
On get, the arguments remain the same, the array passed in gets filled in by the PANEL_LIST
package. The return value is the number of rows that were successfully filled in.
Example D-3 shows a portion of a program which uses the new PANEL_LIST insertion
method.
Example D-3. Program that adds values to a panel list
/*
* Demonstrate the use of the PANEL_LIST_ROW_VALUES attribute
*/
#include <stdio.h>
#include <xview/xview.h>
#include <xview/font.h>
#include <xview/panel.h>
static Attr_attribute MY_KEY;
static void my_clear_proc();
static void my_load_proc();
static void my_print_proc();
typedef struct {
Frame frame;
Panel_list_item list;
Xv_font font;
} My_ui;
void
main ( argc, argv )
int argc;
char **argv;
{
Panel panel;
My_ui ui;
(void) xv_init(XV_INIT_ARGC_PTR_ARGV, &argc, argv, NULL);
MY_KEY = xv_unique_key();
ui.frame = xv_create ( XV_NULL, FRAME,
XV_LABEL, "New Load",
FRAME_SHOW_FOOTER, TRUE,
NULL );
panel = xv_create ( ui.frame, PANEL, NULL );
(void) xv_create ( panel, PANEL_BUTTON,
PANEL_LABEL_STRING, "Load",
PANEL_NOTIFY_PROC, my_load_proc,
XV_KEY_DATA, MY_KEY, &ui,
NULL );
(void) xv_create ( panel, PANEL_BUTTON,
PANEL_LABEL_STRING, "Clear",
692 Version 3.2 and the File Chooser
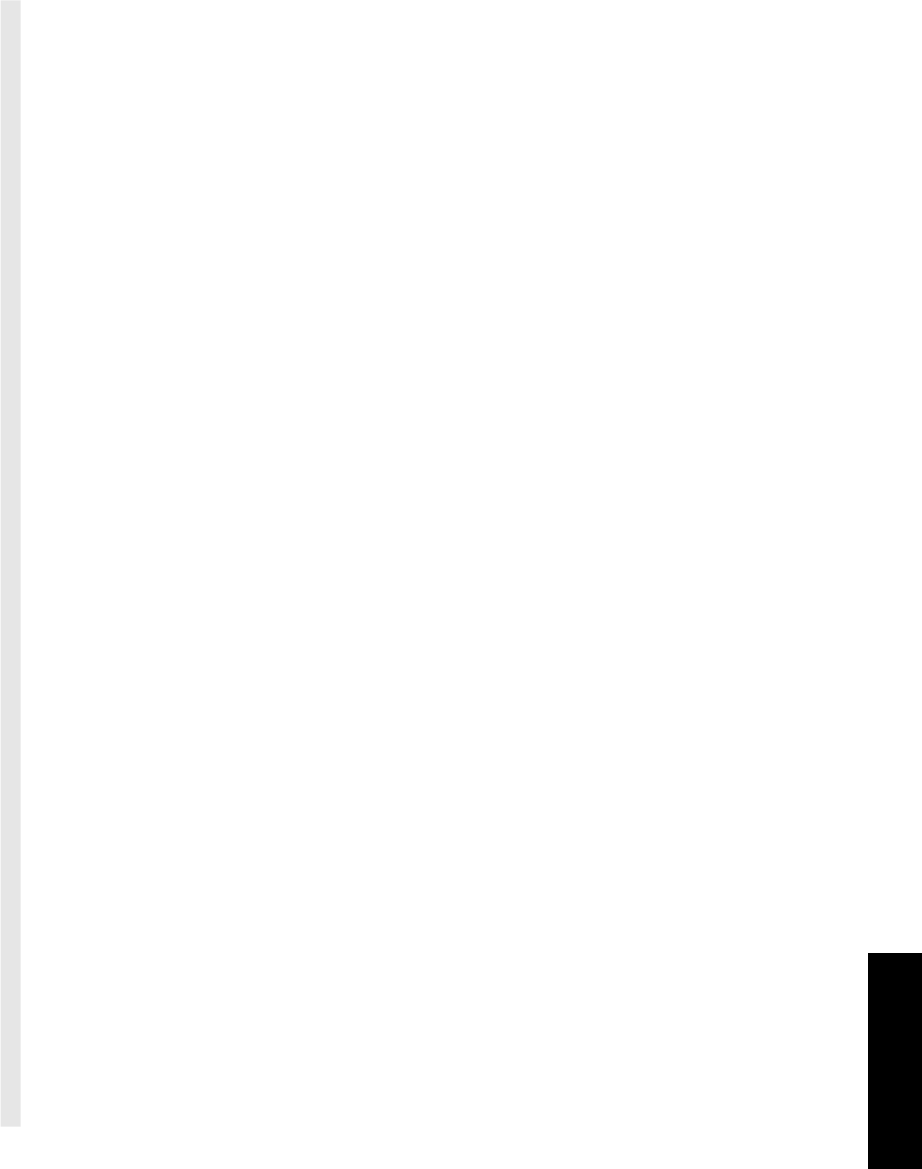
Example D-3. Program that adds values to a panel list (continued)
PANEL_NOTIFY_PROC, my_clear_proc,
XV_KEY_DATA, MY_KEY, &ui,
NULL );
(void) xv_create( panel, PANEL_BUTTON,
PANEL_LABEL_STRING, "Print Selected Row",
PANEL_NOTIFY_PROC, my_print_proc,
XV_KEY_DATA, MY_KEY, &ui,
NULL );
ui.list = xv_create( panel, PANEL_LIST,
PANEL_LIST_WIDTH, 300,
PANEL_LIST_DISPLAY_ROWS, 8,
NULL);
ui.font = xv_create(XV_NULL, FONT,
FONT_FAMILY, FONT_FAMILY_DEFAULT,
FONT_STYLE, FONT_STYLE_BOLD,
NULL);
window_fit ( panel );
window_fit ( ui.frame );
xv_main_loop ( ui.frame );
}
static void
my_clear_proc( item, event )
Panel_item item;
Event *event;
{
My_ui *ui = (My_ui *)xv_get(item, XV_KEY_DATA, MY_KEY);
int rows = (int)xv_get(ui->list, PANEL_LIST_NROWS);
if ( rows > 0 )
xv_set(ui->list,
PANEL_LIST_DELETE_ROWS, 0, rows,
NULL);
xv_set(ui->frame, FRAME_LEFT_FOOTER, "", NULL);
}
static void
my_load_proc( item, event )
Panel_item item;
Event *event;
{
My_ui *ui = (My_ui *)xv_get(item, XV_KEY_DATA, MY_KEY);
int ii;
Panel_list_row_values vals[1000];
char buf[64];
xv_set(ui->frame, FRAME_BUSY, TRUE, NULL);
for(ii=0; ii<1000; ++ii) {
vals[ii].string = "Testing PANEL_LIST_ROW_VALUES";
Version 3.2 and
the File Chooser
Version 3.2 and the File Chooser 693

Example D-3. Program that adds values to a panel list (continued)
vals[ii].font = ui->font;
vals[ii].glyph = XV_NULL;
vals[ii].mask_glyph = XV_NULL;
vals[ii].client_data = ui->font;
vals[ii].selected = FALSE;
vals[ii].inactive = FALSE;
}
xv_set(ui->list,
PANEL_LIST_ROW_VALUES, 0, &vals, 1000,
NULL);
(void) sprintf(buf, "%d rows in list",
(int) xv_get(ui->list, PANEL_LIST_NROWS)
);
xv_set(ui->frame,
FRAME_LEFT_FOOTER, buf,
FRAME_BUSY, FALSE,
NULL);
}
static void
my_print_proc( item, event )
Panel_item item;
Event *event;
{
My_ui *ui = (My_ui *)xv_get(item, XV_KEY_DATA, MY_KEY);
Panel_list_row_values vals;
int row = (int) xv_get(ui->list, PANEL_LIST_FIRST_SELECTED);
int count;
count = (int) xv_get(ui->list, PANEL_LIST_ROW_VALUES, row, &vals, 1);
if ( count != 1 ) {
window_bell( ui->frame );
xv_set(ui->frame,
FRAME_LEFT_FOOTER, "Unable to get row",
FRAME_BUSY, FALSE,
NULL);
return;
}
printf( "Row Number %d:0, row );
printf( " String: %s0, vals.string );
printf( " Selected: %d0, vals.selected );
printf( " Inactive: %d0, vals.inactive );
}
694 Version 3.2 and the File Chooser

D.8.1.2 Other panel list changes
This section describes the additional changes for the panel list package.
The attribute PANEL_LIST_INACTIVE "Grays out” a row in a PANEL_LIST. Note that a row
that is inactive cannot be selected. Also, mouseless model navigation is not effected by the
inactive state of the individual rows.
The attribute PANEL_LIST_DELETE_INACTIVE_ROWS deletes all inactive rows from the list.
This is similar to PANEL_LIST_DELETE_SELECTED_ROWS attribute.
The PANEL_LIST_DO_DBL_CLICK attribute tells PANEL_LIST to interpret two select events
that occur within the timout value as a double-click instead of as a second select or a deselect
(depending on the current mode of the list). The timeout value is specified with Open
Windows.MulticlickTimeout If true, the PANEL_LIST will deliver a new op called
PANEL_LIST_OP_DBL_CLICK instead of the normal PANEL_LIST_OP_SELECT or
PANEL_LIST_OP_DESELECT.
The attributes PANEL_LIST_MASK_GLYPH and PANEL_LIST_MASK_GLYPHS tell the
PANEL_LIST
to use the given Server_image as a clip mask for the corresponding
PANEL_LIST_GLYPH. The Server_image supplied must be of depth 1. PANEL_
LIST_MASK_GLYPHS is like like PANEL_LIST_MASK_GLYPH, but takes a NULL terminated
list of glyphs rather than a row and a single handle.
The attributes PANEL_LIST_EXTENSION_DATA and PANEL_LIST_EXTENSION_DATAS are the
same as PANEL_LIST_CLIENT_DATA, except they are reserved for package implementors.
This is used by the FILE_LIST package.
D.9 Keyboard Menu Accelerators
The keyboard menu accelerator functionality in XView provides attributes to associate an
accelerator with a menu item. Keyboard menu accelerators can be used to invoke menu com-
mands directly without having to display the menu in which the commands appear. These
accelerators provide a more efficient path to familiar menu functionality.
Accelerators are global to a frame of an application. The accelerator key strokes are
displayed on the right side of the menu item. The diamond symbol represents the meta key.
If there are qualifiers such as Control (ctrl), Shift (shift) or Alt (alt), indicated on the menu
item, those keys are to be used in conjunction with the accelerator key and possibly the Meta
key (if the diamond symbol exists on the menu item).
If a menu is pinned, the accelerators will not be displayed on the menu, as a way to conserve
screen space. Although the accelerators are not visible on the menu, they are still active and
can be used as long as the input focus is in the application frame where the menu was first
brought up.
Menu accelerators are activated if the resource OpenWindows.MenuAccelerators is
set to True (the default case for OpenWindows Version 3.2). This resource can be set using
the “Keyboard” category of the OpenWindows(Version 3.2) Workspace Properties program.
Setting “Keyboard Menu Equivalents” to “Application + Window” or “Application Only”
Version 3.2 and
the File Chooser
Version 3.2 and the File Chooser 695
sets the OpenWindows.MenuAccelerators resource to True, while the “None” setting
will set the resource to False.
D.9.0.1 Frame package menu accelerator attributes
The attributes FRAME_MENUS, FRAME_MENU_COUNT, FRAME_MENU_ADD, and
FRAME_MENU_DELETE are used in conjunction with menu accelerators. They are used to
inform the frame object which menus will be used on the frame - this is required because the
frame has to know what menu accelerators to detect. Any menus with accelerators that are
used anywhere on the frame, i.e. on panels, textsw, canvas will not work until they are regis-
tered using these attributes.
The attribute FRAME_MENUS replaces the current menu list with the one passed on the avlist.
For get, this return a pointer to the current list of menus. The list returned should not be
modified by the application.
Menu *menu_list;
xv_set(frame1, FRAME_MENUS,
edit_menu, load_menu, NULL,
NULL);
menu_list = (Menu *)xv_get(frame1, FRAME_MENUS);
The number of menus can be obtained with FRAME_MENU_COUNT.
int menu_count;
menu_count = (int)xv_get(frame2, FRAME_MENU_COUNT);
The attribute FRAME_MENU_COUNT returns the current number of menus registered on the
frame via FRAME_MENUS, FRAME_MENU_ADD, or FRAME_MENU_DELETE.
The attribute FRAME_MENU_ADD appends to the list of accelerated menus on the frame. For
example:
xv_set(frame1,
FRAME_MENU_ADD, print_menu,
NULL);
The attribute FRAME_MENU_DELETE deletes from the list of accelerated menus on the frame.
xv_set(frame1,
FRAME_MENU_DELETE, print_menu,
NULL);
696 Version 3.2 and the File Chooser
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.