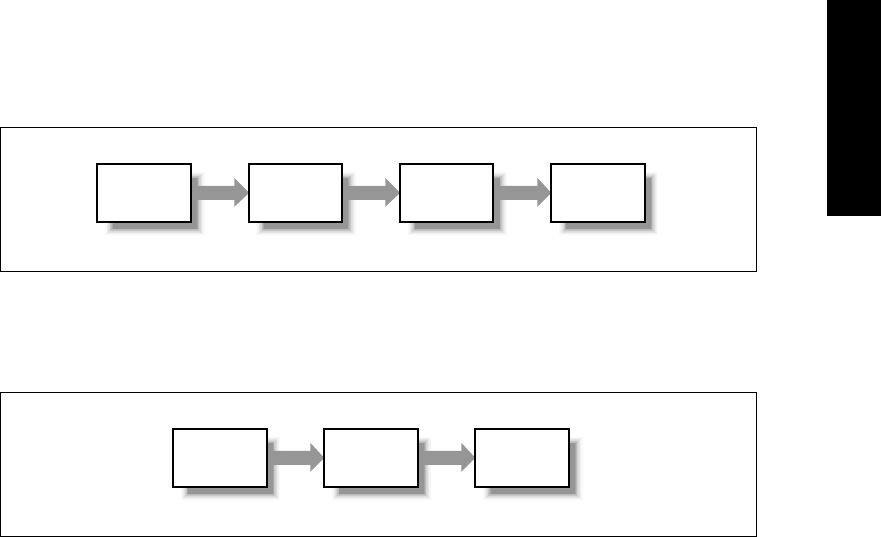
7
Panels
The XView PANEL package implements the OPEN LOOK control area. Panels are used in
many different contexts—property sheets, notices, and menus all use panels in their imple-
mentation. The main function of a panel is to manage a variety of panel items. Figure 7-3
shows examples of panel items from the OPEN LOOK GUI. Because some panel items may
not contain windows that handle their own events, the PANEL package is responsible for pro-
pagating events to the appropriate panel item. This chapter addresses issues specific to pan-
els, the management of panel items and the distribution of events to those items. We look at
basic issues common to all panel items before introducing each of the eight different panel
item packages. Finally, we look at several advanced topics regarding panel usage.
The PANEL package is subclassed from the WINDOW package. In typical usage, you create a
panel and set certain panel-specific attributes. Figure 7-1 shows the class hierarchy for pan-
els and Figure 7-2 shows the class hierarchy for a panel item.
Generic
Object
(Drawable) Window Panel
Figure 7-1. Panel package class hierarchy
Generic
Object
Generic
Panel Item
Your
Panel Item
Figure 7-2. Panel item class hierarchy
Panels
Panels 153
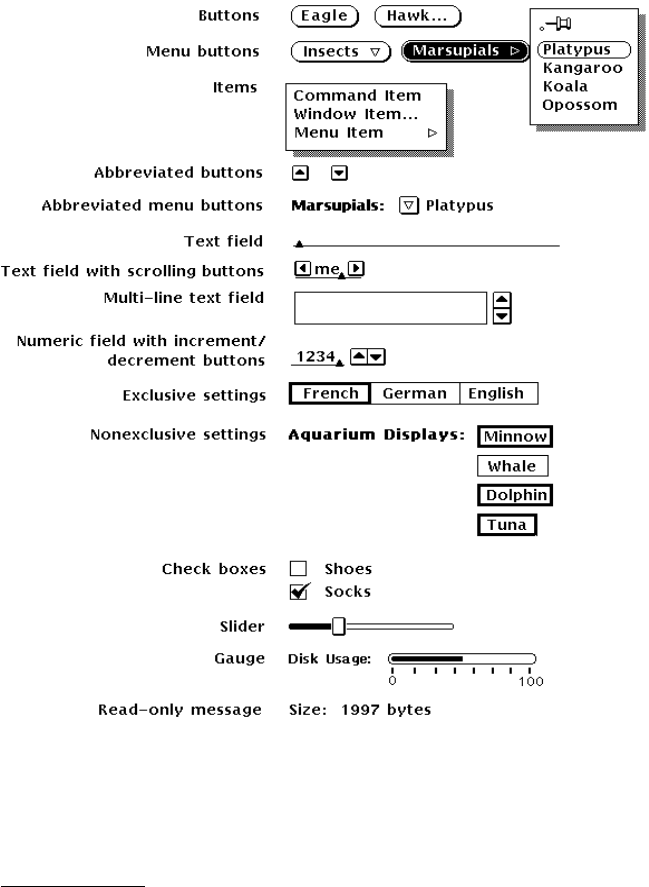
Panels set up and manage event-handling masks and routines for themselves and their panel
items. The application does not set event masks or install an event callback routine unless it
needs to track events above and beyond what the PANEL package does by default (typical
applications will not need to do this). Even so, this is probably better accomplished via inter-
posing functions as discussed in Chapter 20, The Notifier.
Figure 7-3. Controls in an
OPENLOOK GUI
implementation
*
* XView supports the scrolling list item but it is not shown here.
154 XView Programming Manual
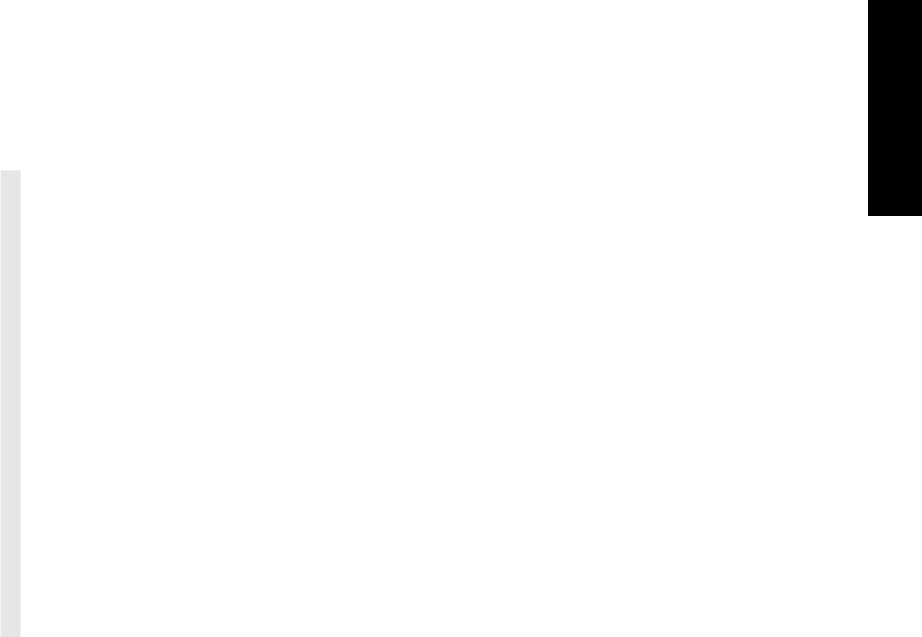
The PANEL package handles all the repainting and resizing events automatically. Panels are
not used to display graphics, so there is no need to capture repaint events. Rather than deal
with other events specifically callback routines are not installed on panels, but set for each
panel item. Because of the varying types of panel items, each item’s callback function may
be invoked by a different action from the user. While clicking on a panel button is all that is
necessary to activate the button’s callback routine, a text panel item might be configured to
call its notification callback routine when the user presses the RETURN key.
Since panel items express interest in different events, it is the responsibility of the PANEL
package to track all events within the panel’s window and dispatch events to the proper panel
item depending on its type. In some cases, if an event happens over a certain panel item and
that item is not interested in that event, the event may be sent to another panel item. For
example, what happens if a key is pressed over a panel button? Because the panel button has
no interest in the event, the panel will send the event to a text panel item, if one exists else-
where in the panel.
Section 7.19, “Advanced Panel Usage,” describes panel event handling and repainting.
7.1 Creating a Panel
You create a panel by calling xv_create() and specifying the PANEL package and a NULL-
terminated list of attribute-value pairs. A panel must be created as a child of the frame. All
programs that use panels or panel items must include <xview/panel.h>. Because panels are
uninteresting without panel items, Example 7-1 shows how to create a simple frame, a panel
and a panel button. Selecting the panel button causes the program to exit. This is the same
quit.c program as shown in Chapter 3, Creating XView Applications.
Example 7-1. The quit.c program
/*
* quit.c -- simple program to display a panel button that says "Quit".
* Selecting the panel button exits the program.
*/
#include <xview/xview.h>
#include <xview/frame.h>
#include <xview/panel.h>
Frame frame;
main (argc, argv)
int argc;
char *argv[ ];
{
Panel panel;
void quit();
xv_init (XV_INIT_ARGC_PTR_ARGV, &argc, argv, NULL);
frame = (Frame)xv_create (NULL, FRAME,
FRAME_LABEL, argv[0],
XV_WIDTH, 200,
Panels
Panels 155

Example 7-1. The quit.c program (continued)
XV_HEIGHT, 100,
NULL);
panel = (Panel)xv_create (frame, PANEL, NULL);
(void) xv_create (panel, PANEL_BUTTON,
PANEL_LABEL_STRING, "Quit",
PANEL_NOTIFY_PROC, quit,
NULL);
xv_main_loop (frame);
exit(0);
}
void
quit()
{
xv_destroy_safe(frame);
}
7.1.0.1 Fonts and panels
OPEN LOOK is somewhat restrictive about the use of fonts within panels. Many panel items
cannot have their fonts changed at all. Of those that can, none can have their fonts set indi-
vidually.* However, if a font is set in the panel itself, it is then inherited by the panel items
whose fonts can be changed. This guarantees the consistency of fonts in panels. In addition,
a panel’s font may only be specified when the panel is created.
7.1.1 Scrollable Panels
Scrollable panels are not OPEN LOOK-compliant, but are provided for historical reasons.
They are basically just like panels, except that typically not all panel items are in view. A
vertical scrollbar attached to the panel allows the user to navigate to the panel items desired.
Again, because this type of interface is not OPEN LOOK-compliant, you are discouraged from
using this package.
In order to deal with the complications involved with attaching a scrollbar to a panel, the
scrollable panel package is subclassed from the CANVAS package and thus, the OPENWIN
package. Scrollable panels are created the same way panels are, but the package name to use
is
SCROLLABLE_PANEL. The scrollable panel package does not create the scrollbars, how-
ever. You must create them separately:
*The exact list of panel items varies and may change. Currently, it includes the text used in the value of panel text
items.
156 XView Programming Manual

Scrollable_panel sp;
Scrollbar sb;
sp = xv_create(frame, SCROLLABLE_PANEL, NULL);
sb = xv_create(sp, SCROLLBAR, NULL);
The principle difference between canvases and scrollable panels is the management of events
and the existence of panel items. In canvases, the programmer installs callback routines for
events and for repaint and resize routines, and an input mask is set for notification of certain
events that the application is interested in. However, like normal panels, the scrollable panel
does this automatically. Other than this, scrollable panels may take the same attributes as
normal panels.
7.2 Creating Panel Items
Like other XView object, panel items are created using xv_create():
Panel_item
xv_create(panel, item_type,
attrs
)
Panel panel;
<item type> item_type;
<attribute-value list>
attrs
;
The value of item_type must be a panel item from one of the panel item packages:
• PANEL_ABBREV_MENU_BUTTON
• PANEL_BUTTON
• PANEL_CHECK_BOX
• PANEL_CHOICE
• PANEL_CHOICE_STACK
• PANEL_DROP_TARGET
• PANEL_GAUGE
• PANEL_LIST
• PANEL_MESSAGE
• PANEL_MULTILINE_TEXT
• PANEL_NUMERIC_TEXT
• PANEL_SLIDER
• PANEL_TEXT
• PANEL_TOGGLE
Panels
Panels 157
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.