compiler knows if a widget allows children, and for those that do, which widget types can be created as their children.
If you try to include a child widget where it isn't allowed or supported, the UIL compiler generates an error message
and the compilation fails, which is one of the advantages of describing a user interface in UIL rather than with a
programming language like C.
You set widget resources, with the exception of callbacks, in a widget's argument subsection. This subsection in the
hello_main widget illustrates several typical resource settings. We used a symbolic constant to set the last two
resources so that it is easy to adjust the Form margins by changing the constant definition.
Callback resource settings are specified separately from other resources in the callbacks subsection of a widget
definition. The hello_main widget does not have any callbacks, but the PushButton does. Here's the relevant part
of its definition:
object hello : XmPushButton
{
! ... arguments ...
callbacks {
XmNactivateCallback = procedure quit ("Goodbye!");
};
};
This subsection sets the PushButton's activate callback to the quit() procedure declared earlier in the module. The
string argument "Goodbye!" is passed as client_data to the procedure when the callback is invoked. You'll see
how this value is used later when we explain registering callback procedures with Mrm.
The widget definitions, along with the value definitions and procedure declaration, are all there is to the "Hello,
World" module. As a whole, they form the interface description, which is the first step in developing an application
with UIL. Our interface is quite simple; the interface for a real application would obviously be much more complex.
The UIL modules for a real application are presented in Chapter 25, Building an Application With UIL.
23.4 Compiling the UIL Module
The UIL module must be compiled to produce a user interface description (UID) file. This compiled file is read at
run−time by Mrm to obtain the interface description and create the widgets. The UID file is generated only if the
source module is free of errors. On a UNIX system, we use the following command to compile our module:
uil −o hello_world.uid hello_world.uil
The −o option specifies the name of the output file. (This option, along with the rest of the compiler options, is
explained in Chapter 23, Using the UIL Compiler). The name of the module to compile, in this case hello_world.uil,
always follows the options. If the compilation is successful, the compiler generates a UID file. But if the compilation
fails, the compiler prints one or more error messages and does not generate a UID file. Warning and informational
messages can also be printed in either situation. The hello_world.uil module is free of errors and warnings, so this
compilation does not print anything.
23.5 Structure of an Mrm Application
The structure of an application that uses Mrm and UIL is similar in most respects to that of an application that uses
only Xt. The main difference is that you create the user interface with calls to Mrm procedures that encapsulate the Xt
widget creation routines. Mrm also takes care of setting up any callbacks for your widgets. Other aspects of an Xt
application, including toolkit initialization and event processing, are the same for both types of applications. the figure
23 Introduction to UIL 23.4 Compiling the UIL Module
640
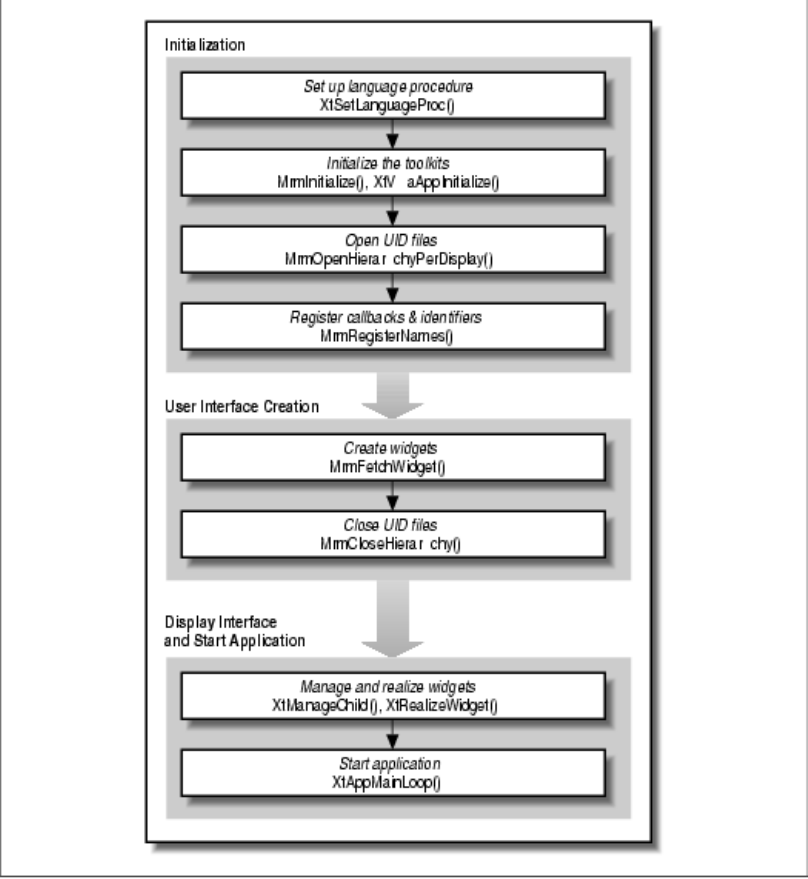
illustrates the structure of an Mrm application.
Structure of the hello_world.c Mrm application
In the remainder of this section, we take a closer look at each of these steps by examining the hello_world.c program
shown in the source code In our explanation of this program, we concentrate only on how it differs from a standard
Motif application. If you are unfamiliar with the details of a particular function call, see Chapter 2, The Motif
Programming Model, in this book or see Volume Four, X Toolkit Intrinsics Programming Manual, and
Volume Five, X Toolkit Intrinsics Reference Manual.
/* hello_world.c −−
* Initialize X Toolkit creating ApplicationShell widget, then create
* the user interface described in the hello_world.uid file.
*/
#include <Xm/Xm.h>
#include <Mrm/MrmPublic.h>
23 Introduction to UIL 23.4 Compiling the UIL Module
641
#include <stdio.h>
/* Global declarations. */
static void quit();
/* Global definitions. */
/* Callback list looks like an action list: */
static MrmRegisterArg callback_list[] = {
{ "quit", (XtPointer) quit },
};
/* error − Print an error message and exit. */
static void
error (message)
char *message;
{
fprintf (stderr, "hello_world: %s0, message);
exit (1);
}
/* quit − The quit callback procedure. Exits the program. */
static void
quit (w, client_data, call_data)
Widget w;
XtPointer client_data;
XtPointer call_data;
{
puts ((char *) client_data);
exit (0);
}
main (argc, argv)
int argc;
char *argv[];
{
XtAppContext app_context;
Widget toplevel, hello_main;
Cardinal status;
static String uid_file_list[] = { "hello_world" };
MrmHierarchy hierarchy;
MrmType class_code;
XtSetLanguageProc (NULL, NULL, NULL);
MrmInitialize();
toplevel =
XtVaAppInitialize (&app_context, /* application context */
"Demos", /* application class name */
NULL, 0, /* command line options */
&argc, argv, /* argc and argv */
NULL, /* fallback resources */
NULL); /* arg list */
status =
MrmOpenHierarchyPerDisplay (XtDisplay (toplevel), /* display */
XtNumber (uid_file_list), /* num files */
uid_file_list, /* file list */
NULL, /* OS data */
&hierarchy); /* hierarchy */
23 Introduction to UIL 23.4 Compiling the UIL Module
642
Get Volume 6A: Motif Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.