
NOTE
These facilities should rarely be used by clients; a client should normally rely on
XView’s default scheduling and prioritizing scheme.
20.12.1 Prioritization
The order in which a particular client’s conditions are notified may be controlled by provid-
ing a prioritizer operation. Assuming asynchronous or immediate notifications have
already been sent, the default prioritizer makes its notifications in the following order:
• Interval timer notifications (ITIMER_REAL and then ITIMER_VIRTUAL).
• Child process control notifications.
• Synchronous signal notifications by ascending signal numbers.
• Exception file descriptor activity notifications by ascending fd numbers.
• Handle client events by order in which received.
• Output file descriptor activity notifications by ascending fd numbers.
• Input file descriptor activity notifications by ascending fd numbers.
20.12.1.1 Providing a prioritizer
This section describes how a client can provide its own prioritizer.
Notify_func
notify_set_prioritizer_func(client, prioritizer_func)
Notify_client client;
Notify_func prioritizer_func;
The function notify_set_prioritizer_func() takes an opaque client handle and
the function to call before any notifications are sent to client. The previous function that
would have been called is returned. If this function was never defined, then the default prior-
itization function is returned. If the prioritizer_func() argument supplied is
NOTIFY_FUNC_NULL, then no client prioritization is done for client and the default priori-
tizer is used.
The calling sequence of a prioritizer function is shown below:
Notify_value
prioritizer_func(client, nfd, ibits_ptr, obits_ptr
ebits_ptr, nsig, sigbits_ptr, auto_sigbits_ptr,
event_count_ptr, events, args)
Notify_client client;
fd_set *ibits_ptr, *obits_ptr, *ebits_ptr;
int nfd, nsig, *sigbits_ptr, *auto_sigbits_ptr;
*event_count_ptr;
Notify_event *events;
504 XView Programming Manual

Notify_arg *args;
#define SIGBIT(sig) (1 << ((sig) -1))
In this function, client from the associated notify_set_prioritizer_func() is
passed to prioritizer_func(). In addition, all the notifications that the Notifier is
planning on sending to client are described in the other parameters. This data reflects
only data that client has expressed interest in by asking for notification of these condi-
tions. The remaining arguments and the return values are described in the XView Reference
Manual.
20.12.1.2 Dispatching events
From within a prioritization routine, the following functions are called to cause the specified
notifications to be sent:
• notify_event()
• notify_input()
• notify_output()
• notify_exception()
• notify_itimer()
• notify_signal()
• notify_wait3()
The notifier won’t send any notifications it wasn’t planning on sending anyway, so one can’t
use these calls to drive clients programmatically. A return value of
NOTIFY_OK indicates that
client was sent the notification. The return value for an unknown client,
NOTIFY_UNKNOWN_CLIENT indicates that client is not recognized by the Notifier and no
notification was sent. A return value of NOTIFY_NO_CONDITION indicates that client does
not have the requested notification pending and no notification was sent.
A client may choose to replace the default prioritizer. Alternatively, a client’s prioritizer
may call the default prioritizer after sending only a few notifications. Any notifications not
explicitly sent by a client prioritizer will be sent by the default prioritizer (when called), in
their normal turn. Once notified, a client will not receive a duplicate notification for the same
event.
Signals indicated by bits in sigbits_ptr should call notify_signal(). Signals in
auto_sigbits_ptr need special treatment:
•
SIGALRM means that notify_itimer() should be called with a which of
ITIMER_REAL.
• SIGVTRM means that notify_itimer() should be called with a which of
ITIMER_VIRTUAL.
• SIGCHLD means that notify_wait3() should be called.
•
SIGTSTP means notify_destroy() should be called with status
Notifier
The Notifier 505

DESTROY_CHECKING.
• SIGTERM means notify_destroy() should be called with status DES-
TROY_CLEANUP
.
• SIGKILL means notify_destroy() should be called with status DESTROY_PRO-
CESS_DEATH
.
Asynchronous signal notifications, destroy notifications, and client event notifications that
were delivered right when they were posted do not pass through the prioritizer.
20.12.1.3 Getting the prioritizer
The routine notify_get_prioritizer_func() returns the current prioritizer of a
client:
Notify_func
notify_get_prioritizer_func(client)
Notify_client client;
This function takes an opaque client handle. The function that will be called before any noti-
fications are sent to client is returned. If this function was never defined for client,
then a default function is returned. A return value of NOTIFY_FUNC_NULL indicates an error.
If client is unknown, then notify_errno is set to NOTIFY_UNKNOWN_CLIENT.
20.12.2 Scheduling the Notifier
Scheduling the notifier allows you to control the order in which clients are notified, which is
done by a particular client’s prioritizer function (refer to the previous section, “Prioritiza-
tion”). The scheduler has the following calling sequence:
Notify_func
notify_set_scheduler_func(scheduler_func)
Notify_func scheduler_func;
The notify_set_scheduler_func() function allows you to arrange the order in
which clients are called. The argument scheduler_func is the function to call to do the
scheduling of clients. The previous function that would have been called is returned. This
returned function will, almost always, be important to store and call later because it is most
likely the default scheduler.
Replacement of the default scheduler is most often done by a client that needs to make sure
that other clients don’t take too much time servicing all of their notifications. For example, if
doing “real-time” cursor tracking in a user process, the tracking client wants to schedule
itself ahead of other clients, whenever there is input pending on the mouse.
The calling sequence of a scheduler function is:
Notify_value
scheduler_func(n, clients)
int n;
Notify_client *clients;
506 XView Programming Manual
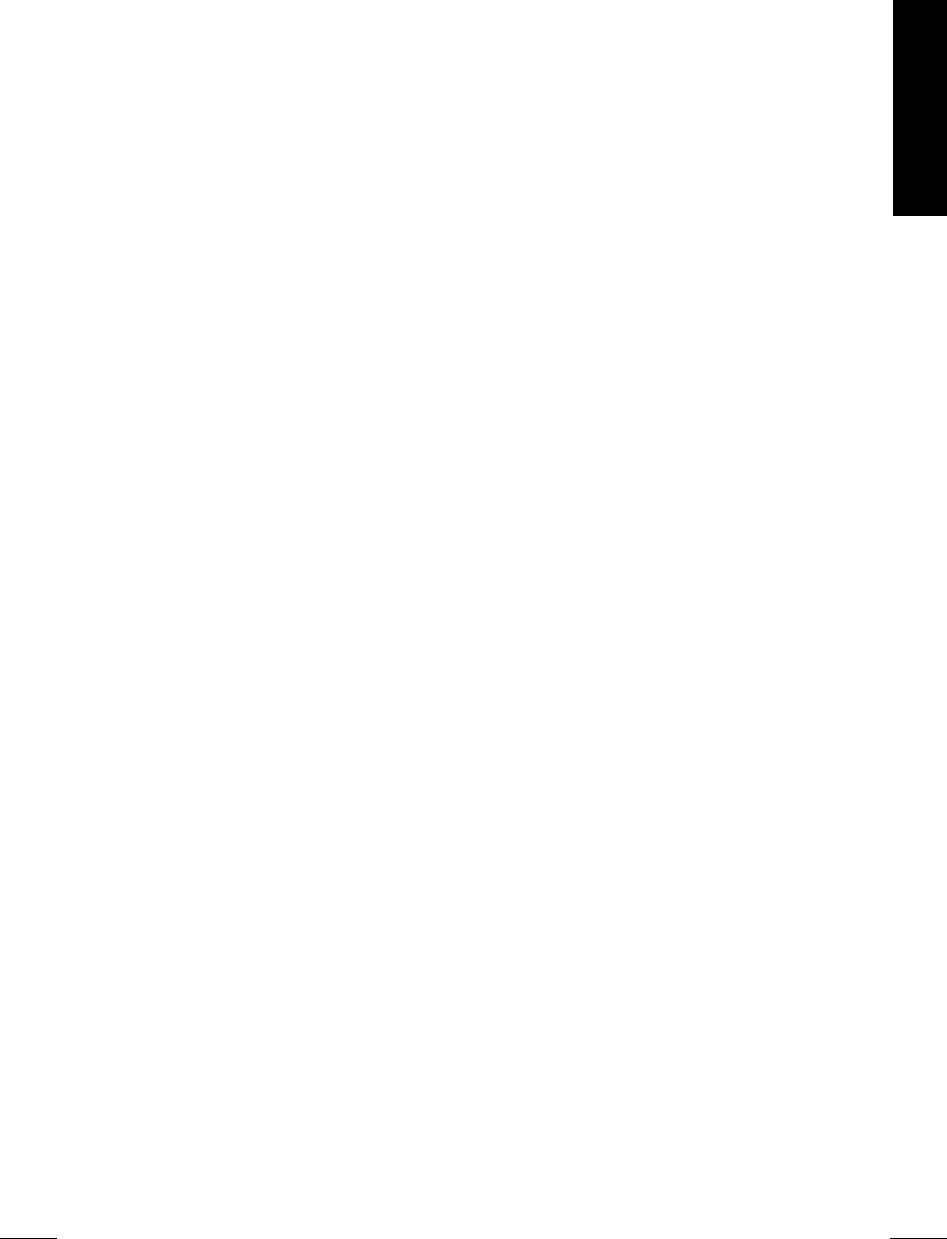
The argument n is passed into scheduler_func(). This is a list of clients, all of which
are slated to receive some notification this time around. The scheduler scans clients and
makes calls to notify_client() (refer to the next section, “Dispatching Clients”).
Clients so notified should have their slots in clients set to NOTIFY_CLIENT_NULL. The
return value from scheduler_func() is one of the following:
• NOTIFY_DONE – All of the clients had a chance to send notifications. The implies that no
further clients should be scheduled this time around the notification loop. Unsent notifi-
cations are preserved for consideration the next time around the notification loop.
• NOTIFY_IGNORED – One or more clients were scheduled; that is, some clients
may have been scheduled, but not all. This implies that another scheduler should try to
schedule any clients in clients that are not NOTIFY_CLIENT_NULL.
20.12.2.1 Dispatching clients
The following routine is called from scheduler routines to cause all pending notifications for
client to be sent:
Notify_error
notify_client(client)
Notify_client client;
The return value is NOTIFY_OK, NOTIFY_NO_CONDITION, or NOTIFY_UNKNOWN_CLIENT.
The return value NOTIFY_OK indicates the client was notified. NOTIFY_NO_CONDITION indi-
cates no conditions for client. This might mean notify_client() was already called
with this client handle. NOTIFY_UNKNOWN_CLIENT indicates an unknown client.
20.12.2.2 Getting the scheduler
The following routine returns the function that will be called to do client scheduling:
Notify_func
notify_get_scheduler_func()
This function is always defined to be the default scheduler.
20.13 Error Codes
This section describes the basic error handling scheme used by the Notifier and lists the
meaning of each of the possible error codes. Every call to the Notifier returns a value that
indicates success or failure. On an error condition, notify_errno describes the failure.
notify_errno is set by the Notifier as errno is set by UNIX system calls. (i.e.,
notify_errno is set only when an error is detected during a call to the Notifier. It is not
reset to NOTIFY_OK on a successful call to the Notifier.)
enum notify_error {
... /* Listed below */
};
Notifier
The Notifier 507

typedef enum notify_error Notify_error;
extern Notify_error notify_errno;
Table 20-1 contains a complete list of error codes.
Table 20-1. Notifier Error Codes
Error Code Description
NOTIFY_OK The call was completed successfully.
NOTIFY_UNKNOWN_CLIENT The client argument is not known by the Notifier. A
notify_set_*_func call needs to be made in order for
the Notifier to recognize it.
NOTIFY_NO_CONDITION A call was made to access the state of a condition, but the
condition was not set with the Notifier for the client in
question. This situation can occur when a
notify_get_*_func() type call is made before the
equivalent
notify_set_*_func()
. Also, the Notifier
automatically clears some conditions after they have
occurred, e.g., when an interval timer expires.
NOTIFY_BAD_ITIMER The which argument to an interval timer routine was not
valid.
NOTIFY_BAD_SIGNAL The signal argument to a signal routine was out of range.
NOTIFY_NOT_STARTED A call to notify_stop() was made, but the Notifier was
never started.
NOTIFY_DESTROY_VETOED A client refused to be destroyed during a call to
notify_die() or notify_post_destroy() when sta-
tus
was DESTROY_CHECKING.
NOTIFY_INTERNAL_ERROR Some internal inconsistency in the Notifier itself has been
detected.
NOTIFY_SRCH The pid argument to a child process control routine was
not valid.
NOTIFY_BADF The fd argument to an input or output routine was not
valid.
NOTIFY_NOMEM The Notifier dynamically allocates memory from the heap.
This error code is generated if the allocator could not get
any more memory.
NOTIFY_INVAL Some argument to a call to the Notifier contained an inva-
lid argument.
NOTIFY_FUNC_LIMIT An attempt to set an interposer function has encountered
the limit of the number of interposers allowed for a single
condition.
The routine notify_perror() acts like library call perror (3).
notify_perror(str)
char *str;
508 XView Programming Manual
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.