
7.4.2.1 General positioning of items
Two functions that are available for general positioning of panel items within windows are
xv_row() and xv_col(). These functions use the values of WIN_ROW_GAP and
WIN_COLUMN_GAP of the panel. While these attributes control the spacing between panel
items, the distance between items and the edge of the panel is set to a constant 4 pixels.
Consider the following code fragment that positions items within regimented rows and
columns:
int rows, cols;
extern char *names[3][5];
frame = (Frame)xv_create(NULL, FRAME, NULL);
panel = (Panel)xv_create(frame, PANEL,
WIN_ROW_GAP, 70,
WIN_COLUMN_GAP, 20,
NULL);
for (rows = 0; rows < 3; rows++)
for (cols = 0; cols < 5; cols++)
(void) xv_create(panel, PANEL_BUTTON,
XV_X, xv_col(panel, cols),
XV_Y, xv_row(panel, rows),
PANEL_LABEL_STRING, names[rows][cols],
PANEL_NOTIFY_PROC, (rows+cols==0)? quit : selected,
PANEL_CLIENT_DATA, frame,
NULL);
This code displays a panel with a 70-pixel distance between the upper-left corners of the pan-
el items. Thus, each panel item must be less than 70 pixels wide or they will overlap one an-
other. This contrasts with
PANEL_ITEM_X_GAP and PANEL_ITEM_Y_GAP, which specify the
gap between the right and left sides of adjacent panel items. Note that this does not affect the
distance between the edge of the panel and the items along the perimeter; that distance re-
mains constant at 4 pixels.
The xv_col() and xv_row() method of explicit panel item placement does not take into
account the sizes of the panel items. If not enough space is given between the rows and
columns (
WIN_COLUMN_GAP, WIN_ROW_GAP), then items will lie on top of one another. Nei-
ther does this method take into account dynamic scaling of items and fonts. As a result, this
and all absolute positioning methods are not recommended.
7.4.3 Layout of Panel Items with Values
For panel items with values, PANEL_VALUE_X and PANEL_VALUE_Y can be used instead of
the XV_X and XV_Y attributes mentioned above to align the value rectangles of panel items.
The label portion of the panel item is then positioned automatically according to the panel
layout characteristics currently in effect. This is useful, for example, when you need to align
a series of PANEL_TEXT items. It is important not to use XV_X and XV_Y when using the label
and value positioning attributes.
Panels
Panels 163

7.5 Sizing Panels
The size of a panel, by default, extends to the bottom and right edges of the frame in which it
is placed (assuming there are no other subwindows in the frame). Alternatively, the panel’s
dimensions can be set explicitly using XV_WIDTH and XV_HEIGHT. If it is important to main-
tain the layout of the panel items in a panel, then the dimensions should be set explicitly.
More often than not, you want the panel to be just the minimum height and width required to
encompass all of its items. You can set the minimum height and width using the macros
window_fit_height() or window_fit_width(), respectively. You can set both in
a single call to window_fit(). These macros are called after all the items have been
created.
The attributes PANEL_EXTRA_PAINT_WIDTH and PANEL_EXTRA_PAINT_HEIGHT specify the
increment by which a panel will grow in the x and y directions, respectively.
7.6 Panel Item Values
Many panel items are associated with a specific value. A text item has a string value, a
numeric text item has an integer value, a choice item has a current-choice value, and so on.*
To set a value, use:
xv_set(item, PANEL_VALUE, value, NULL)
Of course, the type of value is dependent on the type of panel item whose value is being
set. Consider the following examples:
/* Set the text field in the text item to print "Hello World" */
xv_set(text_item, PANEL_VALUE, "Hello world.", NULL);
/* Set the numeric value of the numeric text item to be 10 */
xv_set(text_num_item, PANEL_VALUE, 10, NULL);
/* Set the current choice in choice_item to be the fifth choice */
xv_set(choice_item, PANEL_VALUE, 4, NULL);
NOTE
The values for string-valued attributes are dynamically allocated when they are
created or set. The value you specify is copied into the newly allocated space. If
a previous value was present, the panel item frees its old data first.†
Panel item values are retrieved in a similar way:
xv_get(item, PANEL_VALUE);
*For details about the value type of a panel item, see the corresponding sections on specific panel items (Sections 7.9
through 7.18).
†This contrasts with menu items. See Chapter 11, Menus.
164 XView Programming Manual

Since the xv_get() routines are used to retrieve attributes of all types, you should cast the
value returned into the type appropriate to the attribute being retrieved:
int val;
val = (int)xv_get(num_text_item, PANEL_VALUE);
printf("The int–value in the num_text_item is: ’%d’0, val);
NOTE
xv_get() does not dynamically allocate storage for the values it returns. If the
value returned is a pointer, it points directly into the panel’s private data. It
should be considered read-only—do not change the contents of the pointer; it is
your responsibility to copy the information pointed to.
7.7 Iterating Over a Panel’s Items
You can iterate over each item in a panel with the two attributes PANEL_FIRST_ITEM and
PANEL_NEXT_ITEM. A pair of macros, PANEL_EACH_ITEM() and PANEL_END_EACH are also
provided for this purpose. For example, to destroy each item in a panel:
Panel_item item;
PANEL_EACH_ITEM(browser, item)
xv_destroy(item);
PANEL_END_EACH
Note that a semicolon is not required after PANEL_END_EACH.
7.8 Panel Item Classes
Nine types of panel items are presented here:
• Panel Buttons, Menu Buttons, and Abbreviated Menu Buttons
• Checkboxes
• Exclusive and Nonexclusive Choices
• Abbreviated Choices
• Scrolling Lists
• Message Items
• Sliders
• Text Items (including numeric and multiline text items)
• Drop Target Items
Panels
Panels 165

Items are made up of one or more displayable components. One component shared by all
item types is the label. An item label is either a string or a graphic image.
The user interacts with items through various methods ranging from mouse button selection
to keyboard input. This interaction typically results in a callback function being called for
the panel item. The callback functions also vary on a per-item basis. Each item type is de-
scribed in the following sections.
7.9 Button Items
A button item allows the user to invoke a command or bring up a menu. Examples of various
buttons are listed in Figure 7-6. The button’s label identifies the name of the command or
menu. A button label that ends in three dots ( . . . ) indicates that a pop-up menu will be
displayed when the button is selected.
Figure 7-6. Visual feedback for button controls
A button requires a label specified by the attribute PANEL_LABEL_STRING or PANEL_LA-
BEL_IMAGE
. Buttons do not have a PANEL_VALUE associated with them.
166 XView Programming Manual
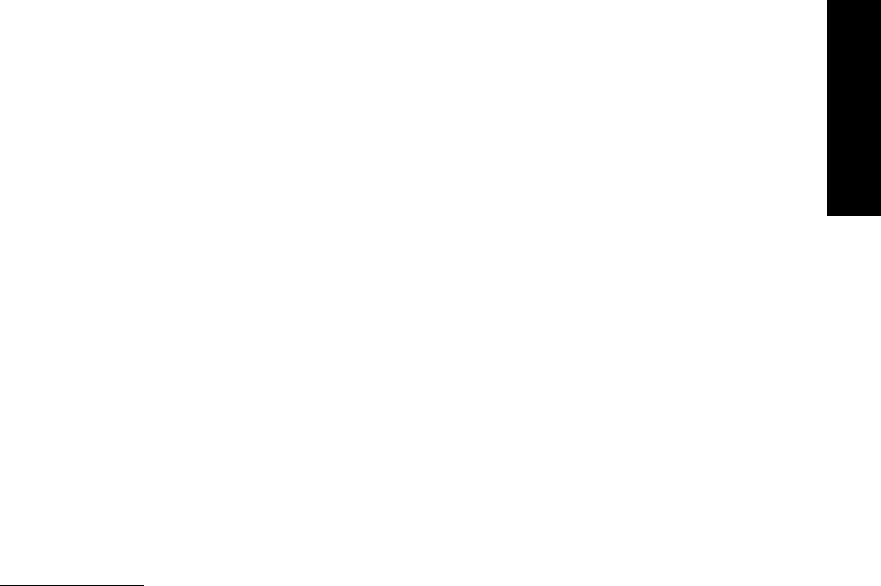
7.9.1 Button Selection
The user invokes a panel button by clicking the SELECT mouse button on it. When this hap-
pens, the button’s notify procedure is called. The notify procedure is installed by specifying
PANEL_NOTIFY_PROC, as in the following example:
xv_create(panel, PANEL_BUTTON,
PANEL_NOTIFY_PROC, quit_proc,
PANEL_LABEL_STRING, "Quit",
NULL);
When the button is selected, the notify procedure is called. The form of the notify procedure
for a button is:
void
button_notify_proc(item, event)
Panel_item item;
Event *event
The function does not return a value, but if the action that the button had intended to take
fails, then you should set the item’s PANEL_NOTIFY_STATUS to XV_ERROR (e.g., if the button
was labeled “save” but the actual save operation failed). It is set to XV_OK by default. If the
button is part of an unpinned Command Frame, setting PANEL_NOTIFY_STATUS to XV_ERROR
will prevent the Command Frame from being dismissed. Use MENU_NOTIFY_STATUS for a
menu. In a callback, setting this to XV_ERROR on the menu that was notified prevents the
frame from being dismissed.
When a button’s notify procedure is called, the button’s busy state is set. When a button’s
busy state is set to TRUE, the button does not accept further input (clicking the SELECT mouse
button will do nothing to the button item). The busy state is cleared when the notify proce-
dure exits. The busy state can be maintained after exiting the notify procedure by setting
PANEL_BUSY to TRUE from within the notify procedure.
7.9.1.1 Making a button inactive
The attributes
PANEL_INACTIVE is used to make a panel button inactive. If TRUE, the button
item cannot be selected. Inactive items are displayed with gray-out pattern as shown in Fig-
ure 7-6.
7.9.2 Menu Buttons
It is often useful to attach a menu to a button. The menu may provide alternate values or
functions for the button to invoke. Since the menu is a separate entity (in other words, it is
not created when the button is created—you have to create it on your own), the menu may
have callback routines associated with it and its menu items.*
*Read Chapter 11, Menus, for details of menus and menu items.
Panels
Panels 167
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.