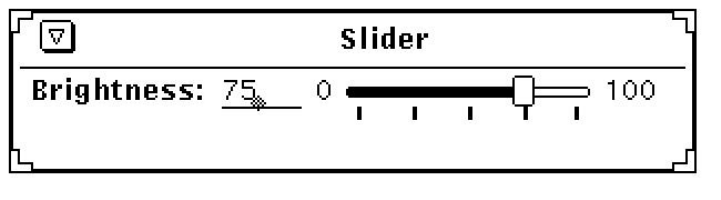
The following code fragment produces a slider with a label:
xv_create(panel, PANEL_SLIDER,
PANEL_LABEL_STRING, "Brightness: ",
PANEL_VALUE, 75,
PANEL_MIN_VALUE, 0,
PANEL_MAX_VALUE, 100,
PANEL_SLIDER_WIDTH, 300,
PANEL_TICKS, 5,
PANEL_NOTIFY_PROC, brightness_proc,
NULL);
The output is shown in Figure 7-17.
Figure 7-17. Sample panel with slider item
7.14 Gauges
Gauges are just like sliders, but they are “output only” items. That is, you set the value of the
item and the display of the gauge changes just as it would for sliders. Also, there is no op-
tional type-in field and there is no drag box for the user to interactively change the value of
the gauge. The gauge is intended to be used only as a feedback item.
To create a gauge, use the PANEL_GAUGE package. To set a gauge’s width or height, use
PANEL_GAUGE_WIDTH. This attribute sets the length of the object, whether it is vertically or
horizontally oriented. As with the slider, the orientation is set by the attribute
PANEL_DIRECTION.
7.15 Text Items
A panel text item contains as its value a NULL-terminated string. It contains only printable
characters with no newlines. When a panel receives keyboard input (regardless of where the
pointer is as long as it is within the boundaries of the panel), the keyboard event is passed to
the item with the keyboard focus. A caret is used to indicate the insertion point where new
text is added. You can type in more text than fits on the text field. If this happens, a right ar-
row pointing to the left will appear on the left on the field, indicating that some text to the left
of the displayed text is no longer visible. Similarly, if text is inserted causing text on the
188 XView Programming Manual
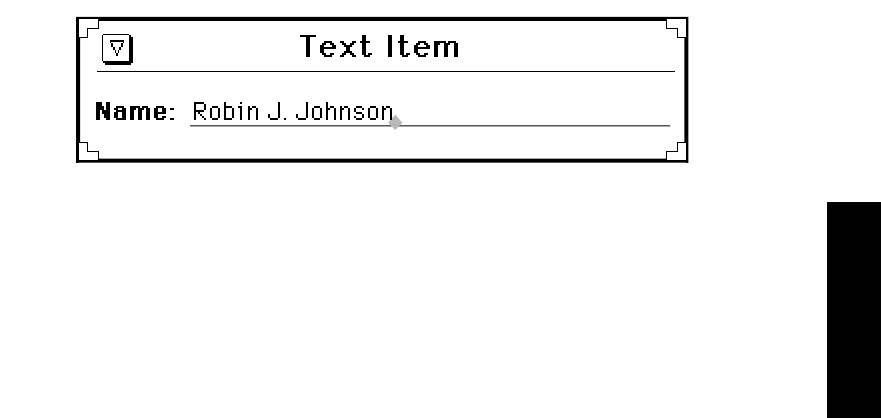
right to move out of the visible portion of the text item, then an arrow pointing to the right
will appear to the right of the text.
Text items use the attribute PANEL_LABEL_STRING as do most other panel items, to label the
text item. The value of a text item is also a string, an is set by the attribute PANEL_VALUE, as
shown by the following code:
xv_create(panel, PANEL_TEXT,
PANEL_LABEL_STRING, "Name:",
PANEL_VALUE, "Edward G. Robinson",
NULL);
The output from this code fragment is shown in Figure 7-18.
Figure 7-18. Sample panel with text item
If the item’s PANEL_LAYOUT is set to PANEL_VERTICAL, the value is placed below the label.
The default is PANEL_HORIZONTAL.
The number of characters of the text item’s value that are displayed is set via
PANEL_VAL-
UE_DISPLAY_LENGTH
. Note that the length of the value display specified by PANEL_VAL-
UE_DISPLAY_LENGTH
may not be less than the combined width of the left and right “more
text” buttons. In 12-point font, this is four characters.
PANEL_VALUE_DISPLAY_LENGTH is useful for fixed width characters. PANEL_VALUE_DIS-
PLAY_LENGTH
converts characters to pixels by multiplying the number of characters by the
default character width for the font being used. To set the number of characters in the text
item’s value that are displayed in a variable width font, use PANEL_VALUE_DISPLAY_WIDTH.
The argument for PANEL_VALUE_DISPLAY_WIDTH is expressed in pixels instead of charac-
ters. The maximum number of characters that can be typed into a text item (independently of
how many are displayable) is set via the attribute
PANEL_VALUE_STORED_LENGTH. When characters are entered beyond the display length,
and when PANEL_VALUE_STORED_LENGTH is greater than the display length, the value string
is scrolled one character to the left so that the most recently entered character is always visi-
ble. As the string scrolls to the left, the leftmost characters move out of the visible display
area. The presence of these temporarily hidden characters is indicated by a small left-point-
ing triangle.
It is sometimes desirable to have a protected field where the user can enter confidential infor-
mation. The attribute PANEL_MASK_CHAR is provided for this purpose. When the user enters
a character, the character specified as the value of PANEL_MASK_CHAR will be displayed in
place of the character the user has typed. Setting PANEL_MASK_CHAR to an asterisk (*) would
Panels
Panels 189
produce a string of asterisks instead of the characters typed. The value of the text is still the
string the user types.
If you want to disable character echo entirely so that the caret does not advance and it is im-
possible to tell how many characters have been entered, use the space character as the mask.
You can remove the mask and display the actual value string at any time by setting the mask
to NULL.
7.15.1 The Current Keyboard Focus
A panel may have several keyboard focus items that can accept keyboard input. Only one of
these items may be current at any given time. The current keyboard focus item is the one to
which keyboard input is directed and is indicated by a caret at the item’s value. Selection of
a keyboard focus item (i.e., pressing and releasing the SELECT mouse button anywhere with-
in the item’s bounding box) causes that item to become the current keyboard focus item.
NOTE
When the resource OpenWindows.KeyboardCommands is set to SunView1
or Basic, only text items will receive the keyboard focus within a panel.
You can find out which keyboard focus item has the caret or give the caret to a specified key-
board focus item by means of the panel attribute
PANEL_CARET_ITEM:
current_item = (Panel_item)xv_get(panel, PANEL_CARET_ITEM);
xv_set(panel, PANEL_CARET_ITEM, another_item, NULL);
You can set the current item to the next or previous keyboard focus item in the panel by using
the following two routines:
Panel_item
panel_advance_caret(panel)
Panel panel;
Panel_item
panel_backup_caret(panel)
Panel panel;
They return the new panel item that has received the keyboard focus. Advancing past the last
keyboard focus item places the keyboard focus at the first keyboard focus item, while back-
ing up past the first keyboard focus item places the keyboard focus at the last keyboard focus
item.
190 XView Programming Manual
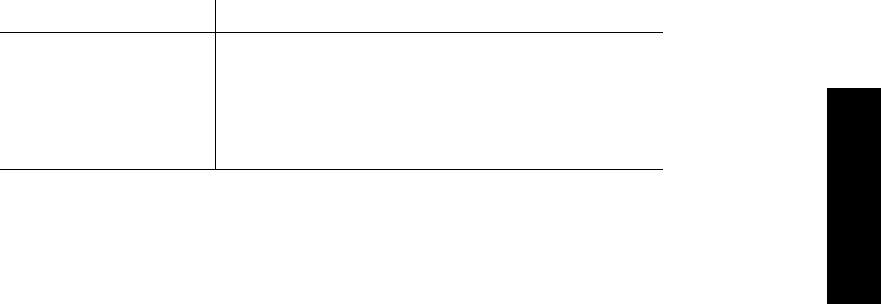
7.15.2 Text Selection
You can use the attribute PANEL_TEXT_SELECT_LINE to select and highlight the entire con-
tents of the text field.
7.15.3 Text Notification
The notification behavior of text items is more complex than that of other item types. You
can control whether your notify procedure is called on each input character or only on select-
ed characters.
When your notify procedure will be called is determined by the value of
PANEL_NOTIFY_LEVEL. Possible values are given in Table 7-1.
Table 7-1. Text Item Notification Level
Notification Level Level Causes Notify Procedure to be Called . . .
PANEL_NONE Never
PANEL_NON_PRINTABLE On each non-printable input character.
PANEL_SPECIFIED If the input char is found in the string given for the
attribute PANEL_NOTIFY_STRING.
PANEL_ALL On each input character.
The default for PANEL_NOTIFY_LEVEL is PANEL_SPECIFIED, and the default for
PANEL_NOTIFY_STRING is \n\r\t (i.e., notification on line-feed, carriage-return and tab).
The value PANEL_SPECIFIED only works for characters that do not map to semantic actions.
To provide notification for characters that map to semantic actions, such as 177 (delete nor-
mally maps to ACTION_ERASE_CHAR_BACKWARD), use either PANEL_EVENT_PROC,
notify_interpose_event_func(), or set PANEL_NOTIFY_LEVEL to PANEL_ALL.
The user’s editing characters are treated specially (for example the backspace character).
They are not appended to the value string. If you have asked for a character by including it
in
PANEL_NOTIFY_STRING, the PANEL package calls your notify procedure. After the notify
procedure returns, the appropriate editing operation will be applied to the value string. (Note
that the editing characters are never appended to the value string, regardless of the return val-
ue of the notify procedure.)
Characters other than the special characters described above are treated as follows. If the
character typed by the user does not result in your notify procedure getting called, then the
character, if printable, is appended to the value string. If it is not printable, it is ignored. If
your notify procedure is called, what happens to the value string and whether the keyboard
focus moves to another item is determined by the notify procedure’s return value. Table 7-2
shows the possible return values.
Panels
Panels 191
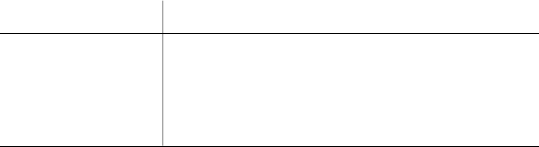
Table 7-2. Return Values for Text Item Notify Procedures
Value Returned Action Caused
PANEL_INSERT Character is appended to item’s value.
PANEL_NEXT Keyboard focus moves to next text item.
PANEL_PREVIOUS Keyboard focus moves to previous text item.
PANEL_NONE Ignore the input character.
If you do not specify your own notify procedure, the default procedure,
panel_text_notify(), is called at the appropriate time as determined by the setting of
PANEL_NOTIFY_LEVEL.
7.15.4 Writing Your Own Text Notify Procedure
By writing your own notify procedure, you can tailor the notification behavior of a given
keyboard focus item to support the needs of any application. At one extreme, you may want
to process each character as the user types it in. For a different application, you may not care
about the characters as they are typed in and may only want to look at the value of the string
in response to some other button. A typical example is getting the value of a filename field
when the user presses a Load File panel button.
The form of the text notification procedure is:
Panel_setting
panel_text_notify(item, event)
Panel_item item;
Event *event;
This procedure returns a panel setting enumeration that has the following effects:
PANEL_NONE Do not advance the keyboard focus to the next keyboard focus item.
The current keyboard focus item and insertion point remain un-
changed.
PANEL_NEXT The keyboard focus moves to the next keyboard focus item (defined
by the keyboard focus item that was created after the current key-
board focus item). If there is no next text item, the first keyboard fo-
cus item in the panel is used.
PANEL_PREVIOUS The keyboard focus moves to the previous keyboard focus item in the
panel (defined by the keyboard focus item that was created before the
current keyboard focus item). If there is no previous keyboard focus
item, the last keyboard focus item in the panel is used.
PANEL_INSERT The character which caused the notification procedure to be called is
inserted into the text item’s value at the location of the caret (insertion
point).
192 XView Programming Manual
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.