
Generic
Object
Selection
Owner
(Selection)
Figure 18-2. Selection owner class hierarchy
If a selection uses a conversion procedure to convert the selection, then the attribute
SEL_CONVERT_PROC specifies the name of the conversion procedure. Section 18.2.4, “Con-
verting the Selection,” describes the conversion procedure. The selection is acquired by set-
ting SEL_OWN to TRUE.
xv_set(sel_owner, SEL_CONVERT_PROC, convert_proc,
SEL_OWN, TRUE,
NULL);
By default, the selection is associated with the primary rank (XA_PRIMARY). If your selection
needs to use another rank, use either SEL_RANK or SEL_RANK_NAME to specify the rank.
xv_set(sel_owner, SEL_CONVERT_PROC, convert_proc,
SEL_RANK_NAME, "SECONDARY",
SEL_OWN, TRUE,
NULL);
SEL_RANK
takes an atom as an argument. SEL_RANK_NAME takes a string as an argument and
creates an atom from the string; if the atom already exists, it is re-used. In X terms, this is
called interning an atom. (See the description for XInternAtom in the Xlib Reference
Manual.)
After an application makes a request and a selection owner responds to that request, the
selection owner waits for notification that the requestor received the data. The maximum
time that the selection owner will wait for an acknowledgement from the selection requestor
is set with SEL_TIMEOUT_VALUE. This value is specified in seconds. If this value is
exceeded, the selection is invalid. This value does not limit how long a selection owner may
hold a selection. A selection owner may hold a selection for any length of time, unless
another owner acquires the same selection, in which case the owner loses the selection own-
ership.
Note that the attributes SEL_RANK, SEL_RANK_NAME, and SEL_TIMEOUT_VALUE are
SELECTION attributes. They apply to both the selection owner and to the selection requestor.
When and if a selection owner receives a selection request, the selection data needs to be
converted. To convert a selection an application can create a selection-item object, or it can
define a selection conversion procedure. We cover these possibilities in later sections.
398 XView Programming Manual
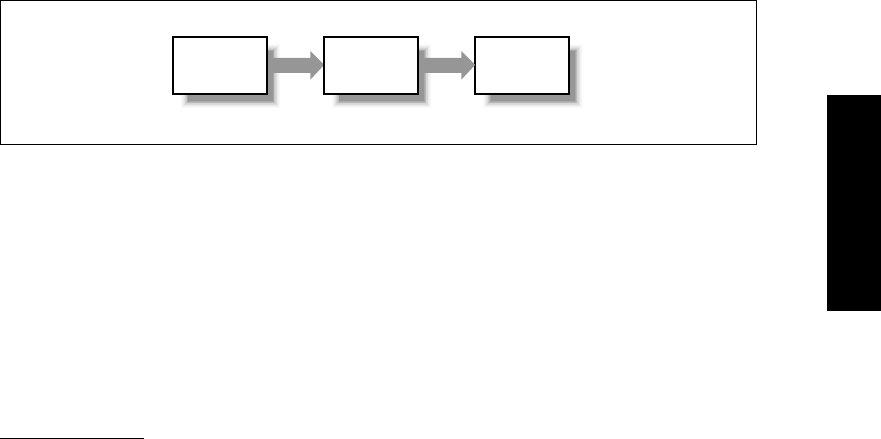
18.2.3 Requesting the Selection (Selection Requestor)
When the user pastes the selection into an application, the ACTION_PASTE event should be
used to trigger the application to become the selection requestor. A selection requestor
requests to receive the selected data. The selection requestor allows an application to obtain
selection data in a specified form. XView permits the selection requestor to receive a
selection in one of two ways:
• A blocking request. The application is blocked until the transfer completes, either suc-
cessfully or unsuccessfully.
• A non-blocking request. The application is not blocked during the selection data transfer.
This section covers both blocking and non-blocking requests.
An application requests a selection from a selection owner by performing two steps: first, the
application creates a selection requestor object and sets its attributes. Second, the request is
“posted.” Posting the request sends a request to the selection owner.
Create a selection requestor from the SELECTION_REQUESTOR package:
Selection_requestor sel_requestor;
sel_requestor = xv_create(window, SELECTION_REQUESTOR,
NULL);
The SELECTION_REQUESTOR package is defined in the header file <xview/sel_pkg.h>. The
owner of a selection requestor object is a window-based object. The class hierarchy for a
selection requestor is shown in Figure 18-3.
Generic
Object
Selection
Requestor
(Selection)
Figure 18-3. Selection requestor class hierarchy
The primary rank is the default rank used in a selection request. To use a rank other than the
primary, use either SEL_RANK or SEL_RANK_NAME to specify the desired rank.
The time of the event that triggered selection request, the ACTION_PASTE event, should be
set using the attribute SEL_TIME. If you do not set this attribute, the selection package does
its best to set the SEL_TIME internally; however, this requires additional processing that is
not required if the application sets SEL_TIME.*
*SEL_TIME fixes certain race conditions that would occur if the time of the events associated with selection the
were not known.
Selections
Selections 399
18.2.3.1 Specifying the target type (selection requestor)
By default, a selection requestor uses the primary rank with the target set to string, which
asks the selection owner to convert string data. When the requestor wants the owner to send
data, it informs the owner of the type of information it expects using a selection target. The
target may represent the type of the selection data; however, the selection transfer may not
actually involve the selection data. A selection requestor may simply request a timestamp
from the selection owner, or some other characteristic of the selection data, such as its length.
The selection requestor can also request that the selection owner send a list of the types it can
convert. When the requestor specifies the target named TARGETS, the owner should return a
list of the types that it is able to convert to.
The attribute SEL_TYPE specifies the atom name for the requested target. The default value
is XA_STRING. This may be set to XA_INTEGER, or to another atom. SEL_TYPE_NAME uses a
string argument for the type, such as “INTEGER,” internally, the package converts this string
to an atom.
A single selection request may be used to request more than one target. For example, the
selection requestor may desire a list of the possible types the owner can convert as well as
selection data. This type of a request, where more than one target is requested at a time, is a
MULTIPLE request. To initiate a multiple request, use the attribute
SEL_TYPES with a NULL-
terminated list of atoms to specify the targets requested, or use SEL_TYPE_NAMES with a
NULL-terminated list of strings.
Two additional attributes allow the requestor to make a multiple request.
SEL_APPEND_TYPES appends a NULL-terminated list of target types on to the existing list.
SEL_APPEND_TYPE_NAMES appends a NULL-terminated list of names on to the current list.
By default, a selection requestor selects a string, so the following request adds TARGETS to
the request list, thus creating a multiple request:
xv_set(sel, SEL_APPEND_TYPE_NAMES, "TARGETS", NULL, NULL);
18.2.3.2 SEL_REPLY_PROC (selection requestor)
The selection requestor uses
SEL_REPLY_PROC to specify the reply procedure. This attribute
takes a function pointer as an argument. The reply procedure is used as a communication
mechanism between the selection owner and the selection requestor. The reply procedure is
invoked by the selection package after the conversion, when the response from the selection
owner arrives.
If the request is a multiple request, the reply procedure is called once for each target
requested:
Selection_requestor sel_requestor;
sel_requestor = xv_create(window, SELECTION_REQUESTOR,
SEL_REPLY_PROC, ReplyProc,
NULL);
The reply procedure is described in Section 18.2.5, “Handling the Response.”
400 XView Programming Manual

18.2.3.3 Timeout for a selection response
The attribute SEL_TIMEOUT_VALUE specifies the maximum time that the selection requestor
expects the particular request conversion to take. This should be set to the time selection
owner will take to convert the selection and return data to the selection requestor.
18.2.3.4 Requesting the CLIPBOARD selection—blocking
To make a blocking request, the selection requestor uses the SEL_DATA attribute to initiate
the request. Note that a blocking request puts the entire application on hold during the
selection transfer. This type of selection transfer is not recommended for large selections,
since the application may be put on hold for a significant amount of time. SEL_DATA takes
two arguments, a pointer to an unsigned long which is set to the number of elements
returned in the selection buffer, and a pointer to an integer which is set to the data format.
Using xv_get() with the selection requestor and SEL_DATA, with the specified arguments
returns the selection data. Example 18-1 shows how the selection requestor requests the clip-
board selection using a blocking request.
Example 18-1. Requesting the
CLIPBOARD
selection--blocking
Selection_requestor sel_requestor;
main()
{
.
.
.
sel_requestor = xv_create(window, SELECTION_REQUESTOR,
SEL_RANK_NAME "CLIPBOARD", NULL);
.
.
.
}
window_event_handler(..., event, ...)
Event *event;
{
char *data;
int format; /* size of data element: 8,16, 32 bits.*/
unsigned long length; /* number of data elements */
switch (event_action(event) {
case ACTION_PASTE:
/* Initiate a blocking request, and get the data */
data = (char *) xv_get(sel_requestor,
SEL_DATA, &length, &format);
/* returns with length set to SEL_ERROR */
/* if the if request fails. */
break;
}
}
Selections
Selections 401

Above, the attribute SEL_DATA with xv_get() sends the selection request to the selection
owner. The returned data is placed in data. If the conversion was successful, the application
needs to free the returned data, data when it is finished using it.
18.2.3.5 Requesting the CLIPBOARD selection—non-blocking
If a selection requestor wants to make a non-blocking request, it calls the procedure
sel_post_req() which is defined by the selection package. This procedure sends a non-
blocking request to the selection owner.
The procedure sel_post_req() has the following format:
int
sel_post_req( sel_req )
Selection_requestor sel_req;
The procedure sel_post_req() returns XV_OK or XV_ERROR. If no reply procedure is
defined, XV_ERROR is returned. Example 18-2 shows how the selection requestor requests the
clipboard selection using a non-blocking request.
Example 18-2. Non-blocking selection request
long *file_buffer; /* contents of selected file */
long file_buffer_size; /* # of longs in file_buffer */
Selection_requestor sel_requestor;
main()
{
.
.
.
sel_requestor = xv_create(window, SELECTION_REQUESTOR,
SEL_REPLY_PROC, ReplyProc,
SEL_RANK_NAME, "CLIPBOARD",
NULL);
}
window_event_handler(..., event, ...)
Event *event;
{
switch (event_action(event) {
case ACTION_PASTE:
/* initiate non-blocking request */
sel_post_req(sel_requestor);
break;
}
}
402 XView Programming Manual
Get Volume 7A: XView Programming Manual now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.