Chapter 9. Intermediate 3D Graphics
Once you’ve got OpenGL drawing basic 3D content, you’ll likely want to create more complicated scenes. In this chapter, you’ll learn how to load meshes from files, how to compose complex objects by creating parent-child relationships between objects, how to position a camera in 3D space, and more.
This chapter builds on the basic 3D graphics concepts covered in Chapter 8, and makes use of the component-based layout shown in Creating a Component-Based Game Layout.
Loading a Mesh
Problem
You want to load meshes from files, so that you can store 3D objects in files.
Solution
First, create an empty text file called MyMesh.json. Put the following text in it:
{
"vertices"
:
[
{
"x"
:-
1
,
"y"
:-
1
,
"z"
:
1
},
{
"x"
:
1
,
"y"
:-
1
,
"z"
:
1
},
{
"x"
:-
1
,
"y"
:
1
,
"z"
:
1
},
{
"x"
:
1
,
"y"
:
1
,
"z"
:
1
},
{
"x"
:-
1
,
"y"
:-
1
,
"z"
:-
1
},
{
"x"
:
1
,
"y"
:-
1
,
"z"
:-
1
},
{
"x"
:-
1
,
"y"
:
1
,
"z"
:-
1
},
{
"x"
:
1
,
"y"
:
1
,
"z"
:-
1
}
],
"triangles"
:
[
[
0
,
1
,
2
],
[
2
,
3
,
1
],
[
4
,
5
,
6
],
[
6
,
7
,
5
]
]
}
Note
JSON is but one of many formats for storing 3D mesh data; many popular 3D modeling tools, such as the open source Blender, support it.
This mesh creates two parallel squares, as shown in Figure 9-1.
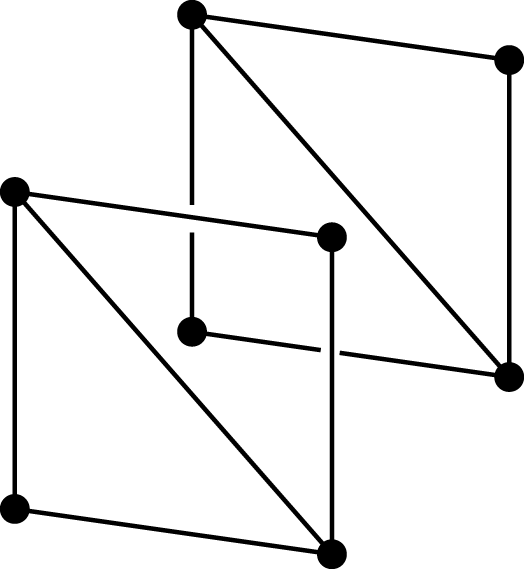
Next, create a new subclass of NSObject
, called Mesh
. Put the following code in Mesh.h:
#import <GLKit/GLKit.h>
typedef
struct
{
GLKVector3
position
;
GLKVector2
textureCoordinates
;
GLKVector3
normal
;
}
Vertex
;
Get iOS Swift Game Development Cookbook, 2nd Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.