19.2.2.1 Preview events
The attribute DROP_SITE_EVENT_MASK sets a mask on the drop-site item to specify if the
region(s) within the drop-site should receive preview events. Preview events indicate the
state of the mouse relative to the drop-site. Preview events are indicated when
event_action() returns ACTION_DRAG_PREVIEW (in this case, event_id() returns
either LOC_WINENTER or LOC_WINEXIT). These events cue the drop-site when the mouse
enters or leaves a drop-site region. Drop-sites can also select for LOC_DRAG events that are
sent as the mouse moves across a region. These events are delivered to the event procedure of
the drop-site item’s owner, (the pointer coordinates are contained within the event). The
coordinates are in the drop-site owner’s coordinate space.
DROP_SITE_EVENT_MASK is only a hint since there is no guarantee the source of the drag will
send previewing events. When preview events arrive, an application may invert the image of
the drop-site. This or some other change that indicates the area is an acceptable drop-site.
An uninterpreted ID specified with the attribute DROP_SITE_ID may be used by the applica-
tion program to distinguish one drop-site from the next. This attribute is useful when more
than one drop-site has been set on an object. This
ID is sent along with the
ACTION_DRAG_PREVIEW, ACTION_DRAG_MOVE, and ACTION_DRAG_COPY events. If no
DROP_SITE_ID is set, the package creates a unique ID for each drop-site region.
19.2.2.2 Event forwarding
The procedure dnd_is_forwarded() lets an application determine if a drop or preview
event is forwarded from some other site. This may happen when the user drops on the win-
dow manager’s decoration window, or on a icon when the application is iconified (only if
DROP_SITE_DEFAULT is set). The corresponding drop or preview event will have its
DND_FORWARDED flag set.
In general, if an application handles previewing, it should check to see if the preview event
was forwarded. If the event was forwarded, it should not invert or highlight the drop-site.
19.2.2.3 Handling drop and preview events
A preview event is sent to the drop-site owner when the user is dragging over a registered
drop-site. A drop event is sent to the drop-site owner when the user is dragging over a regis-
tered drop-site and releases the mouse buttons. There are two ways that the drop-site owner
can handle such events in order to preview or to receive the drop:
• The window can set a
WIN_EVENT_PROC and define a callback procedure to handle the
drop.
• The window can use a notification interposing routine.
If your application uses an interposing routine, two types of interposition procedures must be
registered for receiving both drop and previewing events. Both a safe, NOTIFY_SAFE, and an
immediate, NOTIFY_IMMEDIATE, interposer needs to be registered. If both safe and immedi-
438 XView Programming Manual

ate interposers are not registered, there are cases when events may be delayed in arriving at
the drop-site and may not arrive “on time.”
19.3 Sourcing the Drag
The application containing the source for the drag and drop determines the point to initiate
the drag operation. For example, a drag operation could be initiated when text or a file icon is
selected and dragged. What events determine the initiation point are entirely up to the appli-
cation programmer.
Sometime before, or when an application initiates a drag and drop operation it needs to create
a drag and drop object and set its attributes. An instance of a DRAGDROP object is created
using xv_create():
dnd_object = xv_create(owner, DRAGDROP,
<attribute-value list>,
NULL)
A DRAGDROP object’s owner is an Xv_window and the <attribute-value list> may contain
drag and drop attributes (DND prefix) or since the DND package is subclassed from selection
owner package, any of the SELECTION_OWNER attributes. For example, the drag and drop
object dnd below uses a selection attribute:
dnd = (Xv_drag_drop)xv_create(window, DRAGDROP,
DND_TYPE, DND_COPY
DND_X_CURSOR, arrow_cursor,
DND_ACCEPT_CURSOR, drop_here_cursor,
SEL_CONVERT_PROC, SelectionConvert,
NULL);
The owner of the drag and drop object should be the window in which the drag operation is
initiated from. Since the DRAGDROP object is used as part of the selection transaction that
implements the drag and drop operation, the selection transaction must also be completed
before the DRAGDROP object can be reused.
NOTE
A drag and drop object can only be used in one drag and drop operation at a
time.
The attribute
DND_TYPE defines whether the drag and drop operation will be a copy or a
move. This is just a hint to the destination. If the type is a DND_MOVE operation and if the des-
tination honors the hint, the destination asks the source to convert the DELETE target. The
DND_ACCEPT_CURSOR attribute, and other cursor related attributes are described in the Sec-
tion 19.3.3, “Defining the Drag/Accept Cursor.”
Once the DRAGDROP object is created, it can be used to initiate a drag and drop operation by
calling dnd_send_drop().
Drag and Drop
Drag and Drop 439

19.3.1 Initiating the Drop Operation
To initiate a drag operation, after the drag and drop object is created, an application calls
dnd_send_drop(). This procedure is defined by XView and is responsible for changing
the root cursor, sending previewing events to appropriate drop-sites and sending a trigger
message, an event of type ACTION_DRAG_COPY or ACTION_DRAG_MOVE, to the drop-site that
is to receive the drop. In sequence, dnd_send_drop() does the following:
• Creates a unique selection if this is required.
• Grabs the pointer and changes the cursor.
• Sends preview events to possible drop-sites.
• Sends the kicker message to the drop-site to initiate the transfer.
• Insures that the recipient acknowledges the drop.
• Returns a status.
For example, an application such as filemgr calls this routine when the user drags the
mouse for a number of pixels over a selected file icon.
The form for dnd_send_drop() is:
int
dnd_send_drop(object)
Dnd object;
Dnd is a drag and drop object: dnd_send_drop() does not return until the user releases
all of the mouse buttons, drops the object, or hits the STOP key. The procedure
dnd_send_drop() returns one of the values shown in Table 19-2.
Table 19-2. dnd_send_drop() Return Values
Return Value Description
DND_ILLEGAL_TARGET
The user dropped on an object that has not registered interest in
drag and drop.
DND_ROOT The user dropped on the root window.
DND_SELECTION A unique selection could not be obtained.
DND_TIMEOUT The destination did not respond to the kicker message (the
drop).
DND_ABORTED
The user aborted the drag operation by hitting the STOP key.
XV_OK The drag and drop operation has begun.
XV_ERROR An unexpected error occurred.
440 XView Programming Manual
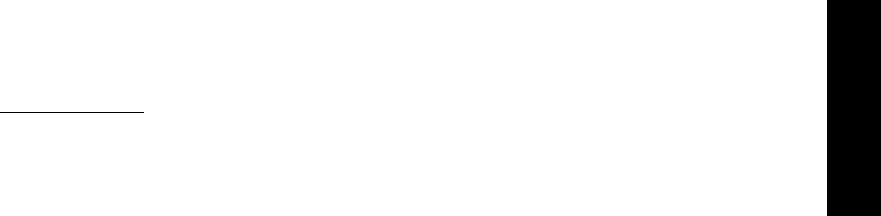
19.3.2 Interaction with the Selection Package
Using drag and drop, data is transferred through selections. Since the DRAGDROP package is
subclassed from the SELECTION_OWNER package, it fully supports selections. A selection is
associated with the drag and drop object which allows the drop-site item’s owner to obtain
the data through selection transactions. The selection is normally a transient selection that
persists only for the duration of the drag and drop operation. A transient selection should be
used even if the dragged object is already associated with a selection such as the primary
selection (PRIMARY). If the primary selection were used, and another application acquired
the primary selection during the drag and drop transfer, the drag and drop operation would
fail.
The application can use the SEL_RANK attribute to associate a selection with the drag and
drop object. If the application does not own a selection (SEL_OWN) at the time
dnd_send_drop() is called, XView creates a transient selection and associates it with the
drag and drop object. This selection can be obtained by using xv_get() on the drag and
drop object with the SEL_RANK attribute. It is the responsibility of the application to disown a
selection associated with the drag and drop object when it has completed the operation. This
is regardless of whether the application or the toolkit initially established ownership of the
selection.
If XView created a transient selection and dnd_send_drop() failed (did not return
XV_OK), the toolkit will disown the selection.
Since the source will be required to reply to requests from the destination for data conver-
sion, the source should have either a conversion procedure or selection items set on the drag
and drop object before it calls dnd_send_drop().
19.3.3 Defining the Drag/Accept Cursor
The cursor changes when an object is being dragged. The cursor used to indicate dragging an
object is set with
DND_CURSOR. This attribute changes the pointer used during the drag por-
tion of the drag and drop operation. The default drag cursor is the predefined OPEN LOOK
drag cursor. DND_X_CURSOR is an alternative to DND_CURSOR; it accepts an XID of a cursor
instead of an Xv_object.
The attribute DND_ACCEPT_CURSOR defines the special “accept” cursor that is used when the
mouse is over an acceptable drop-site. The default value for this attribute is a predefined
OPEN LOOK
drag and drop cursor. DND_ACCEPT_X_CURSOR is an alternative way to set the
accept cursor. This attribute accepts an XID of a cursor instead of an Xv_cursor.*
The cursor package also provides special support for drag and drop cursors for text. Refer to
Chapter 13, Cursors, for information on these special drag and drop cursors.
*Future implementations of this package will also support a DND_REJECT_CURSOR that may be used when drop-
sites are currently unreceptive to drops.
Drag and Drop
Drag and Drop 441
19.3.4 Timeout Value
The attribute DND_TIMEOUT_VALUE defines the amount of time to wait for an acknowledg-
ment from the drop destination after the kicker message has been sent to the source
(ACTION_DRAG_COPY or ACTION_DRAG_MOVE).
19.4 Receiving a Drop
An application that has registered a drop-site may receive a drop. A window or object that
owns the drop-site item may receive one of two events when a valid drop-site is dropped on.
An ACTION_DRAG_COPY indicates a copy and an ACTION_DRAG_MOVE indicates a move.
These events are the trigger message that indicate that a drop has happened.
Upon receiving a drop event, the application should call dnd_decode_drop() and pass in
as a parameter the ACTION_DRAG_MOVE or ACTION_DRAG_COPY event that it received along
with an instantiated
SELECTION_REQUESTOR object. The SELECTION_REQUESTOR object is
used since drag and drop data transfers use the selection package to facilitate the transfer.
The dnd_decode_drop() function initiates a data transfer using the selection mecha-
nism. It decodes the drop event as follows:
• It associates the selection rank defined within the drop event with the selection object that
was passed into dnd_decode_drop().
• It sends an acknowledgment to the source of the drag and drop, informing it that the
transaction has begun.
• It returns the drop-site item that was dropped on if dnd_decode_drop() could begin
the drag and drop transaction or returns
XV_ERROR if it fails to initiate the drag and drop
transaction. This may happen when the selection defined within the drop event does not
exist.
The form of the procedure dnd_decode_drop() is:
Xv_drop_site
dnd_decode_drop(sel_object, drop_event)
Selection_requestor sel_object;
Event *drop_event;
If dnd_decode_drop() returns a drop-site item, it is the responsibility of the application
to continue the drag and drop transaction. This occurs by making selection requests on the
selection object passed into dnd_decode_drop(). See Chapter 18, Selections, for details
on selection transfers.
The data transfer mechanism for sending and receiving drop data is not limited to using
selections. Any mechanism could be used for the transfer, including: the file system, sockets,
or one of the other Alternate Transport Mechanisms. Typically, such an alternate transfer
mechanism would be initiated after the initial selection request.
442 XView Programming Manual
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.