/* edit_cb() −− the callback routine for the items in the edit menu */
void
edit_cb(widget, client_data, call_data)
Widget widget;
XtPointer client_data;
XtPointer call_data;
{
Boolean result = True;
int reason = (int) client_data;
XEvent *event = ((XmPushButtonCallbackStruct *) call_data)−>event;
Time when;
XmTextSetString (text_output, NULL); /* clear message area */
if (event != NULL &&
reason == EDIT_CUT || reason == EDIT_COPY || reason == EDIT_CLEAR) {
switch (event−>type) {
case ButtonRelease :
when = event−>xbutton.time;
break;
case KeyRelease :
when = event−>xkey.time;
break;
default:
when = CurrentTime;
break;
}
}
switch (reason) {
case EDIT_CUT :
result = XmTextCut (text_edit, when);
break;
case EDIT_COPY :
result = XmTextCopy (text_edit, when);
break;
case EDIT_PASTE :
result = XmTextPaste (text_edit);
case EDIT_CLEAR :
XmTextClearSelection (text_edit, when);
break;
}
if (result == False)
XmTextSetString (text_output, "There is no selection.");
}
15.5 Text Callbacks
The Text and TextField widgets use callback routines in the same way as other Motif widgets. The widgets provide
callbacks for a number of different purposes, such as text modification, activation, and selection ownership. Some of
the routines, such as those that monitor keyboard input, may be invoked rather frequently. In the next few sections, we
introduce several of the callback routines for the widgets.
15.5.1 The Activation Callback
We begin by exploring the callback routine that is most commonly used for single−line Text widgets and TextField
widgets. This callback is the XmNactivateCallback, which is invoked when the user presses RETURN in a
TextField widget or a single−line Text widget. The callback is not called for multiline Text widgets. The callback
15 Text Widgets 15.5 Text Callbacks
412
routine for an XmN-activateCallback receives the common XmAnyCallbackStruct as the call_data
parameter to the function. The callback reason is always XmCR_ACTIVATE. the source code shows a callback
function for some TextField widgets. XtSetLanguageProc() is only available in X11R5; there is no
corresponding function in X11R4.
/* text_box.c −− demonstrate simple use of XmNactivateCallback
* for TextField widgets. Create a rowcolumn that has rows of Form
* widgets, each containing a Label and a Text widget. When
* the user presses Return, print the value of the text widget
* and move the focus to the next text widget.
*/
#include <Xm/TextF.h>
#include <Xm/LabelG.h>
#include <Xm/Form.h>
#include <Xm/RowColumn.h>
char *labels[] = { "Name:", "Address:", "City:", "State:", "Zip:" };
main(argc, argv)
int argc;
char *argv[];
{
Widget toplevel, text_w, form, rowcol;
XtAppContext app;
int i;
void print_result();
XtSetLanguageProc (NULL, NULL, NULL);
toplevel = XtVaAppInitialize (&app, "Demos",
NULL, 0, &argc, argv, NULL, NULL);
rowcol = XtVaCreateWidget ("rowcol",
xmRowColumnWidgetClass, toplevel, NULL);
for (i = 0; i < XtNumber (labels); i++) {
form = XtVaCreateWidget ("form", xmFormWidgetClass, rowcol,
XmNfractionBase, 10,
NULL);
XtVaCreateManagedWidget (labels[i],
xmLabelGadgetClass, form,
XmNtopAttachment, XmATTACH_FORM,
XmNbottomAttachment, XmATTACH_FORM,
XmNleftAttachment, XmATTACH_FORM,
XmNrightAttachment, XmATTACH_POSITION,
XmNrightPosition, 3,
XmNalignment, XmALIGNMENT_END,
NULL);
text_w = XtVaCreateManagedWidget ("text_w",
xmTextFieldWidgetClass, form,
XmNtraversalOn, True,
XmNrightAttachment, XmATTACH_FORM,
XmNleftAttachment, XmATTACH_POSITION,
XmNleftPosition, 4,
NULL);
/* When user hits return, print the label+value of text_w */
XtAddCallback (text_w, XmNactivateCallback,
print_result, labels[i]);
15 Text Widgets 15.5 Text Callbacks
413
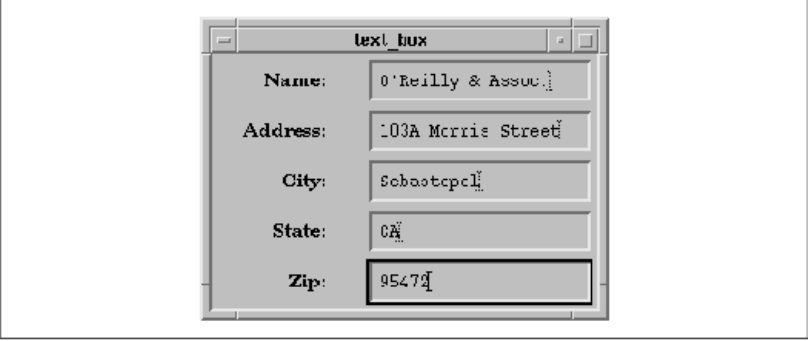
XtManageChild( form);
}
XtManageChild (rowcol);
XtRealizeWidget (toplevel);
XtAppMainLoop (app);
}
/* preint_result() −− callback for when the user hits return in the
* TextField widget.
*/
void
print_result(text_w, client_data, call_data)
Widget text_w;
XtPointer client_data;
XtPointer call_data;
{
char *value = XmTextFieldGetString (text_w);
char *label = (char *) client_data;
printf ("%s %s0, label, value);
XtFree (value);
XmProcessTraversal (text_w, XmTRAVERSE_NEXT_TAB_GROUP);
}
The program displays a data form using a RowColumn widget that manages several rows of Form widgets. Each Form
contains a Label and a TextField widget, as shown in the figure.
When the user enters a value for a field and presses RETURN, the print_result() callback routine is invoked.
The routine prints the value of the field and advances the keyboard focus to the next widget using
XmProcessTraversal(). This function takes a widget and a traversal direction as its two parameters. We use the
XmTRAVERSE_NEXT_TAB_ GROUP direction because each TextField widget is a tab group in and of itself, so we
need to move to the next tab group, rather than to the next item in the same tab group. See Section #skeybtrav, for
more information on tab groups.
Output of text_box.c
When a single−line Text widget or a TextField widget is used as part of a predefined Motif dialog, the
XmNactivateCallback for the widget is automatically hooked up to the OK button in the dialog. As a result, the
15 Text Widgets 15.5 Text Callbacks
414
Get Volume 6A: Motif Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.