Chapter 1. A Taste of Py
Let’s begin with a mini-mystery and its solution. What do you think the following two lines mean?
(Row 1): (RS) K18,ssk,k1,turn work. (Row 2): (WS) Sl 1 pwise,p5,p2tog,p1,turn.
It looks technical, like some kind of computer program. Actually, it’s a knitting pattern; specifically, a fragment describing how to turn the heel of a sock. This makes as much sense to me as the New York Times crossword puzzle does to my cat, but my wife understands it perfectly. If you’re a knitter, you do, too.
Let’s try another example. You’ll figure out its purpose right away, although you might not know its final product.
1/2 c. butter or margarine 1/2 c. cream 2 1/2 c. flour 1 t. salt 1 T. sugar 4 c. riced potatoes (cold) Be sure all ingredients are cold before adding flour. Mix all ingredients. Knead thoroughly. Form into 20 balls. Store cold until the next step. For each ball: Spread flour on cloth. Roll ball into a circle with a grooved rolling pin. Fry on griddle until brown spots appear. Turn over and fry other side.
Even if you don’t cook, you probably recognized that it’s a recipe: a list of food ingredients followed by directions for preparation. But what does it make? It’s lefse, a Norwegian delicacy that resembles a tortilla. Slather on some butter and jam or whatever you like, roll it up, and enjoy.
The knitting pattern and the recipe share some features:
-
A fixed vocabulary of words, abbreviations, and symbols. Some might be familiar, others mystifying.
-
A sequence of operations to be performed in order.
-
Sometimes, a repetition of some operations (a loop), such as the method for frying each piece of lefse.
-
Sometimes, a reference to another sequence of operations (in computer terms, a function). In the recipe, you might need to refer to another recipe for ricing potatoes.
-
Assumed knowledge about the context. The recipe assumes you know what water is and how to boil it. The knitting pattern assumes that you can knit and purl without stabbing yourself too often.
-
An expected result. In our examples, something for your feet and something for your stomach. Just don’t mix them up.
You’ll see all of these ideas in computer programs. I used these nonprograms to demonstrate that programming isn’t that mysterious. It’s just a matter of learning the right words and the rules.
Let’s leave these stand-ins and see a real program. What does this do?
for
countdown
in
5
,
4
,
3
,
2
,
1
,
"hey!"
:
(
countdown
)
If you guessed that it’s a Python program that prints the lines:
5 4 3 2 1 hey!
then you know that Python can be easier to learn than a recipe or knitting pattern. And, you can practice writing Python programs from the comfort and safety of your desk, far from the harrowing dangers of hot water and pointy sticks.
The Python program has some special words and symbols—for
, in
, print
,
commas, colons, parentheses, and so on—that are important parts of the language’s syntax.
The good news is that Python has a nicer syntax,
and less of it to remember,
than most computer languages.
It seems more natural—almost like a recipe.
Here’s another tiny Python program that selects a television news cliché from a Python list and prints it:
cliches
=
[
"At the end of the day"
,
"Having said that"
,
"The fact of the matter is"
,
"Be that as it may"
,
"The bottom line is"
,
"If you will"
,
]
(
cliches
[
3
])
The program prints the fourth cliché:
Be that as it may
A Python list such as cliches
is
a sequence of values,
accessed by their offset from
the beginning of the list.
The first value is at offset 0
,
and the fourth value is at offset 3
.
Note
People count from 1, so it might seem weird to count from 0. It helps to think in terms of offsets instead of positions.
Lists are very common in Python, and Chapter 3 shows how to use them.
Following is another program that also prints a quote, but this time referenced by the person who said it rather than its position in a list:
quotes
=
{
"Moe"
:
"A wise guy, huh?"
,
"Larry"
:
"Ow!"
,
"Curly"
:
"Nyuk nyuk!"
,
}
stooge
=
"Curly"
(
stooge
,
"says:"
,
quotes
[
stooge
])
If you were to run this little program, it would print the following:
Curly says: Nyuk nyuk!
quotes
is a Python dictionary—a
collection of unique keys (in this example, the name of the Stooge)
and associated values (here, a notable quote of that Stooge).
Using a dictionary, you can store and look up things by name,
which is often a useful alternative to a list.
You can read much more about dictionaries in Chapter 3.
The cliché example
uses square brackets ([
and ]
) to make a Python list,
and the stooge example
uses curly brackets ({
and }
, which are no relation to Curly), to make
a Python dictionary.
These are examples of Python’s syntax,
and in the next few chapters, you’ll see much more.
And now for something completely different: Example 1-1 presents a Python program performing a more complex series of tasks. Don’t expect to understand how the program works yet; that’s what this book is for! The intent is to introduce you to the look and feel of a typical nontrivial Python program. If you know other computer languages, evaluate how Python compares.
In earlier printings of this book, this sample program connected to a YouTube website and retrieved information on its most highly rated videos, like “Charlie Bit My Finger.” It worked well until shortly after the ink was dry on the second printing. That’s when Google dropped support for this service and the marquee sample program stopped working. Our new Example 1-1 goes to another site—the Wayback Machine at the Internet Archive, which has saved billions of web pages (and movies, TV shows, music, games, and other digital artifacts) over twenty years.
The program will ask you to type a URL and a date. Then it asks the Wayback Machine if it has a copy of that website around that date. If it found one, it prints the URL and displays it in your web browser. The point is to show how Python handles a variety of tasks—get your typed input, talk across the Internet to a website, get back some content, extract a URL from it, and convince your web browser to display that URL.
If we got back a normal web page full of HTML-formatted text, it would be hard to dig out the information we want (I talk about web scraping in “Crawl and Scrape”). Instead, the program returns data in JSON format, which is meant for processing by computers. JSON, or JavaScript Object Notation, is a human-readable text format that describes the types, values, and order of the values within it. It’s like a little programming language and has become a popular way to exchange data among different computer languages and systems. You read about JSON in “JSON”.
Python programs can translate JSON text into Python data structures—the kind you’ll see in the next two chapters—as though you wrote a program to create them yourself. Our little program just selects one piece (the URL of the old page from the Archive). Again, this is a complete Python program that you can run yourself. We’ve included only a little error-checking, just to keep the example short.
Example 1-1. intro/archive.py
import
webbrowser
import
json
from
urllib.request
import
urlopen
(
"Let's find an old website."
)
site
=
input
(
"Type a website URL: "
)
era
=
input
(
"Type a year, month, and day, like 20150613: "
)
url
=
"http://archive.org/wayback/available?url=
%s
×tamp=
%s
"
%
(
site
,
era
)
response
=
urlopen
(
url
)
contents
=
response
.
read
()
text
=
contents
.
decode
(
"utf-8"
)
data
=
json
.
loads
(
text
)
try
:
old_site
=
data
[
"archived_snapshots"
][
"closest"
][
"url"
]
(
"Found this copy: "
,
old_site
)
(
"It should appear in your browser now."
)
webbrowser
.
open
(
old_site
)
except
:
(
"Sorry, no luck finding"
,
site
)
When I ran this in a terminal window, I typed a site URL and a date, and got this text output:
$ python archive.py Let's find an old website. Type a website URL: lolcats.com Type a year, month, and day, like 20150613: 20151022 Found this copy: http://web.archive.org/web/20151102055938/http://www.lolcats.com/ It should appear in your browser now.
And this appeared in my browser:
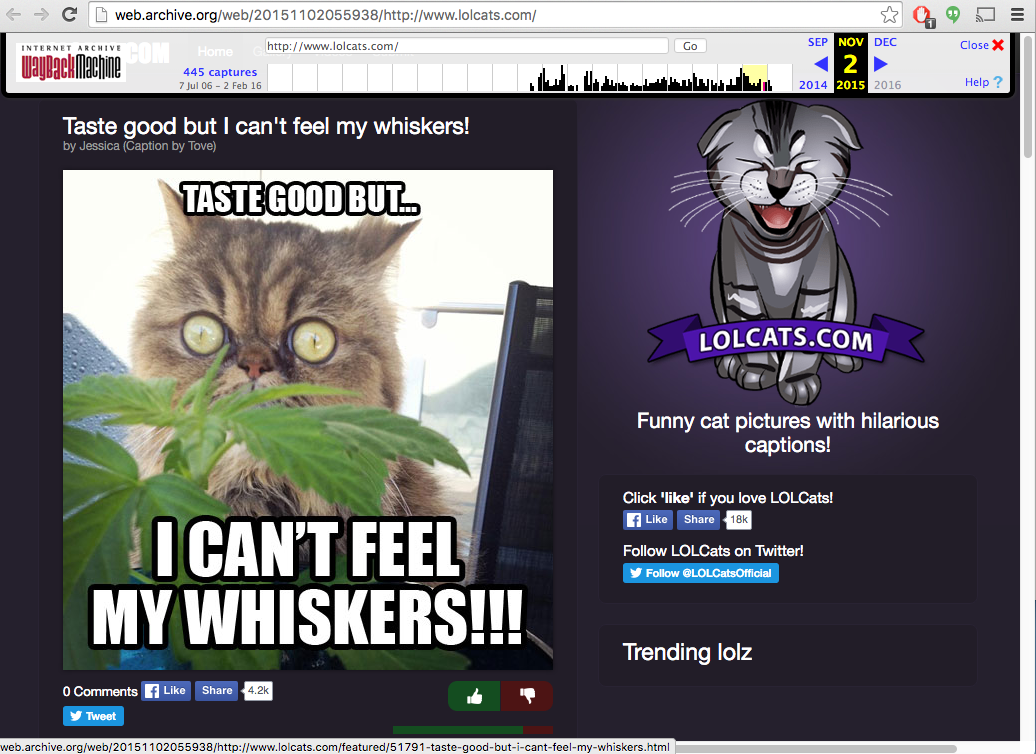
This little Python program did a lot in a few fairly readable lines. You don’t know all these terms, but don’t worry; you will within the next few chapters:
-
Line 1: import (make available to this program) all the code from the Python standard library called
webbrowser
-
Line 2: import all the code from the Python standard library called
json
-
Line 3: import only the
urlopen
function from the standard libraryurllib.request
-
Line 4: a blank line, because we don’t want to feel crowded
-
Line 5: print some initial text to your display
-
Line 6: print a question about a URL, read what you type, and save it in a program variable called
site
-
Line 7: print another question, this time reading a year, month and day, and saving it in a variable called
era
-
Line 8: construct another variable called
url
to make the Wayback Machine look up its copy of the site and date that you typed -
Line 9: connect to the web server at that URL and request a particular web service
-
Line 10: get the response data and assign to the variable
contents
-
Line 11: decode
contents
to a text string in JSON format, and assign to the variabletext
-
Line 12: convert
text
todata
—Python data structures -
Line 13: error-checking:
try
to run the next four lines, and if any fail, run the last line of the program (after theexcept
) -
Line 14: if we got back a match for this site and date, extract its value from a three-level Python dictionary
-
Line 15: print the URL that we found
-
Line 16: print another messsage about what will happen in your browser now
-
Line 17: display the URL we found in your web browser
-
Line 18: if anything failed in the previous four lines, Python jumps down to here
-
Line 19: if it failed, print a message and the site that we were looking for
In the previous example, we used some of Python’s
standard library modules
(programs that are included with Python
when it’s installed),
but there’s nothing sacred about them.
The code that follows shows a rewrite that
uses an external Python software package called requests
:
import
webbrowser
import
requests
(
"Let's find an old website."
)
site
=
input
(
"Type a website URL: "
)
era
=
input
(
"Type a year, month, and day, like 20150613: "
)
url
=
"http://archive.org/wayback/available?url=
%s
×tamp=
%s
"
%
(
site
,
era
)
response
=
requests
.
get
(
url
)
data
=
response
.
json
()
try
:
old_site
=
data
[
"archived_snapshots"
][
"closest"
][
"url"
]
(
"Found this copy: "
,
old_site
)
(
"It should appear in your browser now."
)
webbrowser
.
open
(
old_site
)
except
:
(
"Sorry, no luck finding"
,
site
)
The new version is shorter,
and I’d guess it’s more readable for most people.
I have a lot more to say about requests
and other externally authored
Python software in Chapter 5.
Python in the Real World
So, is learning Python worth the time and effort? Is it a fad or a toy? Actually, it’s been around since 1991 (longer than Java), and is consistently in the top 10 most popular computing languages. People are paid to write Python programs—serious stuff that you use every day, such as Google, YouTube, Dropbox, Netflix, and Hulu. I’ve used it for production applications as varied as an email search appliance and an ecommerce website. Python has a reputation for productivity that appeals to fast-moving organizations.
You’ll find Python in many computing environments, including the following:
-
The command line in a monitor or terminal window
-
Graphical user interfaces, including the Web
-
The Web, on the client and server sides
-
Backend servers supporting large popular sites
-
The cloud (servers managed by third parties)
-
Mobile devices
-
Embedded devices
Python programs range from one-off scripts—such as those you’ve seen so far in this chapter—to million-line systems. We’ll look at its uses in websites, system administration, and data manipulation. We’ll also look at specific uses of Python in the arts, science, and business.
Python versus Language X
How does Python compare against other languages? Where and when would you choose one over the other? In this section, I’ll show code samples from other languages, just so you can see what the competition looks like. You are not expected to understand these if you haven’t worked with them. (By the time you get to the final Python sample, you might be relieved that you haven’t had to work with some of the others.) If you’re only interested in Python, you won’t miss anything if you just skip to the next section.
Each program is supposed to print a number and say a little about the language.
If you use a terminal or terminal window,
the program that reads what you type, runs it, and displays the results
is called the shell program.
The Windows shell is called cmd
; it runs batch files with the suffix .bat
.
Linux and other Unix-like systems
(including Mac OS X)
have many shell programs,
the most popular is called bash or sh
.
The shell has simple abilities,
such as simple logic and expanding wildcard symbols such as
*
into filenames.
You can save commands in files called shell scripts and run them later.
These might be the first programs you encountered as a programmer.
The problem is that shell scripts don’t scale well beyond a few hundred lines,
and they are much slower than the alternative languages.
The next snippet shows a little shell program:
#!/bin/sh language=0 echo "Language $language: I am the shell. So there."
If you saved this in a file as meh.sh
and ran it with sh meh.sh
,
you would see the following on your display:
Language 0: I am the shell. So there.
Old stalwarts C and C++ are fairly low-level languages, used when speed is most important. They’re harder to learn, and require you to keep track of many details, which can lead to crashes and problems that are hard to diagnose. Here’s a look at a little C program:
#include <stdio.h> int main(int argc, char *argv[]) { int language = 1; printf("Language %d: I am C! Behold me and tremble!\n", language); return 0; }
C++ has the C family resemblance but with distinctive features:
#include <iostream> using namespace std; int main() { int language = 2; cout << "Language " << language << \ ": I am C++! Pay no attention to that C behind the curtain!" << \ endl; return(0); }
Java and C# are successors to C and C++ that avoid some of the latters’ problems, but are somewhat verbose and restrictive. The example that follows shows some Java:
public class Overlord { public static void main (String[] args) { int language = 3; System.out.format("Language %d: I am Java! Scarier than C!\n", language); } }
If you haven’t written programs in any of these languages, you might wonder: what is all that stuff? Some languages carry substantial syntactic baggage. They’re sometimes called static languages because they require you to specify some low-level details for the computer. Let me explain.
Languages have variables—names for values that you want to use in a program.
Static languages make you declare the type of each variable:
how much room it will use in memory,
and what you can do with it.
The computer uses this information to compile
the program into very low-level machine language
(specific to the computer’s
hardware and easier for it to understand,
but harder for humans).
Computer language designers often must decide
between making things easier for people
or for computers.
Declaring variable types helps the computer
to catch some mistakes
and run faster,
but it does require more up-front human thinking and typing.
Much of the code that you saw in the C, C++, and Java
examples was required to declare types.
For example, in all of them the int
declaration
was needed to treat the variable language
as an integer.
(Other types include floating-point numbers such as
3.14159
and
character or text data,
which are stored differently.)
Then why are they called static languages? Because variables in those languages can’t ever change their type; they’re static. An integer is an integer, forever and ever.
In contrast, dynamic languages
(also called scripting languages)
do not force you to declare variable types
before using them.
If you type something such as x = 5
,
a dynamic language knows that 5
is an integer; thus, the variable x
is, too.
These languages let you accomplish more with fewer lines of code.
Instead of being compiled,
they are interpreted by a program called—surprise!—an interpreter.
Dynamic languages are often slower than compiled static languages,
but their speed is improving as their interpreters become more optimized.
For a long time, dynamic languages were used mainly for short programs
(scripts),
often to prepare data for processing by longer programs
written in static languages.
Such programs have been called glue code.
Although dynamic languages are well suited for this purpose, today
they are able to tackle most big processing tasks as well.
The all-purpose dynamic language for many years was Perl. Perl is very powerful and has extensive libraries. Yet, its syntax can be awkward, and the language seems to have lost momentum in the last few years to Python and Ruby. This example regales you with a Perl bon mot:
my $language = 4; print "Language $language: I am Perl, the camel of languages.\n";
Ruby is a more recent language. It borrows a little from Perl, and is popular mostly because of Ruby on Rails, a web development framework. It’s used in many of the same areas as Python, and the choice of one or the other might boil down to a matter of taste, or available libraries for your particular application. The code example here depicts a Ruby snippet:
language = 5 puts "Language #{language}: I am Ruby, ready and aglow."
PHP, which you can see in the example that follows, is very popular for web development because it makes it easy to combine HTML and code. However, the PHP language itself has a number of gotchas, and PHP has not caught on as a general language outside of the Web.
<?PHP $language = 6; echo "Language $language: I am PHP. The web is <i>mine</i>, I say.\n"; ?>
The example that follows presents Python’s rebuttal:
language
=
7
(
"Language
%s
: I am Python. What's for supper?"
%
language
)
So, Why Python?
Python is a good general-purpose, high-level language. Its design makes it very readable, which is more important than it sounds. Every computer program is written only once, but read and revised many times, often by many people. Being readable also makes it easier to learn and remember, hence more writeable. Compared with other popular languages, Python has a gentle learning curve that makes you productive sooner, yet it has depths that you can explore as you gain expertise.
Python’s relative terseness makes it possible for you to write a program that’s much smaller than its equivalent in a static language. Studies have shown that programmers tend to produce roughly the same number of lines of code per day—regardless of the language—so, writing half the lines of code doubles your productivity, just like that. Python is the not-so-secret weapon of many companies that think this is important.
Python is the most popular language for introductory computer science courses at the top American colleges. It is also the most popular language for evaluating programming skill by over two thousand employers.
And of course, it’s free, as in beer and speech. Write anything you want with Python, and use it anywhere, freely. No one can read your Python program and say, “That’s a nice little program you have there. It would be too bad if something happened to it.”
Python runs almost everywhere and has “batteries included”—a metric boatload of useful software in its standard library.
But, maybe the best reason to use Python is an unexpected one: people generally like it. They actually enjoy programming with it, rather than treating it as just another tool to get stuff done. Often they’ll say that they miss some feature of Python when they need to work in another language. And that’s what separates Python from most of its peers.
When Not to Use Python
Python isn’t the best language for every situation.
It is not installed everywhere by default. Appendix D shows you how to install Python if you don’t already have it on your computer.
It’s fast enough for most applications, but it might not be fast enough for some of the more demanding ones. If your program spends most of its time calculating things (the technical term is CPU-bound), a program written in C, C++, or Java will generally run faster than its Python equivalent. But not always!
-
Sometimes a better algorithm (a stepwise solution) in Python beats an inefficient one in C. The greater speed of development in Python gives you more time to experiment with alternatives.
-
In many applications, a program twiddles its thumbs while awaiting a response from some server across a network. The CPU (central processing unit, the computer’s chip that does all the calculating) is barely involved; consequently, end-to-end times between static and dynamic programs will be close.
-
The standard Python interpreter is written in C and can be extended with C code. I discuss this a little in “Optimize Your Code”.
-
Python interpreters are becoming faster. Java was terribly slow in its infancy, and a lot of research and money went into speeding it up. Python is not owned by a corporation, so its enhancements have been more gradual. In “PyPy”, I talk about the PyPy project and its implications.
-
You might have an extremely demanding application, and no matter what you do, Python doesn’t meet your needs. Then, as Ian Holm said in the movie Alien, you have my sympathies. The usual alternatives are C, C++, and Java, but a newer language called Go (which feels like Python but performs like C) could be an answer.
Python 2 versus Python 3
The biggest issue that you’ll confront at the moment is that there are two versions of Python out there. Python 2 has been around forever and is preinstalled on Linux and Apple computers. It has been an excellent language, but nothing’s perfect. In computer languages, as in many other areas, some mistakes are cosmetic and easy to fix, whereas others are hard. Hard fixes are incompatible: new programs written with them will not work on the old Python system, and old programs written before the fix will not work on the new system.
Python’s creator (Guido van Rossum) and others decided to bundle the hard fixes together and call it Python 3. Python 2 is the past, and Python 3 is the future. The last version of Python 2 is 2.7, and it will be supported for a long time, but it’s the end of the line; there will be no Python 2.8. New development will be in Python 3.
This book features Python 3.
If you’ve been using Python 2, it’s almost identical.
The most obvious change is how to call print
.
The most important change is the handling of Unicode characters,
which is covered in Chapter 2 and Chapter 7.
Conversion of popular Python software has been gradual,
with the usual chicken-and-egg analogies.
But now, it looks like we’ve finally reached a tipping point.
Installing Python
Rather than cluttering this chapter, the details on how to install Python 3 are in Appendix D. If you don’t have Python 3, or aren’t sure, go there and see what to do for your computer.
Running Python
After you have installed a working copy of Python 3, you can use it to run the Python programs in this book as well as your own Python code. How do you actually run a Python program? There are two main ways:
-
Python’s built-in interactive interpreter (also called its shell) is the easy way to experiment with small programs. You type commands line by line and see the results immediately. With the tight coupling between typing and seeing, you can experiment faster. I’ll use the interactive interpreter to demonstrate language features, and you can type the same commands in your own Python environment.
-
For everything else, store your Python programs in text files, normally with the .py extension, and run them by typing
python
followed by those filenames.
Let’s try both methods now.
Using the Interactive Interpreter
Most of the code examples in this book use the interactive interpreter. When you type the same commands as you see in the examples and get the same results, you’ll know you’re on the right track.
You start the interpreter by typing just the name
of the main Python program on your computer:
it should be
python
, python3
, or something similar.
For the rest of this book,
we’ll assume it’s called python
;
if yours has a different name, type that
wherever you see python
in a code example.
The interactive interpreter works almost exactly the same
as Python works on files, with one exception:
when you type something that has a value,
the interactive interpreter
prints its value for you automatically.
For example, if you start Python
and type the number 61
in the interpreter,
it will be echoed to your terminal.
Note
In the example that follows,
$
is a sample system prompt for
you to type a command like
python
in the
terminal window.
We’ll use it for the code examples in this book,
although your prompt might be different.
$ python Python 3.5.1 (v3.5.1:37a07cee5969, Dec 5 2015, 21:12:44) [GCC 4.2.1 (Apple Inc. build 5666) (dot 3)] on darwin Type "help", "copyright", "credits" or "license" for more information. >>> 61 61 >>>
This automatic printing of a value is a time-saving feature of the interactive interpreter, not a part of the Python language.
By the way,
print()
also works within the interpreter
whenever you want to print something:
>>>
(
61
)
61
If you tried these examples with the interactive interpreter and saw the same results, you just ran some real (though tiny) Python code. In the next few chapters, you’ll graduate from one-liners to longer Python programs.
Use Python Files
If you put 61
in a file by
itself and run it through Python,
it will run,
but it won’t print anything.
In normal noninteractive Python programs,
you need to call the print
function to print things, as is demonstrated in the code that follows:
(
61
)
Let’s make a Python program file and run it:
-
Open your text editor.
-
Type the line
print(61)
, as it appears above. -
Save this to a file called 61.py. Make sure you save it as plain text, rather than a “rich” format such as RTF or Word. You don’t need to use the .py suffix for your Python program files, but it does help you remember what they are.
-
If you’re using a graphical user interface—that’s almost everyone—open a terminal window.1
-
Run your program by typing the following:
$ python 61.py
You should see a single line of output:
61
Did that work? If it did, congratulations on running your first standalone Python program.
What’s Next?
You’ll be typing commands to an actual Python system, and they need to follow legal Python syntax. Rather than dumping the syntax rules on you all at once, we’ll stroll through them over the next few chapters.
The basic way to develop Python programs is by using a plain-text editor and a terminal window. I use plain-text displays in this book, sometimes showing interactive terminal sessions and sometimes pieces of Python files. You should know that there are also many good integrated development environments (IDEs) for Python. These may feature graphical user interfaces with advanced text editing and help displays. You can learn about details for some of these in Chapter 12.
Your Moment of Zen
Each computing language has its own style.
In the preface, I mentioned that there is often a
Pythonic way to express yourself.
Embedded in Python is a bit of free verse
that expresses the Python philosophy succinctly
(as far as I know, Python is the only language to
include such an Easter egg).
Just type import this
into your interactive interpreter and then press the Enter key
whenever you need this moment of Zen:
>>>
import
this
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one--and preferably only one--obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea--let's do more of those!
I’ll bring up examples of these sentiments throughout the book.
Things to Do
This chapter was an introduction to the Python language—what it does, how it looks, and where it fits in the computing world. At the end of each chapter, I’ll suggest some mini-projects to help you remember what you just read and prepare you for what’s to come.
1.1 If you don’t already have Python 3 installed on your computer, do it now. Read Appendix D for the details for your computer system.
1.2 Start the Python 3 interactive interpreter.
Again, details are in Appendix D.
It should print a few lines about itself and then
a single line starting with >>>
. That’s your
prompt to type Python commands.
1.3 Play with the interpreter a little.
Use it like a calculator and type this:
8 * 9
.
Press the Enter key to see the result.
Python should print 72
.
1.4 Type the number 47
and press the Enter key.
Did it print 47
for you on the next line?
1.5 Now, type print(47)
and press Enter.
Did that also print 47
for you on the next line?
1 If you’re not sure what this means, see Appendix D for details for different operating systems.
Get Introducing Python now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.