
25
XView Internals
This chapter discusses the internal mechanisms that XView uses to implement the existing
object classes. The information in this chapter should give you the ability to build your own
objects that are “extensible” or are extensions of other existing classes. By writing exten-
sions to XView classes, you can modify their appearance or functionality. By creating new
classes, you can create objects that go beyond what the existing XView library provides.
However, you should be forewarned that building XView extensions is not intended to be a
solution to every problem. You are strongly encouraged to implement the type of object or
enhancements you need using the facilities provided by XView and the existing XView
objects. Furthermore, this chapter should be used as an introductory resource. It does not
contain enough information to fully explain how all the internal XView objects work, nor
does it give you the ability to build an entire library of user interface objects.
If OPEN LOOK compliance is important in your applications, you should be sure that you
fully understand the OPEN LOOK specifications before attempting to build new objects or
modify existing ones. Because the XView internals do not enforce user interface policy, you
could build non-OPEN LOOK-compliant user interface code. However, the existing XView
objects were written to conform to OPEN LOOK as much as possible. While you are strongly
encouraged to examine the XView source as a model, this chapter only acts as a guide to that
model and may not address all issues involved with all XView packages.
In Chapter 2, The XView Programmer’s Model, we introduced and discussed the hierarchy of
XView objects and the use of the basic functions intrinsic to XView: xv_create(),
xv_set(), xv_get(), xv_find(), and xv_destroy(). We will now take a closer
look at how that model is utilized by XView internals.
We are going to start with a general discussion of the concepts that XView uses as a frame-
work. The methods described are intrinsic to all XView packages. After that, we examine
how attributes and their associated “values” interact with XView and its packages. Once
these issues have been addressed, we illustrate how to write your own XView packages and
extensions using these concepts.
The Logo package is a simple package that displays an X logo in the middle of a window.
The Bitmap package is similar, but it allows the programmer to display an arbitrary bitmap in
a window. The Image package is used to demonstrate how to write an extension to an exist-
ing XView package. In this case, it is an extension from the server image package found in
XView Internals
XView Internals 579
Chapter 15, Nonvisual Objects. Finally, the Wizzy package shows how to write a panel item
extension. Note that the PANEL package provides several special attributes that should only
be used by panel item extension writers.
25.1 Methods
The intrinsics layer of XView is the mechanism that defines and controls the class inheri-
tance model; in other words, it establishes parent/child relationships among XView classes.
The XView intrinsics handle the creation, modification, query, and destruction of actual
instances of object classes. Each object class contains a method (as it is called in object-ori-
ented programming terminology) to respond to any XView-intrinsic request. A method is a
function that is written and compiled into the executable program to perform the designated
task.
For example, when the programmer calls xv_create() to create an instance of a particular
class, the XView intrinsics invokes the initialize method from that class. For xv_set(), it
invokes the set method, and so on. The XView intrinsics define the methods while the actual
classes provide the functions that perform them.
Each class is represented programmatically by declaring a data structure consisting of point-
ers to functions that correspond to each of the methods. When the programmer calls
xv_get(), the intrinsics de-reference the pointer to the get method and call it as a function.
25.1.0.1 Static subclassing
You recall from Chapter 2, The XView Programmer’s Model, that XView classes are sub-
classed from one another starting from the generic class. This class contains basic informa-
tion about the object such as its x,y position, its geometry specification, its reference count
(how many other objects refer to it in some way), what server and display the object is asso-
ciated with and so on. Most classes share this information and are therefore subclassed from
the generic class.
From the generic class, new subclasses are created to describe more specifics about that par-
ticular class’s appearance, functionality, or other attributes. Subclassing causes each new
class to inherit everything from its parent class, so the child class does not need to be rede-
fined or reinitialized. New subclasses define their own methods so that the intrinsics can uti-
lize the parts of new classes that differentiate them from their parent classes. When the pro-
grammer calls xv_create() to create an instance from a particular class, the intrinsics call
the initialize method for each subclass in the hierarchy in succession.
To implement this using the C language, each class is physically defined by a data structure
that contains pointers to functions previously written for that class. All this information must
be compiled into the XView library (or at least linked with the rest of the object modules at
580 XView Programming Manual
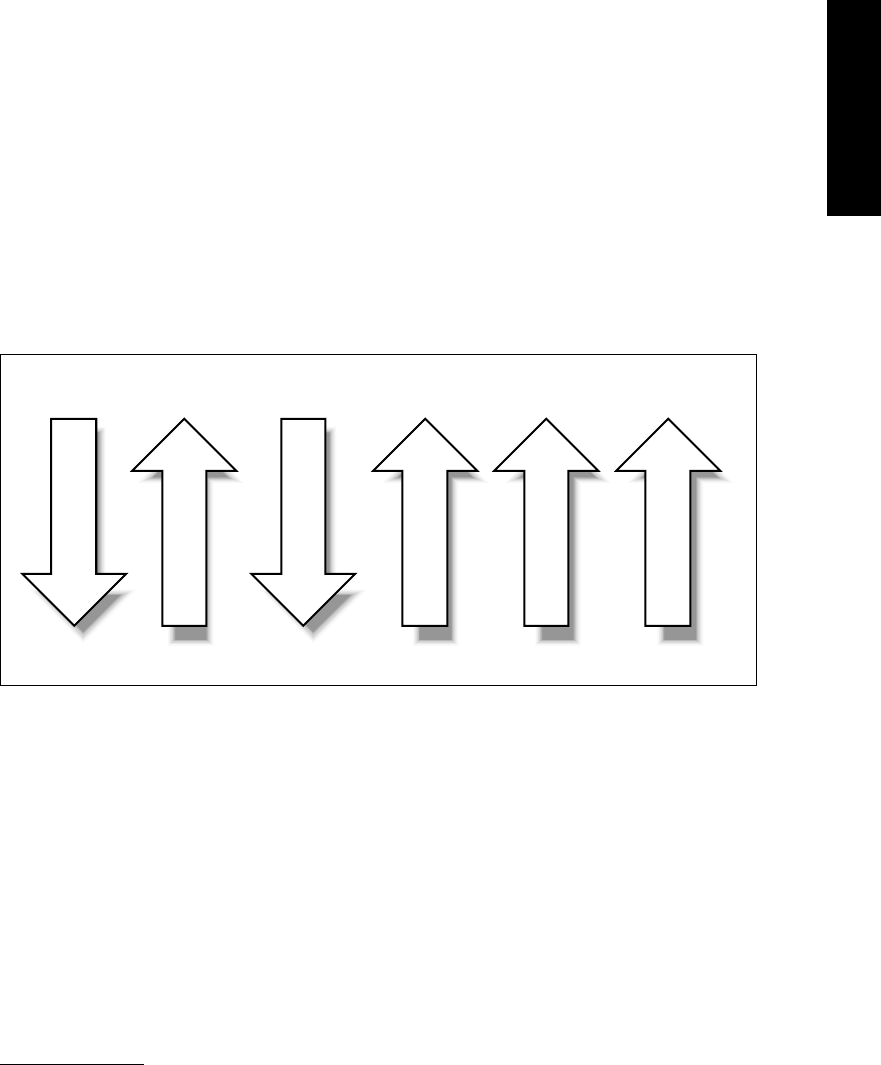
compile time). Because the functions and data structures are pre-written, the subclasses are
static—they cannot be changed during the execution of the program.* In sum, XView uses
static subclassing.
25.1.1 Order of Methods
As discussed in Chapter 2, The XView Programmer’s Model, whenever an object is created,
the XView intrinsics initialize each class from top to bottom. Thus, the generic class is first
instantiated by calling its initialize method, followed by the next subclass, all the way down
until the class of the type requested is instantiated. When completed, an instance of the class
has been created with all the default properties of the classes set.
However, the initialization sequence does not stop there. As Figure 25-1 shows, the initiali-
zation sequence consists of three phases.
set
Init
xv_create()
(end of init)
Get Destroy Find
Figure 25-1. Calling order for init, set, get, destroy, and find
After the initialization methods are called for each of the packages, XView calls the set
methods to handle any attribute-value pairs specified in the programmer’s call to xv_
create(). This is done even if there were no attributes specified. This phase is executed
in the reverse order of the initialize method, moving from the bottom up.
The final phase of the initialization sequence calls the set function again, but in the original
order (e.g., from the top down). Here, the set method is called with only one attribute,
XV_END_CREATE. This is the only time that the set method is called from the top down. This
final phase indicates that the creation phase is over and that the class should resolve any un-
finished work. Prior to this point, be careful not to use xv_set() on the object being creat-
ed. During this final phase, you may use xv_set() on the object being created. Each
phase of this operation is discussed in more detail in later sections.
*Limitations of the C language prevent the ability to do dynamic subclassing.
XView Internals
XView Internals 581
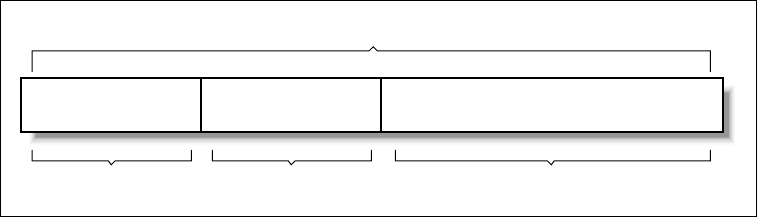
25.2 Internal Attribute-value Lists
Each of the methods introduced above (with the exception of the destroy method) must deal
with attributes and attribute-value lists. Before we begin to discuss the details of how the
methods work, the fundamentals of attributes must be understood. This includes the nature
of attributes, how their internal values are constructed, the nature of the values associated
with attributes, package IDs, and so on.
25.2.1 Attribute Values
The semantics of the term value can be confusing. There are two values that are used when
referring to attributes. The type most commonly used is the value associated with the attri-
bute. That is, “brown” is the value associated with the attribute PANEL_ITEM_COLOR. How-
ever, PANEL_ITEM_COLOR is declared as an enumerated type that has a “value” just as C vari-
ables have values.
The value of an attribute variable contains information about the type of attribute it is, the
package it belongs to, how many “value” parameters are associated with it, and the types of
those “values.” This is all accomplished by setting particular bits within segments of the
32-bit data type, Attr_attribute.
The breakdown of the bits in the attribute is shown in Figure 25-2.
(package ID)
Attr_attribute
(Ordinal Value) (Type of Attribute)
0 1 0 1 0 0 0 0 0 1 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0
Figure 25-2. The bits in an attribute
All this is done via macros defined in <xview/attrs.h> along with a complete listing of all the
different types of attributes that may be used.
Let’s examine a real attribute,
XV_RECT. This attribute can be used to set or get the bounding
box of an XView object (e.g., x and y, width and height). The attribute is declared in
<xview/generic.h> as:
XV_RECT = XV_ATTR(ATTR_RECT_PTR, 74)
582 XView Programming Manual

The value of XV_RECT expands to a long value set by the macros XV_ATTR and
ATTR_RECT_PTR. These are macros whose values are used as masks that can identify the at-
tribute later. The value of 74 is a unique number with respect to the other attributes in the
package.
Thus, when the internals of an XView procedure want to determine the value of XV_RECT,
they know that the “value” that the programmer specified must be a Rect *. Furthermore,
it is known that xv_get() should return the same type. This loose type-checking is by no
means enforced. It is used for various reasons, including the ability of the programmer to
identify the type of value a particular attribute should take.
The attribute’s “type” is also used to determine how many programmer-supplied “values” are
associated with it. For example, the attribute PANEL_CHOICE_STRINGS indicates that the
value associated with it is a NULL-terminated list of char pointers. We can check this by
looking at the value of the attribute in <xview/panel.h>:
PANEL_CHOICE_STRINGS =
PANEL_ATTR(ATTR_LIST_INLINE(ATTR_NULL, ATTR_STRING), 22)
Here, the use of
PANEL_ATTR shows that the attribute is part of the PANEL package. The
macro ATTR_LIST_INLINE shows that the value associated with the attribute is a NULL-
terminated list. The macro ATTR_STRING shows that it is a list of strings or char pointers.
The list of possible types of attributes is in <xview/attr.h>.
25.2.2 Creating Attribute Lists
When functions that take attribute-value lists are called, XView internally converts the entire
list into an array of Attr_attribute values. The size of the array cannot exceed
ATTR_STANDARD_SIZE. This array of attributes and values is assigned the type,
Attr_avlist.
The array is created and the actual variable argument list of attributes and values is stored in
the array using the function attr_create_list().
Any function whose interface is called with a variable argument list has a corresponding (in-
ternal) function that takes the Attr_avlist parameter rather than the variable argument
list. This is due to the fact that variable argument lists cannot be passed to subsequent func-
tions reliably. XView overcomes this problem by converting the entire list of attributes and
values into the Attr_attribute array and passing it around to functions.
25.2.2.1 Attribute lists within attribute lists
Sometimes, the same attribute-value list is used in multiple calls to xv_create() or
xv_set(). Specifying the same list all the time is wasteful since it requires the same pro-
cessing for each call. In order to optimize and simplify this problem, XView provides an in-
terface for both the XView programmer and the package implementor by introducing the at-
tribute, ATTR_LIST.
XView Internals
XView Internals 583
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.