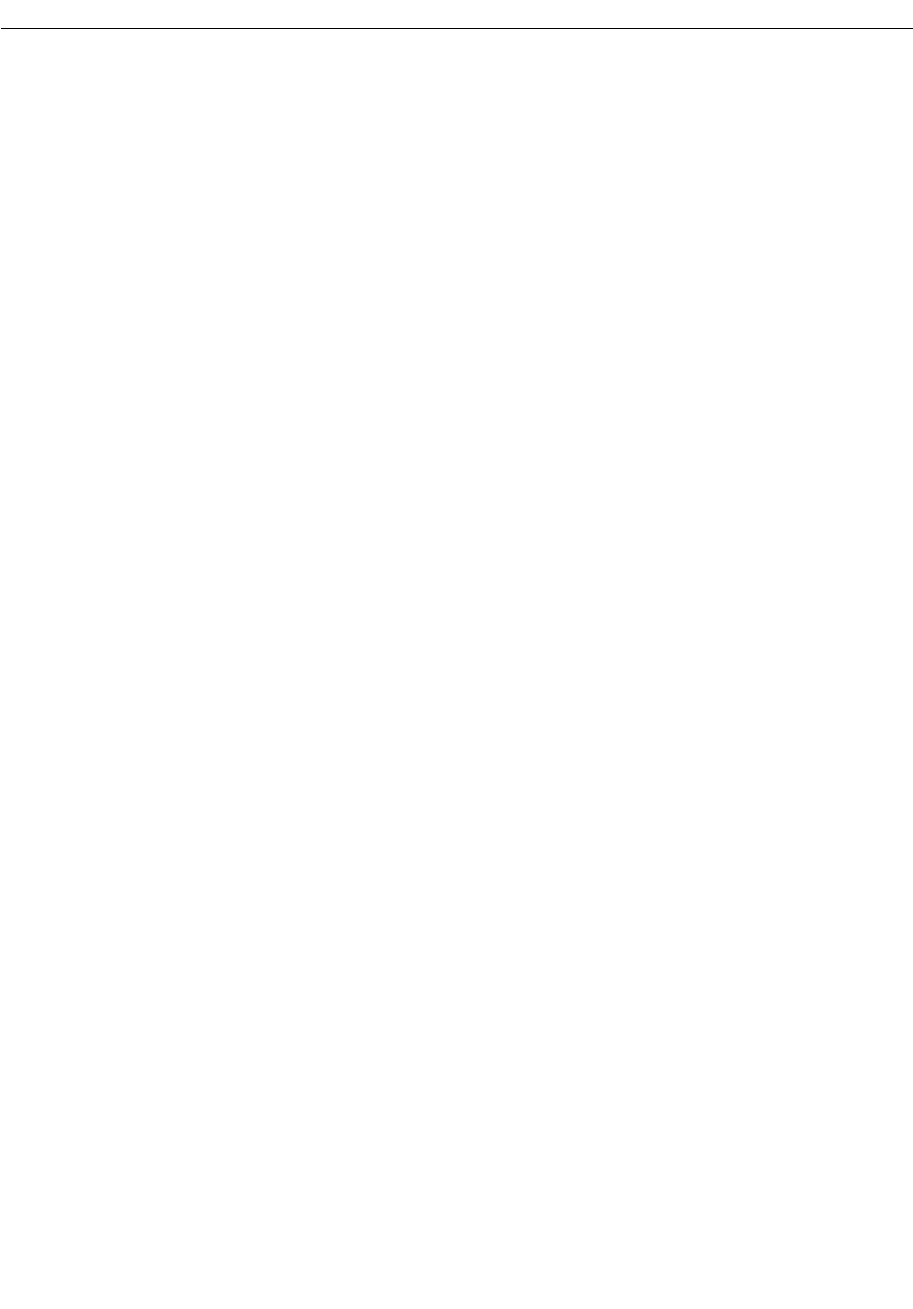
Here is an example that uses a function to raise an integer to a positive
integer power.
1: // Raise an integer to a positive integer power
2: #include <iostream>
3: using namespace std;
4: int ipower(const int num, const int exp); // Prototype
5:
6: int main()
7: {
8: int a, b;
9: cout << "Enter two integers: ";
10: cin >> a >> b;
11: cout << a << " to the power of " << b << " is " <<
ipower(a,b) << '\n';
12: return 0;
13: }
14: int ipower(const int num, const int exp)
15: {
16: int value = 1;
17: for (int cnt = 1; cnt <= exp; cnt++)
18: value *= num;
19: return value;
20: }
Here ipower is the programmer’s own function, being called from main.
Before ipower is even used, right at the top of the program, on line 4,
it is prototyped. The prototype shows how many arguments are passed,
what their types are and what the return type is. ipower is a function of
two integers and returns an integer.
int ipower(const int num, const int exp); // Prototype
The consts in the prototype mean that the function ipower() will not
change the values of its parameters.
Within main, on line 11, ipower is called by naming it:
ipower(a,b)
Note that the names used for arguments in a function do not have to
agree with those used by the caller. But the types have to agree.
Below main, from line 14 to line 20, the actual definition of ipower
is given. The first line:
int ipower(const int num, const int exp)
is almost identical to the prototype, but is followed by the function
itself (between { and }) rather than a semicolon.
The value returned by a function is specified using the return
statement as on line 19. A return may happen from anywhere within
a function; it does not have to be the last statement. Of course, the
returned type must agree with the prototype. In this case an int has to
be returned.
Raising a number to a power is an example of a function that could
be implemented recursively. A recursive alternative to the ipower()
Beginning programming in C 71
Get Software Design for Engineers and Scientists now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.