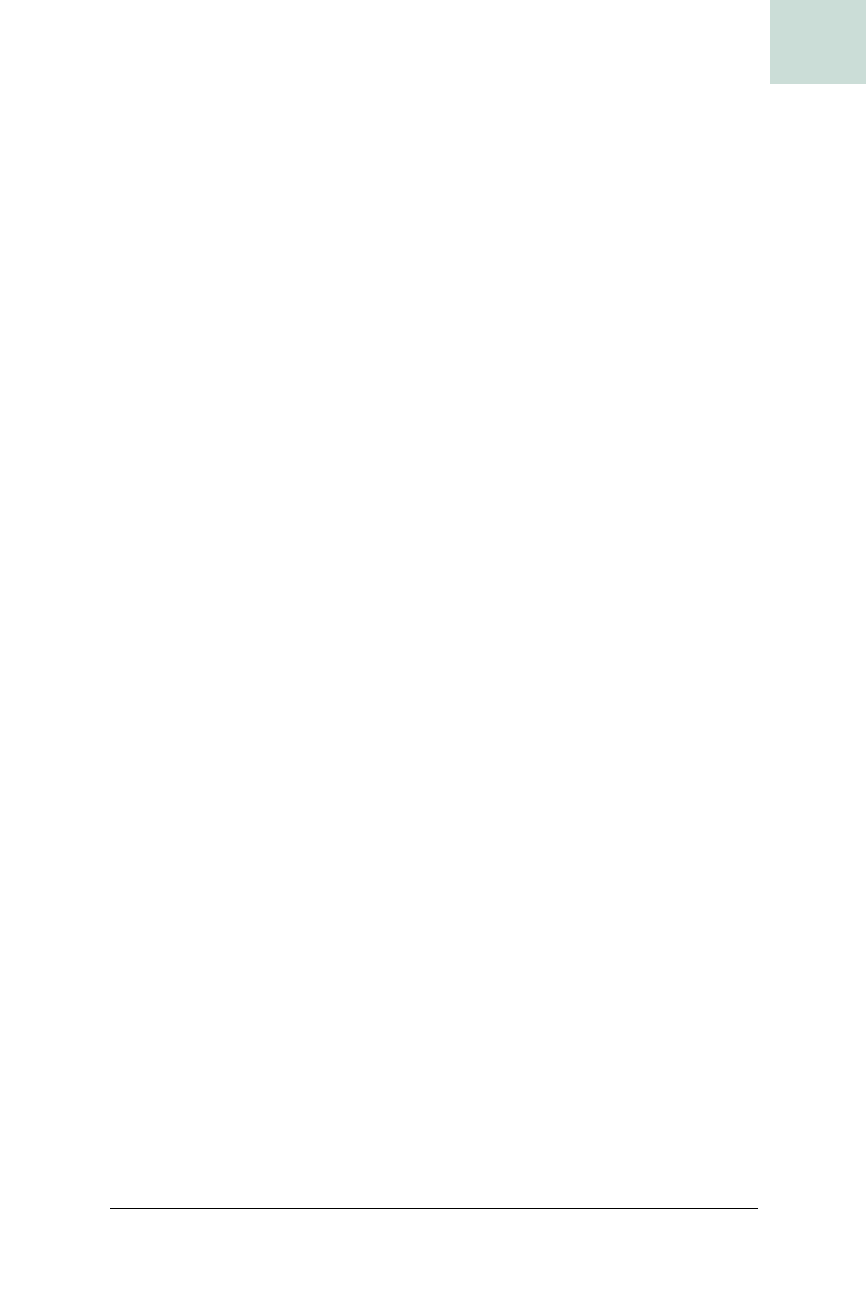
Force Text Input into Specific Formats #49
Chapter 7, Text
|
263
HACK
This class holds onto a Pattern and a Matcher to perform the regex match-
ing. The pattern can be set in the constructor, or later with a call to
setPatternByString( ). In either case, the method compiles the pattern and
creates a
Matcher with the Document’s text. Changing the pattern could, of
course, create a mismatch with any existing text in the
Document, so the
Matcher immediately calls matches( ) and if the text does not match, it
deletes all the text from the
Document.
Perhaps the more typical case is when the
Document.insertString() method is
called. This will happen on every keystroke in a
JTextComponent. Assuming the
Matcher is not null, meaning that the Pattern has been set at some point, you
simply need to call
Matcher.matches( ) against the Document’s new contents to
see if they comply with the regex constraints. If not, return early, never call-
ing the superclass’s
insert( ) method, and thus disallowing the input.
Adding Constrained Text Fields
Since the Document isn’t actually visible, you need to put it in something in
order to test it. The
TestRegexConstrainedDocument class creates a JTextField
for you to type in a regular expression to enforce, and a longer JTextField
for text to test. You create this latter JTextField with the seldom-seen con-
structor that takes a
Document, an initial-value String, and a width (specified
in columns). Obviously, you use a
RegexConstrainedDocument for the first
argument. There’s also a Set
JButton that takes the String value of the regex
field and sets that as the new pattern to match the document against, with
RegexConstrainedDocument’s setPatternByString() method. The rest is mostly
layout code. The test class is shown in Example 7-3.
// consider whether this insert will match
String proposedInsert =
getText (0, offs) +
s +
getText (offs, getLength( ) - offs);
System.out.println ("proposing to change to: " +
proposedInsert);
if (matcher != null) {
matcher.reset (proposedInsert);
System.out.println ("matcher reset");
if (! matcher.matches( )) {
System.out.println ("insert doesn't match");
return;
}
}
super.insertString (offs, s, a);
}
}
Example 7-2. A document allowing input that matches only a regex (continued)
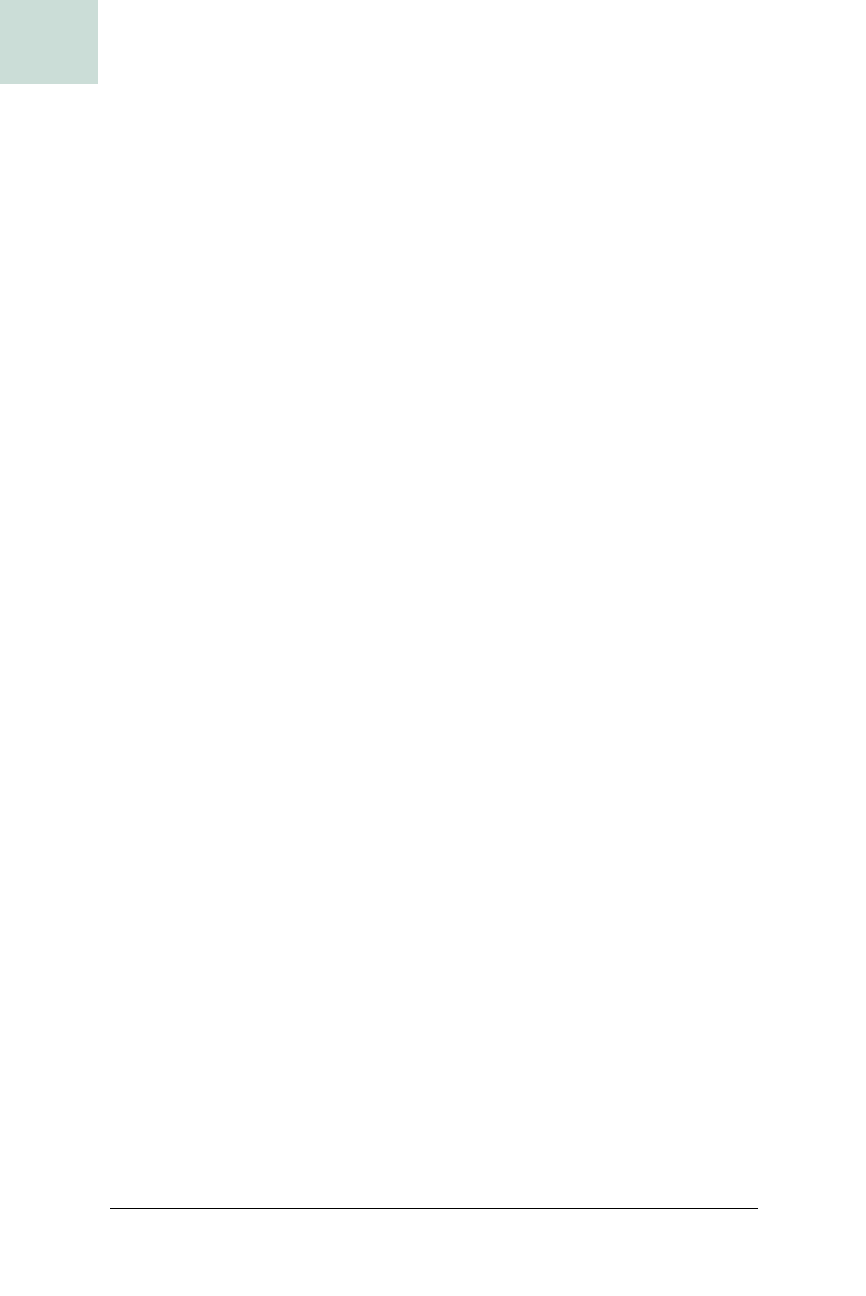
264
|
Chapter 7, Text
#49 Force Text Input into Specific Formats
HACK
When run, the displayed GUI looks like Figure 7-2. Notice that by using the
regex
[A-Z ]*, you can enter uppercase letters and spaces, but nothing else.
Example 7-3. GUI to test the RegexConstrainedDocument in a JTextField
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class TestRegexConstrainedDocument extends JPanel
implements ActionListener {
JTextField regexField, filterField;
JButton regexButton;
RegexConstrainedDocument regexDoc;
public TestRegexConstrainedDocument( ) {
setLayout (new BoxLayout (this, BoxLayout.Y_AXIS));
// top - regex stuff
JPanel topPanel = new JPanel( );
JLabel rLabel = new JLabel ("regex:" );
topPanel.add (rLabel);
regexField = new JTextField (20);
topPanel.add(regexField);
regexButton = new JButton ("Set");
regexButton.addActionListener (this);
topPanel.add (regexButton);
add (topPanel);
// bottom - filterfield
regexDoc =
new RegexConstrainedDocument ( );
filterField = new JTextField (regexDoc, "", 50);
add (filterField);
}
public void actionPerformed (ActionEvent e) {
System.out.println ("actionperformed");
if (e.getSource( ) == regexButton) {
System.out.println ("regexbutton");
regexDoc.setPatternByString (regexField.getText( ));
}
}
public static void main (String[] args) {
JComponent c = new TestRegexConstrainedDocument( );
JFrame f = new JFrame ("Regex filtering");
f.getContentPane( ).add (c);
f.pack( );
f.setVisible(true);
}
}
Get Swing Hacks now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.