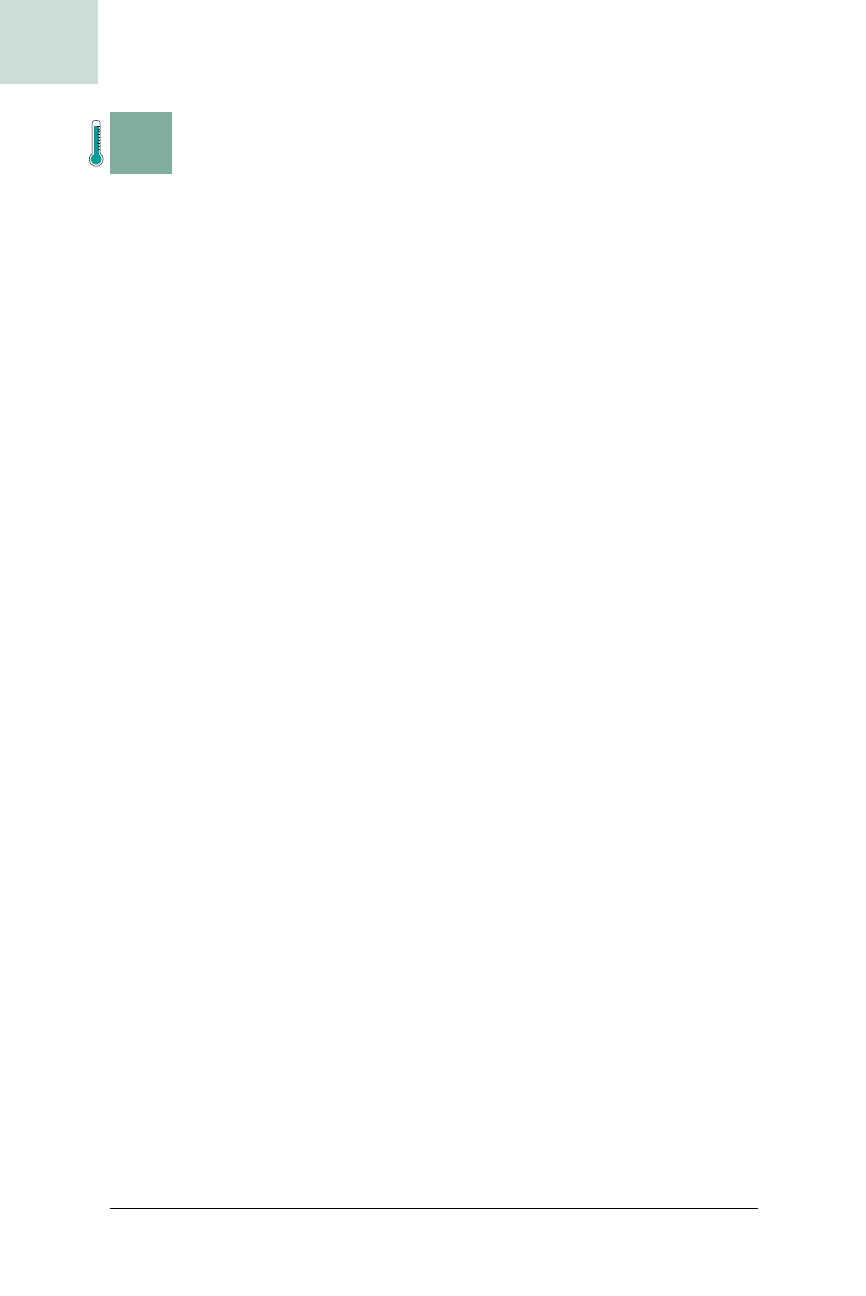
450
|
Chapter 12, Miscellany
#90 Create Demonstrations with the Robot Class
HACK
H A C K
#90
Create Demonstrations with the Robot Class
Hack #90
Use the Robot class to control the mouse cursor and create interactive
software features.
One of the coolest things about Swing is that you can often use a class for
something completely different than what it was originally intended. The
java.awt.Robot class, for example, can move the mouse cursor programmat-
ically. This feature was originally intended for use by automated testing
tools (hence the name Robot), but I’ve found it very useful to demonstrate
software features by moving the mouse cursor through the same actions as
the user. Instead of just describing something in a help file, you can actually
show the user what to do—and this hack explains how.
I, Robot
To create a mouse animation, you need three things:
1. The ability to move the cursor
2. The start and end points of the animation
3. A way to smoothly interpolate the cursor position
The
java.awt.Robot class has a variety of methods for capturing program
state and controlling the user interface, including
Robot.mouseMove( ), which
allows you to move the mouse cursor programmatically.
The following code is the implementation of a method
moveMouse( ), which
takes three arguments: a starting component, an ending component, and a
duration for the animation (in milliseconds). Because most demonstrations
involve showing the user a particular component, the easiest points to use
are the centers of start and end components. The mouse will smoothly move
from the center of the starting component to the center of the ending one.
We normally think of components as being positioned relative to their par-
ents, but since the
Robot class uses absolute mouse positions, you’ll need to
convert the components to their screen locations:
public void moveMouse(JComponent start, JComponent end,
final int duration) throws Exception {
final Robot robot = new Robot( );
// get middle of start
final Point start_coords = start.getLocationOnScreen( );
start_coords.translate(start.getWidth( )/2,
start.getHeight( )/2);
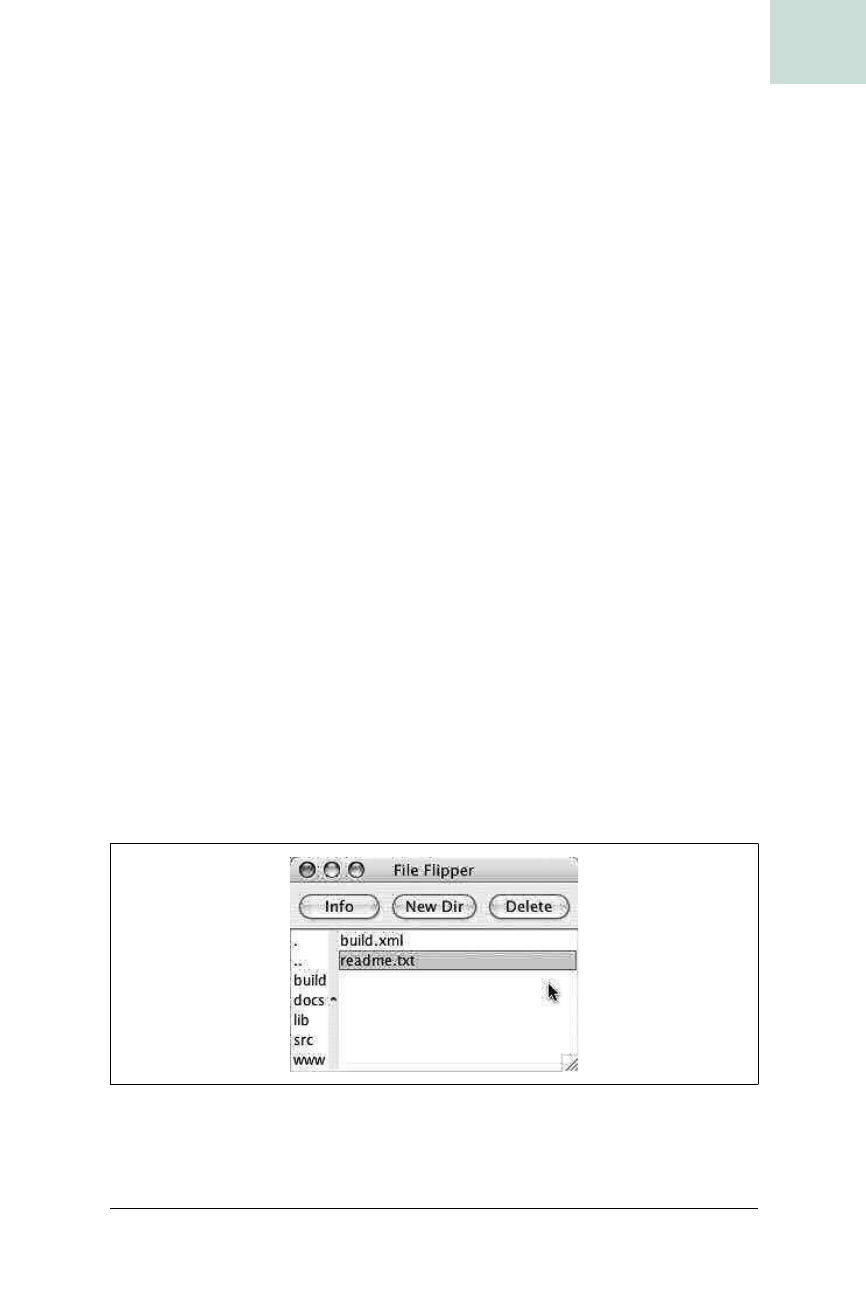
Create Demonstrations with the Robot Class #90
Chapter 12, Miscellany
|
451
HACK
// get middle of end
final Point end_coords = end.getLocationOnScreen( );
end_coords.translate(end.getWidth( )/2,
end.getHeight( )/2);
// create interpolation point and offsets
int steps = duration/50;
//Point current = new Point(start_coords);
int distx = (end_coords.x - start_coords.x);
int disty = (end_coords.y - start_coords.y);
// move the mouse over 10 steps
for(int i=1; i<=steps; i++) {
int x = start_coords.x + i*distx/steps;
int y = start_coords.y + i*disty/steps;
robot.mouseMove(x,y);
try { Thread.currentThread( ).sleep(50);
} catch (Exception ex) {}
}
}
This code creates a new Robot and calculates the center positions of the start
and end components using screen coordinates.
steps is the number of
frames required to fill the specified duration. Since each frame will be 1/20
of a second long, the number of frames is the total duration divided by 50
ms.
distx and disty are the distances between the two components in the
horizontal and vertical directions. With these values in hand, it is a simple
matter to interpolate a new position for each frame and then move the
mouse cursor there.
To test the system, you’ll need some sort of a demonstration program. The
code in Example 12-4 creates a fake file browser with a list of directories, a
list of files, and a toolbar with three buttons: Info, New Dir, and Delete. You
can see this in Figure 12-2.
Figure 12-2. Sample program to demonstrate mouse automation
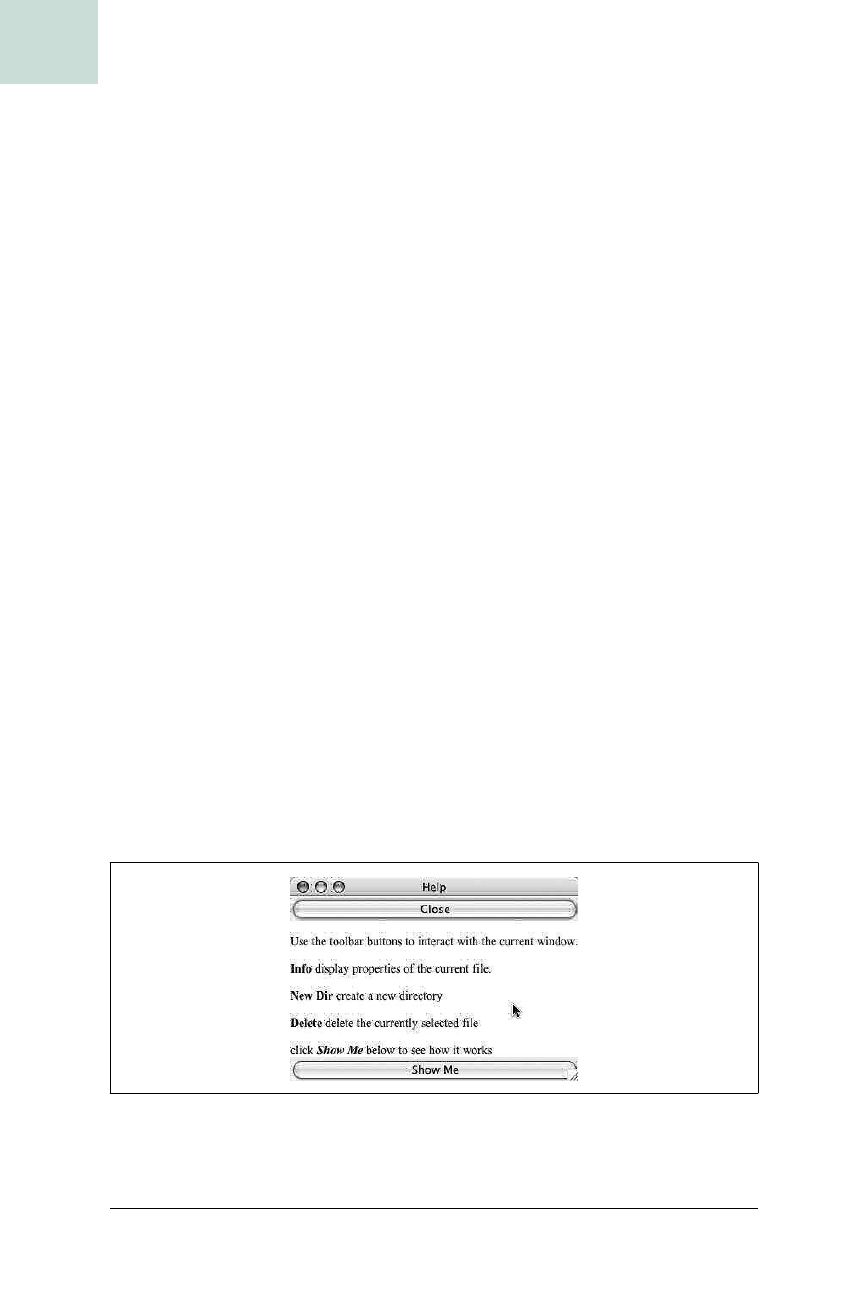
452
|
Chapter 12, Miscellany
#90 Create Demonstrations with the Robot Class
HACK
The demonstration program also contains a Help screen (shown in
Figure 12-3).
Example 12-4. A simple demonstration for the power of Robot
public class AutoMouseHack implements ActionListener {
public JButton info, new_dir, delete;
public void createDemo( ) {
JFrame frame = new JFrame("File Flipper");
String[] dirs = {".","..","build","docs","lib","src","www"};
String[] files = {"build.xml","readme.txt"};
JList dir_list = new JList(dirs);
JList files_list = new JList(files);
JSplitPane split = new JSplitPane(
JSplitPane.HORIZONTAL_SPLIT,
dir_list,files_list);
info = new JButton("Info");
new_dir = new JButton("New Dir");
delete = new JButton("Delete");
JPanel toolbar = new JPanel( );
toolbar.setLayout(new FlowLayout( ));
toolbar.add(info);
toolbar.add(new_dir);
toolbar.add(delete);
frame.getContentPane().setLayout(new BorderLayout( ));
frame.getContentPane( ).add("North",toolbar);
frame.getContentPane( ).add("Center",split);
frame.pack( );
frame.show( );
}
Figure 12-3. Help text
Get Swing Hacks now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.