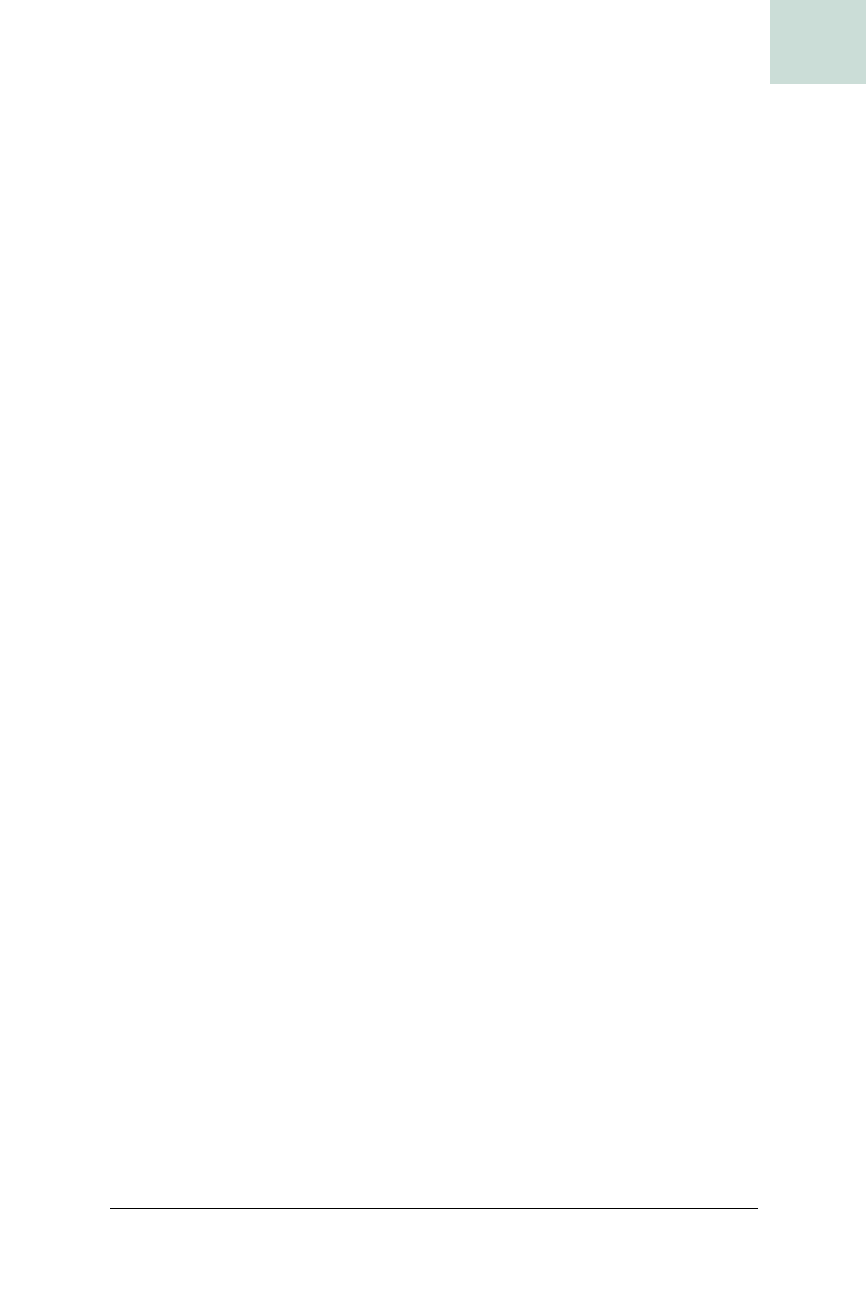
Stuff Stuff in JARs #85
Chapter 11, Native Integration and Packaging
|
429
HACK
An Obvious Secret
JAR files—the acronym is short for Java ARchive—must be the best-known,
least-used feature in Java. Many developers throw a JAR in their classpath to
pick up some standard extension API or third-party library, but how many
actually distribute their software that way?
And JARs aren’t just about code. It’s really easy to put the files your pro-
gram needs into a JAR. This has the added advantage of hiding your images,
sounds, default settings, and so forth from end users.
But to load these items, you need to make a change in how you load stuff in
your code. Instead of specifying a known path or URL, you ask a
ClassLoader to find these resources along the classpath. By doing this, you
can get your resources from flat files while you’re developing, and then eas-
ily switch to getting them from inside a JAR when the code is deployed in
the field.
The key is the
ClassLoader’s getResource( ) and getResources( ) methods,
which take a path relative to the loaded class and return a
URL and an array
of
URLs respectively. A getResourceAsStream( ) method converts the URL to an
InputStream as a convenience.
To clarify the relative path: say you have a directory that includes your com-
piled classes in a path like com/mycompany/mypackage/…, an images direc-
tory, and a sounds directory. A relative path would be one of the form
images/something.png, sounds/something.aiff, etc. By using a resource on the
classpath, there is no difference (to the user) between files in a JAR and files
in sub-directories on a filesystem. Either way, you get a
URL that you can use
by passing it to methods that take
URL arguments, by opening an
InputStream from it, etc.
Showing Off
Example 11-7 shows an example of this technique. It uses the getResource( )
method to load an image and put it in an ImageIcon, which in turn is used to
create a
JButton. It also loads in a sound, which is played via JavaSound
when you click the button.
Example 11-7. Loading image and sound as resources along the classpath
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.sound.sampled.*;
import java.net.*;
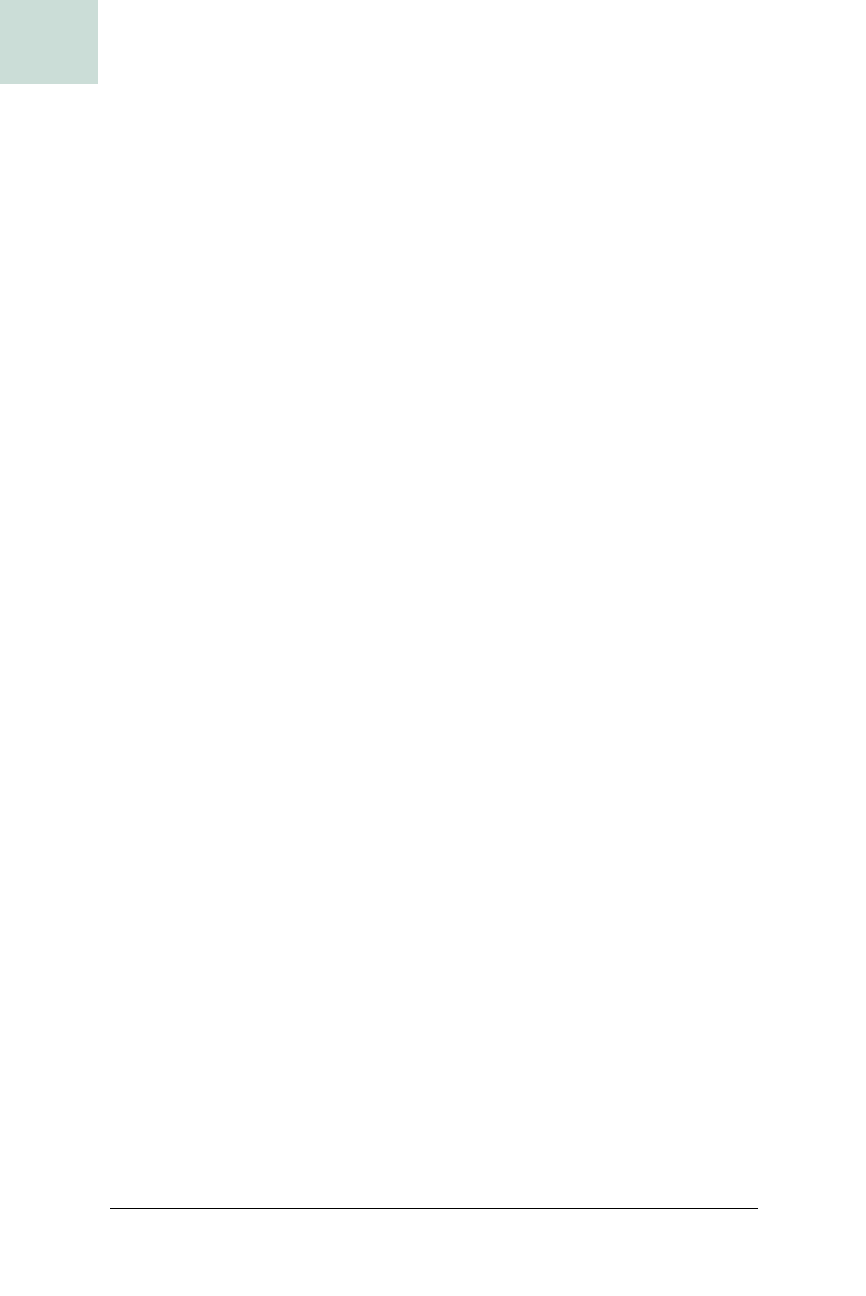
430
|
Chapter 11, Native Integration and Packaging
#85 Stuff Stuff in JARs
HACK
public class JarResourceLoading extends JFrame
implements ActionListener {
JButton button;
ImageIcon buttonIcon;
Clip buhClip;
public final static String SOUND_PATH = "sounds/buhbuhbuh.aiff";
public final static String IMAGE_PATH = "images/keagan-buh.jpeg";
public JarResourceLoading ( ) {
super ("Resources from .jar");
// get image and make button
URL imageURL = getClass().getClassLoader( ).getResource (IMAGE_PATH);
System.out.println ("found image at " + imageURL);
buttonIcon = new ImageIcon (imageURL);
button = new JButton ("Click to Buh!", buttonIcon);
button.setHorizontalTextPosition (SwingConstants.CENTER);
button.setVerticalTextPosition (SwingConstants.BOTTOM);
button.addActionListener (this);
getContentPane( ).add (button);
// load sound into Clip
try {
URL soundURL = getClass().getClassLoader( ).getResource (SOUND_PATH);
System.out.println ("found sound at " + soundURL);
Line.Info linfo = new Line.Info (Clip.class);
Line line = AudioSystem.getLine (linfo);
buhClip = (Clip) line;
AudioInputStream ais = AudioSystem.getAudioInputStream(soundURL);
buhClip.open(ais);
} catch (Exception e) {
e.printStackTrace( );
}
}
public void actionPerformed (ActionEvent e) {
System.out.println ("click!");
if (buhClip != null) {
buhClip.setFramePosition (0);
buhClip.start( );
}
else
JOptionPane.showMessageDialog (this,
"Couldn't load sound",
"Error",
JOptionPane.ERROR_MESSAGE);
}
public static final void main (String[] args) {
JFrame frame = new JarResourceLoading( );
Example 11-7. Loading image and sound as resources along the classpath (continued)
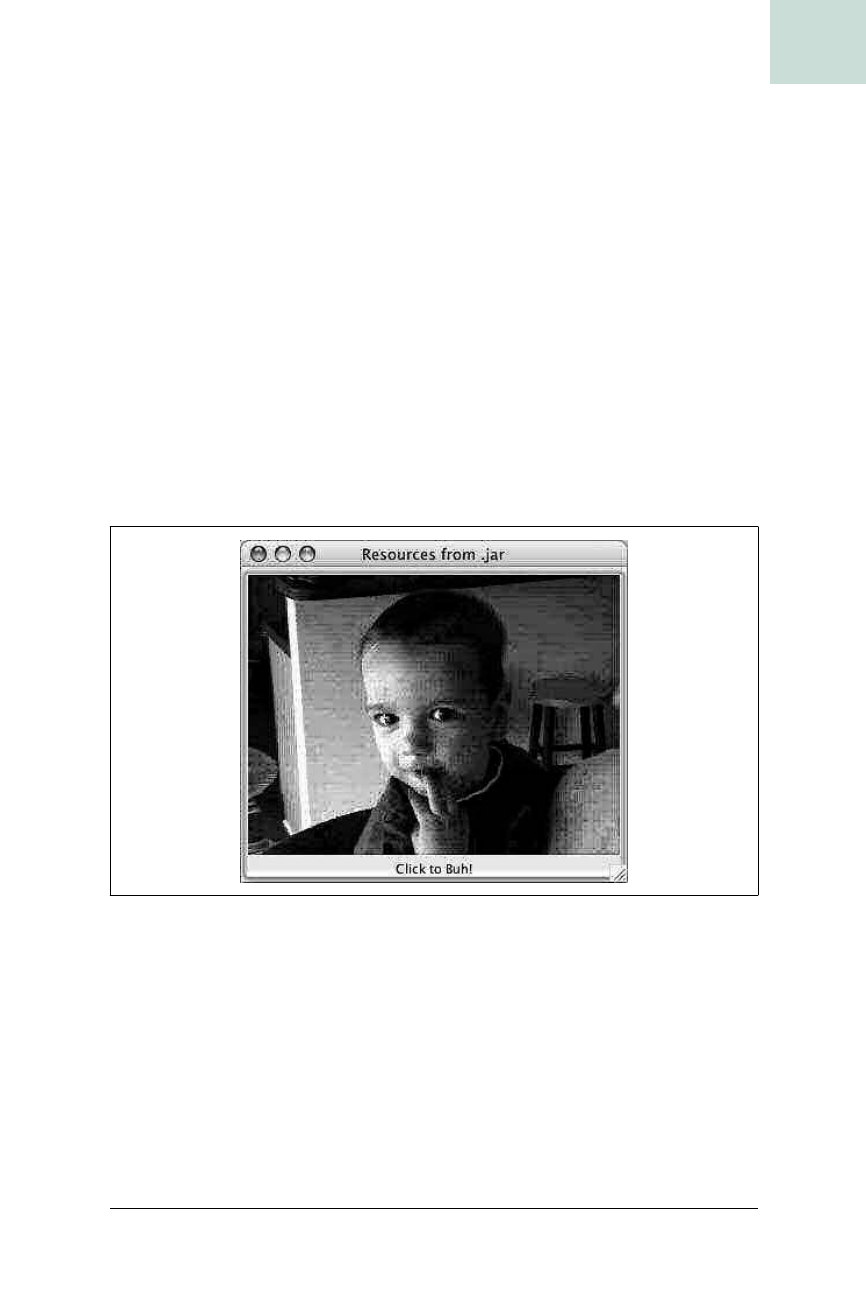
Stuff Stuff in JARs #85
Chapter 11, Native Integration and Packaging
|
431
HACK
Notice how the paths to the sound and the image are relative:
public final static String SOUND_PATH = "sounds/buhbuhbuh.aiff";
These paths specify the path from a given starting point. As
getResource( )
checks each entry in its classpath—whether they’re directories or JAR files
(ZIP files are also allowed)—the class loader looks for a directory called
sounds and, if that’s found, for an entry inside it called buhbuhbuh.aiff. It’s
also worth noting that you use the Unix-style forward slashes, regardless of
what operating system this is run on.
When you compile and run this code from the source directory, the result
looks like Figure 11-10.
Along with showing this simple GUI, it also prints to standard out the URLs
returned by
getResource( ). Running from the source directory, the output
looks like this:
[tonberry:] cadamson% java JarResourceLoading
found image at file:/Users/cadamson/Documents/O'Reilly/books/swing%20hacks/
HacksBook/PackagingInstalling/97/images/keagan-buh.jpeg
found sound at file:/Users/cadamson/Documents/O'Reilly/books/swing%20hacks/
HacksBook/PackagingInstalling/97/sounds/buhbuhbuh.aiff
frame.pack( );
frame.setVisible(true);
}
}
Figure 11-10. Application using image and sound loaded with getResource( )
Example 11-7. Loading image and sound as resources along the classpath (continued)
Get Swing Hacks now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.