Getting Started
1.0 Introduction
The Arduino environment has been designed to be easy to use for beginners who have no software or electronics experience. With Arduino, you can build objects that can respond to and/or control light, sound, touch, and movement. Arduino has been used to create an amazing variety of things, including musical instruments, robots, light sculptures, games, interactive furniture, and even interactive clothing.
Arduino is used in many educational programs around the world, particularly by designers and artists who want to easily create prototypes but do not need a deep understanding of the technical details behind their creations. Because it is designed to be used by nontechnical people, the software includes plenty of example code to demonstrate how to use the Arduino board’s various facilities.
Though it is easy to use, Arduino’s underlying hardware works at the same level of sophistication that engineers employ to build embedded devices. People already working with microcontrollers are also attracted to Arduino because of its agile development capabilities and its facility for quick implementation of ideas.
Arduino is best known for its hardware, but you also need software to program that hardware. Both the hardware and the software are called “Arduino.” The hardware (Arduino and Arduino-compatible boards) is inexpensive to buy, or you can build your own (the hardware designs are open source). The software is free, open source, and cross-platform. The combination enables you to create projects that sense and control the physical world.
In addition, there is an active and supportive Arduino community that is accessible worldwide through the Arduino forums, tutorials, and project hub. These sites offer learning resources, project development examples, and solutions to problems that can provide inspiration and assistance as you pursue your own projects.
Arduino Software and Sketches
Software programs, called sketches, are created on a computer using the Arduino integrated development environment (IDE). The IDE enables you to write and edit code and convert this code into instructions that Arduino hardware understands. The IDE also transfers those instructions, in the form of compiled code, to the Arduino board (a process called uploading).
Tip
You may be used to referring to software source code as a “program” or just “code.” In the Arduino community, source code that contains computer instructions for controlling Arduino functionality is referred to as a sketch. The word sketch will be used throughout this book to refer to Arduino program code.
The recipes in this chapter will get you started by explaining how to set up the development environment and how to compile and run an example sketch.
The Blink sketch, which is preinstalled on most Arduino boards and compatibles, is used as an example for recipes in this chapter, though the last recipe in the chapter goes further by adding sound and collecting input through some additional hardware, not just blinking the light built into the board. Chapter 2 covers how to structure a sketch for Arduino and provides an introduction to programming.
Note
If you already know your way around Arduino basics, feel free to jump forward to later chapters. If you’re a first-time Arduino user, patience in these early recipes will pay off with smoother results later.
Arduino Hardware
The Arduino board is where the code you write is executed. The board can only control and respond to electricity, so you’ll attach specific components to it that enable it to interact with the real world. These components can be sensors, which convert some aspect of the physical world to electricity so that the board can sense it, or actuators, which get electricity from the board and convert it into something that changes the world. Examples of sensors include switches, accelerometers, and ultrasonic distance sensors. Actuators are things like lights and LEDs, speakers, motors, and displays.
There are a variety of official boards that you can use with Arduino software and a wide range of Arduino-compatible boards produced by companies and individual members of the community. In addition to all the boards on the market, you’ll even find Arduino-compatible controllers inside everything from 3D printers to robots. Some of these Arduino-compatible boards and products are also compatible with other programming environments such as MicroPython or CircuitPython.
The most popular boards contain a USB connector that is used to provide power and connectivity for uploading your software onto the board. Figure 1-1 shows a basic board that most people start with, the Arduino Uno. It is powered by an 8-bit processor, the ATmega328P, which has 2 kilobytes of SRAM (static random-access memory, used to store program variables), 32 kilobytes of flash memory for storing your sketches, and runs at 16 MHz. A second chip handles USB connectivity.
Note
Despite having the word “static” in its name, static RAM is a form of volatile memory. This is in contrast to dynamic RAM (DRAM), which needs to be occasionally refreshed to maintain an electrical charge. SRAM holds its state as long as power is maintained without needing additional circuitry to refresh it.
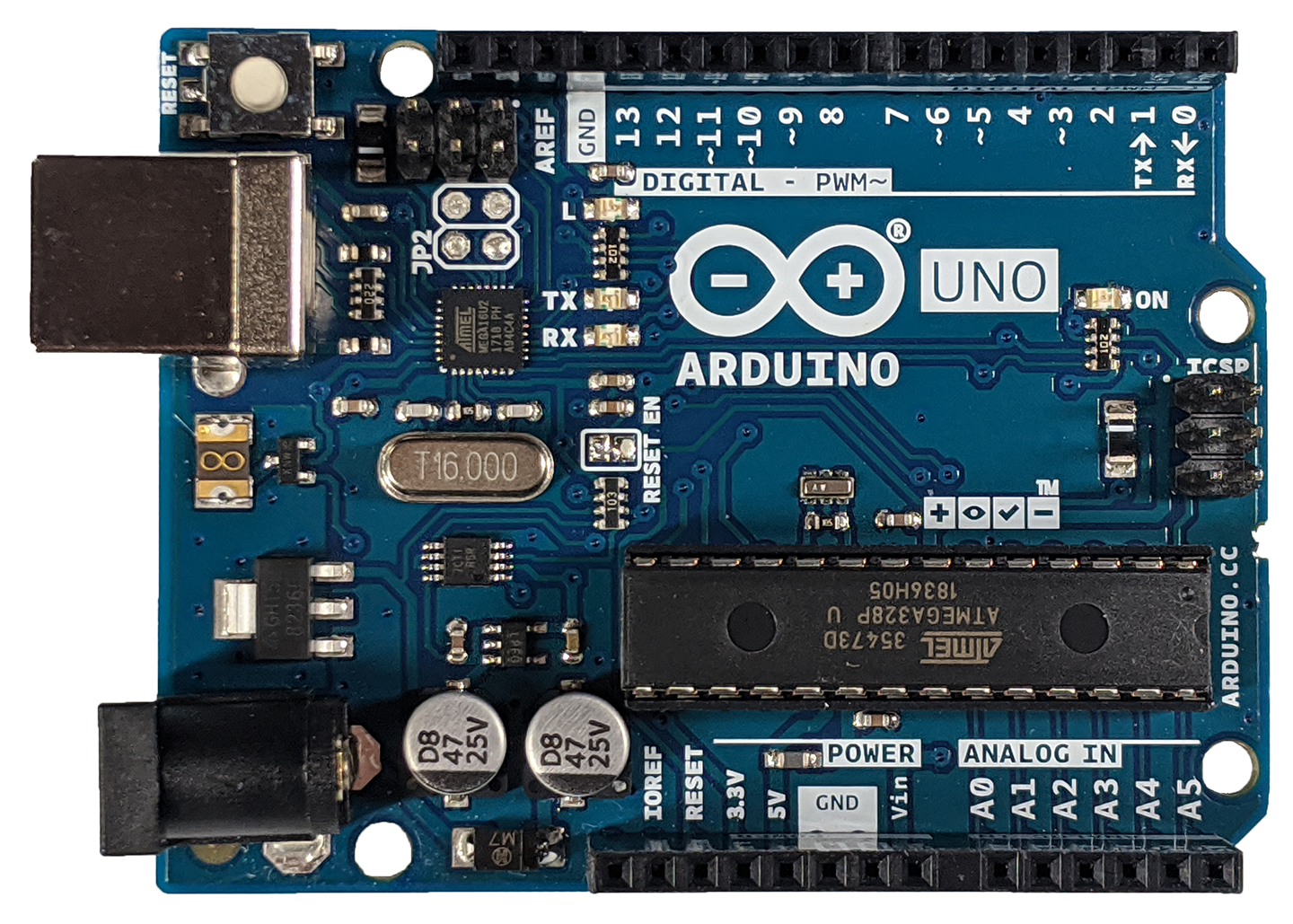
Basic board: the Arduino Uno
The Arduino Leonardo board uses the same form factor (the layout of the board and its connector pins) as the Uno, but uses a different processor, the ATmega32U4, which runs your sketches and also takes care of USB connectivity. It is slightly cheaper than the Uno, and also offers some interesting features, such as the ability to emulate various USB devices such as mice and keyboards. The Arduino-compatible Teensy and Teensy++ boards from PJRC (http://www.pjrc.com/teensy) are also capable of emulating USB devices.
Another board with a similar pin layout and even faster processor is the Arduino Zero. Unlike the Arduino Uno and Leonardo, it cannot tolerate input pin voltages higher than 3.3 volts. The Arduino Zero has a 32-bit processor running at 48 MHz and has 32 kilobytes of RAM and 256 kilobytes of flash storage. Adafruit’s Metro M0 Express and SparkFun’s RedBoard Turbo come in the same form factor as the Arduino Zero and also offer compatibility with multiple environments, including the Arduino IDE and CircuitPython.
If you want a board for learning that will run the majority of sketches in this book, the Uno is a great choice. If you want more performance than the Uno, but still want to use the Uno form factor, then consider the Zero, or a similar board such as the Metro M0 Express or RedBoard Turbo. The MKR and Nano 33 series of boards also offer excellent performance, but in a smaller form factor than the Uno.
Caution Needed with Some 3.3-Volt Boards
Many of the newer boards operate on 3.3 volts rather than the 5 volts used by older boards such as the Uno. Some such boards can be permanently damaged if an input or output pin receives 5 volts, even for a fraction of a second, so check the documentation for your board to see if it is tolerant of 5 volts before wiring things up when there is a risk of pin levels higher than 3.3 volts. Most 3.3-volt boards are powered by a 5-volt power supply (for example, through the USB port), but a voltage regulator converts it to 3.3 volts before it reaches the board’s 3.3-volt electronics. This means that it is not unusual to see a 5-volt power supply pin on a board whose input and output pins are not 5-volt tolerant.
Arduino boards come in other form factors, which means that the pins on such boards have a different layout and aren’t compatible with shields designed for the Uno. The MKR1010 is an Arduino board that uses a much smaller form factor. Its pins are designed for 3.3V I/O (it is not 5V tolerant) and like the Zero, it uses an ARM chip. However, the MKR1010 also includes WiFi and and a circuit to run from and recharge a LIPO battery. Although the MKR family of boards is not compatible with shields designed for the Uno, Arduino offers a selection of add-on boards for the MKR form factor called carriers.
You can get boards as small as a postage stamp, such as the Adafruit Trinket M0; larger boards that have more connection options and more powerful processors, such as the Arduino Mega and Arduino Due; and boards tailored for specific applications, such as the Arduino LilyPad for wearable applications, the Arduino Nano 33 IoT for wireless projects, and the Arduino Nano Every for embedded applications (standalone projects that are often battery-operated).
Other third-party Arduino-compatible boards are also available, including the following:
- Bare Bones Board (BBB)
- Low-cost Bare Bones Boards are available with or without USB capability from Modern Device, and from Educato in a shield-compatible version.
- Adafruit Industries
- Adafruit has a vast collection of Arduino and Arduino-compatible boards and accessories (modules and components).
- SparkFun
- SparkFun has lots of Arduino and Arduino-compatible accessories.
- Seeed Studio
- Seeed Studio sells Arduino and Arduino-compatible boards as well as many accessories. It also offers a flexible expansion system for Arduino and other embedded boards called Grove, which uses a modular connector system for sensors and actuators.
- Teensy and Teensy++
- These tiny but extremely versatile boards are available from PJRC.
Wikipedia has an exhaustive list of Arduino-compatible boards. You can also find an overview of Arduino boards on the Arduino site.
1.1 Installing the Integrated Development Environment (IDE)
Solution
Download the Arduino software for Windows, MacOS, or Linux. Here are notes on installing the software on these platforms:
- Windows
-
If you are running Windows 10, you can use the Microsoft Store to install Arduino without needing admin privileges. But for earlier versions of Windows you’ll need admin privileges to double-click and run Windows installer when it’s downloaded. Alternatively, you can download the Windows ZIP file, and unzip it to any convenient directory that you have write access to.
Unzipping the file will create a folder named Arduino-<nn> (where <nn> is the version number of the Arduino release you downloaded). The directory contains the executable file (named Arduino.exe), along with other files and folders.
Note
The first time you run Arduino on Windows, you may see a dialog that says “Windows Defender Firewall has blocked some features of this app,” specifying
javaw.exe
as the source of the warning. The Arduino IDE is a Java-based application, which is why the warning comes from the Java program instead of Arduino.exe. This is used by boards that support the ability to upload sketches over a local network. If you plan to use this kind of board, you should use this dialog to permit javaw.exe access to the network.When you plug in the board, it automatically associates the installed driver with the board (this may take a little time). If this process fails, or you installed Arduino using the ZIP file, then go to the Arduino Guide page, click the link for your board from the list there, and follow the instructions.
If you are using an earlier board (any board that uses FTDI drivers) and are online, you can let Windows search for drivers and they will install automatically. If you don’t have internet access, or are using Windows XP, you should specify the location of the drivers. Use the file selector to navigate to the drivers\FTDI USB Drivers directory, located in the directory where you unzipped the Arduino files. When this driver has installed, the Found New Hardware Wizard will reappear, saying a new serial port has been found. Follow the same process again.
Tip
You may need to go through the sequence of steps to install the drivers twice to ensure that the software is able to communicate with the board.
- macOS
-
The Arduino download for the Mac is a ZIP file. If the ZIP file does not extract automatically after you download it, locate the download and double-click it to extract the application. Move the application to somewhere convenient—the Applications folder is a sensible place. Double-click the application. The splash screen will appear, followed by the main program window.
Current Arduino boards such as the Uno can be used without additional drivers. When you first plug the board in, a notification may pop up saying a new network port has been found; you can dismiss this. If you are using earlier boards that need FTDI drivers, you can get these from FTDI.
- Linux
-
Linux versions are increasingly available from your distribution’s package manager, but these versions are often not the most current release, so it is best to download the version from http://arduino.cc/download. It is available for 32 or 64 bit, and offers an ARM version that can be used on the Raspberry Pi and other Linux ARM boards. Most distributions use a standard driver that is already installed, and usually have FTDI support as well. See this Arduino Linux page for instructions on installing Arduino on Linux. You will need to follow those instructions to run the install script, and you may need to do so to permit your user account to access the serial port.
For Arduino-compatible boards that is not made by Arduino, you may need to install support files using the Boards Manager (see Recipe 1.7). You should also check the specific board’s documentation for any additional steps needed.
After you’ve installed Arduino, double-click its icon, and the splash screen should appear (see Figure 1-2).
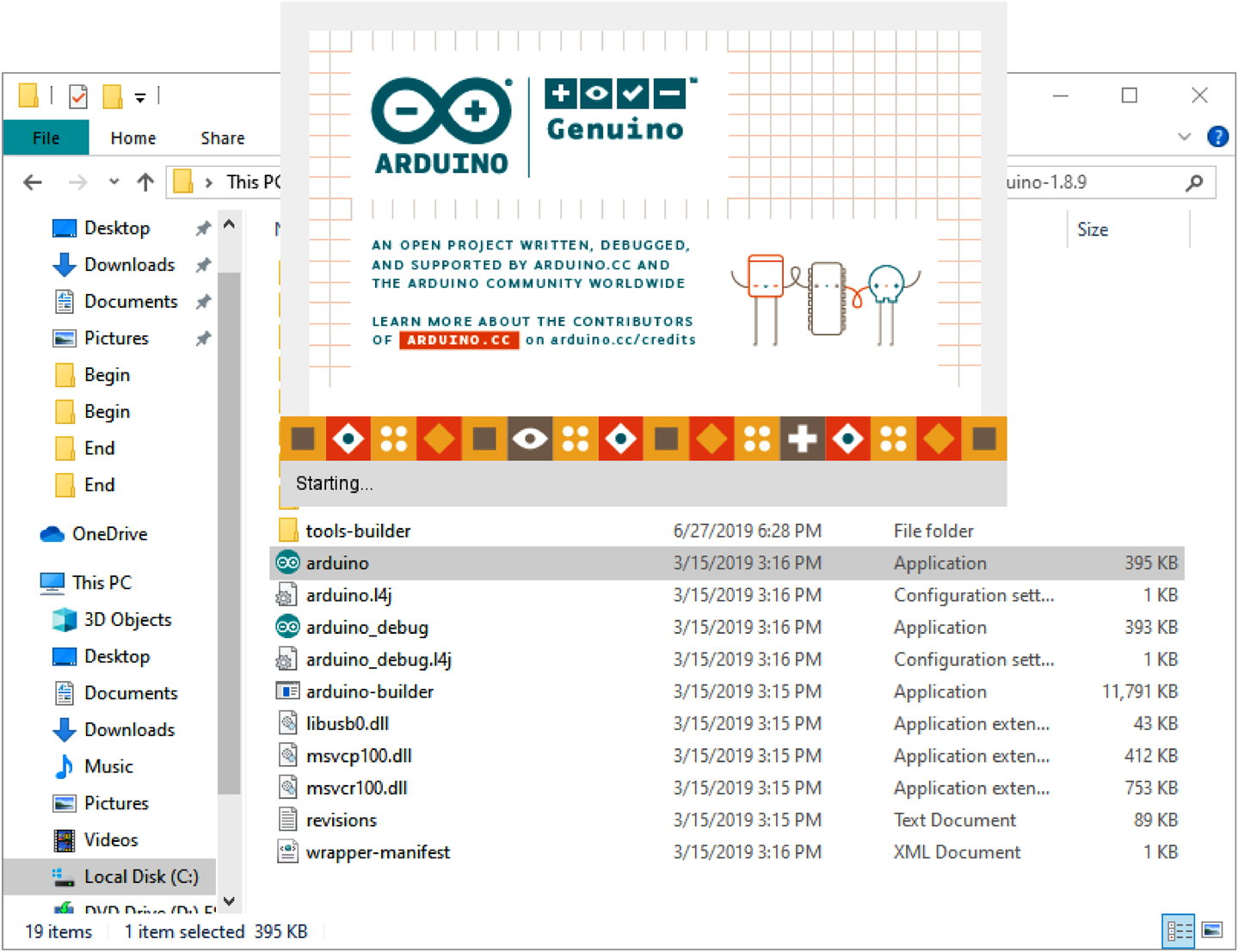
Arduino splash screen (Arduino 1.8.9 on Windows 10)
The initial splash screen is followed by the main program window (see Figure 1-3). Be patient, as it can take some time for the software to load. You can find the icon for Arduino on the Start menu (Windows), the Applications folder (macOS), or possibly on the desktop. On Linux, you may need to run the Arduino executable from the Terminal shell.

IDE main window (Arduino 1.8.9 on a Mac)
Discussion
If the software fails to start, check the troubleshooting section of the Arduino website for help solving installation problems.
See Also
Online guides for getting started with Arduino are available at for Windows, for macOS, and for Linux.
The Arduino Pro IDE is a development environment for Arduino that’s aimed at the needs of professional users. At the time of this writing, it was in an early state. See the Arduino Pro IDE GitHub repo.
The Arduino CLI is a command-line tool for compiling and uploading sketches. You can also use it in place of the Library and Boards Manager. See the Arduino CLI GitHub repo.
There is an online editing environment called Arduino Create. In order to use this you will need to create an account and download a plug-in that enables the website to communicate with the board to upload code. It has cloud storage where your sketches are saved and provides facilities for sharing code. At the time this book was written, Arduino Create was a fairly new, still-evolving service. If you would like the ability to create Arduino sketches without having to install a development environment on your computer, then have a look at Arduino Create.
If you are using a Chromebook, Arduino Create’s Chrome App requires a monthly subscription of US$1. It has a time-limited trial so you can try it out. There is another alternative to compiling and uploading Arduino code from a Chromebook: Codebender is a web-based IDE like Arduino Create, but it also supports a number of third-party Arduino-compatible boards. Pricing plans are available for classrooms and schools as well. See this Codebender page.
1.2 Setting Up the Arduino Board
Solution
Plug the board into a USB port on your computer and check that the LED power indicator on the board illuminates. Most Arduino boards have an LED power indicator that stays on whenever the board is powered.
The onboard LED (labeled in Figure 1-4) should flash on and off when the board is powered up (most boards come from the factory preloaded with software to flash the LED as a simple check that the board is working).
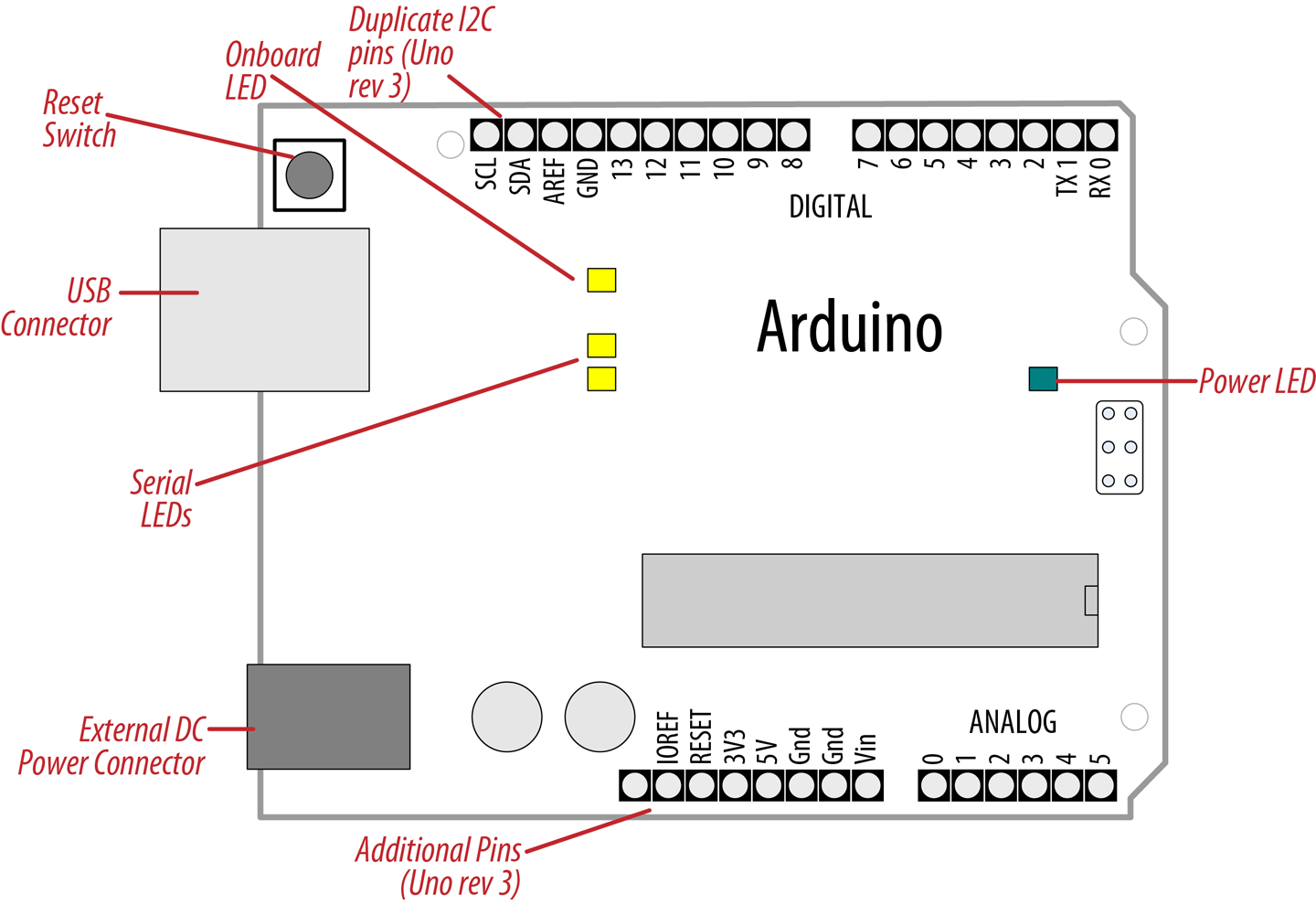
Basic Arduino board, the Uno Rev3
Note
The current boards in the Arduino Uno form factor have some pins that weren’t present on older boards, and you may encounter some older Arduino shields that don’t have these pins. Fortunately, this usually does not affect the use of older shields; most will continue to work with the new boards, just as they did with earlier boards (but your mileage may vary).
The new connections provide a pin (IOREF) for shields to detect the analog reference voltage (so that analog input values can be correlated with the supply voltage), and SCL and SDA pins to enable a consistent pin location for I2C devices. The location of the I2C pins had varied on some earlier boards such as the Mega due to different chip configurations, and in some cases certain shields required workarounds such as the addition of jumper wires to connect the shield’s I2C pins to the ones on the Mega. Shields designed for the new layout should work on any board with the new pin locations. An additional pin (next to the IOREF pin) is unused at the moment, but enables new functionality to be implemented in the future without needing to change the pin layout again.
Discussion
If the power LED does not illuminate when the board is connected to your computer, the board is probably not receiving power (try a different USB socket or cable).
The flashing LED is being controlled by code running on the board (new boards are preloaded with the Blink example sketch). If the onboard LED is flashing, the sketch is running correctly, which means the chip on the board is working. If the power LED is on but the onboard LED (usually labeled L) is not flashing, it could be that the factory code is not on the chip; follow the instructions in Recipe 1.3 to load the Blink sketch onto the board to verify that the board is working. If you are not using a standard board, it may not have an onboard LED, so check the documentation for details of your board.
The Leonardo and Zero-class boards (Arduino Zero, Adafruit Metro M0, SparkFun RedBoard Turbo) have the same footprint as the Uno (its headers are in the same position, enabling shields to be attached). They are significantly different in other respects. The Leonardo has an 8-bit chip like the Uno, but because it doesn’t have a separate chip for handling USB communications, the Leonardo only accepts program uploads immediately after the board has been reset. You’ll see the Leonardo’s onboard LED pulse gently while it’s waiting for an upload. The Leonardo is 5V tolerant. The Zero has a 32-bit ARM chip, with more memory for storing your program and running it. There is also a pin that provides a digital-to-analog converter (DAC), which means you can get a varying analog voltage from it. This can be used to generate audio signals at much higher quality than an Uno. The Zero is not 5V tolerant, nor are the similar boards from Adafruit (Metro M0 Express) or SparkFun (RedBoard Turbo).
The MKR1010 uses the same chip as the Zero (and like the Zero, is not 5V tolerant), but in a smaller form factor. It also includes WiFi, so it is able to connect to the internet through a WiFi network. The MKR form factor does not support shields that are designed for the Uno pin layout.
All the 32-bit boards have more pins that support interrupts than most of the 8-bit boards, which are useful for applications that must quickly detect signal changes (see Recipe 18.2). The one 8-bit exception to this is the Arduino Uno WiFi Rev2, which supports interrupts on any of its digital pins.
See Also
Online guides for getting started with Arduino are available at for Windows, for macOS, and for Linux. See the Arduino Guide for board-specific instructions.
A troubleshooting guide can be found on the Arduino site.
1.3 Using the Integrated Development Environment to Prepare an Arduino Sketch
Solution
Source code for Arduino is called a sketch. The process that takes a sketch and converts it into a form that will work on the board is called compilation. Use the Arduino IDE to create, open, and modify sketches that define what the board will do. You can use buttons along the top of the IDE to perform these actions (shown in Figure 1-6), or you can use the menus or keyboard shortcuts (visible in Figure 1-5).
The Sketch Editor area is where you view and edit code for a sketch. It supports common text-editing shortcuts such as Ctrl-F (⌘+F on a Mac) for find, Ctrl-Z (⌘+Z on a Mac) for undo, Ctrl-C (⌘+C on a Mac) to copy highlighted text, and Ctrl-V (⌘+V on a Mac) to paste highlighted text.
Figure 1-5 shows how to load the Blink sketch (the sketch that comes preloaded on a new Arduino board).
After you have started the IDE, go to the File→Examples menu and select 01. Basics→Blink, as shown in Figure 1-5. The code for blinking the built-in LED will be displayed in the Sketch Editor window (refer to Figure 1-6).
Before you can send the code to the board, it needs to be converted into instructions that can be read and executed by the Arduino controller chip; this is called compiling. To do this, click the verify/compile button (the top-left button with a checkmark inside), or select Sketch→Verify/Compile (Ctrl-R; ⌘+R on a Mac).
You should see a message that reads “Compiling sketch...” and a progress bar in the message area below the text-editing window. After a second or two, a message that reads “Done Compiling” will appear. The black console area will contain the following additional message:
Sketch
uses
930
bytes
(
2
%
)
of
program
storage
space
.
Maximum
is
32256
bytes
.
Global
variables
use
9
bytes
(
0
%
)
of
dynamic
memory
,
leaving
2039
bytes
for
local
variables
.
Maximum
is
2048
bytes
.
The exact message may differ depending on your board and Arduino version; it is telling you the size of the sketch and the maximum size that your board can accept.
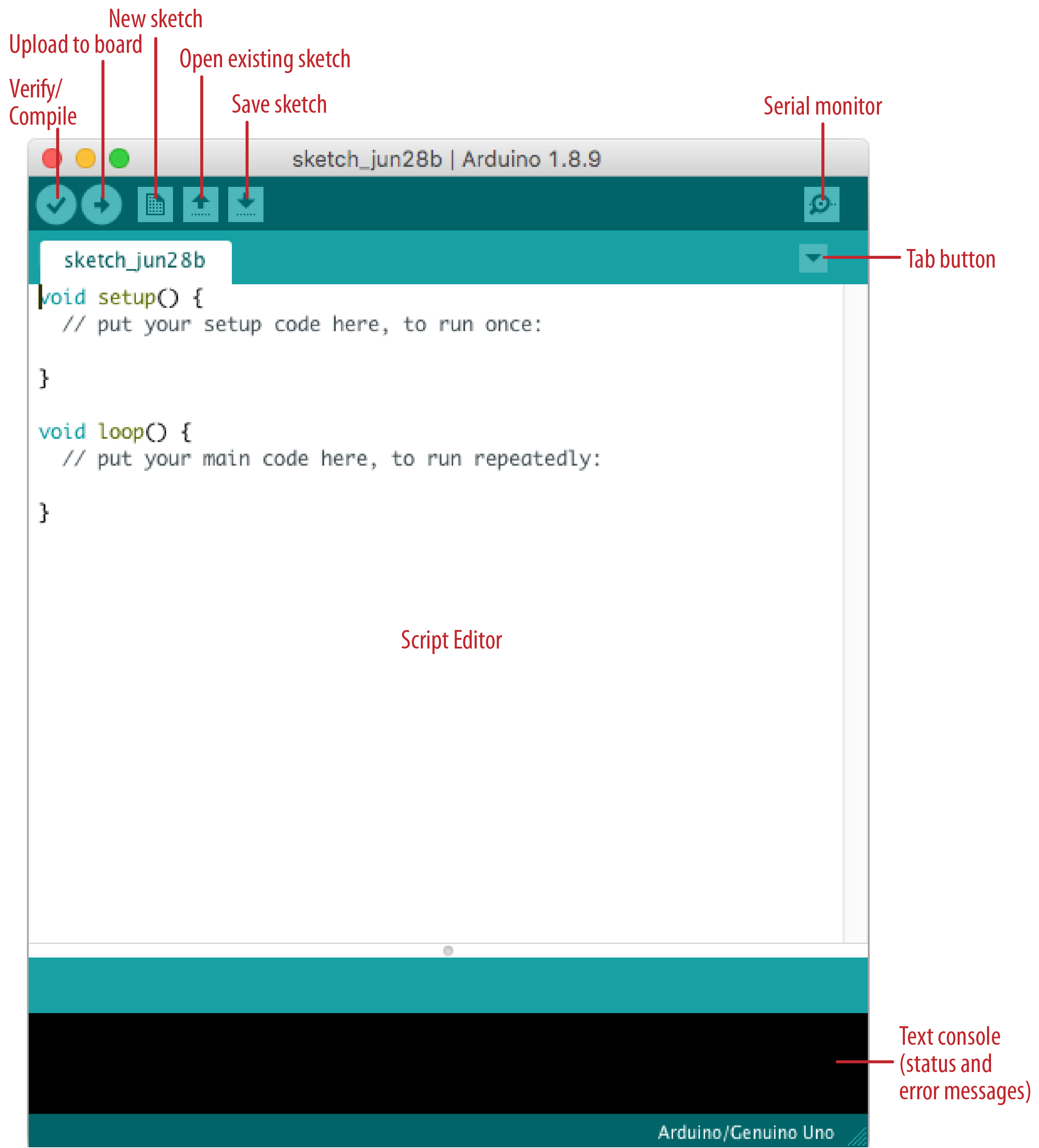
Arduino IDE on macOS
Discussion
The IDE uses a number of command-line tools behind the scenes to compile a sketch. For more information on this, see Recipe 17.1.
The final message telling you the size of the sketch indicates how much program space is needed to store the controller instructions on the board. If the size of the compiled sketch is greater than the available memory on the board, the following error message is displayed:
Sketch
too
big
;
see
http
:
//www.arduino.cc/en/Guide/Troubleshooting#size for tips on reducing it.
If this happens, you need to make your sketch smaller to be able to put it on the board, or get a board with higher flash memory capacity. If your global variables are using too much memory, you’ll see a different error instead:
Not
enough
memory
;
see
http
:
//www.arduino.cc/en/Guide/Troubleshooting#size
for
tips
on
reducing
your
footprint
.
In that case, you’ll need to go through your code and reduce the amount of memory that you are allocating to global variables, or get a board with a higher SRAM (dynamic memory) capacity.
Tip
To prevent you from accidentally overwriting the example code, the Arduino IDE does not allow you to save changes to the built-in example sketches. You must rename them using the File→Save As menu option. You can save sketches you write yourself with the Save button (see Recipe 1.5).
If there are errors in the code, the compiler will print one or more error messages in the console window. These messages can help identify the error—see Appendix D on software errors for troubleshooting tips.
The compiler can also generate warnings if it decides there are some peculiarities about your sketch that could cause problems. These can be very helpful to avoid problems that could trip you up later. You can configure your warning level by opening File→Preferences (Windows or Linux) or Arduino→Preferences (macOS) and setting Compiler Warnings to None, Default, More, or All. Despite the name, Arduino defaults to None. We suggest you set this to Default or More.
Note
Code uploaded onto the board cannot be downloaded back onto your computer. Make sure you save your sketch code on your computer. You cannot save changes that you’ve made to the example files; you need to use Save As and give the changed file another name.
See Also
Recipe 1.5 shows an example sketch. Appendix D has tips on troubleshooting software problems.
1.4 Uploading and Running the Blink Sketch
Solution
Connect your Arduino board to your computer using the USB cable. Load the Blink sketch into the IDE by choosing File→Examples and selecting 01. Basics→Blink.
Next, select Tools→Board from the drop-down menu and select the name of the board you have connected (if it is the standard Uno board, it is probably one of the first entries in the board list).
Now select Tools→Serial Port. You will get a drop-down list of available serial ports on your computer. Each machine will have a different combination of serial ports, depending on what other devices you have used with your computer.
On Windows, they will be listed as numbered COM entries. If there is only one entry, select it. If there are multiple entries, your board will probably be the last entry.
On the Mac, if your board is an Uno it will be listed as:
/dev/cu.usbmodem-XXXXXXX
(Arduino/Genuino Uno)
If you have an older board, it will be listed as follows:
/dev/tty.usbserial-XXXXXXX
/dev/cu.usbserial-XXXXXXX
Each board will have different values for XXXXXXX
. Select either entry.
On Linux, if your board is an Uno it will probably be listed as:
/dev/ttyACMX
(Arduino/Genuino Uno)
If you have an older board, it may be listed as follows:
/dev/ttyUSB-X
X
is usually 0, but you will see 1, 2, etc. if you have multiple boards connected at once. Select the entry that corresponds to your Arduino.
Tip
If you have so many entries in the Port menu that you can’t figure out which one goes to your Arduino, try this: look at the menu with the Arduino unplugged from your computer, then plug the Arduino in and look for the menu option that wasn’t there before. Another approach is to select the ports one by one, and try uploading until you see the lights on the board flicker to indicate that the code is uploading.
Click the upload button (in Figure 1-6, it’s the second button from the left), or choose Sketch→Upload (Ctrl-U; ⌘+U on a Mac).
The IDE will compile the code, as in Recipe 1.3. After the software is compiled, it is uploaded to the board. If this is a fresh-out-of-the-box Arduino that’s preloaded with the Blink sketch, you will see the onboard LED (labeled as Onboard LED in Figure 1-4) stop blinking. When the upload begins, two LEDs (labeled as Serial LEDs in Figure 1-4) near the onboard LED should flicker for a couple of seconds as the code uploads. The onboard LED should then start flashing as the code runs. The location of the onboard LED differs across some Arduino models, such as the Leonardo, MKR boards, and third-party Arduino clones.
Discussion
For the IDE to send the compiled code to the board, the board needs to be plugged into the computer, and you need to tell the IDE which board and serial port you are using.
When an upload starts, whatever sketch is running on the board is stopped (if you were running the Blink sketch, the LED will stop flashing). The new sketch is uploaded to the board, replacing the previous sketch. The new sketch will start running when the upload has successfully completed.
Note
Some older Arduino boards and compatibles do not automatically interrupt the running sketch to initiate upload. In this case, you need to press the Reset button on the board just after the software reports that it is done compiling (when you see the message about the size of the sketch). It may take a few attempts to get the timing right between the end of the compilation and pressing the Reset button.
The IDE will display an error message if the upload is not successful. Problems are usually due to the wrong board or serial port being selected or the board not being plugged in. The currently selected board and serial port are displayed in the status bar at the bottom of the Arduino window.
See Also
For more, see the Arduino troubleshooting page.
1.5 Creating and Saving a Sketch
Solution
To open an editor window ready for a new sketch, launch the IDE (see Recipe 1.3), go to the File menu, and select New. Delete the boilerplate code that is in the Sketch Editor window, and paste the following code in its place (it’s similar to the Blink sketch, but the blinks last twice as long):
void
setup
()
{
pinMode
(
LED_BUILTIN
,
OUTPUT
);
}
void
loop
()
{
digitalWrite
(
LED_BUILTIN
,
HIGH
);
// set the LED on
delay
(
2000
);
// wait for two seconds
digitalWrite
(
LED_BUILTIN
,
LOW
);
// set the LED off
delay
(
2000
);
// wait for two seconds
}
Compile the code by clicking the verify/compile button (the top-left button with a checkmark inside), or select Sketch→Verify/Compile (see Recipe 1.3).
Upload the code by clicking the upload button, or choose Sketch→Upload (see Recipe 1.4). After uploading, the LED should blink, with each flash lasting two seconds.
You can save this sketch to your computer by clicking the Save button, or select File→Save. You can save a sketch using a new name by selecting the Save As menu option. A dialog box will open where you can enter the filename.
Discussion
When you save a file in the IDE, a standard dialog box for the operating system will open. It suggests that you save the sketch to a folder called Arduino in your My Documents folder (or your Documents folder on a Mac). You can replace the default sketch name with a meaningful name that reflects the purpose of your sketch. Click Save to save the file.
Note
The default name is the word sketch followed by the current date. Sequential letters starting from a are used to distinguish sketches created on the same day. Replacing the default name with something meaningful helps you to identify the purpose of a sketch when you come back to it later.
If you use characters that the IDE does not allow (e.g., the space character), the IDE will automatically replace these with valid characters.
Arduino sketches are saved as plain-text files with the extension .ino. Older versions of the IDE used the .pde extension, also used by Processing. They are automatically saved in a folder with the same name as the sketch.
You can save your sketches to any folder, but if you use the default folder (the Arduino folder in your Documents folder; ~/Arduino on Linux) your sketches will appear in the Sketchbook menu of the Arduino software and be easier to locate.
If you have edited one of the built-in examples, you will not be able to save the changed file into the examples folder, so you will be prompted to save it into a different folder.
After you have made changes, you will see a dialog box asking if you want to save the sketch when a sketch is closed. The § symbol following the name of the sketch in the top bar of the IDE window indicates that the sketch code has changes that have not yet been saved on the computer. This symbol is removed when you save the sketch.
As you develop and modify a sketch, you will want a way to keep track of changes. The easiest way to do this is to use the Git version control system (see this Atlassian Git Tutorial page for installation information). Git is typically accessed using a command-line interface (there are graphical clients available as well). The basic workflow for putting a sketch under version control in Git is:
-
Figure out which folder your sketch resides in. You can find this using Sketch→Show Sketch Folder. This will open the sketch folder in your computer’s file manager.
-
Open a command line (on Windows, Command Prompt; on Linux or macOS, open a Terminal). Use the
cd
command to change to the directory where your sketch is located. For example, if you saved a sketch called Blink in the default sketch folder location, you’d be able to change to that directory with the following on macOS, Linux, and Windows, respectively:$ cd ~/Documents/Arduino/Blink $ cd ~/Arduino/Blink > cd %USERPROFILE%\Documents\Arduino\Blink
-
Initialize the Git repository with the
git init
command. -
Add the Sketch file to Git with
git add Blink.ino
(replaceBlink.ino
with the name of your sketch). If you add any additional files to your sketch folder, you’ll need to add them with thegit add filename
command. -
After you have made substantial changes, type
git commit -a -m "your comment here"
. Replace “your comment here” with something that describes the change you made.
After you’ve committed a change to Git, you can use git log
to see a history of your changes. Each one of those changes will have a commit hash associated with it:
commit 87e962e54fe46d9e2a00575f7f0d1db6b900662a (HEAD -> master) Author: Brian Jepson <bjepson@gmail.com> Date: Tue Jan 14 20:58:56 2020 -0500 made massive improvements commit 0ae1a1bcb0cd245ca9427352fc3298d6ccb91cef (HEAD -> master) Author: Brian Jepson <bjepson@gmail.com> Date: Tue Jan 14 20:56:45 2020 -0500 your comment here
With these hashes, you can work with older versions of files (you don’t need the full hash, just enough of it to differentiate between versions). You can restore an old version with git checkout hash filename
, as in git checkout 0ae1 Blink.ino
. You can compare versions with git diff firsthash..secondhash
, as in git diff 0ae1..7018
. See https://git-scm.com/doc for complete documentation on Git.
Frequent compiling as you modify or add code is a good way to check for errors. It will be easier to find and fix any errors because they will usually be associated with what you have just written.
Note
Once a sketch has been uploaded onto the board there is no way to download it back to your computer. Make sure you save any changes to your sketches that you want to keep.
If you try to save a sketch file that is not in a folder with the same name as the sketch, the IDE will inform you that this can’t be opened as is and suggest you click OK to create the folder for the sketch with the same name.
Note
Sketches must be located in a folder with the same name as the sketch. The IDE will create the folder automatically when you save a new sketch.
Sketches made with older versions of Arduino software have a different file extension (.pde). The IDE will open them, and when you save the sketch it will create a file with the new extension (.ino). Code written for early versions of the IDE may not be able to compile in version 1.0. Most of the changes to get old code running are easy to do.
1.6 An Easy First Arduino Project
Solution
This recipe provides a taste of some of the techniques that are covered in detail in later chapters.
The sketch is based on the LED blinking code from the previous recipe, but instead of using a fixed delay, the rate is determined by a light-sensitive sensor called a photoresistor or light-dependent resistor (LDR for short, see Recipe 6.3). Wire the photoresistor as shown in Figure 1-7.
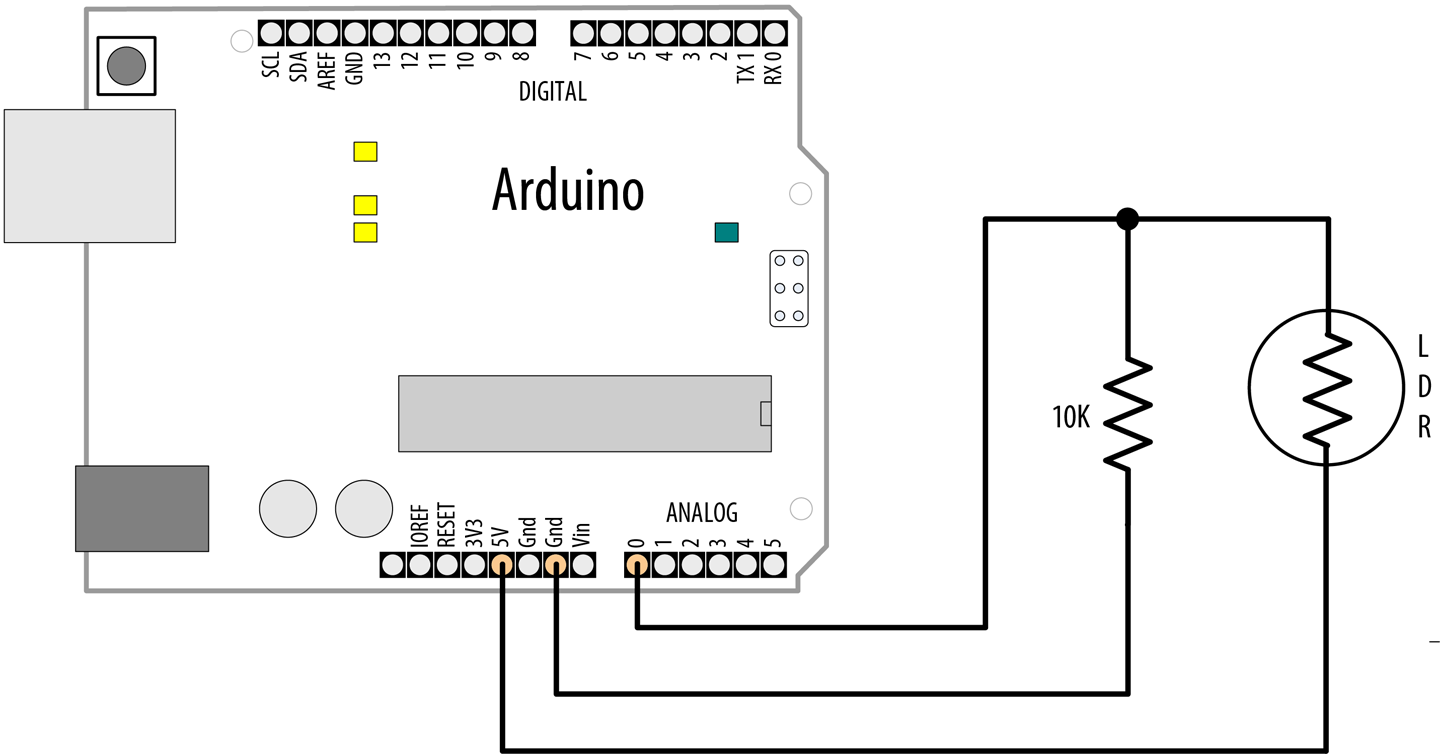
Arduino with photoresistor
If you are not familiar with building a circuit from a schematic, see Appendix B for step-by-step illustrations on how to make this circuit on a breadboard.
Note
Photoresistors contain a compound (cadmium sulfide) that is a hazardous substance. You can use a phototransistor if you live in a jurisdiction where it is difficult to obtain a photoresistor, or if you simply prefer to not use a photoresistor. A phototransistor has a long lead and a short lead, much like an LED. You can wire it exactly as shown in the figure, but you must connect the long lead to 5V and the short lead to the resistor and pin 0. Be sure to buy a phototransistor such as Adafruit part number 2831 that can sense visible light so you can test it with a common light source.
The following sketch reads the light level of a photoresistor connected to analog pin 0. The light level striking the photoresistor will change the blink delay of the internal onboard LED:
/*
* Blink with photoresistor sketch
*/
const
int
sensorPin
=
A0
;
// connect sensor to analog input 0
void
setup
()
{
pinMode
(
LED_BUILTIN
,
OUTPUT
);
// enable output on the led pin
}
void
loop
()
{
int
delayval
=
analogRead
(
sensorPin
);
// read the analog input
digitalWrite
(
LED_BUILTIN
,
HIGH
);
// set the LED on
delay
(
delayval
);
// delay is dependent on light level
digitalWrite
(
LED_BUILTIN
,
LOW
);
// set the LED off
delay
(
delayval
);
}
The code in this recipe and throughout this book uses the const int
expression to provide meaningful names (sensorPin
) for constants instead of numbers (0
). See Recipe 17.5 for more on the use of constants.
Discussion
The value of the resistor shown in Figure 1-7 depends on the range of your photoresistor: you will want a resistor that is in the ballpark of the maximum (dark) resistance of your photoresistor (you can find this by covering the photoresistor while you measure its resistance on a multimeter). So if your photoresistor measures 10K ohms in darkness, use a 10K resistor. If you are using a phototransistor, you will generally be OK with a value between 1K and 10K. The light level on the sensor will change the voltage level on analog pin 0. The analogRead
command (see Chapter 6) provides a value that ranges from around 200 when the sensor is dark to 800 or so when it is very bright (the sensitivity will vary depending on the type of photoresistor and resistor you use, and whether you use a phototransistor in place of the photoresistor). The analog reading determines the duration of the LED on and off times, so the blink delay increases with light intensity.
You can scale the blink rate by using the Arduino map
function as follows:
/*
* Blink with photoresistor (scaled) sketch
*/
const
int
sensorPin
=
A0
;
// connect sensor to analog input 0
// low and high values for the sensor readings; you may need to adjust these
const
int
low
=
200
;
const
int
high
=
800
;
// The next two lines set the min and max delay between blinks.
const
int
minDuration
=
100
;
// minimum wait between blinks
const
int
maxDuration
=
1000
;
// maximum wait between blinks
void
setup
()
{
pinMode
(
LED_BUILTIN
,
OUTPUT
);
// enable output on the LED pin
}
void
loop
()
{
int
delayval
=
analogRead
(
sensorPin
);
// read the analog input
// the next line scales the delay value between the min and max values
delayval
=
map
(
delayval
,
low
,
high
,
minDuration
,
maxDuration
);
delayval
=
constrain
(
delayval
,
minDuration
,
maxDuration
);
digitalWrite
(
LED_BUILTIN
,
HIGH
);
// set the LED on
delay
(
delayval
);
// delay is dependent on light level
digitalWrite
(
LED_BUILTIN
,
LOW
);
// set the LED off
delay
(
delayval
);
}
Note
If you’re not seeing any change in values as you adjust the light, you will need to play with the values for low
and high
. If you are using a phototransistor and aren’t getting changes in the blink rate, try a value of 10 for low
.
Recipe 5.7 provides more details on using the map
function to scale values. Recipe 3.5 has details on using the constrain
function to ensure values do not exceed a given range. If, for some reason, your delay value is outside the range between low
and high
, map
will return a value outside the range between minDuration
and maxDuration
. If you call constrain
after map
as shown in the sketch, you will avoid the problem of out-of-range values.
If you want to view the value of the delayval
variable on your computer, you can print this to the Arduino Serial Monitor as shown in the revised loop code that follows. The sketch will display the delay value in the Serial Monitor. You open the Serial Monitor window in the Arduino IDE by clicking the icon on the right of the top bar (see Chapter 4 for more on using the Serial Monitor):
/*
* Blink sketch with photoresistor (scaled with serial output)
*/
const
int
sensorPin
=
A0
;
// connect sensor to analog input 0
// Low and high values for the sensor readings. You may need to adjust these.
const
int
low
=
200
;
const
int
high
=
800
;
// the next two lines set the min and max delay between blinks
const
int
minDuration
=
100
;
// minimum wait between blinks
const
int
maxDuration
=
1000
;
// maximum wait between blinks
void
setup
()
{
pinMode
(
LED_BUILTIN
,
OUTPUT
);
// enable output on the led pin
Serial
.
begin
(
9600
);
// initialize Serial
}
void
loop
()
{
int
delayval
=
analogRead
(
sensorPin
);
// read the analog input
// the next line scales the delay value between the min and max values
delayval
=
map
(
delayval
,
low
,
high
,
minDuration
,
maxDuration
);
delayval
=
constrain
(
delayval
,
minDuration
,
maxDuration
);
Serial
.
println
(
delayval
);
// print delay value to serial monitor
digitalWrite
(
LED_BUILTIN
,
HIGH
);
// set the LED on
delay
(
delayval
);
// delay is dependent on light level
digitalWrite
(
LED_BUILTIN
,
LOW
);
// set the LED off
delay
(
delayval
);
}
You can use the sensor to control the pitch of a sound by connecting a small speaker to the pin, as shown in Figure 1-8.
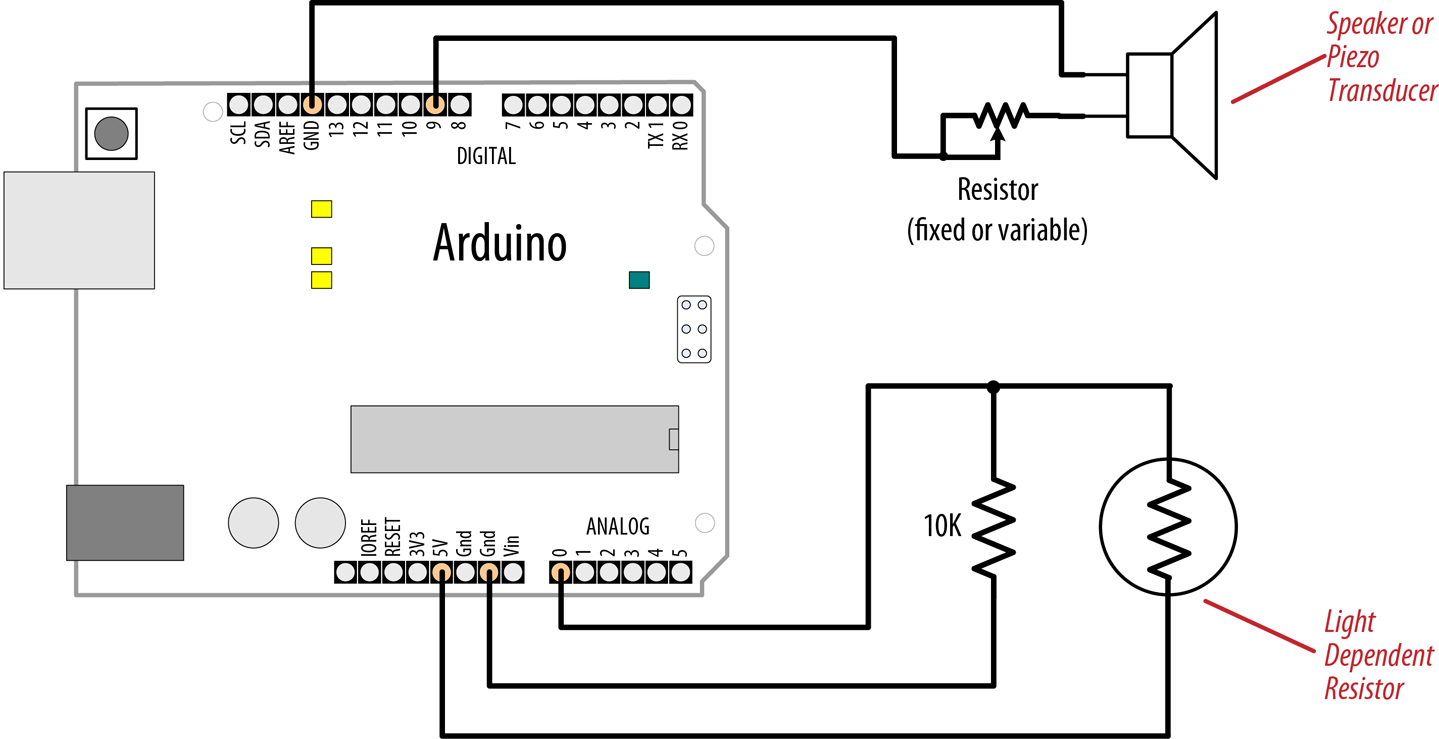
Connections for a speaker with the photoresistor circuit
You will need to increase the on/off rate on the pin to a frequency in the audio spectrum. This is achieved, as shown here, by decreasing the min and max durations:
/* * Speaker sketch with photoresistor */
const
int
outputPin
=
9
;
// Speaker connected to digital pin 9
const
int
sensorPin
=
A0
;
// connect sensor to analog input 0
const
int
low
=
200
;
const
int
high
=
800
;
const
int
minDuration
=
1
;
// 1 ms on, 1 ms off (500 Hz)
const
int
maxDuration
=
10
;
// 10 ms on, 10 ms off (50 Hz)
void
setup
(
)
{
pinMode
(
outputPin
,
OUTPUT
)
;
// enable output on the led pin
}
void
loop
(
)
{
int
sensorReading
=
analogRead
(
sensorPin
)
;
// read the analog input
int
delayval
=
map
(
sensorReading
,
low
,
high
,
minDuration
,
maxDuration
)
;
delayval
=
constrain
(
delayval
,
minDuration
,
maxDuration
)
;
digitalWrite
(
outputPin
,
HIGH
)
;
// set the pin on
delay
(
delayval
)
;
// delay is dependent on light level
digitalWrite
(
outputPin
,
LOW
)
;
// set the pin off
delay
(
delayval
)
;
}
See Also
For a full discussion on audio output with Arduino, see Chapter 9.
1.7 Using Arduino with Boards Not Included in the Standard Distribution
Problem
You want to use a board such as the Arduino MKR 1010, but it does not appear in the boards menu.
Solution
To use the MKR 1010 with Arduino, you need to add its details to the Arduino software you have already downloaded. To do this go to Tools→Board→Boards Manager (Figure 1-9).
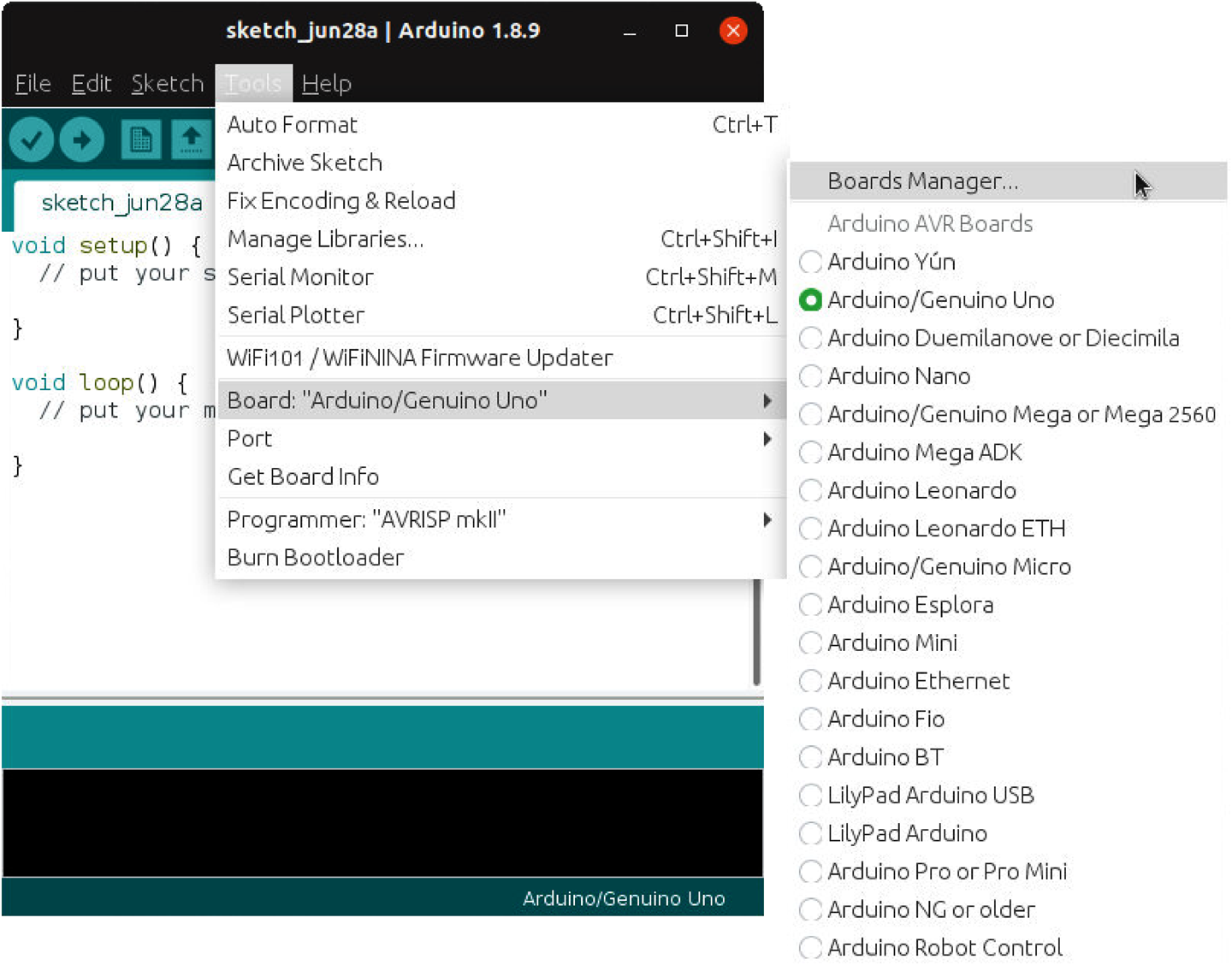
Selecting Boards Manager (Linux version of Arduino IDE shown)
As this window opens, the list of board definitions available online will be checked to ensure you have the latest versions available, so wait till this has finished.
Adding Other Boards to the Boards Menu
The procedure described here is similar for other boards you may want to add to the boards menu. Check the documentation for your board to find the location of the definition files.
The window that opens (Figure 1-10) shows you the board definitions that are already installed and ones that are available to download.
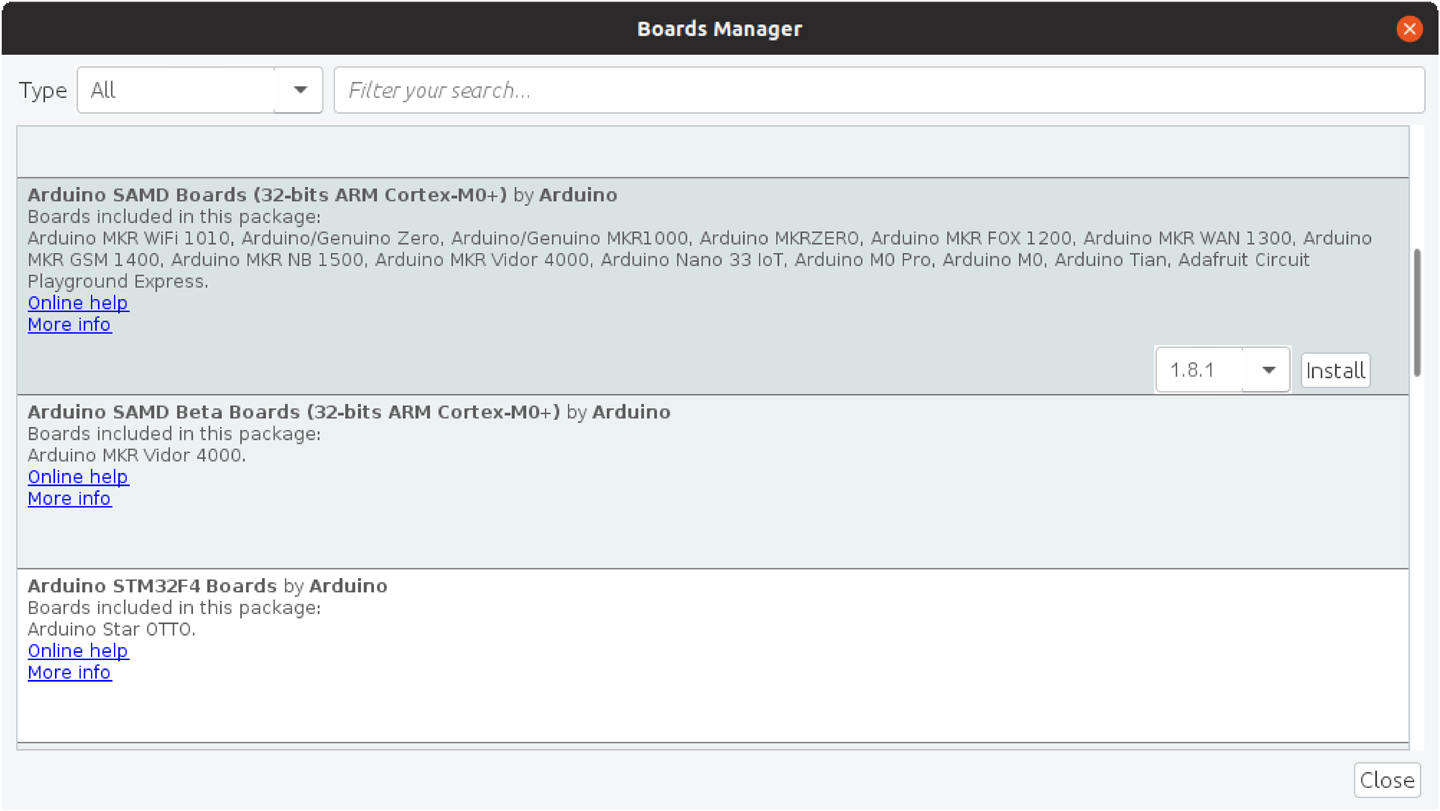
The Boards Manager
To find the MKR 1010 you can scroll down the list, or type its name in the filter box. For the MKR 1010, you’ll need to select the Arduino SAMD Boards entry from the list. Once you have selected it, click install and it will be downloaded and added to the Arduino IDE. This may take some time.
Once it has finished you can add other boards, or click Close to finish using the Boards Manager. If you open Tools→Board, you should now have the option of selecting the MKR 1010 as shown in Figure 1-11.

The MKR 1010 is now installed and can be programmed using the Arduino IDE
Discussion
The files that you download when you do this describe how to map the programming concepts in Arduino that connect to specific bits of hardware in the board’s microcontroller chip, to where that hardware is located in a specific chip or family of chips.
Once you have added the description for a particular chip, you will often be able to work with a family of boards that use that chip. For example, adding support for the MKR 1010 board also provides support for the Arduino Zero as both boards use the same microcontroller chip.
To facilitate support for the growing number of Arduino and Arduino-compatible boards, the Arduino IDE added a Boards Manager in release 1.6. The Boards Manager was developed to enable people to easily add and remove board details from their installation. It also enables you to update the board support files if newer versions are available, or choose the version you use if you need to use a particular one. The Arduino IDE no longer includes the description files for all the Arduino boards, so even if you download the latest IDE you may not get the descriptions for the board you have.
The Boards Manager also enables third parties to add the details of their boards to the system. If their board descriptions are available online in the correct format, you can add the location as one of the places for Boards Manager to use to populate the list it produces. This means those files will also get checked whenever the Boards Manager updates its details, so you get notified of updates and can use the same mechanism to update them once they are installed. To do this, go to Arduino→Preferences and click the icon to the right of the Additional Boards Manager URLs field, and the Additional Boards Manager URL dialog will appear as shown in Figure 1-12.
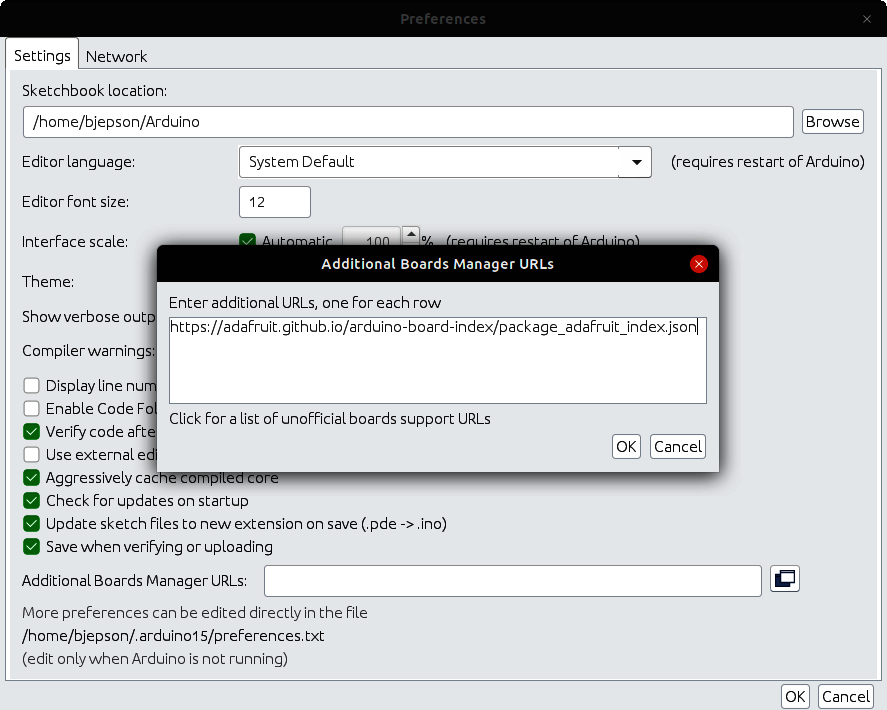
Preferences after clicking the icon to the right of the Additional Boards Manager URLs entry
If the people who made the board provide a URL to add to Arduino, paste it into the “additional URLs” dialog box (on a separate line if there are any other entries). If there isn’t an explicit URL, click the text below the box to go to the web page that maintains a list of unofficial Arduino board description URLs and see if you can find a link there.
If you want to use a Teensy board, you need to download a separate installer program from the Teensy website. It is important that you use a Teensy installer that has support for the IDE version that you are using. A compatible version is usually produced within a week or two of a new Arduino release.
See Also
Quick start guides for various Arduino boards
1.8 Using a 32-Bit Arduino (or Compatible)
Problem
You want 32-bit performance in the Uno form factor.
Solution
The Arduino Zero (Figure 1-13) has the familiar pin layout of the Uno but has much more memory and a faster processor. If you have trouble obtaining a Zero, Adafruit’s Metro M0 Express and SparkFun’s RedBoard Turbo are compatible alternatives.
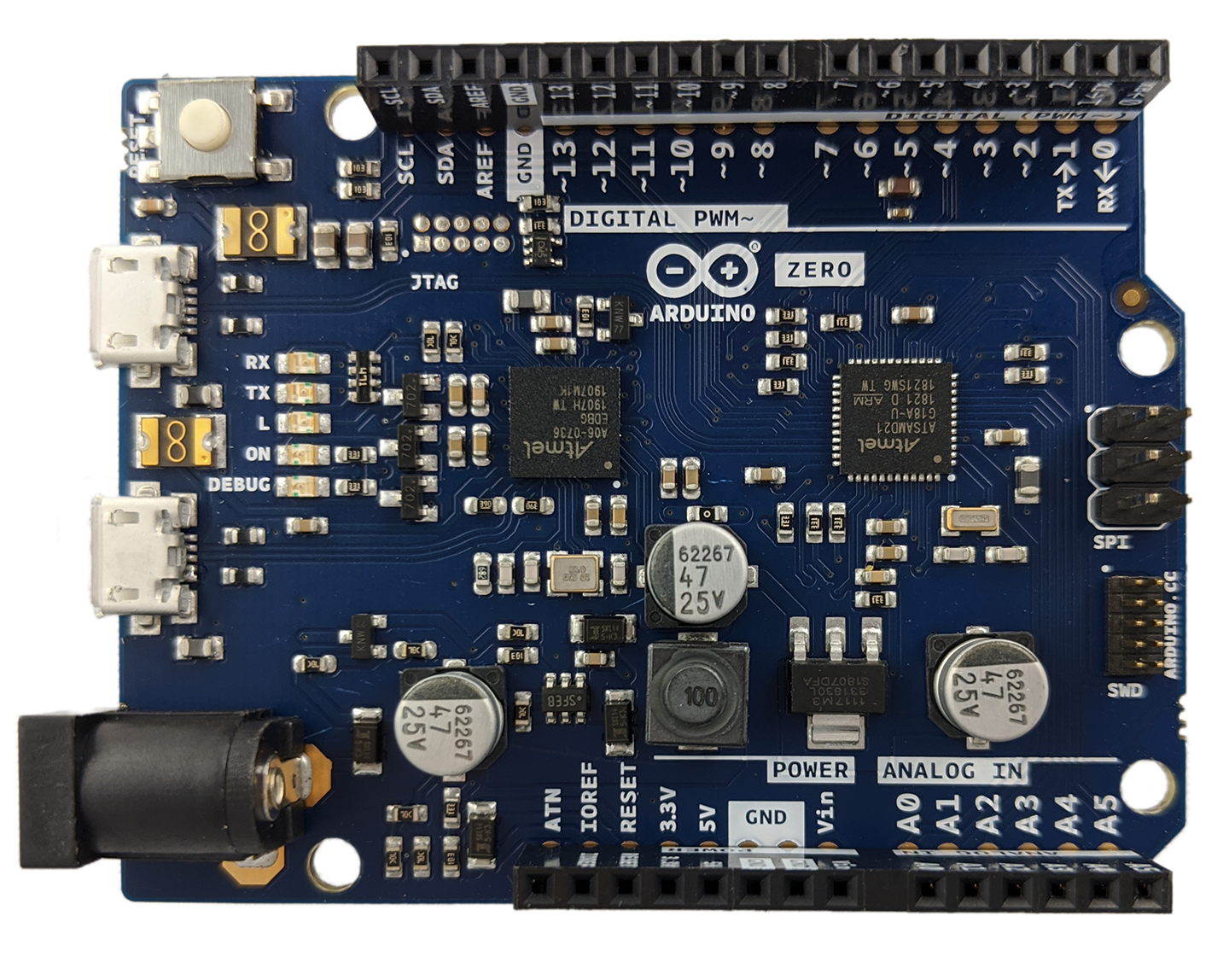
The Arduino/Genuino Zero board
Despite the similar physical layout of the pins, there are a number of differences. What distinguishes these boards from the Uno is that they use a 32-bit ARM chip, the Microchip SAMD21. The following sketch, similar to the previous recipe, highlights some significant differences between the ARM-based boards and the Uno:
Note
If you’re not hearing any change in values as you adjust the light, you will need to play with the values for low
and high
. If you are using a phototransistor and aren’t getting changes in the blink rate, try a value of 10 for low
. If your ambient light is from a fluorescent or LED source, you may hear a distinct warbling to the sound due to flicker in such sources that are visually imperceptible.
/*
* Zero wave sketch
*/
const
int
outputPin
=
A0
;
// headphones connected to analog 0
const
int
sensorPin
=
A1
;
// connect sensor to analog input 1
const
int
low
=
200
;
const
int
high
=
800
;
const
int
sampleCount
=
16
;
// number of samples used to render one cycle
const
int
minDur
=
1000000
/
(
sampleCount
*
500
);
// period in uS for 500 Hz
const
int
maxDur
=
1000000
/
(
sampleCount
*
50
);
// period for 50 Hz
// table of values for 16 samples of one sine wave cycle
static
int
sinewave
[
sampleCount
]
=
{
0x1FF
,
0x2B6
,
0x355
,
0x3C7
,
0x3FF
,
0x3C7
,
0x355
,
0x2B6
,
0x1FF
,
0x148
,
0x0A9
,
0x037
,
0x000
,
0x037
,
0x0A9
,
0x148
};
void
setup
()
{
analogWriteResolution
(
10
);
// set the Arduino DAC resolution to maximum
}
void
loop
()
{
int
sensorReading
=
analogRead
(
sensorPin
);
// read the analog input
int
duration
=
map
(
sensorReading
,
low
,
high
,
minDur
,
maxDur
);
duration
=
constrain
(
duration
,
minDur
,
maxDur
);
duration
=
constrain
(
duration
,
minDur
,
maxDur
);
for
(
int
sample
=
0
;
sample
<
sampleCount
;
sample
++
)
{
analogWrite
(
outputPin
,
sinewave
[
sample
]);
delayMicroseconds
(
duration
);
}
}
Before you can load sketches on the Zero, Adafruit Metro M0 or M4, or SparkFun RedBoard, open the Arduino Boards Manager and install the appropriate package (see Recipe 1.7). If you are using an Adafruit or SparkFun board, you’ll need to add its board manager URL to the Arduino IDE first. See Adafruit or SparkFun for details. After you’ve installed support for your SAMD board, use the Tools menu to configure the Arduino IDE to use that board and set the correct serial port for connecting to it. Connect a resistor, potentiometer, and photoresistor (also known as a light-dependent resistor) as shown in Figure 1-14. Next, upload the code using the Arduino IDE.
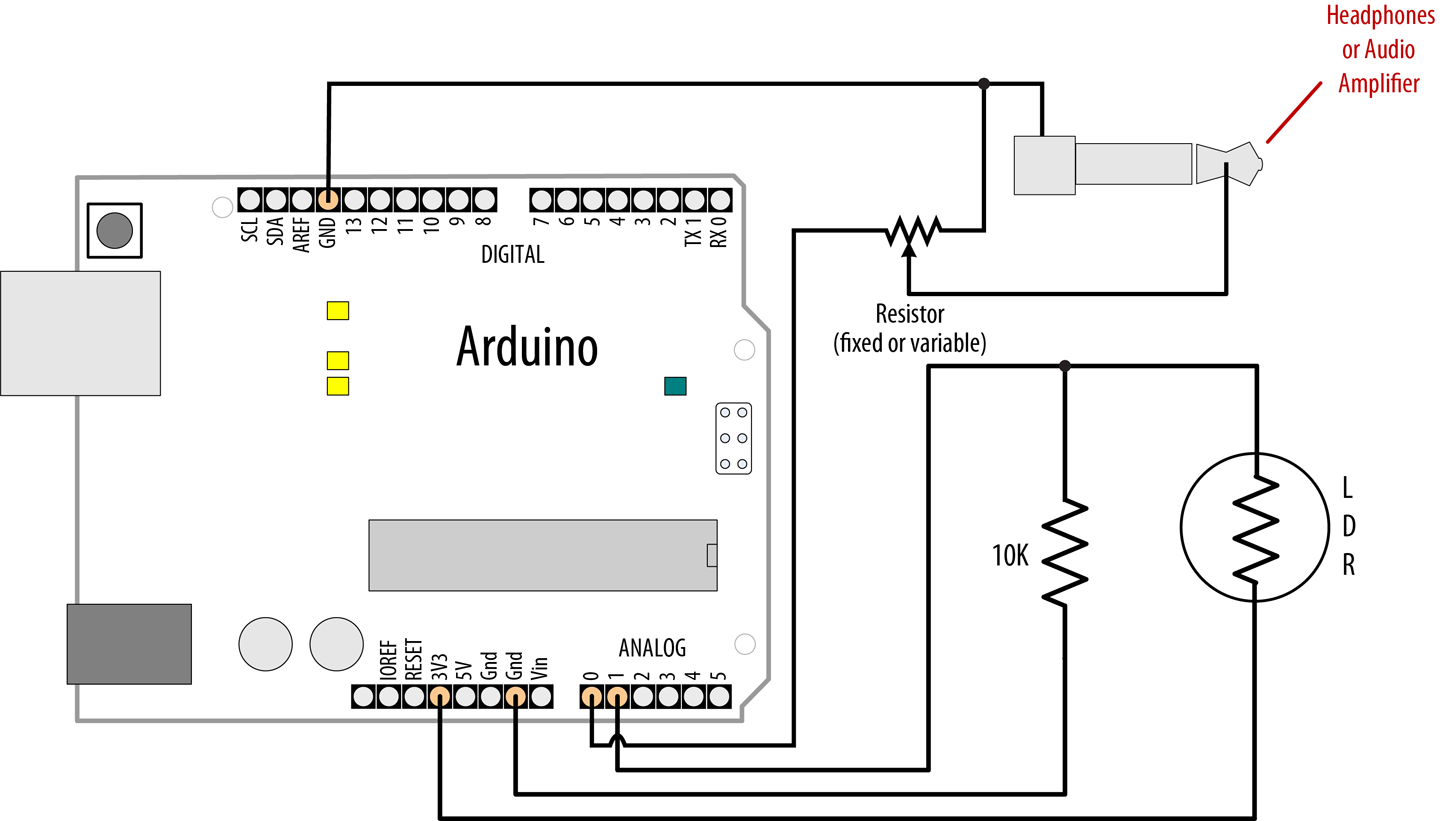
Connections for audio output with the photoresistor circuit for the Zero board
Discussion
Although the wiring may appear similar to Figure 1-8 at first glance, the sensor input and audio output use different pins. These boards have a digital-to-analog converter (DAC) that can create more realistic audio than the binary output of standard digital pins. However, the DAC is only available on analog pin 0 so the sensor input is here connected to analog pin 1.
Another difference that may not be obvious from the figure is that these boards can only drive up to 7 mA on a pin, compared to 40 mA on the Uno. And because the pin voltage ranges from 0 to 3.3 volts, compared to the 0- to 5-volt range of the Uno, the maximum power delivered to a pin is almost 10 times less than the Uno. For that reason, the output pins should be connected to headphones or an amplifier input as they will not drive a speaker directly.
The sketch uses a lookup table of 16 samples per sine wave cycle, however these boards are fast enough to handle much higher resolutions, and you can increase the number of samples to improve the purity of the signal.
Get Arduino Cookbook, 3rd Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.