
Example 13-2. The hot_spot.c program (continued)
/*
* create_cursor() creates a bull’s eye cursor and assigns it
* to the window (parameter).
*/
create_cursor(window)
Xv_Window window;
{
Xv_Cursor cursor;
Server_image image;
Pixmap pixmap;
Display *dpy = (Display *)xv_get(window, XV_DISPLAY);
GC gc;
XGCValues gcvalues;
image = (Server_image)xv_create(XV_NULL, SERVER_IMAGE,
XV_WIDTH, 16,
XV_HEIGHT, 16,
NULL);
pixmap = (Pixmap)xv_get(image, XV_XID);
/* Create GC with reversed foreground and background colors to
* clear pixmap first. Use 1 and 0 because pixmap is 1-bit deep.
*/
gcvalues.foreground = 0;
gcvalues.background = 1;
gc = XCreateGC(dpy, pixmap, GCForeground|GCBackground, &gcvalues);
XFillRectangle(dpy, pixmap, gc, 0, 0, 16, 16);
/*
* Reset foreground and background values for XDrawArc() routines.
*/
gcvalues.foreground = 1;
gcvalues.background = 0;
XChangeGC(dpy, gc, GCForeground | GCBackground, &gcvalues);
XDrawArc(dpy, pixmap, gc, 2, 2, 12, 12, 0, 360 * 64);
XDrawArc(dpy, pixmap, gc, 6, 6, 4, 4, 0, 360 * 64);
/* Create cursor and assign it to the window (parameter) */
cursor = xv_create(XV_NULL, CURSOR,
CURSOR_IMAGE, image,
CURSOR_XHOT, 7,
CURSOR_YHOT, 7,
NULL);
xv_set(window, WIN_CURSOR, cursor, NULL);
/* free the GC -- the cursor and the image must not be freed. */
XFreeGC(dpy, gc);
}
When the program is running, each time the panel button is pushed, it prints the cursor’s
position relative to the panel’s window and relative to the root window (absolute screen coor-
dinates). You can move the base frame around on the screen to see how the root window
coordinates change.
332 XView Programming Manual
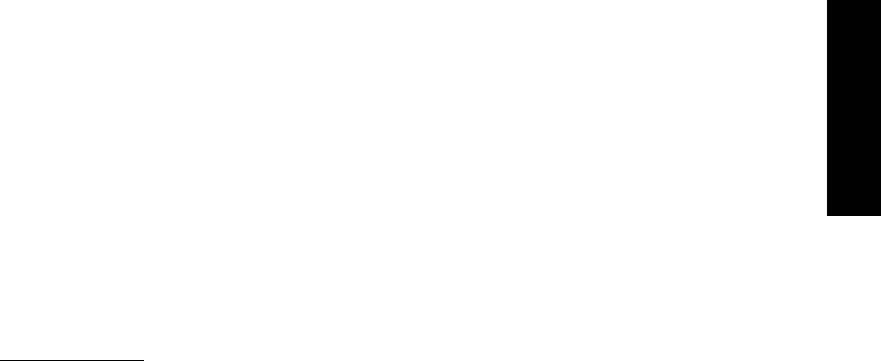
The routine create_cursor() creates a bull’s eye cursor for the window passed as the
parameter to the routine. The cursor image must be a Server_image, so we first create a
server image, then get the Pixmap associated with it using the XV_XID, and lastly use Xlib
graphics to draw two circles in the pixmap.
We need a GC, so we create one based on the pixmap obtained from the server image. The
pixmap is one-bit deep, so the foreground and background colors are set to 0, 1 (to clear the
pixmap first), then to 1, 0 so as to draw the two circles. We then create the cursor using the
server image and setting the hotspots accordingly. We free the gc, but the Server_image
(which contains the pixmap) and the cursor must not be freed so the cursor can be main-
tained by the window.
If you would rather set the cursor for a window using raw Xlib calls such as XCreatePix-
mapCursor, XCreateGlyphCursor, or XCreateFontCursor, use the X window
associated with the Xv_Window parameter. To get it, use:
xv_get(
window
, XV_XID)
and assign an X Cursor object to that window.*
13.4 Color Cursors
You can define the foreground and background colors of a cursor independently of the win-
dow the cursor is assigned to. You may not have more than two colors per cursor because X
does not support color images as cursor glyphs. Thus, to create or modify an existing cursor
to have color, you need to specify foreground and background colors. Because of the use of
color, the header file <xview/cms.h> must be included. The colors are of type
Xv_singlecolor and should be initialized before use:
#include <xview/cms.h>
...
Xv_singlecolor fg, bg;
bg.red = 250, bg.green = 230, bg.blue = 30;
fg.red = 180, fg.green = 100, fg.blue = 20;
cursor = xv_create(NULL, CURSOR,
CURSOR_IMAGE, image,
CURSOR_FOREGROUND_COLOR, &fg,
CURSOR_BACKGROUND_COLOR, &bg,
NULL);
Note, by default, a cursor is created with a mask equal to the image; therefore, there is no
background color. To use a background color, set the cursor’s CURSOR_BACKGROUND_COLOR
and the CURSOR_IMAGE attributes, and then set the CURSOR_OP as follows:
xv_set(cursor,CURSOR_OP,PIX_SRC,NULL);
*See Volume One, Xlib Programming Manual.
Cursors
Cursors 333

13.5 Support for Text Drag and Drop
The Cursor package supports drag and drop cursors for text. There are attributes that change
the cursor to indicate that text is being dragged. When the currently selected item is over an
acceptable drop site, a preview cursor is displayed. There is also an attribute to support a
reject cursor as well, but it is an unsupported attribute.
The attribute CURSOR_DRAG_STATE indicates whether the cursor is over a neutral zone
(CURSOR_NEUTRAL), a valid drop zone (CURSOR_ACCEPT), or an invalid drop zone
(CURSOR_REJECT). The shape of the cursor varies depending on the state.*
CURSOR_DRAG_TYPE changes the cursor to indicate whether a move (CURSOR_MOVE) or copy
(CURSOR_DUPLICATE) operation is being performed. The duplicate version has a shadow.
When combined with CURSOR_STRING, you get either a text move or a text duplicate cursor.
CURSOR_STRING creates a drag and drop Cursor for text. The value of the attribute is the
string which is to be displayed inside the flying punch card. If the string exceeds 3 characters,
only the first 3 characters are displayed, and an arrow is shown within the cursor.
CURSOR_STRING is mutually exclusive of CURSOR_IMAGE, CURSOR_SRC_CHAR, and
CURSOR_MASK_CHAR. The string is not copied. Once the Drag and Drop operation is com-
plete, the objects used in the operation must be destroyed.
Example 13-3 shows how to use a drag and drop text cursor with a drag and drop object.
Example 13-3. Using drag and drop text cursors
if (drag and drop started) {
type = event_ctrl_is_down(event) ? CURSOR_DUPLICATE : CURSOR_MOVE;
neutral_drag_cursor = xv_create(window, CURSOR,
CURSOR_STRING, selected_string,
CURSOR_DRAG_TYPE, type,
CURSOR_DRAG_STATE, CURSOR_NEUTRAL,
NULL);
accept_drag_cursor = xv_create(window, CURSOR,
CURSOR_STRING, selected_string,
CURSOR_DRAG_TYPE, type,
CURSOR_DRAG_STATE, CURSOR_ACCEPT,
NULL);
xv_set(dnd_object,
DND_CURSOR, neutral_drag_cursor,
DND_ACCEPT_CURSOR, accept_cursor,
NULL);
}
*Note: The current drag and drop protocol does not support a reject cursor.
334 XView Programming Manual

13.6 Cursor Package Summary
Table 13-1 shows the CURSOR package procedure. Table 13-2 lists the attributes for the
CURSOR package. This information is described fully in the XView Reference Manual.
Table 13-1. Cursor Procedure
cursor_copy()
Table 13-2. Cursor Attributes
CURSOR_BACKGROUND_COLOR CURSOR_OP
CURSOR_DRAG_STATE CURSOR_SRC_CHAR
CURSOR_DRAG_TYPE CURSOR_STRING
CURSOR_FOREGROUND_COLOR CURSOR_XHOT
CURSOR_IMAGE CURSOR_YHOT
CURSOR_MASK_CHAR
XV_SHOW XV_XID
Cursors
Cursors 335
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.