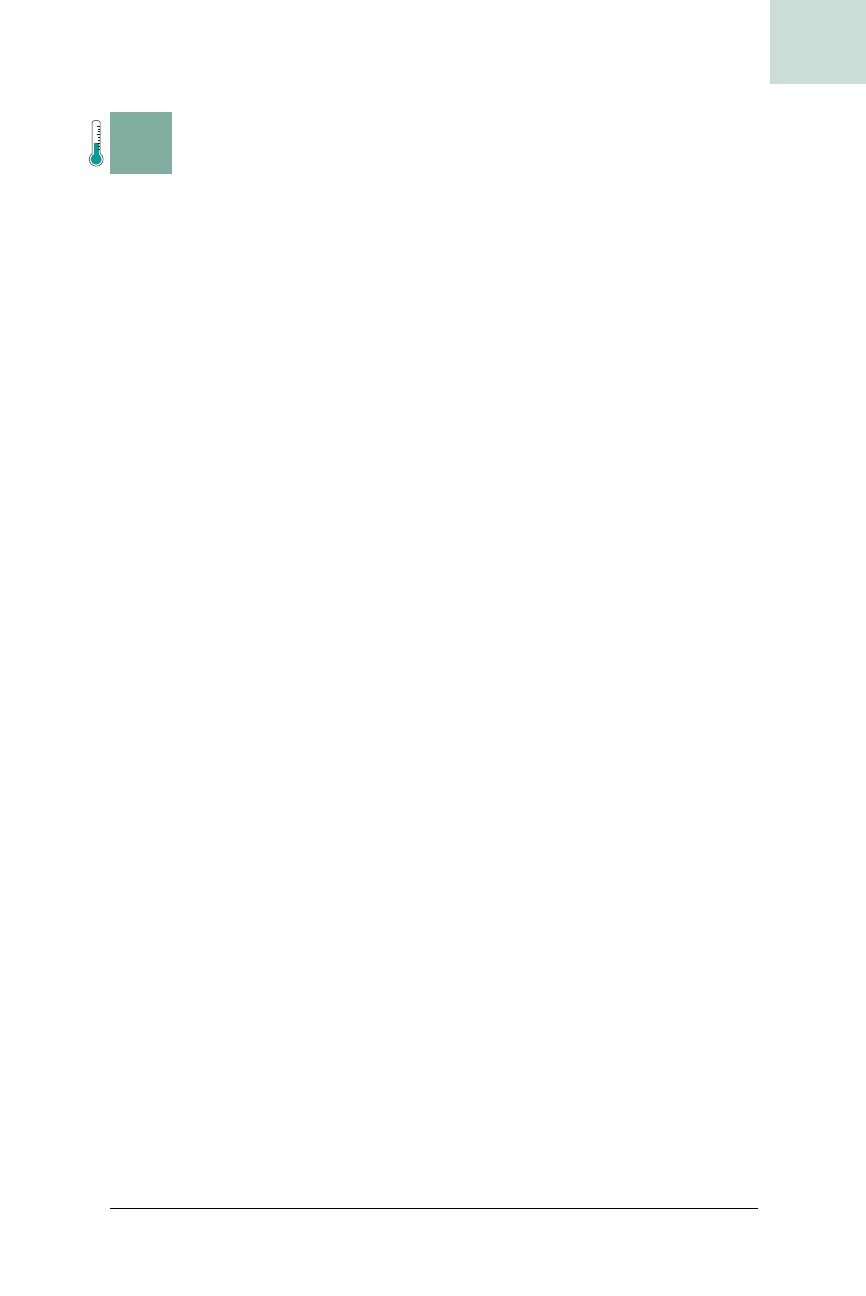
Play a Sound in an Applet #70
Chapter 10, Audio
|
359
HACK
H A C K
#70
Play a Sound in an Applet
Hack #70
If you’re forced to write to the old Applet API for sound, here’s how you do it.
Good luck.
Let me be very clear up front: applet-based sound sucks. If you are in a
hurry to get sound into your application and can count on your users hav-
ing Java 1.3 or better, go ahead and use JavaSound instead
[Hack #71]. Applet-
based sound is going to be most useful to those who must deliver applets
(and only applets) to very old browsers and JVMs. And it’s not going to be
pretty.
Java 1.0 and 1.1 shipped with no support for application-based sound.
None. The only sound support was for applets, presumably so they could
punt the responsibilities for audio to the enclosing browser. The idea in the
early JDKs is built around an
AudioClip, a class found in the java.awt.
applet
package—as David Flanagan says in Java Foundation Classes in a
Nutshell (O’Reilly), “only because there is no better place for it.”
The Code
To demonstrate AudioClips, Example 10-1 shows a hacked up little applet.
Example 10-1. Playing an AudioClip in an applet
public class AppletSound extends Applet
implements ActionListener {
JButton fileButton, loadButton, playButton, loopButton, stopButton;
JLabel urlLabel;
JTextField urlField;
AudioClip clip;
public AppletSound( ) {
setLayout (new GridLayout (2,1));
// first row layout
JPanel topPanel = new JPanel( );
urlLabel = new JLabel ("URL:");
topPanel.add (urlLabel);
urlField = new JTextField (25);
urlField.addActionListener (this);
topPanel.add (urlField);
loadButton = new JButton ("Load");
loadButton.addActionListener (this);
topPanel.add (loadButton);
fileButton = new JButton ("File");
fileButton.addActionListener (this);
topPanel.add (fileButton);
add (topPanel);
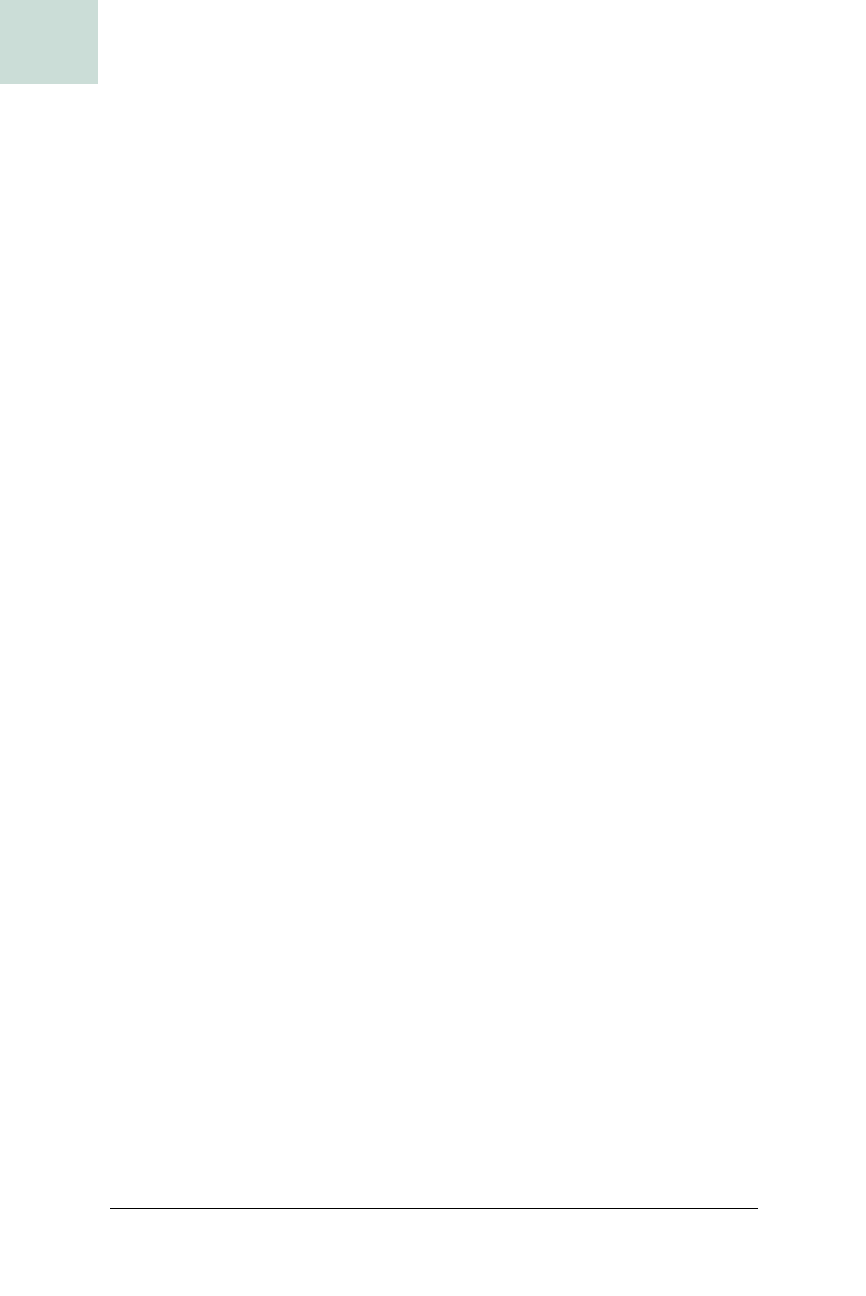
360
|
Chapter 10, Audio
#70 Play a Sound in an Applet
HACK
// second row layout
JPanel bottomPanel = new JPanel( );
playButton = new JButton ("Play");
playButton.addActionListener (this);
bottomPanel.add (playButton);
stopButton = new JButton ("Stop");
stopButton.addActionListener (this);
bottomPanel.add (stopButton);
loopButton = new JButton ("Loop");
loopButton.addActionListener (this);
bottomPanel.add (loopButton);
add (bottomPanel);
}
public void stop( ) {
clip.stop( );
}
public void actionPerformed (ActionEvent e) {
Object source = e.getSource( );
if (source == fileButton) {
JFileChooser chooser = new JFileChooser( );
int pick = chooser.showOpenDialog(this);
if (pick == JFileChooser.APPROVE_OPTION) {
try {
File file = chooser.getSelectedFile( );
urlField.setText (file.toURL().toString( ));
} catch (MalformedURLException murle) {
murle.printStackTrace( );
}
}
} else if (source == loadButton ) {
try {
System.out.println ("field: " + urlField.getText( ));
URL clipURL = new URL (urlField.getText( ));
System.out.println ("loading " + clipURL);
clip = getAudioClip (clipURL);
System.out.println ("got clip");
} catch (MalformedURLException murle) {
murle.printStackTrace( );
}
} else if (source == playButton ) {
clip.play( );
} else if (source == stopButton ) {
clip.stop( );
} else if (source == loopButton ) {
clip.loop( );
}
}
Example 10-1. Playing an AudioClip in an applet (continued)
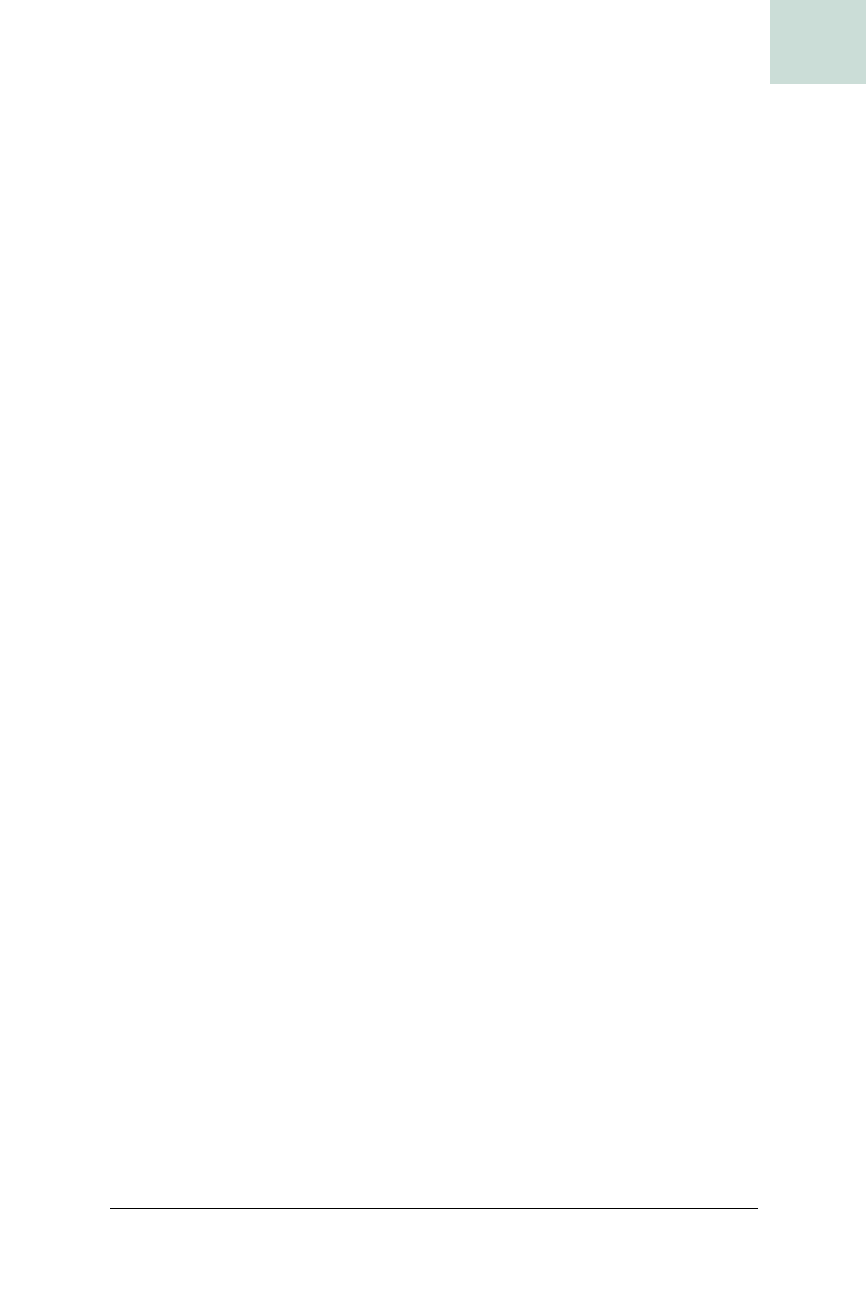
Play a Sound in an Applet #70
Chapter 10, Audio
|
361
HACK
If you’ve never worked with an applet, you need to be aware that they can’t
simply be launched from the command line with the
java
command. They
need to live in some sort of applet-aware container, typically a browser, with
their size and parameters specified by HTML tags. Example 10-2 shows a
simple index.html file to show the
AppletAudio applet in a web page.
Notice how the applet doesn’t have a
setVisible(true) line anywhere. The
applet is an AWT panel that does its own layout; its constructor will be
called from the browser plug-in (or equivalent) to get its pixels into the
browser window.
The constructor of this applet is concerned exclusively with layout. It pro-
vides a
JTextField for a URL, a Load JButton to load an AudioClip from that
URL; a File
JButton that can be used instead of typing in a file:/// URL by
hand; and Play, Stop, and Loop
JButtons to control the AudioClip.
actionPerformed( ) is the guts of this applet. If you click the File button, it
simply shows a
JFileChooser, converts the chosen file to a URL, and puts
that in the
TextField.
The handler for the Load button is also critical. When this button is clicked,
the applet gets the text from the
JTextField, makes a URL out of the text,
and passes that URL to
getAudioClip( ). getAudioClip( ) is an Applet method
that’s a shortcut for getting an
AppletContext object, which describes the
environment the applet runs in and calls its
getAudioClip( ).
public static void main (String args[]) {
JFrame f = new JFrame ("Applet Sound");
f.getContentPane().add (new AppletSound( ));
f.pack( );
f.setVisible(true);
}
}
Example 10-2. HTML to display AppletSound applet
<html>
<head><title>Applet Sound</title></head>
<body>
<p>Playing sound with <code>java.applet</code></p>
<APPLET
CODE="AppletSound.class"
WIDTH="600"
HEIGHT="75">
</APPLET>
</body>
</html>
Example 10-1. Playing an AudioClip in an applet (continued)
Get Swing Hacks now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.