
Example 19-1. Sourcing a drag (continued)
if (dragging && drag_pixels++ == 5) {
xv_set(frame, FRAME_LEFT_FOOTER, "Drag and Drop:", 0);
switch (dnd_send_drop(dnd)) {
case XV_OK:
xv_set(frame, FRAME_LEFT_FOOTER,
"Drag and Drop: Began", 0);
break;
case DND_TIMEOUT:
xv_set(frame, FRAME_LEFT_FOOTER,
"Drag and Drop: Timed Out",0);
break;
case DND_ILLEGAL_TARGET:
xv_set(frame, FRAME_LEFT_FOOTER,
"Drag and Drop: Illegal Target",0);
break;
case DND_SELECTION:
xv_set(frame, FRAME_LEFT_FOOTER,
"Drag and Drop: Bad Selection",0);
break;
case DND_ROOT:
xv_set(frame, FRAME_LEFT_FOOTER,
"Drag and Drop: Root Window",0);
break;
case XV_ERROR:
xv_set(frame, FRAME_LEFT_FOOTER,
"Drag and Drop: Failed",0);
break;
}
drag_pixels = 0;
}
}
break;
}
}
PaintObject(object, win, dpy)
DragObject object;
Window win;
Display *dpy;
{
static GC gc;
static int gcCreated = False;
if (!gcCreated) {
XGCValues gcv;
gcv.stipple = (Pixmap) xv_get(object.image, XV_XID);
gcv.fill_style = FillStippled;
gc = XCreateGC(dpy, win, GCStipple|GCForeground|GCBackground|
GCFillStyle, &gcv);
XSetForeground(dpy, gc, BlackPixel(dpy, XDefaultScreen(dpy)));
XSetBackground(dpy, gc, WhitePixel(dpy, XDefaultScreen(dpy)));
}
if (object.inverted) {
XSetFillStyle(dpy, gc, FillSolid);
448 XView Programming Manual
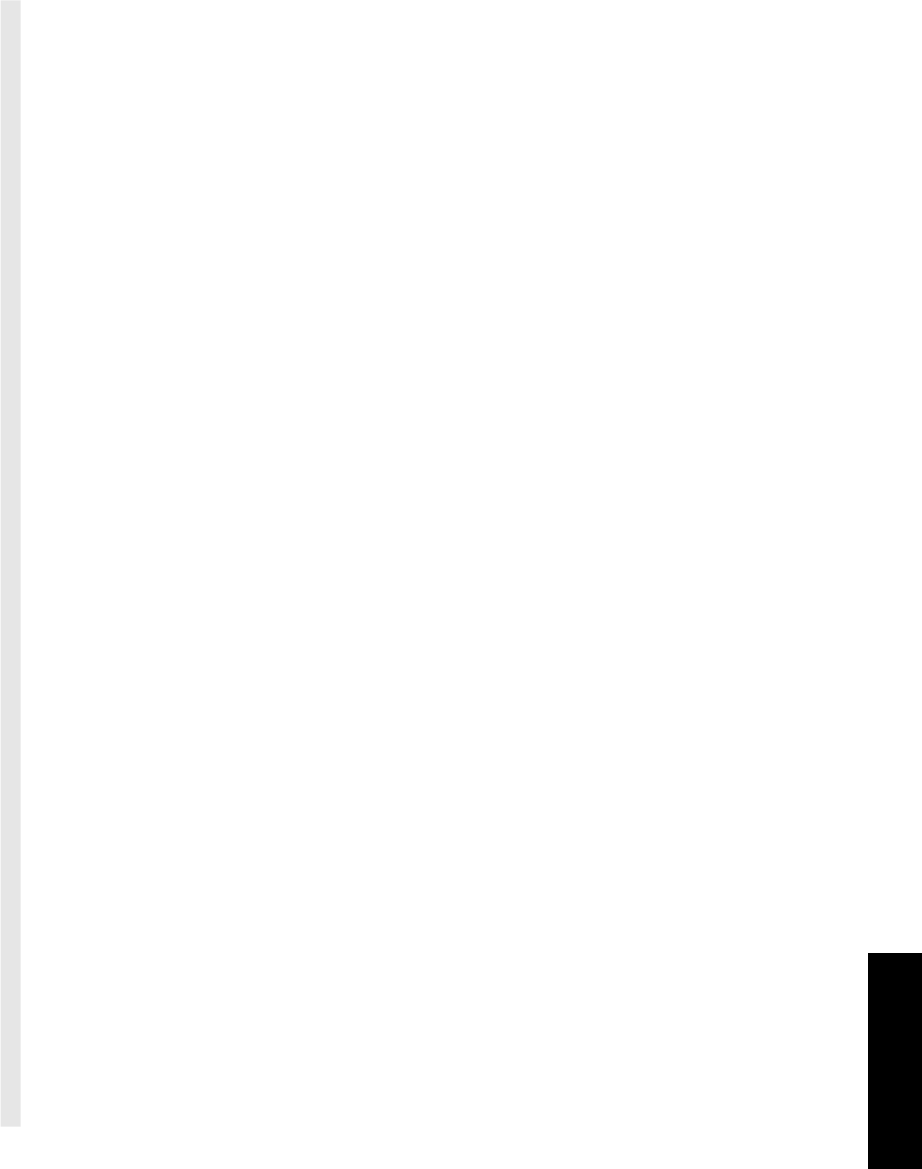
Example 19-1. Sourcing a drag (continued)
XDrawRectangle(dpy, win, gc, object.x-1, object.y-1, 66, 66);
XSetFillStyle(dpy, gc, FillStippled);
} else
XClearWindow(dpy, win);
XSetTSOrigin(dpy, gc, object.x, object.y);
XFillRectangle(dpy, win, gc, object.x, object.y, 65, 65);
}
void
PaintCanvas(canvas, paint_window, dpy, xwin, xrects)
Canvas canvas; /* unused */
Xv_Window paint_window; /* unused */
Display *dpy;
Window xwin;
Xv_xrectlist *xrects; /* unused */
{
unsigned width, height;
int x, y;
width = xv_get(paint_window, XV_WIDTH);
height = xv_get(paint_window, XV_HEIGHT);
x = (width/2)-(dO.w/2);
y = (height/2)-(dO.h/2);
dO.x = x;
dO.y = y;
PaintObject(dO, xwin, dpy);
}
/* The convert proc is called whenever someone makes a request
* to the dnd selection. Two cases we handle within the convert
* proc: DELETE and _SUN_SELECTION_END. Everything else we pass
* on to the default convert proc which knows about our selection
* items.
*/
int
SelectionConvert(seln, type, data, length, format)
Selection_owner seln;
Atom *type;
Xv_opaque *data;
long *length;
int *format;
{
Xv_Server server = XV_SERVER_FROM_WINDOW(xv_get(seln,
XV_OWNER));
if (*type == (Atom)xv_get(server,
SERVER_ATOM, "_SUN_SELECTION_END")) {
/* Destination has told us it has completed the drag
* and drop transaction. We should respond with a
* zero-length NULL reply.
*/
xv_set(dnd, SEL_OWN, False, 0);
xv_set(frame, FRAME_LEFT_FOOTER, "Drag and Drop: Completed",0);
Drag and Drop
Drag and Drop 449

Example 19-1. Sourcing a drag (continued)
*format = 32;
*length = 0;
*data = NULL;
*type = (Atom)xv_get(server, SERVER_ATOM, "NULL");
return(True);
} else if (*type == (Atom)xv_get(server,
SERVER_ATOM, "DELETE")) {
/* Destination asked us to delete the selection.
* If it is appropriate to do so, we should.
*/
*format = 32;
*length = 0;
*data = NULL;
*type = (Atom)xv_get(server, SERVER_ATOM, "NULL");
return(True);
} else
/* Let the default convert procedure deal with the
* request.
*/
return(sel_convert_proc(seln, type, data, length, format));
}
/* When we lose the primary selection, this procedure is called.
* We dehigh-light our selection.
*/
void
SelectionLose(seln)
Selection_owner seln;
{
Xv_Window owner = xv_get(seln, XV_OWNER);
if (xv_get(seln, SEL_RANK) == XA_PRIMARY) {
dO.inverted = False;
PaintObject(dO, xv_get(owner,
XV_XID), XV_DISPLAY_FROM_WINDOW(owner));
}
}
19.6 Sample Program—Drop Site Item and Destination
Example 19-2 provides a example of a program that creates a drop-site item and sets up to
receive a drop.
Example 19-2. A drop-site item example
/*
* dest.c - Example of how to register interest in receiving
* drag and drop events and how to complete a drag
* and drop operation.
*
*/
#include <stdio.h>
450 XView Programming Manual
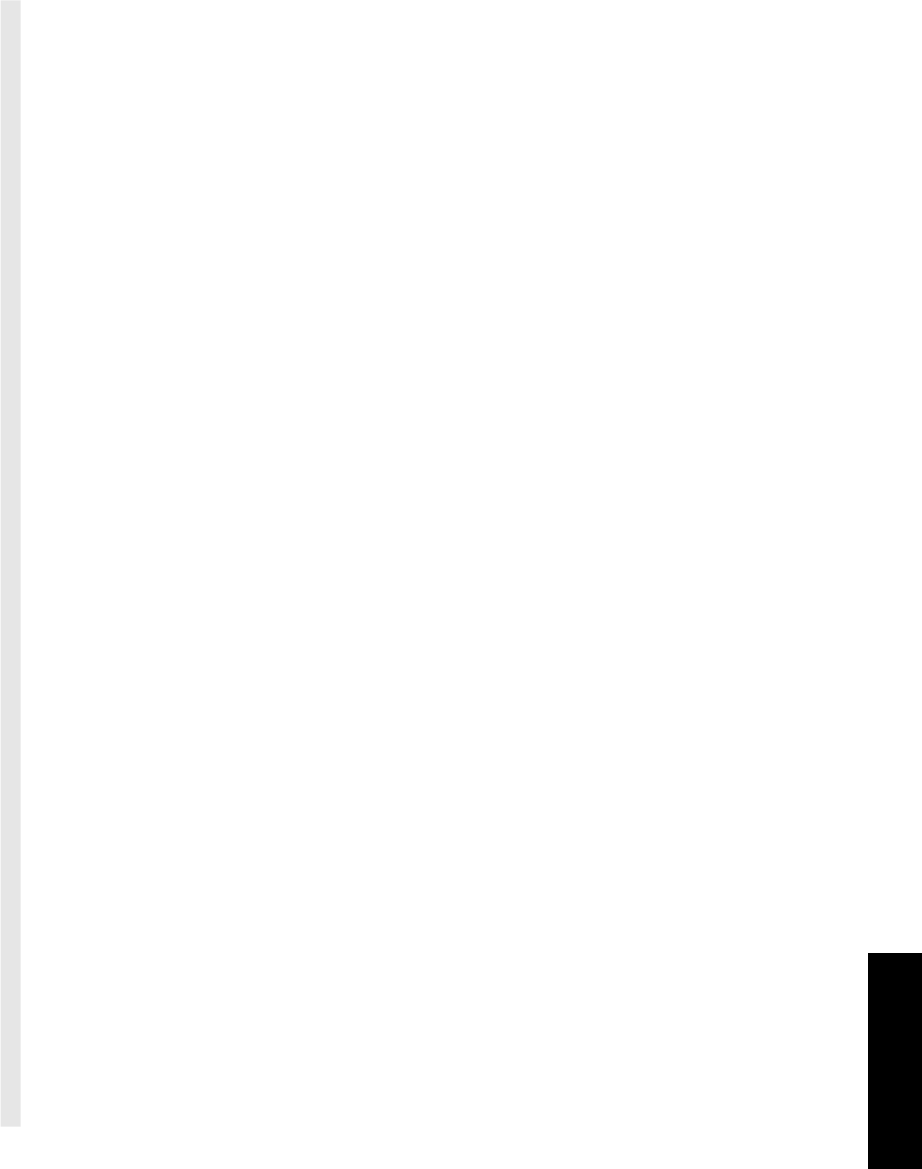
Example 19-2. A drop-site item example (continued)
#include <xview/xview.h>
#include <xview/frame.h>
#include <xview/canvas.h>
#include <xview/panel.h>
#include <xview/font.h>
#include <xview/dragdrop.h>
#include <xview/xv_xrect.h>
#include <X11/Xlib.h>
#define DROP_WIDTH 65
#define DROP_HEIGHT 65
#define BULLSEYE_SITE 1
Frame frame;
Canvas canvas;
Panel panel;
Xv_drop_site drop_site;
Server_image drop_image;
Server_image drop_image_inv;
Panel_item p_string,
p_length,
p_host;
Selection_requestor sel;
int inverted;
main(argc, argv)
int argc;
char **argv;
{
void EventProc(),
PaintCanvas(),
ResizeCanvas();
Xv_Font font;
xv_init(XV_INIT_ARGC_PTR_ARGV, &argc, argv, NULL);
frame = xv_create((Window)NULL, FRAME,
XV_X, 330,
XV_Y, 10,
XV_WIDTH, 10,
XV_LABEL, "Drag & Drop Destination",
NULL);
panel = xv_create(frame, PANEL,
PANEL_LAYOUT, PANEL_VERTICAL,
NULL);
p_string = xv_create(panel, PANEL_TEXT,
PANEL_LABEL_STRING, "Dropped Text:",
PANEL_VALUE_DISPLAY_LENGTH, 50,
NULL);
p_host = xv_create(panel, PANEL_TEXT,
Drag and Drop
Drag and Drop 451

Example 19-2. A drop-site item example (continued)
PANEL_LABEL_STRING, "From host:",
PANEL_VALUE_DISPLAY_LENGTH, 15,
NULL);
p_length = xv_create(panel, PANEL_TEXT,
PANEL_LABEL_STRING, "Length:",
PANEL_VALUE_DISPLAY_LENGTH, 6,
NULL);
window_fit(panel);
canvas = xv_create(frame, CANVAS,
XV_HEIGHT, 100,
XV_WIDTH, WIN_EXTEND_TO_EDGE,
XV_X, 0,
WIN_BELOW, panel,
CANVAS_REPAINT_PROC, PaintCanvas,
CANVAS_RESIZE_PROC, ResizeCanvas,
CANVAS_X_PAINT_WINDOW, TRUE,
NULL );
xv_set(canvas_paint_window(canvas),
WIN_BIT_GRAVITY, ForgetGravity,
WIN_CONSUME_EVENTS,
WIN_RESIZE,
NULL,
WIN_EVENT_PROC, EventProc,
NULL);
drop_image = xv_create(NULL, SERVER_IMAGE,
SERVER_IMAGE_BITMAP_FILE, "./bullseye.bm",
NULL);
drop_image_inv = xv_create(NULL, SERVER_IMAGE,
SERVER_IMAGE_BITMAP_FILE, "./bullseyeI.bm",
NULL);
/* Selection requestor object that will be
* passed into dnd_decode_drop() and later used
* to make requests to the source of the
* drop.
*/
sel = xv_create(canvas, SELECTION_REQUESTOR, NULL);
/* This application has one drop site with
* site id BULLSEYE_SITE and whose shape will
* be described by a rectangle. If
* animation is supported, it would like to
* receive LOC_DRAG, LOC_WINENTER and
* LOC_WINEXIT events.
*/
drop_site = xv_create(canvas_paint_window(canvas), DROP_SITE_ITEM,
DROP_SITE_ID, BULLSEYE_SITE,
DROP_SITE_EVENT_MASK, DND_ENTERLEAVE,
NULL);
inverted = False;
452 XView Programming Manual
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.