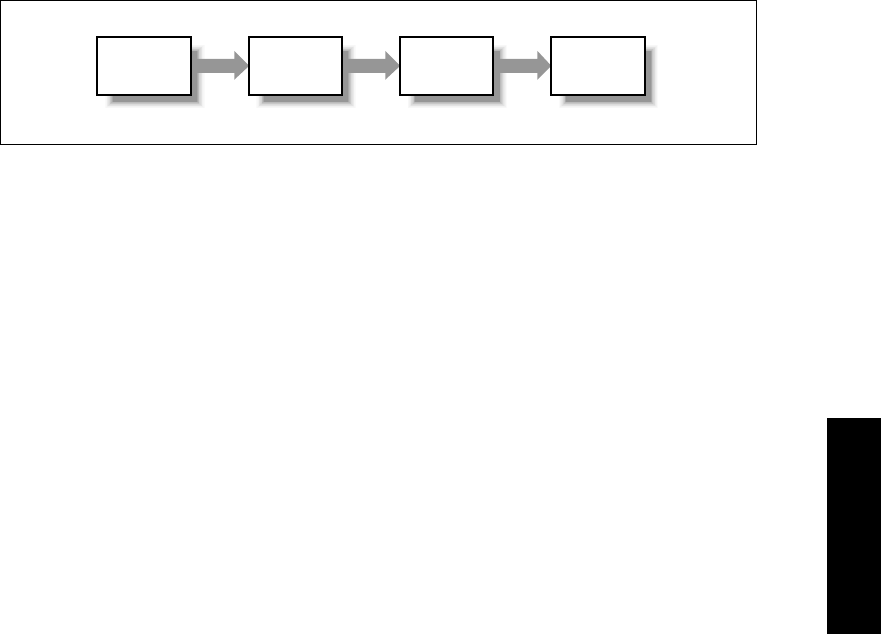
9
TTY Subwindows
The TTY (or terminal emulator) subwindow emulates a standard terminal, the principal dif-
ference being that the row and column dimensions of a TTY subwindow can vary from that
of a standard terminal. In a TTY subwindow, you can run arbitrary programs, including a
complete interactive shell. Or you can emulate terminal interface applications that use the
curses(3X) terminal screen optimization package without actually running a separate pro-
cess. The TTY subwindow accepts the standard ANSI escape sequences for doing ASCII
screen manipulation, so you can use termcap or termio screen-handling routines. This chap-
ter discusses the TTYSW package. Figure 9-1 shows the class hierarchy for the TTYSW pack-
age.
Generic
Object
(Drawable) Window Tty
Figure 9-1. TTY package class hierarchy
9.1 Creating a TTY Subwindow
Programs using TTY subwindows must include the file <xview/tty.h>. Like all XView win-
dows, you create a TTY subwindow by calling xv_create() with the appropriate type
parameter, as in:
Tty tty;
tty = xv_create(frame, TTY, NULL);
The default TTY subwindow will fork a shell process and the user can use it interactively to
enter commands. This program does not interact with the processing of the application in
which the TTY subwindow resides; it is an entirely separate process. For example, if you
want to start the TTY subwindow with another program, say man, you can do so by specify-
ing the name of the program to run via the TTY_ARGV attribute, as shown in Example 9-1.
TTY Subwindows
TTY Subwindows 241

Example 9-1. The sample_tty.c program
/*
* sample_tty.c -- create a base frame with a tty subwindow.
* This subwindow runs a UNIX command specified in an argument
* vector as shown below. The example does a "man cat".
*/
#include <xview/xview.h>
#include <xview/tty.h>
char *my_argv[ ] = { "man", "cat", NULL };
main(argc, argv)
char *argv[ ];
{
Tty tty;
Frame frame;
xv_init(XV_INIT_ARGC_PTR_ARGV, &argc, argv, NULL); |
frame = (Frame)xv_create(NULL, FRAME, NULL);
tty = (Tty)xv_create(frame, TTY,
WIN_ROWS, 24,
WIN_COLUMNS, 80,
TTY_ARGV, my_argv,
NULL);
window_fit(frame);
xv_main_loop(frame);
}
The output of Example 9-1 is shown in Figure 9-2. Note that you can have only one TTY
subwindow per process.
9.2 Driving a TTY Subwindow
You can drive the terminal emulator programmatically. There are procedures both to send
input to the terminal emulator (as if the user had typed it in the TTY subwindow) and to send
output (as if a program running in the TTY subwindow had output it). You can send input to
a TTY subwindow programmatically with the function:
int
ttysw_input(tty, buf, len)
Tty tty;
char *buf;
int len;
ttysw_input() appends the character sequence in buf that is len characters long onto
tty’s input queue. It returns the number of characters accepted. The characters are treated
as if they were typed from the keyboard in the TTY subwindow. ttysw_input() pro-
242 XView Programming Manual
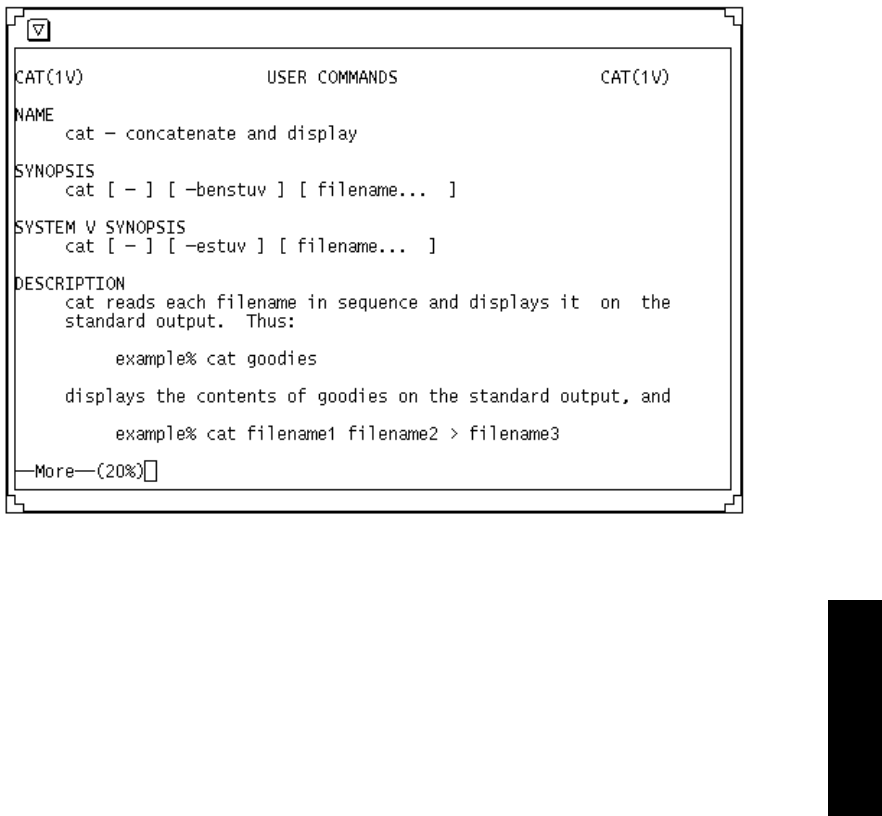
vides a simple way for a window program to send input to a program running in its TTY
subwindow. You can send output to a TTY subwindow programmatically with the function:
int
ttysw_output(tty, buf, len)
Tty tty;
char *buf;
int len;
ttysw_output() runs the character sequence in buf that is len characters long through
the terminal emulator of tty. It returns the number of characters accepted. The effect is
similar to executing this:
echo
character_sequence
> /dev/ttyN
where ttyN is the pseudo-TTY associated with the TTY subwindow. ttysw_output()
can be used to send ANSI escape sequences to the TTY subwindow.
Figure 9-2. Output of sample_tty.c
Note the differences between the input and output TTY routines. If an application is running
in the TTY subwindow, then the characters sent to the TTY subwindow using
ttysw_input() are sent to that program as its stdin. Characters sent to the TTY
subwindow using ttysw_ouput() are sent to the TTY subwindow itself and have nothing
to do with the application that might be running in the window.
TTY Subwindows
TTY Subwindows 243

The program in Example 9-2 creates a text subwindow in which the user can type input.
There is a panel button called “Text to TTY” which, if selected, reads the data in the text
subwindow and sends it to the TTY subwindow using ttysw_input().
Example 9-2. The textsw_to_ttysw.c program
/*
* textsw_to_ttysw.c -- send text from a text subwindow to a
* tty subwindow using ttysw_output()
*/
#include <stdio.h>
#include <xview/panel.h>
#include <xview/xview.h>
#include <xview/textsw.h>
#include <xview/tty.h>
Textsw textsw;
Tty ttysw;
main(argc,argv)
int argc;
char *argv[ ];
{
Frame frame;
Panel panel;
void text_to_tty(), exit();
xv_init(XV_INIT_ARGC_PTR_ARGV, &argc, argv, NULL);
frame = (Frame)xv_create(XV_NULL, FRAME,
FRAME_LABEL, argv[0 ],
NULL);
panel = (Panel)xv_create(frame, PANEL,
PANEL_LAYOUT, PANEL_VERTICAL,
NULL);
(void) xv_create(panel, PANEL_BUTTON,
PANEL_LABEL_STRING, "Quit",
PANEL_NOTIFY_PROC, exit,
NULL);
(void) xv_create(panel, PANEL_BUTTON,
PANEL_LABEL_STRING, "Text To Tty",
PANEL_NOTIFY_PROC, text_to_tty,
NULL);
window_fit(panel);
textsw = (Textsw)xv_create(frame, TEXTSW,
WIN_ROWS, 10,
WIN_COLUMNS, 80,
NULL);
ttysw = (Tty)xv_create(frame, TTY,
WIN_BELOW, textsw,
WIN_X, 0,
TTY_ARGV, TTY_ARGV_DO_NOT_FORK,
NULL);
window_fit(frame);
xv_main_loop(frame);
}
244 XView Programming Manual
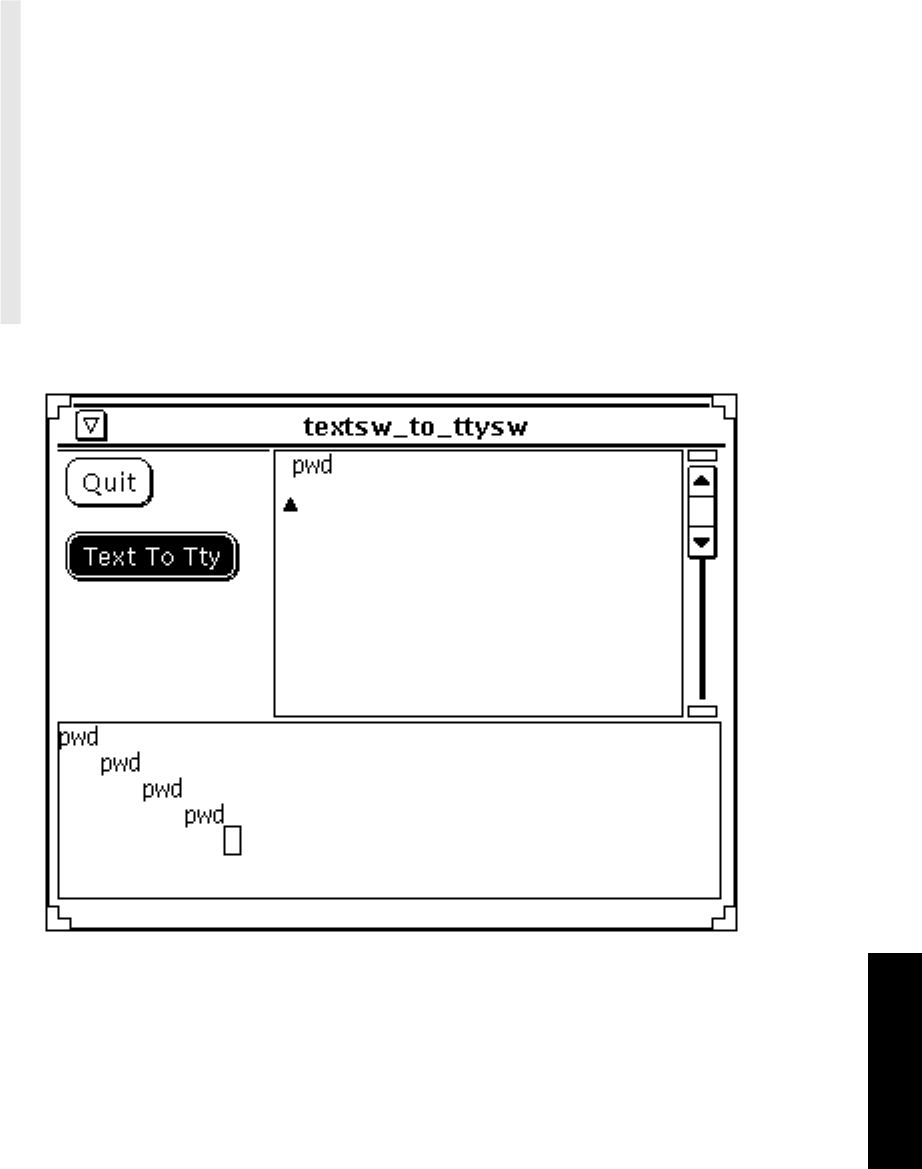
Example 9-2. The textsw_to_ttysw.c program (continued)
/*
* callback routine for the panel button -- read text from textsw
* and send it to the ttysw using ttysw_output()
*/
void
text_to_tty(item, event)
Panel_item item;
Event *event;
{
char buf[BUFSIZ];
(void) xv_get(textsw, TEXTSW_CONTENTS, 0, buf, sizeof buf);
ttysw_output(ttysw, buf, strlen(buf));
}
Figure 9-3 shows the output of Example 9-2.
Figure 9-3. Output of textsw_to_ttysw.c
TTY Subwindows
TTY Subwindows 245
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.