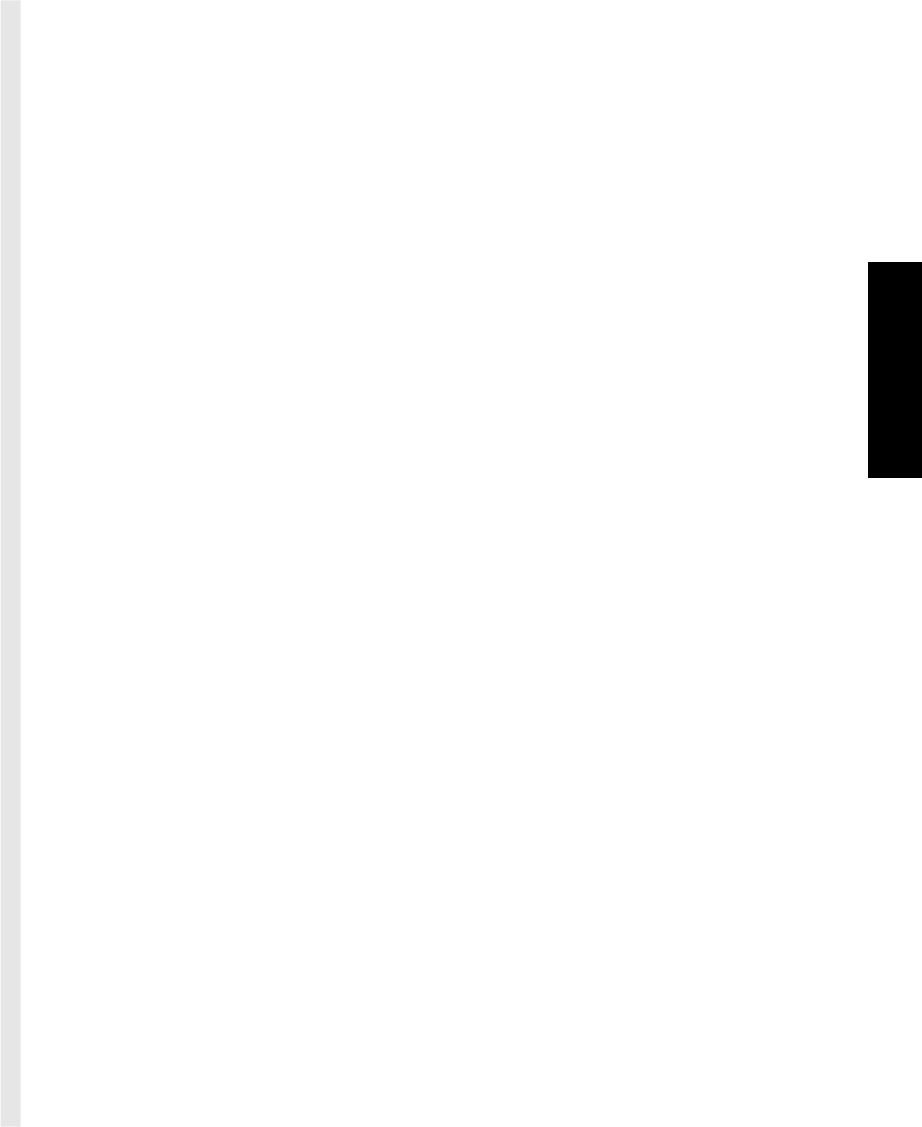
Example F-8. The Bitmap.c module (continued)
WIN_NOTIFY_IMMEDIATE_EVENT_PROC, bitmap_redraw,
NULL);
return XV_OK;
}
/* bitmap_set() -- the function called to set attributes in a bitmap
* object. This function is called when a bitmap is created after
* the init routine as well as when the programmer calls xv_set.
*/
static Xv_opaque
bitmap_set(bitmap_public, avlist)
Bitmap_public *bitmap_public;
Attr_avlist avlist;
{
Bitmap_private *bitmap_private = BITMAP_PRIVATE(bitmap_public);
Attr_attribute *attrs;
for (attrs = avlist; *attrs; attrs = attr_next(attrs))
switch ((int) attrs[0 ]) {
case BITMAP_FILE : {
int val, x, y;
Display *dpy =
(Display *)xv_get(bitmap_public, XV_DISPLAY);
Window window =
(Window)xv_get(bitmap_public, XV_XID);
Pixmap old = bitmap_private->bitmap;
if (XReadBitmapFile(dpy, window, attrs[1],
&bitmap_private->width, &bitmap_private->height,
&bitmap_private->bitmap, &x, &y) != BitmapSuccess)
{
xv_error(bitmap_public,
ERROR_STRING, "Unable to load bitmap file",
ERROR_PKG, BITMAP,
NULL);
bitmap_private->bitmap = old;
}
break;
}
case BITMAP_PIXMAP :
xv_error(bitmap_public,
ERROR_CANNOT_SET, attrs[0 ],
ERROR_PKG, BITMAP,
NULL);
break;
case XV_END_CREATE : {
/* this stuff *must* be here rather than in the "init"
* routine because the CMS is not loaded into the
* window object until the "set" routines are called.
*/
Cms cms = xv_get(bitmap_public, WIN_CMS);
XGCValues gcvalues;
Display *dpy =
(Display *)xv_get(bitmap_public, XV_DISPLAY);
gcvalues.foreground =
(unsigned long)xv_get(cms, CMS_FOREGROUND_PIXEL);
Example Programs
Example Programs 743

Example F-8. The Bitmap.c module (continued)
gcvalues.background =
(unsigned long)xv_get(cms, CMS_BACKGROUND_PIXEL);
gcvalues.graphics_exposures = False;
bitmap_private->gc =
XCreateGC(dpy, xv_get(bitmap_public, XV_XID),
GCForeground|GCBackground|GCGraphicsExposures,
&gcvalues);
}
default :
xv_check_bad_attr(BITMAP, attrs[0]);
break;
}
return XV_OK;
}
static Xv_opaque
bitmap_get(bitmap_public, status, attr, args)
Bitmap_public *bitmap_public;
int *status;
Attr_attribute attr;
Attr_avlist args;
{
Bitmap_private *bitmap_private = BITMAP_PRIVATE(bitmap_public);
switch ((int) attr) {
case BITMAP_PIXMAP :
return (Xv_opaque)bitmap_private->bitmap;
case BITMAP_FILE : /* can’t get this attribute */
default :
*status = xv_check_bad_attr(BITMAP, attr);
return (Xv_opaque)XV_OK;
}
}
/* destroy method: free the pixmap and the GC before freeing object */
static int
bitmap_destroy(bitmap_public, status)
Bitmap_public *bitmap_public;
Destroy_status status;
{
Bitmap_private *bitmap_private = BITMAP_PRIVATE(bitmap_public);
if (status == DESTROY_CLEANUP) {
if (bitmap_private->bitmap)
XFreePixmap(xv_get(bitmap_public, XV_DISPLAY),
bitmap_private->bitmap);
XFreeGC(xv_get(bitmap_public, XV_DISPLAY),
bitmap_private->gc);
free(bitmap_private);
}
return XV_OK;
}
744 XView Programming Manual

F.9 The panel_dnd.c Program
In Chapter 7, Panels, a Drop Target Items is described. This program creates and uses a
Panel Drop Target Item.
Example F-9. The panel_dnd.c program
/*
* panel_dnd.c - provides text fields, a textsw, and
* several drop targets to demonstate ways to receive
* and illustrate drag and drop operations.
*/
#include <malloc.h>
#include <xview/xview.h>
#include <xview/dragdrop.h>
#include <xview/panel.h>
#include <xview/svrimage.h>
#include <xview/textsw.h>
static unsigned short normal_bitmap[ ] = {
#include "normal.icon"
};
static unsigned short busy_bitmap[ ] = {
#include "busy.icon"
};
static unsigned short normal2_bitmap[ ] = {
#include "normal2.icon"
};
static unsigned short busy2_bitmap[ ] = {
#include "busy2.icon"
};
static char * dnd_codes[7] = {
"OK",
"Error",
"Illegal Target",
"Timeout",
"Unable to obtain selection",
"Dropped on root window",
"*** Unknown return code"
};
Frame frame;
Panel panel;
Panel_item drop_target[3];
Drag_drop dnd;
/*ARGSUSED*/
static void
hide_drop_targets(item, event)
Panel_item item;
Example Programs
Example Programs 745

Example F-9. The panel_dnd.c program (continued)
Event *event;
{
int i;
int show;
show = !xv_get(drop_target[0 ], XV_SHOW);
for (i=0; i<=2; i++)
xv_set(drop_target[i ],
XV_SHOW, show,
0);
xv_set(item,
PANEL_LABEL_STRING, show ? "Hide drop targets" : "Show drop targets",
0);
}
/*ARGSUSED*/
static void
inactivate_drop_targets(item, event)
Panel_item item;
Event *event;
{
int i;
int inactive;
inactive = !xv_get(drop_target[0 ], PANEL_INACTIVE);
for (i=0; i<=2; i++)
xv_set(drop_target[i ],
PANEL_INACTIVE, inactive,
0);
xv_set(item,
PANEL_LABEL_STRING, inactive ? "Activate drop targets" :
"Inactivate drop targets",
0);
}
static void
get_primary_selection(sel_req)
Selection_requestor sel_req;
{
long length;
int format;
char *sel_string;
char *string;
xv_set(sel_req, SEL_TYPE, XA_STRING, 0);
string = (char *) xv_get(sel_req, SEL_DATA, &length, &format);
if (length != SEL_ERROR) {
/* Create a NULL-terminated version of ’string’ */
sel_string = (char *) xv_calloc(1, length+1);
strncpy(sel_string, string, length);
/* Print out primary selection string */
printf("Primary selection=
} else
printf("*** Unable to get primary selection.0);
746 XView Programming Manual
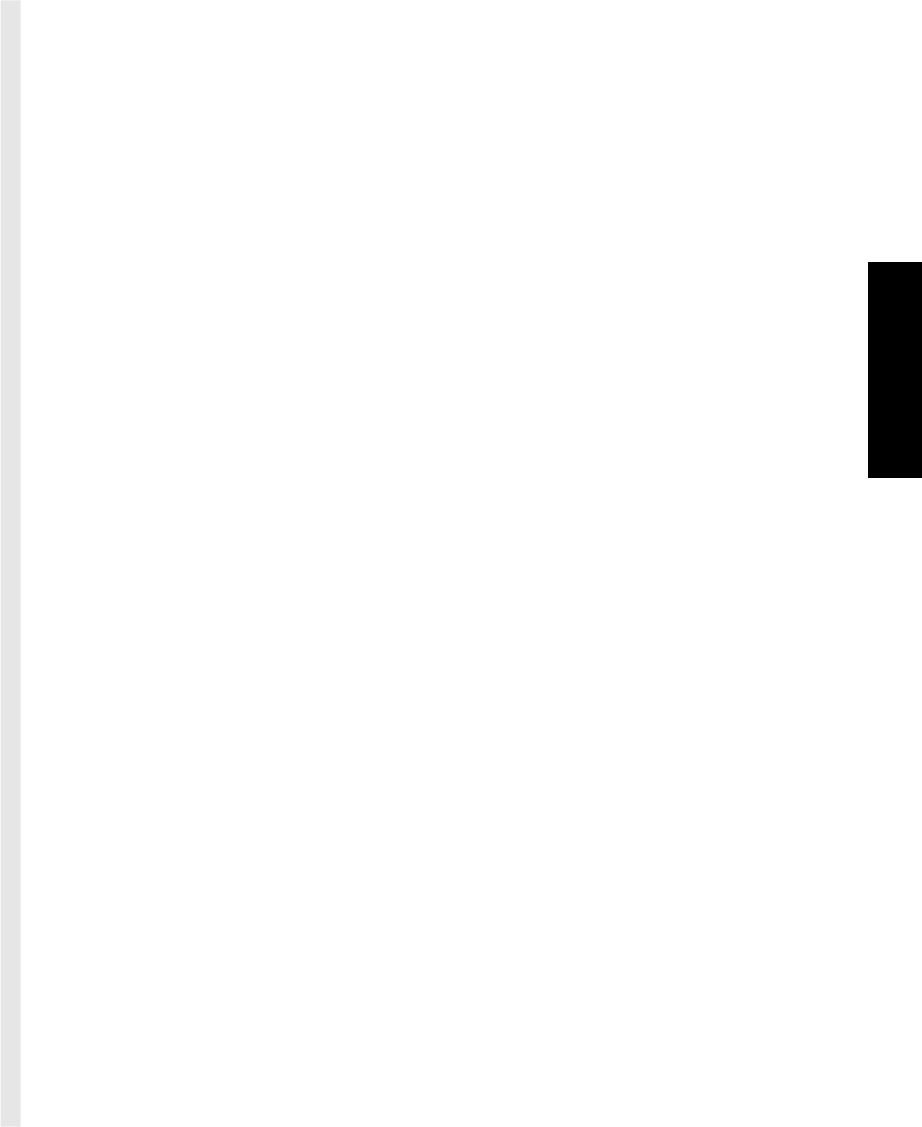
Example F-9. The panel_dnd.c program (continued)
}
static int
drop_target_notify_proc(item, value, event)
Panel_item item;
unsigned int value;
Event *event;
{
Selection_requestor sel_req;
printf("(drop_target_notify_proc) %s action= ",
xv_get(item, PANEL_LABEL_STRING));
sel_req = xv_get(item, PANEL_DROP_SEL_REQ);
switch (event_action(event)) {
case ACTION_DRAG_COPY:
printf("ACTION_DRAG_COPY,0);
get_primary_selection(sel_req);
break;
case ACTION_DRAG_MOVE:
printf("ACTION_DRAG_MOVE0);
get_primary_selection(sel_req);
break;
case LOC_DRAG:
printf("LOC_DRAG, result= %s0, dnd_codes[MIN(value, 6)]);
break;
default:
printf("%d0, event_action(event));
break;
}
return XV_OK;
}
main(argc, argv)
int argc;
char **argv;
{
Server_image busy_glyph[2];
Server_image normal_glyph[2];
Panel panel2;
xv_init(XV_INIT_ARGS, argc, argv, 0);
frame = xv_create(NULL, FRAME,
FRAME_LABEL, "Drag and Drop Test",
0);
panel = xv_create(frame, PANEL,
PANEL_LAYOUT, PANEL_VERTICAL,
0);
xv_create(panel, PANEL_BUTTON,
PANEL_LABEL_STRING, "Inactivate Drop Targets",
PANEL_NOTIFY_PROC, inactivate_drop_targets,
0);
xv_create(panel, PANEL_BUTTON,
PANEL_LABEL_STRING, "Hide Drop Targets",
Example Programs
Example Programs 747
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.