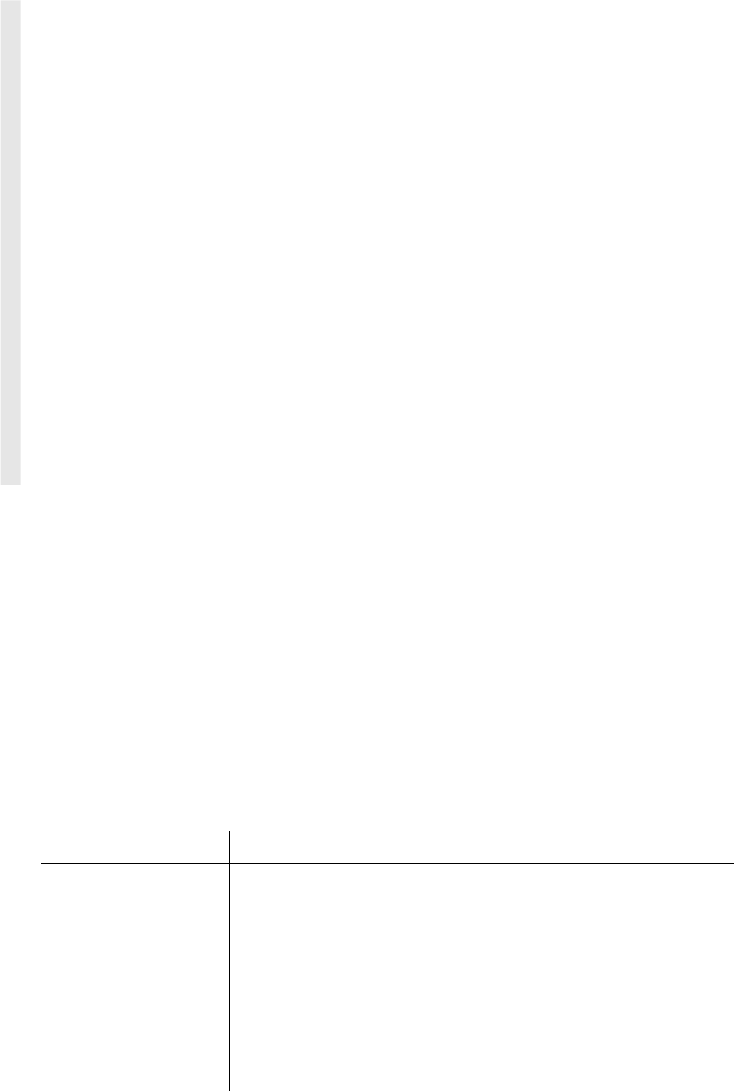
Example 18-4. Sample reply procedure
–
SelectionReplyProc (continued)
if (target == ATOM(server, "STRING")) {
static int incr = False;
if (type == ATOM(server, "INCR")) {
textsw_insert(textsw, "Contents of the selection:\n", 27);
incr = True;
}
else
if (length) {
if (!incr)
textsw_insert(textsw, "Contents of the selection:\n", 27);
textsw_insert(textsw, (char *)value, length);
textsw_insert(textsw, "\n", 1);
}
else
incr = False;
}
else
if (target == ATOM(server, "DELETE")) {
textsw_insert(textsw, "The Selection has been deleted\n", 31);
}
textsw_insert(textsw, LINE, strlen(LINE));
}
18.2.5.1 Handling selection reply procedure errors
If the selection conversion fails, and you are using a non-blocking request, the reply proce-
dure is called with replyValue set to an error code and length set to SEL_ERROR. The reply
procedure error codes are defined in sel_pkg.h and shown in Table 18-2.
If the selection conversion fails, and you are using a blocking request without a reply proce-
dure defined, the xv_get() returns with the length argument set to SEL_ERROR, format set
to 0, and a NULL value is returned. If a reply procedure is defined, its replyValue argument is
set to one of the error codes shown in Table 18-2.
Table 18-2. Error Codes
Code Description
SEL_BAD_CONVERSION If the conversion is refused by the selection holder or there
is no holder of the selection, this value is returned. This
may mean that there is no owner for the selection, that the
owner does not support the conversion implied by target, or
that the server did not have sufficient space.
SEL_BAD_TIME The SelectionNotify time does not match the package time
value.
SEL_BAD_WIN_ID The SelectionNotify requestor ID does not match the pack-
age requestor ID.
408 XView Programming Manual

Table 18-2. Error Codes (continued)
Code Description
SEL_BAD_PROPERTY Obsolete.
SEL_BAD_PROPERTY_EVENT Obsolete.
SEL_PROPERTY_DELETED Obsolete.
SEL_TIMEDOUT Selection timed out.
A sample application-defined errror routine, for a non-blocking error handler, is shown in
Example 18-5. Code similar to this should be invoked to handle errors in your selection
application.
Example 18-5. Sample selection reply error handler
SelectionError(sel, target, errorCode)
Selection_requestor sel;
Atom target;
int errorCode;
{
Atom rank;
char *rank_string;
char *target_string = (char *)xv_get(server,
SERVER_ATOM_NAME, target);
char msg[100];
rank = (Atom)xv_get(sel, SEL_RANK);
rank_string = (char *)xv_get(server, SERVER_ATOM_NAME, rank);
sprintf(msg, "Selection failed for rank ‘‘%s’’ on target ‘‘%s’’: ",
rank_string, target_string);
textsw_insert(textsw, msg, strlen(msg));
switch(errorCode) {
case SEL_BAD_CONVERSION :
textsw_insert(textsw, "Conversion Rejected",
strlen("Conversion Rejected"));
break;
case SEL_BAD_TIME:
textsw_insert(textsw, "Bad Time Match",
strlen("Bad Time Match"));
break;
case SEL_BAD_WIN_ID:
textsw_insert(textsw, "Bad Window Match",
strlen("Bad Window Match"));
break;
case SEL_TIMEDOUT:
textsw_insert(textsw, "Timeout", strlen("Timeout"));
break;
}
}
Selections
Selections 409
18.2.6 If the Selection is Lost (Selection Owner)
If the owner loses the selection, because someone else acquired the same rank selection, the
lose procedure is called. The selection-owner object attribute SEL_LOSE_PROC specifies the
selection owner’s lose procedure. This procedure is called by the toolkit, to inform the
selection owner that it has lost the ownership of the given selection. The form of the lose
procedure is shown below:
void
lose_proc( sel )
Selection_owner sel;
sel specifies the selection-owner object.
One example of lose_proc() usage is for unhighlighting the highlighted text in a
textsw. The code fragment below shows a selection lose procedure that simply informs the
user that the selection was lost.
void
SelectionLoseProc(sel)
Selection_owner sel;
{
xv_set(frame, FRAME_LEFT_FOOTER, "Lost Selection...", NULL);
}
18.2.7 Cleanup – When the Selection Completes (Selection Owner)
The attribute
SEL_DONE_PROC
specifies the application-defined done procedure that is called
by the toolkit when a selection request has successfully completed. It is called once follow-
ing each successful transfer of data to the requestor. Thus, if the selection request is a multi-
ple, or results in an incremental reply, the done procedure is called more than once. This pro-
cedure can be used to deallocate any selection replies allocated in the conversion procedure.
void
done_proc( sel, replyBuff, target )
Selection_owner sel;
Xv_opaque replyBuff;
Atom target;
The argument sel specifies the selection-owner object. replyBuff specifies the address which
contains the converted data. target specifies the target type returned by the conversion pro-
cedure.
410 XView Programming Manual
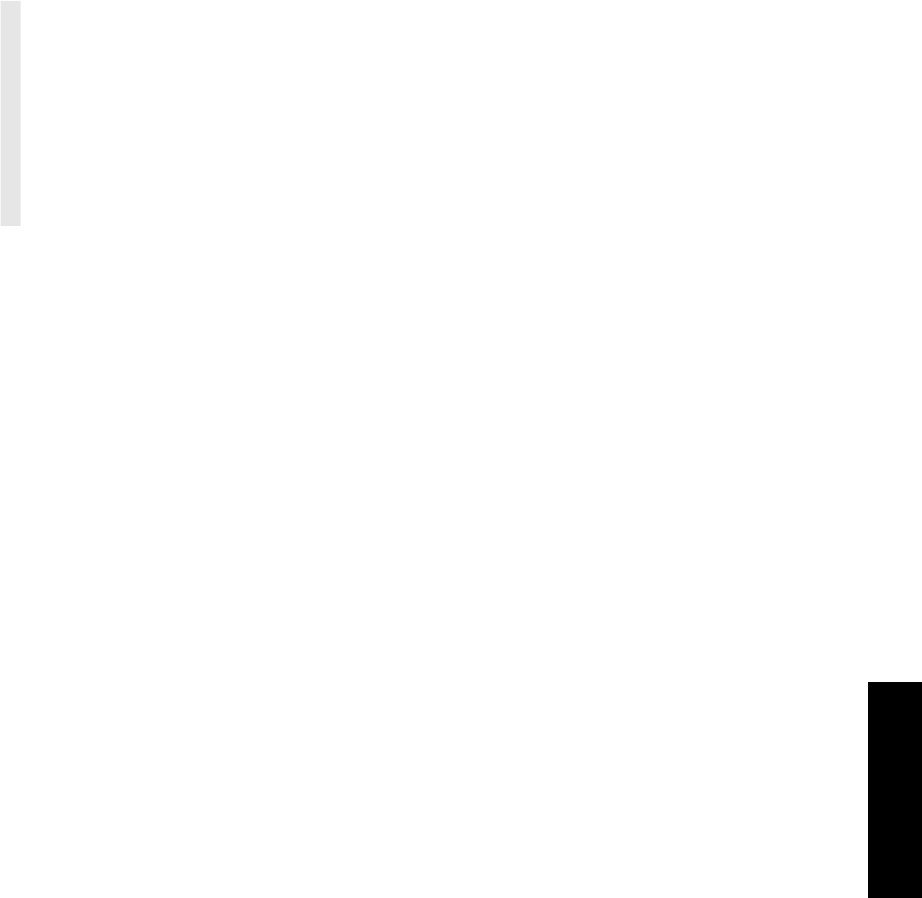
Example 18-6 shows a sample done procedure.
Example 18-6. Sample done procedure
–
SelectionDoneProc
void
SelectionDoneProc(sel, data, target)
Selection_owner sel;
Xv_opaque data;
Atom target;
{
if (target == ATOM(server, "STRING"))
free((char *)data);
}
18.3 How Selection Works (With a Selection Item)
This section describes using a selection item that can eliminate the selection owner’s need for
a conversion procedure. This may simplify the programmer’s job of implementing a
selection transfer.
Selection items are good to use for conversions that involve static data—data that will not
change over the lifetime of the selection. Do not use a selection item for selections where the
data associated with the selection is not known until the selection request is received (using a
selection conversion procedure is best for this case). Also, do not use a selection item for any
selection conversion that has side effects (for example, a delete request).
Below is a brief overview of the steps that take place during a single transfer of data from
one XView application to another using a selection item. We’ll assume we have two applica-
tions, application A and application B, either of which can operate as the owner or the reques-
tor.
1. (Owner side.) The user makes a selection in application A and the application highlights
the selection.
2. (Owner side.) The user may pre-register a conversion for a particular target by creating a
selection-item object and setting its attributes.
3. (Owner side.) In the XView Selection package, nothing further happens until an event of
interest is detected, for example, an ACTION_COPY event. At this time, a selection item
may claim ownership of the current selection in application A. Also at this time,
selection data may be attached to the selection-item object.
4. (Requestor side.) The user pastes the selected data into application B, causing an
ACTION_PASTE event to occur.
5. (Requestor side.) The ACTION_PASTE event may cause the Selection Requestor to make
a non-blocking request to receive the selected data into application B.
Selections
Selections 411
6. (Owner side.) When there is a match between a selection-item object’s target type, and a
requested target, the selection package converts and sends the selection data to the
selection requestor. XView internally handles the data conversion by calling the pack-
age’s default conversion procedure sel_convert_proc().
7. (Requestor side.) Once the data conversion has completed (either successfully or unsuc-
cessfully), the application-defined reply procedure is invoked. The data may displayed in
application B (if the conversion routine was successful) or the user may simply be given
some indication that data was received. Other possibilities include: the kind of data
selected in application A cannot be pasted in application B, or that the kind of data
requested by B cannot be supplied by A.
8. (Owner side.) Once the selection transfer is complete, an application-defined “done” pro-
cedure may be called by the selection owner. The done procedure may be used to free the
memory associated with the selection, or to perform other cleanup that is required.
9. (Owner side.) If another application acquires the selection, application A’s “lose” proce-
dure is called. This procedure is used to handle notification of loosing selection owner-
ship. It tells the selection owner (application A) that it has lost ownership of the
selection. For example, the lose procedure might unhighlight text that was previously
selected and highlighted.
18.3.1 The Selection Item
The first task for the application that is to become the selection owner, using a selection-item
object, is to pre-register a conversion or several conversions. Below, we describe this step in
detail. Also, the selection needs to be marked. For example, when the pointer is placed over
text in a canvas, an
ACTION_SELECT should highlight the selection. While LOC_DRAG occurs,
the highlight would be extended over the selection.
After the user makes a selection and it is marked, the application waits to receive an event of
interest. For example, in a text subwindow, the act of selecting an item causes the primary
rank to be acquired. For the text subwindow example, an ACTION_COPY event causes the
CLIPBOARD selection to be acquired (note that in this case two selections are acquired since
the
COPY operation in OPEN LOOK uses the clipboard).
With a selection item, applications perform three steps to become the selection owner:
1. A Selection_owner object must be created using xv_create() (assuming a previ-
ously created selection-owner object is not being re-used.) A selection-item object is
created and its attributes are set.
2. The selection must be acquired.
3. The selection data is associated with the selection item.
412 XView Programming Manual
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.