A handle to the new panel object is returned and stored in the variable panel. This handle
is not a pointer and does not contain any useful information about the object itself.
The next section goes into detail about the use of xv_set() and xv_get(). Chapter 3,
Creating XView Applications, discusses the use of xv_init(), xv_destroy(), and
xv_find().
2.2.2 Changing Object Attributes
The programmer uses the handle returned from the xv_create() function as a parameter
to the functions xv_get() and xv_set() to get and set attributes of the object.
panel = xv_create(...)
xv_set(panel, PANEL_LAYOUT, PANEL_HORIZONTAL, NULL);
Here, the handle to the panel (panel) is used to change a PANEL package attribute,
PANEL_LAYOUT, whose value is set to PANEL_HORIZONTAL. The attribute and value form an
attribute-value pair. The functions xv_create(), xv_destroy(), xv_find(),
xv_set(), and, to some extent, xv_get() use attribute-value pairs. The functions can
have any number of pairs associated with the function call. These variable argument lists are
always terminated by a
NULL pointer as the last argument in the list. Note that NULL, not the
constant 0 (zero), should be used as the terminating argument.
The effect of this function call is to change the layout of the panel from the previous value,
whatever it might be, to horizontal.
2.2.3 Types of Attributes
Attributes can be divided into three categories. Those that apply to all XView objects are
termed generic attributes. Attributes that are supported by many, but not all objects, are
termed common attributes. Attributes that are associated with a particular package or class of
objects are called specific attributes.
XView uses naming conventions to simplify the identification of the task of an attribute.
Those attributes that apply to a specific package have their name prefixed by the package
name. The attributes have prefixes that indicate the type of object they apply to, i.e., CAN-
VAS_
*, CURSOR_*, FRAME_*, ICON_*, MENU_*, PANEL_*, SCROLLBAR_*, TEXTSW_*,
TTY_*, etc.
Common and generic attributes apply to several different object types and are prefixed by
XV_. For example, the generic attribute
XV_HEIGHT applies to all objects since all objects
must have a height. In contrast, attributes that apply only to windows are prefixed by WIN_.
Attributes such as WIN_HEIGHT and WIN_WIDTH apply to all windows regardless of whether
they happen to be panels or canvases.
The value part of an attribute-value pair can differ from attribute to attribute. The reason for
this is that the attribute may describe a wide range of values. If the attribute describes the
height or width of an object, the value associated with the attribute will be an integer.
22 XView Programming Manual

However, sometimes the attribute requires a variable-length list of values—this too must be
NULL-terminated.
Look at the following code fragment that specifies an attribute-value list at the creation of a
panel item:
Panel_item panel_item;
panel_item = xv_create(panel, PANEL_CHOICE_STACK,
XV_WIDTH, 50,
XV_HEIGHT, 25,
PANEL_LABEL_X, 100,
PANEL_LABEL_Y, 100,
PANEL_LABEL_STRING, "Open File"
PANEL_CHOICE_STRINGS, "Append to file",
"Overwrite contents",
NULL,
NULL);
All the attributes except PANEL_CHOICE_STRINGS take a single value. The
PANEL_CHOICE_STRINGS attribute takes a list of strings, and that list is NULL-terminated.
The last
NULL terminates the list of attribute-value pairs passed to the xv_create() func-
tion.
Don’t worry for now what each of these attributes does. Simply notice the mixture of generic
attributes (XV_WIDTH and XV_HEIGHT) and class-specific attributes (all the PANEL_* attri-
butes). Because all packages are subclasses of the XV_OBJECT package, the XV_* attributes
can be used with all xv_create() calls.
2.3 Internal Attribute-Value Lists
For a discussion of the way that XView handles attribute-value lists internally, see Chapter
25, XView Internals. The subject is important for those who wish to write XView extensions
or utilize the advanced features of the error package, but programmers interested in general
XView programming usage can skip that chapter.
2.4 Types of Objects
The following section describes on a conceptual level the different types of objects that
XView offers. In many cases, figures taken from the OPEN LOOK GUI Specification Guide
are used to show the appearance of the object. Details about the objects themselves, how to
create them, their properties, their default values, and so forth are discussed in later chapters
that are specific to those object packages. A list of the objects that can be created include:
• Generic Objects
• Windows
• Frames
• Openwins
XView Programmer’s
Model
The XView Programmer’s Model 23
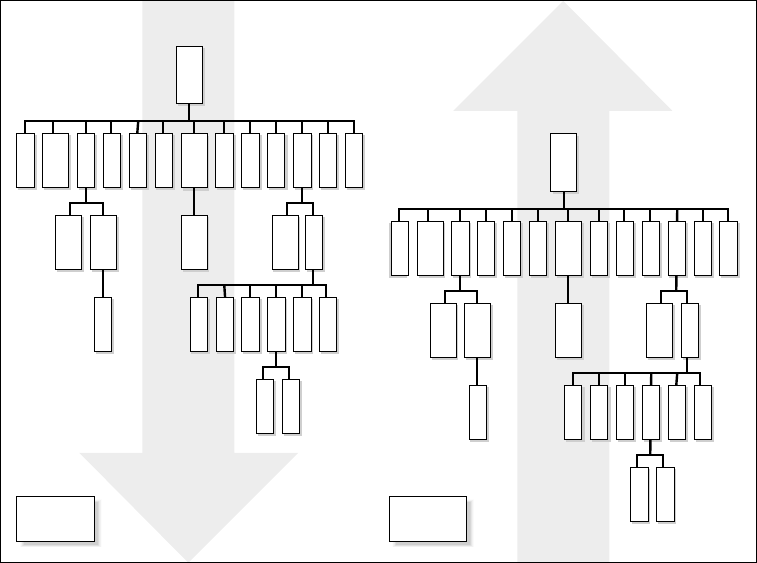
• Canvases
• Text Windows
• Menus
• Scrollbars
2.4.1 Generic Objects
The Generic Object is the root object of the class hierarchy. One never creates an instance of
a Generic Object because, by itself, it has no function. Figure 2-2 shows the path taken when
an object is created.
Server
Cursor
Generic
Object
Screen
(Drawable)
Fullscreen
Font
Menu
Selection
Item
(Selection)
Drop Site
Notice
Frame
Openwin
Tty
Icon
Scrollbar
DRAGDROP
Window
Server
Image
Selection
Owner
Selection
Requestor
Canvas
Textsw
Cms
Generic
Panel Item
Your
Panel Item
Panel
Server
Cursor
Generic
Object
Screen
(Drawable)
Fullscreen
Font
Menu
Selection
Item
(Selection)
Drop Site
Notice
Frame
Openwin
Tty
Icon
Scrollbar
DRAGDROP
Window
Server
Image
Selection
Owner
Selection
Requestor
Canvas
Textsw
Cms
Generic
Panel Item
Your
Panel Item
Panel
Attribute
Setting
Object
Creation
Figure 2-2. Object creation is top down; attribute setting is bottom up
First, the Generic Object is created; then the subclass of that object is created all the way
down until the object class of the type of object desired is created. At that point, a complete
instance of the object has been created with all the default properties of the classes set. If
there were any attribute-value pairs specified in the xv_create() call, those attributes are
24 XView Programming Manual

set in reverse order—the attributes specific to the class of the instance of the object are set
first, followed by its parent’s class attributes and so on, until the generic attributes are set.
Consider the code below, which creates a panel:
extern Xv_Font font;
Panel panel;
panel = xv_create(frame, PANEL,
XV_Y, 5,
WIN_HEIGHT, 50,
PANEL_FONT, font,
NULL);
When the panel is created, the first thing created is a generic object. Next a window instance
is created, followed by a panel object. Each is created with the default properties of the
object specific to each class.
Here, the reverse traversal takes place, and the attributes specified in the xv_create() call
for each class are set to override the default properties inherited from the class. First, the
panel package attributes are set. The panel’s default font is controlled by the attribute
PANEL_FONT; its assigned value,
font
, must be previously initialized. Then the window
package attributes are set. The panel’s window width is controlled by the attribute
WIN_WIDTH
which is not explicitly set, so its assigned value defaults to
WIN_EXTEND_TO_EDGE. This value indicates that the width of the window should always be
the width of its parent. The height of the window, however, is specified. So the window
package sets the height to be 50 pixels.
Finally, the generic attributes are set. The panel’s x and y location, indicating where it should
be placed within its parent, is controlled by setting the XV_X and XV_Y attributes. The
example sets the y position only; the x position is not set because the window package is told
to extend the width of the panel to the edges of its parent. The parent in this case is the
object frame (which is presumed to be from the FRAME package).
2.4.2 Window Objects
Many XView objects contain X windows in order to display themselves and receive events.
Examples include frames, tty windows, scrollbars, and icons.
The XView window class, like the Generic Object class, is a hidden class: a window object
is never explicitly created. Rather, an object that is a subclass of the window class is created.
This includes most visual objects with the exception of panel items.
Nonvisual objects are so named because they do not contain, or are not a subclass of, win-
dows. Fonts, for example, are displayed in windows, or in a memory image or somewhere
that contains a bitmap, but fonts do not contain or require windows to be used.
Some attributes of windows include depth (
XV_DEPTH), the border width around their perim-
eter (WIN_BORDER_WIDTH) as well as foreground and background colors.
XView Programmer’s
Model
The XView Programmer’s Model 25
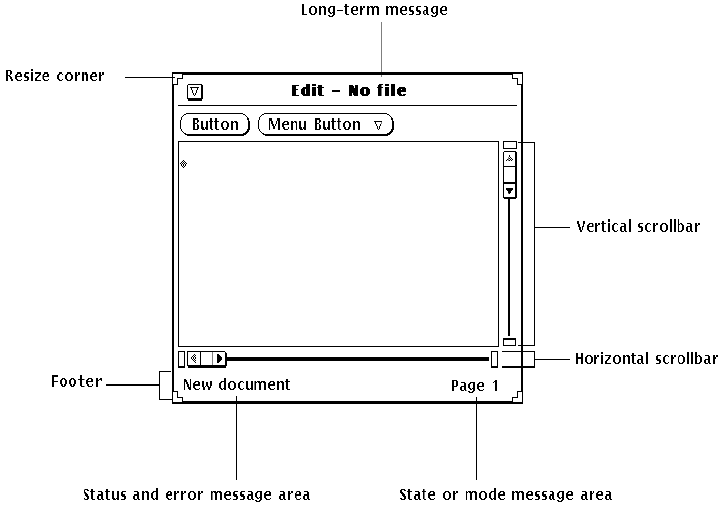
2.4.3 Frames and Subframes
There are two kinds of frames:
• Base Frames
• Pop-up Frames
With one exception, all frames are free-floating windows that contain subwindows that are
bound by the frame and tiled (they do not overlap one another). Base frames reside on the
root window and are not constrained by any other window, though all frames can overlap one
another. The base frame is also known as the application’s frame. (More than one base
frame may be associated with an application.) Subframes are frames whose owner is a
frame; they are controlled by the base frames of the application. For example, extraneous
dialog boxes (subframes) will go away if the main application’s base frame is iconified
(closed). Figure 2-3 shows an example of a fully-featured base frame from the OPEN LOOK
GUI Specification Guide.
Figure 2-3. Fully-featured base frame (includes optional elements)
Chapter 4, Frames, goes into more detail about the elements of a frame and how to set attri-
butes and override default values. It should be noted that many features of the frame are
attributes of the window manager. Figure 2-3 assumes an OPEN LOOK-compliant window
manager; if another window manager is used, base frames might not look the same. XView
26 XView Programming Manual
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.