XmDIALOG_COMMAND
XmDIALOG_FILE_SELECTION
These values should be self−explanatory, with the exception of XmDIALOG_WORK_AREA. This value is set when a
SelectionBox is not the child of a DialogShell and it is not one of the other types of dialogs. In other words, if you
create a SelectionDialog using XmCreateSelectionDialog(), the value is XmDIALOG_SELECTION, but if
you use XmCreateSelectionBox(), the value is XmDIALOG_WORK_AREA. When a SelectionBox is created as
the child of a DialogShell, the Apply button is automatically managed, except if XmNdialogType is set to
XmDIALOG_PROMPT. Otherwise, the button is created but not managed.
The different types of SelectionDialogs are meant to be used for unique purposes. Each dialog provides different
components that the user can interact with to perform a task. In the following sections, we examine each of the
SelectionDialogs in turn.
7.2 SelectionDialogs
The SelectionDialog provides a ScrolledList that allows the user to select from a list of choices, as well as a TextField
where the user can type in choices. When the user makes a selection from the list, the selected item is displayed in the
text entry area. The user can also type new or existing choices into the text entry area directly. The dialog does not
take any action until the user activates one of the buttons in the action area or presses the RETURN key. If the user
double−clicks on an item in the List, the item is displayed in the text area and the OK button is automatically
activated. the source code demonstrates the use of a SelectionDialog. XtSetLanguageProc() is only available in
X11R5; there is no corresponding function in X11R4. XmStringCreateLocalized() is only available in Motif
1.2; XmStringCreateSimple() is the corresponding function in Motif 1.1. XmFONTLIST_DEFAULT_TAG
replaces XmSTRING_DEFAULT_CHARSET in Motif 1.2.
/* select_dlg.c −− display two pushbuttons: days and months.
* When the user selections one of them, post a selection
* dialog that displays the actual days or months accordingly.
* When the user selects or types a selection, post a dialog
* the identifies which item was selected and whether or not
* the item is in the list.
*
* This program demonstrates how to use selection boxes,
* methods for creating generic callbacks for action area
* selections, abstraction of data structures, and a generic
* MessageDialog posting routine.
*/
#include <Xm/SelectioB.h>
#include <Xm/RowColumn.h>
#include <Xm/MessageB.h>
#include <Xm/PushB.h>
Widget PostDialog();
char *days[] = {
"Sunday", "Monday", "Tuesday", "Wednesday",
"Thursday", "Friday", "Saturday"
};
char *months[] = {
"January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"
};
typedef struct {
char *label;
char **strings;
7 Selection Dialogs 7.2 SelectionDialogs
144
int size;
} ListItem;
ListItem month_items = { "Months", months, XtNumber (months) };
ListItem days_items = { "Days", days, XtNumber (days) };
/* main() −−create two pushbuttons whose callbacks pop up a dialog */
main(argc, argv)
char *argv[];
{
Widget toplevel, button, rc;
XtAppContext app;
void pushed();
XtSetLanguageProc (NULL, NULL, NULL);
toplevel = XtVaAppInitialize (&app, "Demos", NULL, 0,
&argc, argv, NULL, NULL);
rc = XtVaCreateWidget ("rowcolumn",
xmRowColumnWidgetClass, toplevel, NULL);
button = XtVaCreateManagedWidget (month_items.label,
xmPushButtonWidgetClass, rc, NULL);
XtAddCallback (button, XmNactivateCallback, pushed, &month_items);
button = XtVaCreateManagedWidget (days_items.label,
xmPushButtonWidgetClass, rc, NULL);
XtAddCallback (button, XmNactivateCallback, pushed, &days_items);
XtManageChild (rc);
XtRealizeWidget (toplevel);
XtAppMainLoop (app);
}
/* pushed() −−the callback routine for the main app's pushbutton.
* Create a dialog containing the list in the items parameter.
*/
void
pushed(widget, client_data, call_data)
Widget widget;
XtPointer client_data;
XtPointer call_data;
{
Widget dialog;
XmString t, *str;
int i;
extern void dialog_callback();
ListItem *items = (ListItem *) client_data;
str = (XmString *) XtMalloc (items−>size * sizeof (XmString));
t = XmStringCreateLocalized (items−>label);
for (i = 0; i < items−>size; i++)
str[i] = XmStringCreateLocalized (items−>strings[i]);
dialog = XmCreateSelectionDialog (widget, "selection", NULL, 0);
XtVaSetValues (dialog,
XmNlistLabelString, t,
XmNlistItems, str,
XmNlistItemCount, items−>size,
XmNmustMatch, True,
NULL);
7 Selection Dialogs 7.2 SelectionDialogs
145
XtSetSensitive (
XmSelectionBoxGetChild (dialog, XmDIALOG_HELP_BUTTON), False);
XtAddCallback (dialog, XmNokCallback, dialog_callback, NULL);
XtAddCallback (dialog, XmNnoMatchCallback, dialog_callback, NULL);
XmStringFree (t);
while (−−i >= 0)
XmStringFree (str[i]); /* free elements of array */
XtFree (str); /* now free array pointer */
XtManageChild (dialog);
XtPopup (XtParent (dialog), XtGrabNone);
}
/* dialog_callback() −−The OK button was selected or the user
* input a name by himself. Determine whether the result is
* a valid name by looking at the "reason" field.
*/
void
dialog_callback(widget, client_data, call_data)
Widget widget;
XtPointer client_data;
XtPointer call_data;
{
char msg[256], *prompt, *value;
int dialog_type;
XmSelectionBoxCallbackStruct *cbs =
(XmSelectionBoxCallbackStruct *) call_data;
switch (cbs−>reason) {
case XmCR_OK:
prompt = "Selection: ";
dialog_type = XmDIALOG_MESSAGE;
break;
case XmCR_NO_MATCH:
prompt = "Not a valid selection: ";
dialog_type = XmDIALOG_ERROR;
break;
default:
prompt = "Unknown selection: ";
dialog_type = XmDIALOG_ERROR;
}
XmStringGetLtoR (cbs−>value, XmFONTLIST_DEFAULT_TAG, &value);
sprintf (msg, "%s%s", prompt, value);
XtFree (value);
(void) PostDialog (XtParent (XtParent (widget)), dialog_type, msg);
if (cbs−>reason != XmCR_NO_MATCH) {
XtPopdown (XtParent (widget));
XtDestroyWidget (widget);
}
}
/*
* PostDialog() −− a generalized routine that allows the programmer
* to specify a dialog type (message, information, error, help,
* etc..), and the message to show.
*/
Widget
PostDialog(parent, dialog_type, msg)
Widget parent;
int dialog_type;
char *msg;
7 Selection Dialogs 7.2 SelectionDialogs
146
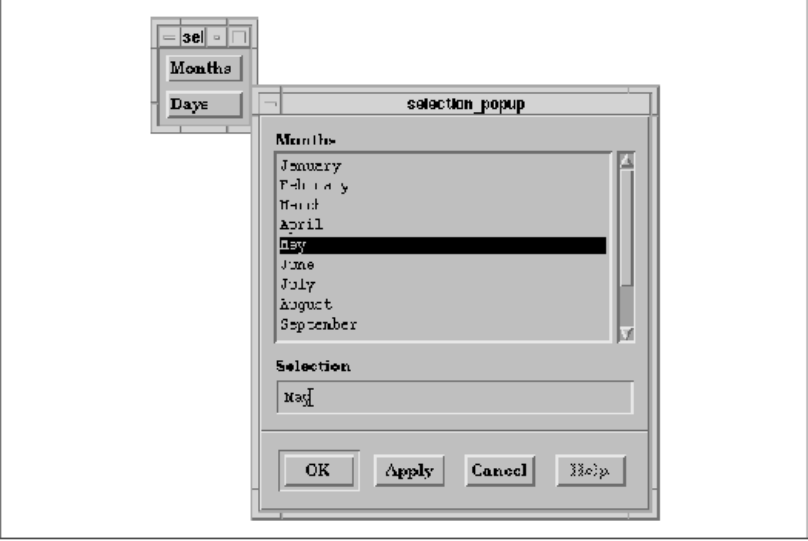
{
Widget dialog;
XmString text;
dialog = XmCreateMessageDialog (parent, "dialog", NULL, 0);
text = XmStringCreateLocalized (msg);
XtVaSetValues (dialog,
XmNdialogType, dialog_type,
XmNmessageString, text,
NULL);
XmStringFree (text);
XtUnmanageChild (
XmMessageBoxGetChild (dialog, XmDIALOG_CANCEL_BUTTON));
XtSetSensitive (
XmMessageBoxGetChild (dialog, XmDIALOG_HELP_BUTTON), False);
XtAddCallback (dialog, XmNokCallback, XtDestroyWidget, NULL);
XtManageChild (dialog);
return dialog;
}
The output of the program is shown in the figure.
Output of select_dlg.c
The program displays two PushButtons, one for months and one for the days of the week. When either button is
activated, a SelectionDialog that displays the list of items corresponding to the button is popped up. In keeping with
the philosophy of modular programming techniques, we have broken the application into three routines −− two
callbacks and one general−purpose message posting function. The lists of day and month names are stored as arrays of
strings. We have declared a data structure, ListItem, to store the label and the items for a list. Two instances of this
data structure are initialized to the correct values for the lists of months and days. We pass these data structures as the
client_data to the callback function pushed(). This callback routine is associated with both of the
7 Selection Dialogs 7.2 SelectionDialogs
147
Get Volume 6A: Motif Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.