Chapter 5. Statements and Control Structures
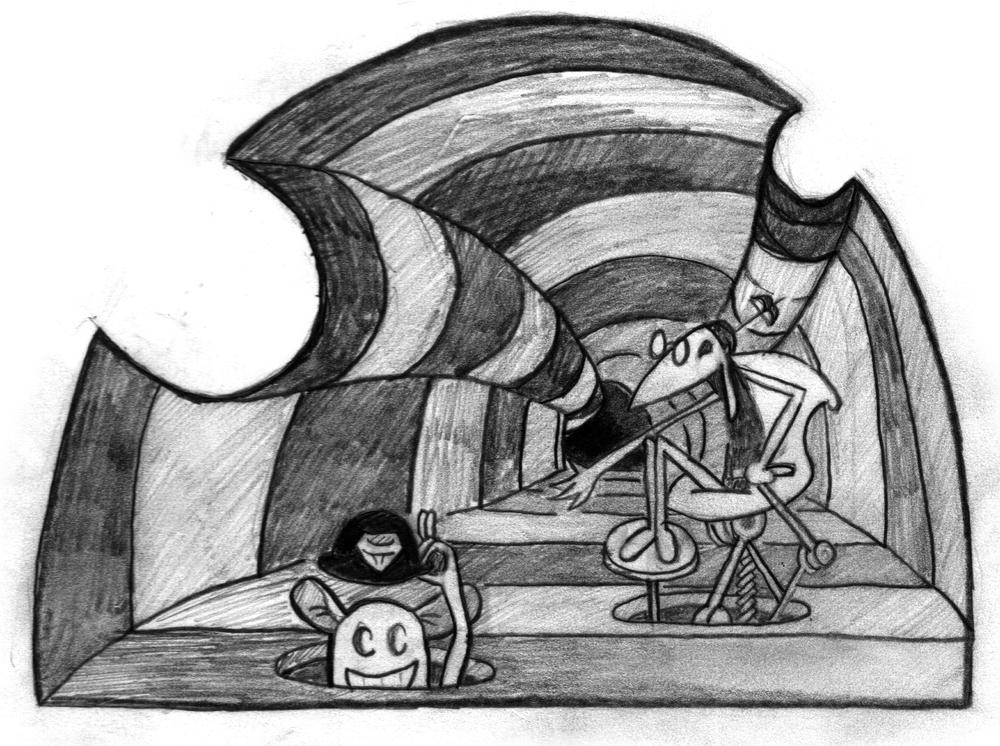
Consider the following Ruby program. It adds two numbers passed to it on the command line and prints the sum:
x = ARGV[0].to_f # Convert first argument to a number y = ARGV[1].to_f # Convert second argument to a number sum = x + y # Add the arguments puts sum # Print the sum
This is a simple program that consists primarily of variable assignment and method invocations. What makes it particularly simple is its purely sequential execution. The four lines of code are executed one after the other without branching or repetition. It is a rare program that can be this simple. This chapter introduces Ruby’s control structures, which alter the sequential execution, or flow-of-control, of a program. We cover:
Conditionals
Loops
Iterators and blocks
Flow-altering statements like
return
andbreak
Exceptions
The special-case BEGIN and END statements
The esoteric control structures known as fibers and continuations
Get The Ruby Programming Language now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.