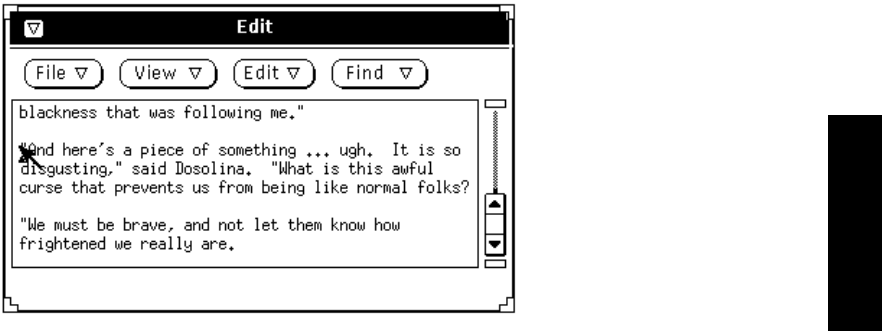
8
Text Subwindows
This chapter describes the TEXTSW package, which allows a user or client to display and edit
a sequence of ASCII characters. Figure 8-1 shows an example of a text subwindow. The text
contains a vertical scrollbar but may not contain a horizontal scrollbar. The vertical scrollbar
can be used to split views into several views (see Chapter 5, Canvases and Openwin). The
font used by the text can be specified using the TEXTSW_FONT attribute, but only one font per
text subwindow can be used, regardless of how many views there may be.
The contents of a text subwindow are stored in a file or in memory on the client side, not on
the X server. Whether the source of the text is stored on disk or in memory is transparent to
the user. When the user types characters in the text subwindow, the source might be changed
immediately or synchronized later depending on how the text subwindow is configured. The
TEXTSW package provides basic text editing features such as inserting arbitrary text into a
file. It also provides complex operations such as searching for and replacing a string of text.
Figure 8-1. A sample text subwindow
Text Subwindows
Text Subwindows 215

8.1 Creating Text Subwindows
Applications need to include the file <xview/textsw.h> to use text subwindows. You create a
text subwindow the same way you create any XView object, by calling xv_create() with
the appropriate type parameters:
Textsw textsw;
textsw = (Textsw)xv_create(base_frame, TEXTSW, NULL);
The font used by the text can be specified using TEXTSW_FONT; only one font per text
subwindow can be used. Figure 8-2 shows the class hierarchy for the text subwindows.
Generic
Object
(Drawable) Window Textsw(Openwin)
Figure 8-2. Textsw class hierarchy
8.2 Setting Text Subwindow Attributes
As for all XView objects, you can set attribute-value pairs to configure the text subwindow
accordingly. Like the
CANVAS
package, the text subwindow object is subclassed from the
OPENWIN package and can therefore be split into separate views. The package handles all of
its own events and redisplaying of text, so none of these things is handled by the application.
Most text subwindow attributes are orthogonal; that is, attribute order does not effect the
object. In a few cases, however, the attributes in a list might interact, so you must specify
them in a particular order. Such cases are noted in the sections that follow. For example, you
must pass
TEXTSW_STATUS first in any call to xv_create(), if you want to find the status
after setting some other attribute in the same call.
8.3 Text Subwindow Contents
The contents of a text subwindow are a sequence of characters. Each character can be
uniquely identified by its position in the sequence (type Textsw_index). Editing opera-
tions, such as inserting and deleting text, can cause the index of successive characters to
change. The valid indices are 0 through length –1 inclusive, where length is the number of
characters currently in the text subwindow, returned by the TEXTSW_LENGTH attribute.
216 XView Programming Manual
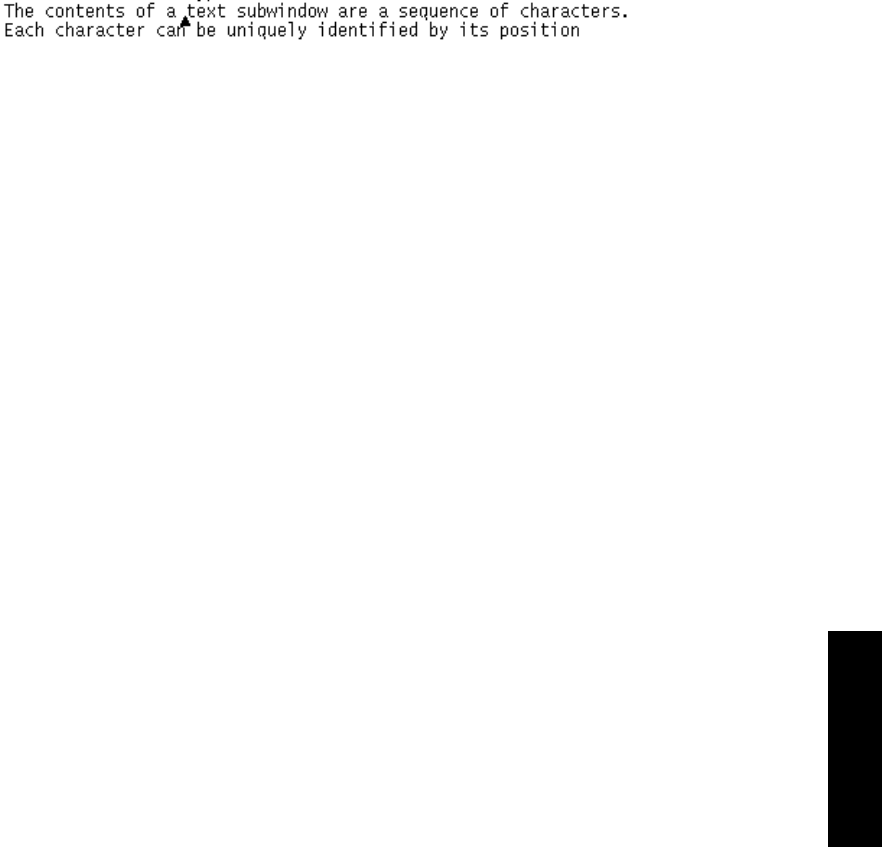
The text subwindow has a notion of the current index after which the next character will be
inserted. This is called the insertion point and is indicated by a caret, as shown in Figure 8-3.
Figure 8-3. A caret marks the insertion point
8.4 Editing a Text Subwindow
A text subwindow can be edited by the user or by a client program. When you create a text
subwindow, the user is normally allowed to edit it. By using the special attributes discussed
in this section, the client program can edit the subwindow. These edits are then stored in
/tmp/textProcess-id.Counter.
The next five sections explain the functions and attributes that you will use to load, read,
write, edit, and finally save a text file.
8.4.1 Loading a File
You can load a file into a text subwindow by using
TEXTSW_FILE, as in:
xv_set(textsw, TEXTSW_FILE, file_name, NULL);
Keep in mind that if the existing text has been edited, then these edits will be lost. To avoid
such loss, first check whether there are any outstanding edits by calling:
int modified = (int)xv_get(textsw, TEXTSW_MODIFIED)
If there have been updates, you may choose to synchronize with the source if necessary. That
is, if the existing text is part of a file, you can overwrite the existing changes before loading
in a new file.
The above call to xv_set(), which loads the new file, positions the new text so that the first
character displayed has the same index as the first character that was displayed in the previ-
ous file. This is probably not what you want. The code segment below shows how to load
the file at a set position:
xv_set(textsw,
TEXTSW_FILE, file_name,
TEXTSW_FIRST, position,
NULL);
The first character displayed has its index set by position. The order of these attributes
matters. Because attributes are evaluated in the order given, reversing the order would first
reposition the existing file, then load the new file. This would cause an unnecessary repaint.
It would also mis-position the old file if it was shorter than position.
Text Subwindows
Text Subwindows 217
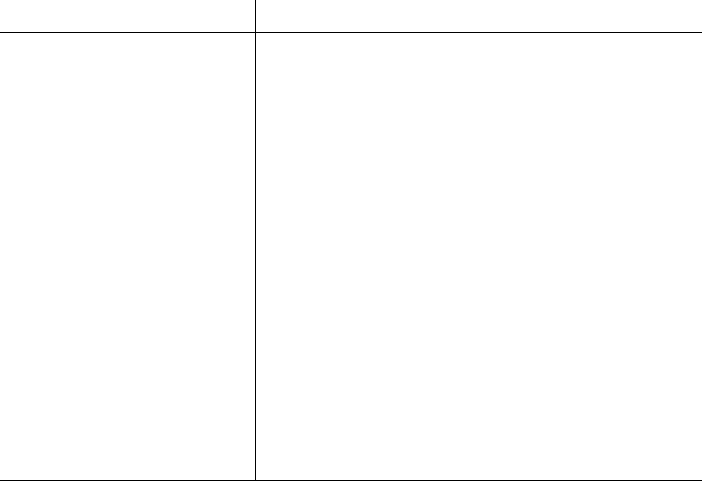
8.4.2 Checking the Status of the Text Subwindow
Both of the calls in the previous example blindly trust that the load of the new file was suc-
cessful. This is, in general, a bad idea. The following code segment shows how to find out
whether the load succeeded, and if not, why it failed:
Textsw textsw;
Textsw_status status;
textsw = (Textsw)xv_create(base_frame,
TEXTSW,
TEXTSW_STATUS, &status,
TEXTSW_FILE, file_name,
TEXTSW_FIRST, position,
NULL);
NOTE
The
TEXTSW_STATUS attribute and handle must appear in the attribute list before
the operation whose status you want to determine.
The
TEXTSW_STATUS attribute is only valid for xv_create().
The range of values for such a variable are enumerated in Table 8-1. Note that in the first
column, each value begins with the prefix TEXTSW_STATUS_, which has been omitted from
the table to improve readability.
Table 8-1. Range of Values for Status Variables
Value (TEXTSW_STATUS_ . . . ) Description
OKAY The operation encountered no problems.
BAD_ATTR
The attribute list contained an illegal or unrecog-
nized attribute.
BAD_ATTR_VALUE
The attribute list contained an illegal value for an
attribute, usually an out-of-range value for an enu-
meration.
CANNOT_ALLOCATE
A call to calloc(2) or malloc(2) failed.
CANNOT_OPEN_INPUT The specified input file does not exist or cannot be
accessed.
CANNOT_INSERT_FROM_FILE The operation encountered a problem when trying to
insert from file.
OUT_OF_MEMORY The operation ran out of memory while editing in
memory.
OTHER_ERROR The operation encountered a problem not covered by
any of the other error indications.
218 XView Programming Manual

8.4.3 Writing to a Text Subwindow
To insert text into a text subwindow at the current insertion point, call:
Textsw_index
textsw_insert(textsw, buf, buf_len)
Textsw textsw;
char *buf;
int buf_len;
The return value is the number of characters actually inserted into the text subwindow. This
number will equal buf_len unless either the text subwindow has had a memory allocation
failure or the portion of text containing the insertion point is read only. The insertion point is
moved forward by the number of characters inserted.
This routine does not do terminal-style interpretation of the input characters. Thus, editing
characters (such as CTRL-H or DEL for character erase) are simply inserted into the text
subwindow rather than performing edits to the existing contents of the text subwindow. To
emulate a terminal, scan the characters to be inserted and invoke textsw_edit() where
appropriate, as described in the next section.
8.4.3.1 Setting the insertion point
The attribute
TEXTSW_INSERTION_POINT is used to interrogate and set the insertion point.
For instance, the following call determines where the insertion point is:
Textsw_index point;
point = (Textsw_index)xv_get(textsw, TEXTSW_INSERTION_POINT);
Whereas the following call sets the insertion point to be just before the third character of the
text:
xv_set(textsw, TEXTSW_INSERTION_POINT, 2, NULL);
To set the insertion point at the end of the text, set TEXTSW_INSERTION_POINT to the special
index TEXTSW_INFINITY. This call does not ensure that the new insertion point will be visi-
ble in the text subwindow, even if TEXTSW_INSERT_MAKES_VISIBLE is TRUE. To guarantee
that the caret will be visible afterwards, call textsw_possibly_normalize(), a pro-
cedure that is described later in this chapter.
8.4.4 Reading from a Text Subwindow
Many applications that incorporate text subwindows never need to read the contents of the
text directly from the text subwindow. For instance, the text subwindow might display text
for the user to view but not to edit.
Even when the user is allowed to edit text, some applications simply wait for the user to per-
form some action that indicates that all of the edits have been made. The application can
then use either textsw_save() or textsw_store_file() to place the text in the file.
Text Subwindows
Text Subwindows 219
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.