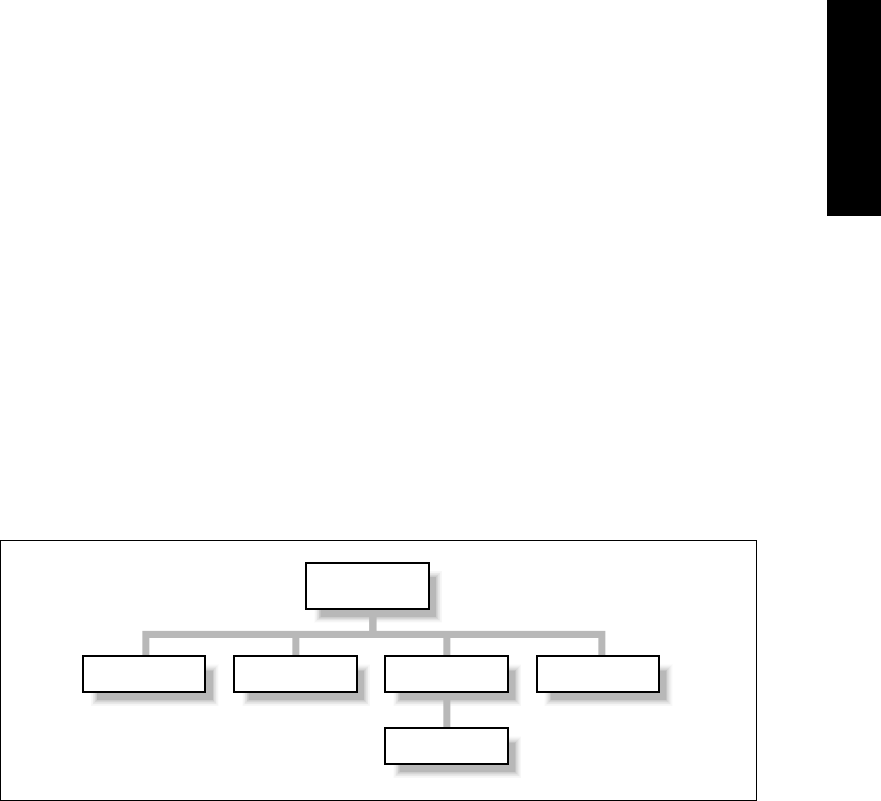
15
Nonvisual Objects
This chapter addresses nonvisual objects—objects that are not elements of the user interface.
Nonvisual objects include the screen, the display, the X11 server, and server images. The
FULLSCREEN package is used to grab the X server, and an instance of it is considered a non-
visual object. Nonvisual objects are not viewed on the screen, but they have a place in the
XView object hierarchy. Like all XView objects, they share many of the generic and com-
mon properties and can be manipulated using xv_create(), xv_set(), xv_get(), or
xv_find().
Nonvisual objects are typically used internally by XView and are seldom used directly in an
application. Therefore, this chapter contains advanced material that may not be essential to
all programmers. Figure 15-1 shows the class hierarchy for nonvisual objects.
Generic
Object
Server Screen (Drawable) Fullscreen
Server Image
Figure 15-1. Nonvisual objects class hierarchy
Nonvisual Objects
Nonvisual Objects 349
15.1 The Display
There is no XView Display object. If you need the Display data structure as defined by X,
you can get the value of the attribute XV_DISPLAY. This can be used on virtually any visible
XView object except for panel items. For example, to get the display associated with the
Frame, use:
Display *dpy;
dpy = (Display *)xv_get(frame, XV_DISPLAY);
The object does not have to be displayed or visible—just created. To do this, the header file
<X11/Xlib.h> must be included for the declaration of the Display data structure. This
structure contains a great deal of information that describes attributes of the workstation, the
server being used, and more. See Volume One, Xlib Programming Manual, and Volume
Two, Xlib Reference Manual, for more information.
15.2 The Screen Object
An Xv_Screen is associated with virtually all XView objects. To use the Xv_Screen
object, you must include the file <xview/screen.h>. To get a handle on the current screen, use
xv_get() on an object:
Xv_Screen xv_screen;
xv_screen = (Xv_Screen)xv_get(frame, XV_SCREEN);
The Xv_Screen object carries useful information such as the screen number of the root
window, all the visuals, the colormap, the server, and so on, that are associated with that
screen.
The Xv_Screen object differs from the Screen data structure defined by Xlib and, in fact,
has nothing to do with the X11 Screen data type (defined in <X11/Xlib.h>). That is, you
cannot use the following call to xv_get() to get a corresponding Screen pointer.
xv_get(xv_screen, XV_XID) /* Doesn’t work */
There is no associated XID for the SCREEN package.
Because the X Screen type provides information not available from the Xv_Screen
object, it may be useful to get the X Screen. Once the XView screen is obtained, the X
Screen type can be gotten by using the
SCREEN_NUMBER of the screen and the Display
pointer associated with an arbitrary visual object. Example 15-1 demonstrates how to do this
for a frame.
350 XView Programming Manual
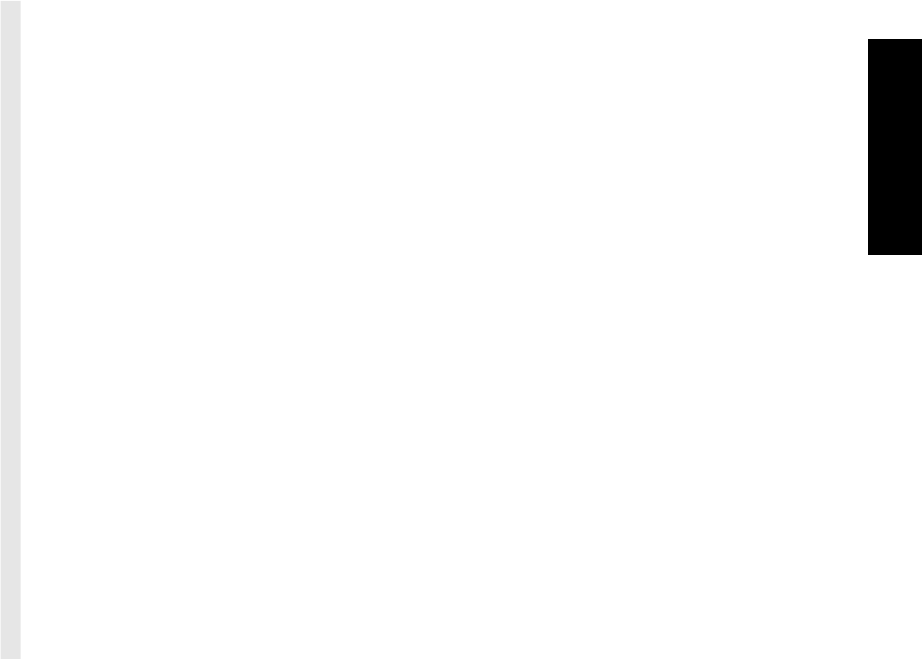
Example 15-1. Getting a pointer for a particular frame object (screen.c)
/*
* screen.c -- get some simple info about the current screen:
* width, height, depth.
*/
#include <xview/xview.h>
#include <xview/screen.h>
main(argc, argv)
int argc;
char *argv[ ];
{
Frame frame;
Xv_Screen screen;
Display *dpy;
int screen_no;
xv_init(XV_INIT_ARGC_PTR_ARGV, &argc, argv, NULL);
frame = (Frame)xv_create(XV_NULL, FRAME, NULL);
dpy = (Display *)xv_get(frame, XV_DISPLAY);
printf("Server display = ’%s’0, dpy–>vendor);
screen = (Xv_Screen)xv_get(frame, XV_SCREEN);
screen_no = (int)xv_get(screen, SCREEN_NUMBER);
printf("Screen #%d: width: %d, height: %d, depth: %d0,
screen_no,
DisplayWidth(dpy, screen_no),
DisplayHeight(dpy, screen_no),
DefaultDepth(dpy, screen_no));
}
As shown in Example 15-1, you can use any of the macros defined in <X11/Xlib.h> to get
information about the default screen such as the width, height, depth, and so on. From this
information, you can get information about the physical frame buffer. These are Xlib-related
issues not covered in this book.
15.2.1 Multiple Screens
Each X11 server supports multiple screens. Each screen can have different attributes such as
colormaps, depth, size and so on. The screens can actually be different physical devices,
although they are connected to the same physical computer. Each screen has its own root
window as well, and since the root window is the parent for all base frames, XView can
allow windows to exist on any screen.
The way we take advantage of this capability is to first establish a connection to the X11
server and then to get the root window of each screen. With a handle to the root window, we
can use it as the parent to any frame we create.
Example 15-2 demonstrates how two frames can be created on two different screens attached
to a single server. Note that this code relies on the fact that the server supports more than one
screen.
Nonvisual Objects
Nonvisual Objects 351

Example 15-2. Display a base frame on two screens
/*
* multiscreen.c -- display a base frame on two different screens
* attached to the same X11 server. In order for this program to
* work, you must have two screens.
*/
#include <xview/xview.h>
main(argc,argv)
int argc;
char *argv[ ];
{
Xv_Server server;
Xv_Screen screen_0, screen_1;
Xv_Window root_0, root_1;
Frame frame_0, frame_1;
server = xv_init(XV_INIT_ARGC_PTR_ARGV, &argc, argv, 0);
screen_0 = (Xv_Screen) xv_get(server, SERVER_NTH_SCREEN, 0);
root_0 = (Xv_Window) xv_get(screen_0, XV_ROOT);
screen_1 = (Xv_Screen) xv_get(server, SERVER_NTH_SCREEN, 1);
root_1 = (Xv_Window) xv_get(screen_1, XV_ROOT);
frame_0 = (Frame) xv_create(root_0, FRAME,
FRAME_LABEL, "SCREEN 0",
NULL);
frame_1 = (Frame) xv_create(root_1, FRAME,
FRAME_LABEL, "SCREEN 1",
NULL);
xv_set(frame_1, XV_SHOW, TRUE, NULL);
xv_main_loop(frame_0);
}
The program implements the design discussed above: xv_init() opens a connection to the
server and returns a handle to the Xv_Server object (see the following section for details).
It also retrieves the Xv_Screen object as well as the root window for each screen. Next, a
base frame is created for each root window. However, since we are going to call
xv_main_loop() on frame_0, we need to insert frame_1 into the window tree. Other-
wise, it will never be mapped to its screen because xv_main_loop() only installs and
maps the window of the object passed to it.
352 XView Programming Manual

15.3 The SERVER Package
The SERVER package may be used to initialize the connection with the X server running on
any workstation on the network. Once the connection has been made, the package allows
you to query the server for information. xv_init(), the routine that initializes the XView
Toolkit, opens a connection to the server and returns a handle to an Xv_Server object.
While more than one server can be created, xv_init() only establishes a connection to
one server. The server object returned by xv_init() is also the server pointed to by the
external global variable, xv_default_server. Programs that do not save the
Xv_Server object returned by xv_init() can reference this global variable instead.
Subsequent connections to other X11 servers must be made using separate calls to xv_cre-
ate(). Note that using separate screens is not the same as establishing a connection to
other servers—the same server can support multiple screens. See the previous section for
ways to access multiple screens in a server.
15.3.1 Creating a Server (Establishing a Connection)
When making any reference to Xv_Server objects, applications should include
<xview/server.h>. You can open a connection to any server by using xv_create():
Xv_Server server;
extern char *server_name;
server = (Xv_Server)xv_create(NULL, SERVER,
XV_NAME, server_name,
NULL);
Because there is no owner for a server, the owner parameter is ignored and you may pass
NULL. The server described by server_name is assumed to have been initialized already.
It should be set to the standard format:
hostname:display.screen
For example:
zipcode:0.1
connects the second screen on the first display to the host named zipcode. If the connection
fails, NULL is returned.
Remember that the user can specify which display is the default by using the -display option:
% program_name –display zipcode:0
Remember that this command-line switch is parsed internally by XView when you call
xv_init() in the following way:
xv_init(XV_INIT_ARGC_PTR_ARGV, &argc, argv, NULL);
If xv_init() has not been called by the time the first call to xv_create() is called, the
call to xv_create() calls xv_init() internally. This means that if the program gets
around to calling xv_init() after it has made any calls to xv_create(), it is a no-op.
Nonvisual Objects
Nonvisual Objects 353
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.