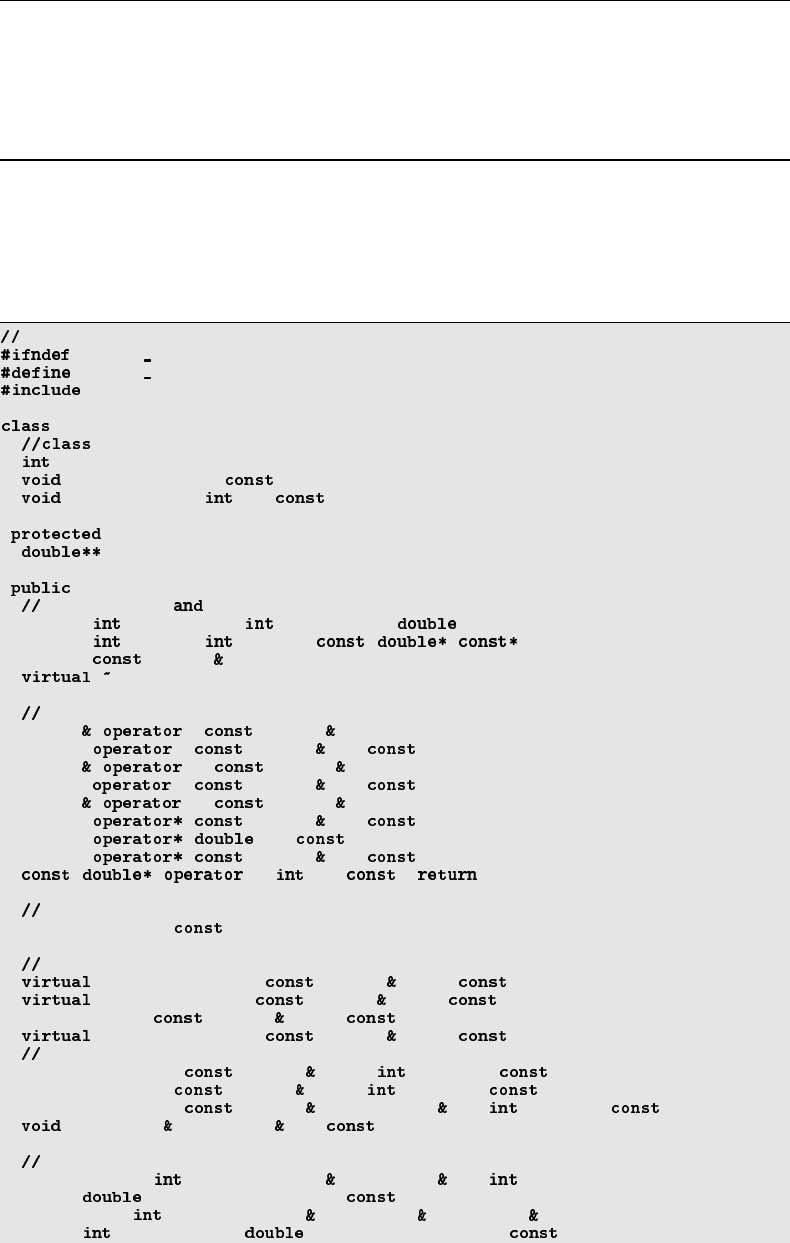
APPENDIX D
The Matrix and Vector classes
The “complete” versions of the classes Matrix and Vector that were used, e.g., in Chapters
3 and 7 are given in this appendix. We begin with the class Matrix header file.
Listing D.1 matrix.h
matrix.h
MATRIX H
MATRIX H
"vector.h"
Matrix{
members
nRows , nCols ;
pointerCheck () ;
pointerCheck( i) ;
:
pA ;
:
constructors destructor
Matrix( nrows = 0, ncols = 0, a=0.);
Matrix( nrows , ncols , pa );
Matrix( Matrix A);
Matrix ();
overloaded operators
Matrix =( Matrix B);
Matrix +( Matrix B) ;
Matrix +=( Matrix B);
Matrix -( Matrix B) ;
Matrix -=( Matrix B);
Matrix ( Matrix B) ;
Matrix ( b) ;
Vector ( Vector v) ;
[]( i) { pA [ i];}
matrix operations
Matrix trans () ;
solution of a linear system
Vector backward( Vector RHS ) ;
Vector forward ( Vector RHS ) ;
Vector gauss( Vector RHS ) ;
Vector cholesky( Vector RHS ) ;
banded systems
Vector bandBack( Vector RHS , bWidth) ;
Vector bandFor ( Vector RHS, bWidth) ;
Vector bandChol( Vector RHS , M atr ix G, bWidth) ;
QR ( Mat rix Q, Matrix R) ;
computation of spectra
Vector eigen( Nvals , Vector v, Matrix U, itMax = 38,
delta = .00000001) ;
Vector SVD( Nvals , Vector v, Matrix U, Matrix V,
itMax = 38, delta = .00000001) ;
511
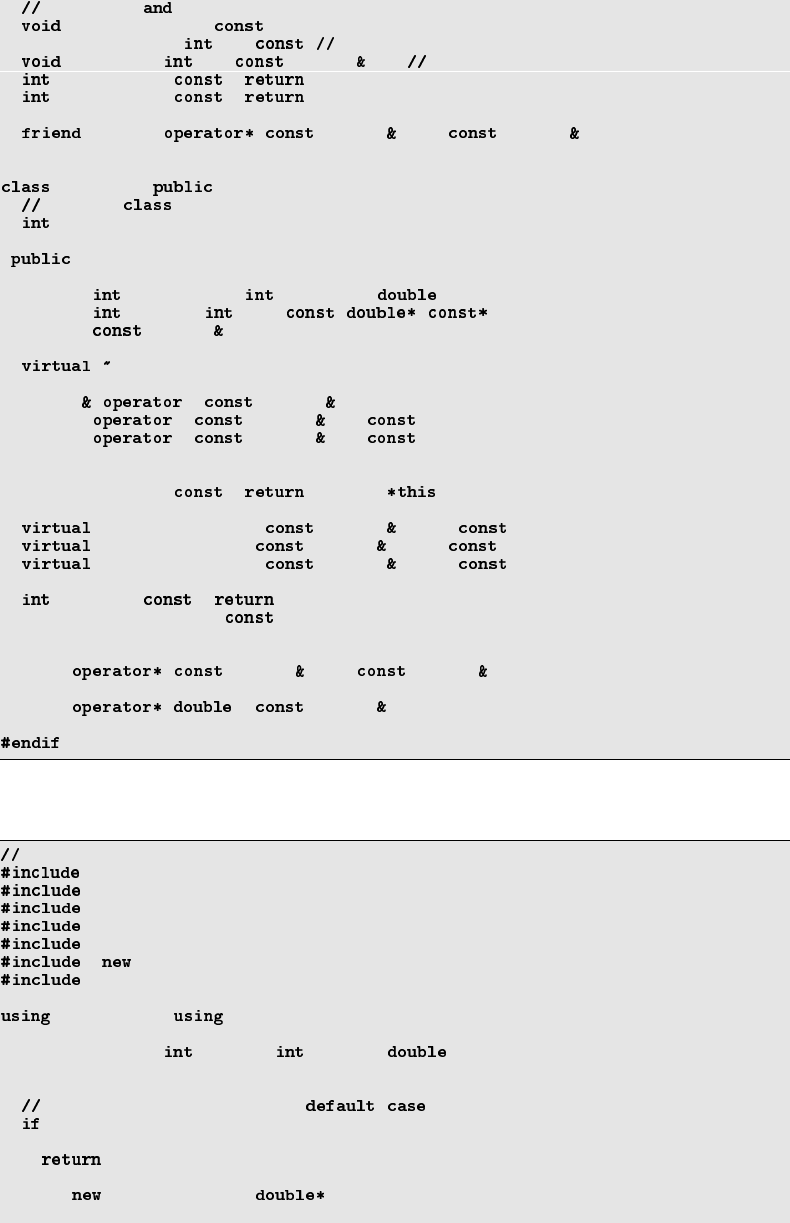
512 THE MATRIX AND VECTOR CLASSES
utilities accessors
printMatrix () ;
Vector matToVec( j) ; jth column to a vector
vecToMat( j, Vector v); vector into jth column
getnRows () { nRows ;}
getnCols () { nCols ;}
Matrix ( Vector v1, Vector v2 );
};
pdBand : Matrix {
derived member
bW ;
:
pdBand( nrows = 0, bw = -1, a=0.);
pdBand( nrows , bw , pa );
pdBand( pdBand A);
pdBand (){};
pdBand =( pdBand B);
pdBand +( pdBand B) ;
Matrix +( Matrix B) ;
pdBand trans () { pdBand( );}
Vector backward( Vector RHS ) ;
Vector forward ( Vector RHS ) ;
Vector cholesky( Vector RHS ) ;
getBW () { bW ;}
Matrix bandToFull () ;
};
Matrix ( Vector v1, Vector v2 );
Matrix ( , Matrix A);
The metho d definitions are then provided by
Listing D.2 matrix.cpp
matrix.cpp
<algorithm>
<iostream>
<cmath >
<cstdlib>
<limits >
< >
"matrix.h"
std :: cout; std:: endl ;
Matrix :: Matrix ( nrows , ncols , a){
nRows = nrows;
nCols = ncols;
set pA to null pointer in
(nRows == 0 || nCols == 0){
pA = 0;
;
}
pA = (std::nothrow) [nRows];
pointerCheck ();
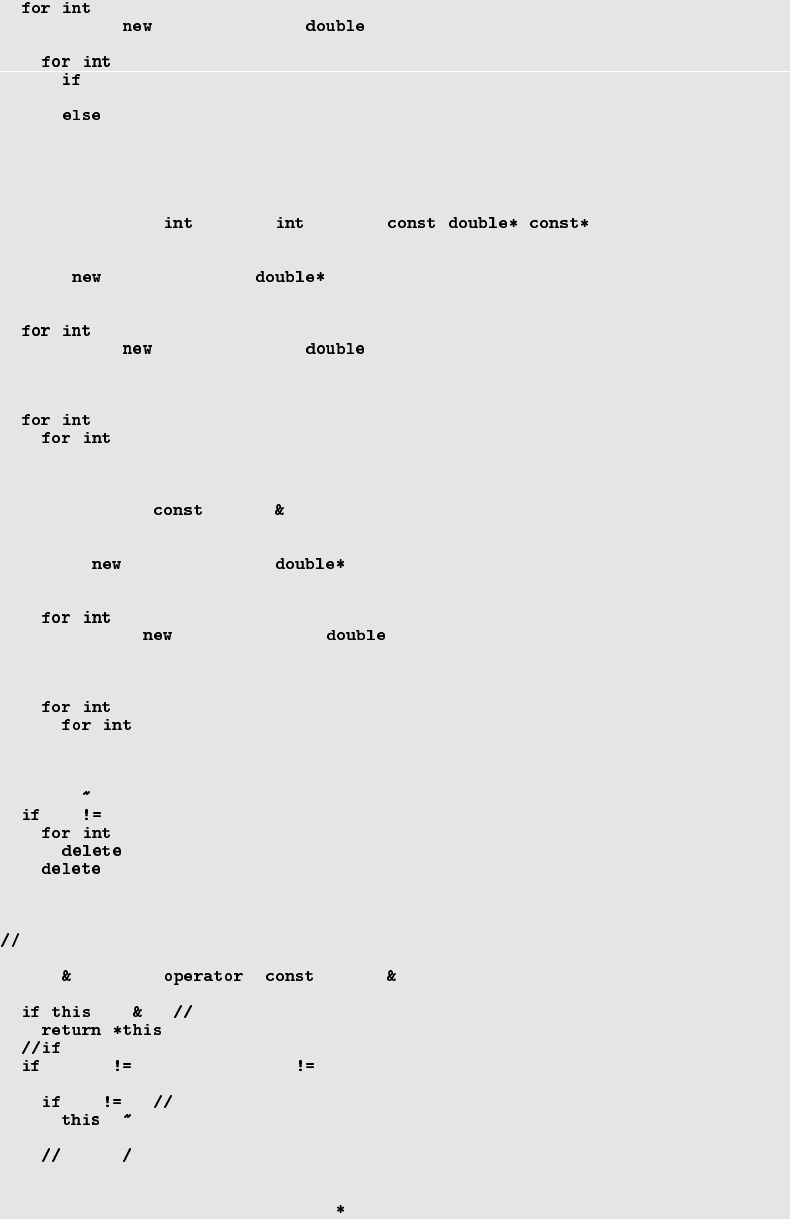
THE MATRIX AND VECTOR CLASSES 513
( i=0;i<nRows;i++){
pA [i ] = (std::nothrow) [nCols];
pointerCheck(i);
( j=0;j<nCols;j++){
(i == j)
pA [i ][ j] = a ;
pA [i ][ j] = 0.;
}
}
}
Matrix :: Matrix ( nrows , ncols , pa ){
nRows = nrows;
nCols = ncols;
pA = (std::nothrow) [nRows];
pointerCheck ();
( i=0;i<nRows;i++){
pA [i ] = (std::nothrow) [nCols];
pointerCheck(i);
}
( i=0;i<nRows;i++)
( j=0;j<nCols;j++)
pA [i ][ j] = pa [i ][ j];
}
Matrix :: Matrix( Matrix A){
nRows = A. nRows;
nCols = A. nCols;
pA = (std::nothrow) [nRows];
pointerCheck ();
( i=0;i<nRows;i++){
pA [i ] = (std::nothrow) [nCols];
pointerCheck(i);
}
( i=0;i<nRows;i++)
( j=0;j<nCols;j++)
pA [i ][ j] = A .pA[i ][ j];
}
Matrix :: Matrix (){
(pA 0){
( i=0;i<nRows;i++)
[] pA [i ];
[] pA ;
}
}
Overloaded operators:
Matrix Matrix :: =( Matrix A){
( == A) avoid self assignment
;
dimensions match we can just overwrite; otherwise ....
(nRows A.nRows||nCols A.nCols){
(pA 0) check first before releasing memory
-> Matrix ();
define redefine object ’s members
nRows = A. nRows;
nCols = A. nCols;
pA = new (std ::nothr ow ) d ouble [nRows];
Get Statistical Computing in C++ and R now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.