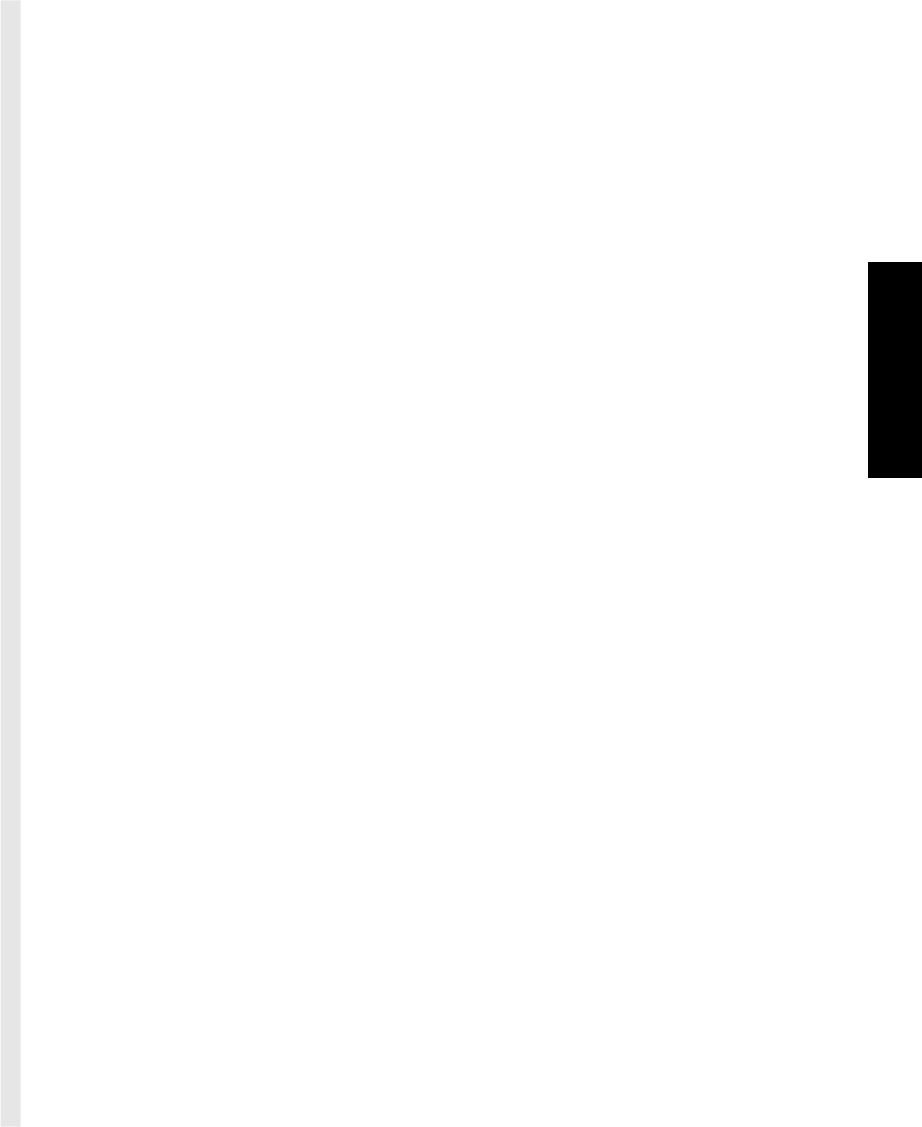
Example F-5. The fonts.c program (continued)
void
change_font(item, value, event)
Panel_item item;
Event *event;
{
static int family, style, scale;
char buf[128];
Frame frame;
char *family_name;
char *style_name;
int scale_value;
Xv_Font font;
frame = (Frame)xv_get(xv_get(item, PANEL_PARENT_PANEL), XV_OWNER);
family_name = (char *)xv_get(family_item, PANEL_CHOICE_STRING,
xv_get(family_item, PANEL_VALUE));
style_name = (char *)xv_get(style_item, PANEL_CHOICE_STRING,
xv_get(style_item, PANEL_VALUE));
scale_value = (int) xv_get(scale_item, PANEL_VALUE);
xv_set(frame, FRAME_BUSY, TRUE, NULL);
font = (Xv_Font)xv_find(frame, FONT,
FONT_FAMILY, family_name,
FONT_STYLE, style_name,
/* scale_value happens to coincide with Window_rescale_state */
FONT_SCALE, scale_value,
/*
* If run on a server that cannot rescale fonts, only font
* sizes that exist should be passed
*/
FONT_SIZES_FOR_SCALE, 12, 14, 16, 22,
NULL);
xv_set(frame, FRAME_BUSY, FALSE, NULL);
if (!font) {
if (item == family_item) {
sprintf(buf, "cannot load ’%s’", family_name);
xv_set(family_item, PANEL_VALUE, family, NULL);
} else if (item == style_item) {
sprintf(buf, "cannot load ’%s’", style_name);
xv_set(style_item, PANEL_VALUE, style, NULL);
} else {
sprintf(buf, "Not available in %s scale.",
xv_get(scale_item, PANEL_CHOICE_STRING, scale));
xv_set(scale_item, PANEL_VALUE, scale, NULL);
}
xv_set(frame, FRAME_RIGHT_FOOTER, buf, NULL);
return;
}
if (item == family_item)
family = value;
else if (item == style_item)
style = value;
else
scale = value;
cur_font = (XFontStruct *)xv_get(font, FONT_INFO);
XSetFont(dpy, gc, cur_font->fid);
Example Programs
Example Programs 733

Example F-5. The fonts.c program (continued)
sprintf(buf, "Current font: %s", xv_get(font, FONT_NAME));
xv_set(frame, FRAME_LEFT_FOOTER, buf, NULL);
}
change_font_by_name(item, event)
Panel_item item;
Event *event;
{
char buf[128];
char *name = (char *)xv_get(item, PANEL_VALUE);
Frame frame = (Frame)xv_get(xv_get(item, XV_OWNER), XV_OWNER);
Xv_Font font;
xv_set(frame, FRAME_BUSY, TRUE, NULL);
font = (Xv_Font)font = (Xv_Font)xv_find(frame, FONT,
FONT_NAME, name,
NULL);
xv_set(frame, FRAME_BUSY, FALSE, NULL);
if (!font) {
sprintf(buf, "cannot load ’%s’", name);
xv_set(frame, FRAME_RIGHT_FOOTER, buf, NULL);
return PANEL_NONE;
}
cur_font = (XFontStruct *)xv_get(font, FONT_INFO);
XSetFont(dpy, gc, cur_font->fid);
sprintf(buf, "Current font: %s", xv_get(font, FONT_NAME));
xv_set(frame, FRAME_LEFT_FOOTER, buf, NULL);
return PANEL_NONE;
}
F.6 x_draw.c
This program uses several Xlib drawing functions to draw various types of geometric objects.
We integrate the XView color model (see Chapter 21, Color) to render each object in a dif-
ferent color.
Example F-6. The x_draw.c program
/*
* x_draw.c -- demonstrates the use of Xlib drawing functions
* inside an XView canvas. Color is used, but not required.
*/
#include <xview/xview.h>
#include <xview/canvas.h>
#include <xview/cms.h>
#include <xview/xv_xrect.h>
/* indices into color table renders specified colors. */
#define WHITE 0
#define RED 1
#define GREEN 2
734 XView Programming Manual
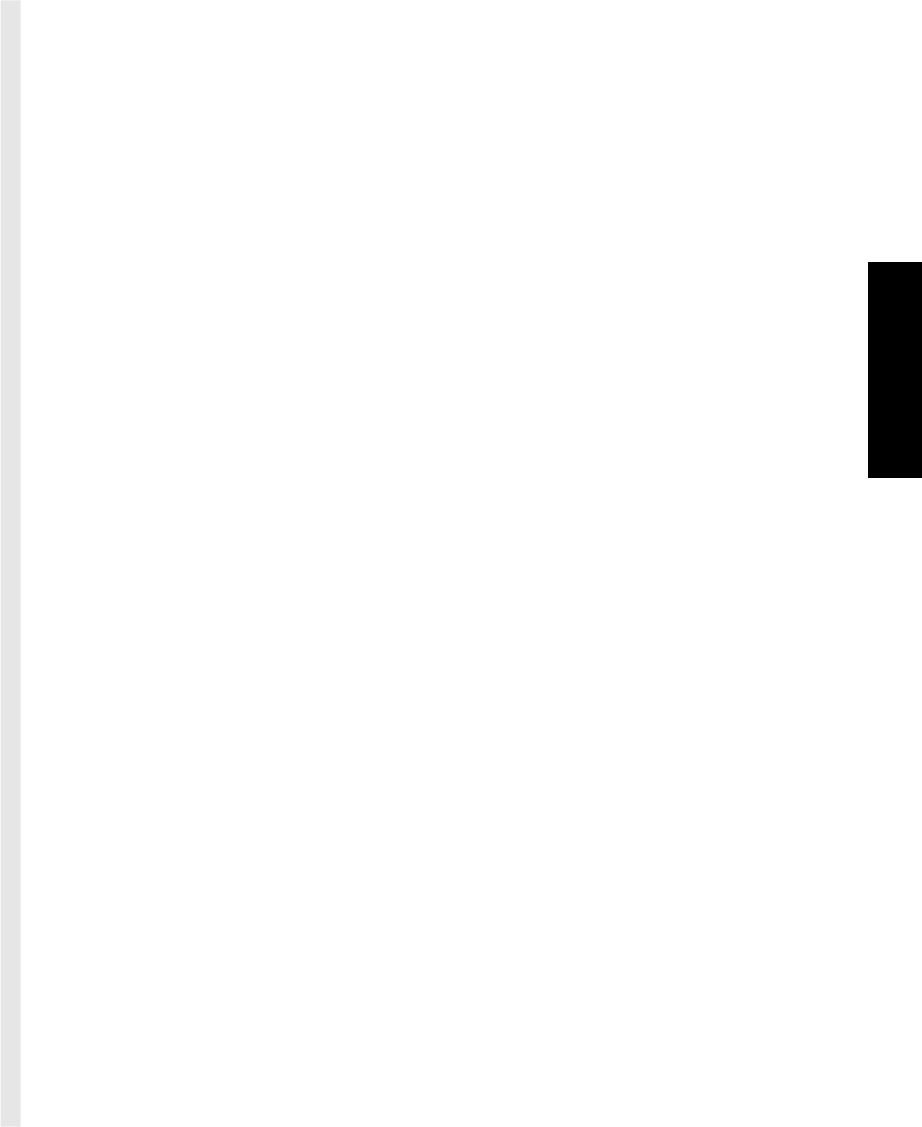
Example F-6. The x_draw.c program (continued)
#define BLUE 3
#define ORANGE 4
#define AQUA 5
#define PINK 6
#define BLACK 7
GC gc; /* GC used for Xlib drawing */
unsigned long *colors; /* the color table */
/*
* initialize cms data to support colors specified above. Assign
* data to new cms -- use either static or dynamic cms depending
* on -dynamic command line switch.
*/
main(argc, argv)
int argc;
char *argv[ ];
{
static char stipple_bits[ ] = {0xAA, 0xAA, 0x55, 0x55};
static Xv_singlecolor cms_colors[ ] = {
{ 255, 255, 255 },
{ 255, 0, 0 },
{ 0, 255, 0 },
{ 0, 0, 255 },
{ 250, 130, 80 },
{ 30, 230, 250 },
{ 230, 30, 250 },
};
Cms cms;
Frame frame;
Canvas canvas;
XFontStruct *font;
Display *display;
XGCValues gc_val;
XID xid;
void canvas_repaint();
Xv_cmsdata cms_data;
int use_dynamic = FALSE;
/* Create windows */
xv_init(XV_INIT_ARGC_PTR_ARGV, &argc, argv, NULL);
if (*++argv && !strcmp(*argv, "-dynamic"))
use_dynamic = TRUE;
frame = xv_create(NULL,FRAME,
FRAME_LABEL, "xv_canvas_x_draw",
XV_WIDTH, 400,
XV_HEIGHT, 300,
NULL);
cms = xv_create(NULL, CMS,
CMS_SIZE, 7,
CMS_TYPE, use_dynamic? XV_DYNAMIC_CMS : XV_STATIC_CMS,
CMS_COLORS, cms_colors,
NULL);
Example Programs
Example Programs 735

Example F-6. The x_draw.c program (continued)
canvas = xv_create(frame, CANVAS,
CANVAS_REPAINT_PROC, canvas_repaint,
CANVAS_X_PAINT_WINDOW, TRUE,
/* WIN_DYNAMIC_VISUAL, use_dynamic, */
XV_VISUAL_CLASS, PseudoColor,
WIN_CMS, cms,
NULL);
/* Get display and xid */
display = (Display *)xv_get(frame, XV_DISPLAY);
xid = (XID)xv_get(canvas_paint_window(canvas), XV_XID);
if (!(font = XLoadQueryFont(display, "fixed"))) {
puts("cannot load fixed font");
exit(1);
}
/* Create and initialize GC */
gc_val.font = font->fid;
gc_val.stipple =
XCreateBitmapFromData(display, xid, stipple_bits, 16, 2);
gc = XCreateGC(display, xid, GCFont | GCStipple, &gc_val);
/* get the colormap from the canvas now that
* the cms has been installed
*/
colors = (unsigned long *)xv_get(canvas, WIN_X_COLOR_INDICES);
/* Start event loop */
xv_main_loop(frame);
}
/*
* Draws onto the canvas using Xlib drawing functions.
*/
void
canvas_repaint(canvas, pw, display, xid, xrects)
Canvas canvas;
Xv_Window pw;
Display *display;
Window xid;
Xv_xrectlist *xrects;
{
static XPoint box[ ] = {
{0,0}, {100,100}, {0,-100}, {-100,100}, {0,-100}
};
static XPoint points[ ] = {
{0,0}, /* this point to be overwritten below */
{25,0}, {25,0}, {25,0}, {25,0}, {-100,25},
{25,0}, {25,0}, {25,0}, {25,0}, {-100,25},
{25,0}, {25,0}, {25,0}, {25,0}, {-100,25},
{25,0}, {25,0}, {25,0}, {25,0}, {-100,25},
{25,0}, {25,0}, {25,0}, {25,0}, {-100,25},
};
XSetForeground(display, gc, colors[RED ]);
736 XView Programming Manual

Example F-6. The x_draw.c program (continued)
XDrawString(display, xid, gc, 30, 20, "XFillRectangle", 14);
XFillRectangle(display, xid, gc, 25, 25, 100, 100);
XSetFunction(display, gc, GXinvert);
XFillRectangle(display, xid, gc, 50, 50, 50, 50);
XSetFunction(display, gc, GXcopy);
XSetForeground(display, gc, colors[BLACK]);
XDrawString(display, xid, gc, 155, 20, "XFillRect - stipple", 19);
XSetFillStyle(display, gc, FillStippled);
XFillRectangle(display, xid, gc, 150, 25, 100, 100);
XSetFillStyle(display, gc, FillSolid);
XSetForeground(display, gc, colors[BLUE]);
XDrawString(display, xid, gc, 280, 20, "XDrawPoints", 11);
points[0 ].x = 275; points[0].y = 25;
XDrawPoints(display, xid, gc, points,
sizeof(points)/sizeof(XPoint), CoordModePrevious);
XSetForeground(display, gc, colors[ORANGE]);
XDrawString(display, xid, gc, 30, 145, "XDrawLine - solid", 17);
XDrawLine(display, xid, gc, 25, 150, 125, 250);
XDrawLine(display, xid, gc, 25, 250, 125, 150);
XSetForeground(display, gc, colors[AQUA]);
XDrawString(display, xid, gc, 155, 145, "XDrawLine - dashed", 18);
XSetLineAttributes(display, gc, 5,
LineDoubleDash, CapButt, JoinMiter);
XDrawLine(display, xid, gc, 150, 150, 250, 250);
XDrawLine(display, xid, gc, 150, 250, 250, 150);
XSetLineAttributes(display, gc, 0, LineSolid, CapButt, JoinMiter);
XSetForeground(display, gc, colors[PINK]);
XDrawString(display, xid, gc, 280, 145, "XDrawLines", 10);
box[0 ].x = 275; box[0 ].y = 150;
XDrawLines(display, xid, gc, box, 5, CoordModePrevious);
XSetForeground(display, gc, colors[GREEN]);
XDrawRectangle(display, xid, gc,
5, 5, xv_get(pw, XV_WIDTH)-10, xv_get(pw, XV_HEIGHT)-10);
XDrawRectangle(display, xid, gc,
7, 7, xv_get(pw, XV_WIDTH)-14, xv_get(pw, XV_HEIGHT)-14);
}
Example Programs
Example Programs 737
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.