2.14. Accepting User Text Input with UITextField
Problem
You want to accept text input in your user interface.
Solution
Use the UITextField
class.
Discussion
A text field is very much like a label in that it can display text, but a text field can also accept text entry at runtime. Figure 2-44 shows two text fields in the Twitter section of the Settings app on an iPhone.
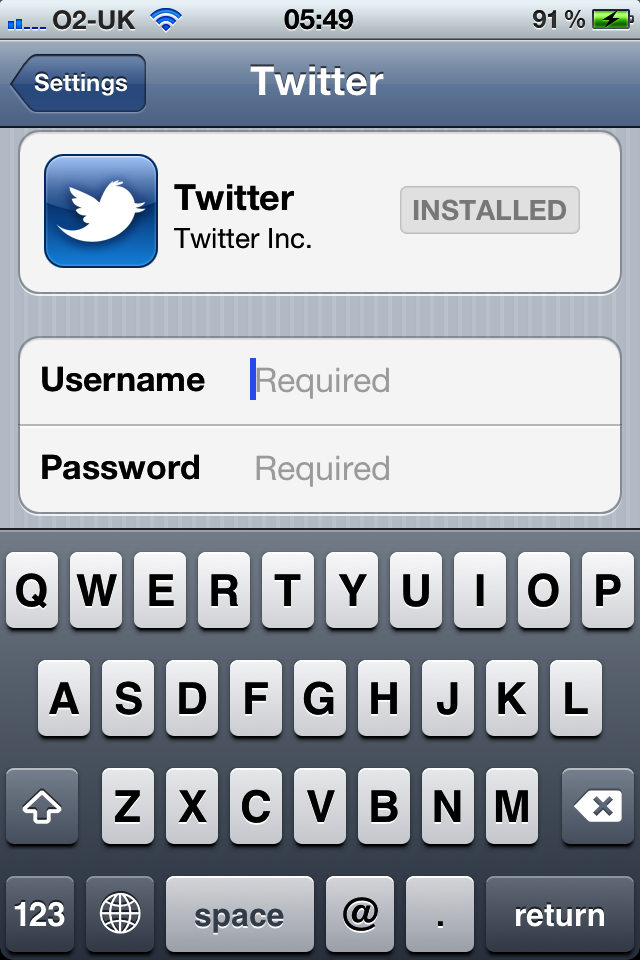
Figure 2-44. Username and Password text fields allowing text entry
Note
A text field allows only a single line of text to be input/displayed. As a result, the default height of a text field is only 31 points. In Interface Builder, this height cannot be modified, but if you are creating your text field in code, you can change the text field’s height. A change in height, though, will not change the number of lines you can render in a text field, which is always 1.
Let’s start with the header file of our view controller to define our text field:
#import <UIKit/UIKit.h> @interface Accepting_User_Text_Input_with_UITextFieldViewController : UIViewController @property (nonatomic, strong) UITextField *myTextField; @end
This is followed by synthesizing the myTextField
property:
#import "Accepting_User_Text_Input_with_UITextFieldViewController.h" @implementation Accepting_User_Text_Input_with_UITextFieldViewController @synthesize myTextField; ...
And then let’s create the text field:
- (void)viewDidLoad{ [super viewDidLoad]; self.view.backgroundColor ...
Get iOS 5 Programming Cookbook now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.